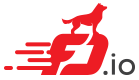 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
16 #ifndef VNET_VNET_URI_TRANSPORT_TYPES_H_
17 #define VNET_VNET_URI_TRANSPORT_TYPES_H_
25 #define TRANSPORT_MAX_HDRS_LEN 140
65 typedef struct _spacer
70 f32 tokens_per_period;
74 #define TRANSPORT_CONN_ID_LEN 44
79 typedef struct _transport_connection
89 ip46_address_t rmt_ip;
90 ip46_address_t lcl_ip;
127 #define c_lcl_ip connection.lcl_ip
128 #define c_rmt_ip connection.rmt_ip
129 #define c_lcl_ip4 connection.lcl_ip.ip4
130 #define c_rmt_ip4 connection.rmt_ip.ip4
131 #define c_lcl_ip6 connection.lcl_ip.ip6
132 #define c_rmt_ip6 connection.rmt_ip.ip6
133 #define c_lcl_port connection.lcl_port
134 #define c_rmt_port connection.rmt_port
135 #define c_proto connection.proto
136 #define c_fib_index connection.fib_index
137 #define c_s_index connection.s_index
138 #define c_c_index connection.c_index
139 #define c_is_ip4 connection.is_ip4
140 #define c_thread_index connection.thread_index
141 #define c_elog_track connection.elog_track
142 #define c_cc_stat_tstamp connection.cc_stat_tstamp
143 #define c_rmt_fei connection.rmt_fei
144 #define c_rmt_dpo connection.rmt_dpo
145 #define c_opaque_id connection.opaque_conn_id
146 #define c_stats connection.stats
147 #define c_pacer connection.pacer
148 #define c_flags connection.flags
149 #define s_ho_handle pacer.bytes_per_sec
158 "moved into 3rd cache line");
160 #define foreach_transport_proto \
161 _ (TCP, "tcp", "T") \
162 _ (UDP, "udp", "U") \
163 _ (NONE, "ct", "C") \
164 _ (TLS, "tls", "J") \
165 _ (QUIC, "quic", "Q") \
166 _ (DTLS, "dtls", "D") \
167 _ (SRTP, "srtp", "R")
169 typedef enum _transport_proto
171 #define _(sym, str, sstr) TRANSPORT_PROTO_ ## sym,
185 #define foreach_transport_endpoint_fields \
186 _(ip46_address_t, ip) \
189 _(u32, sw_if_index) \
194 #define _(type, name) type name;
206 #define foreach_transport_endpoint_cfg_fields \
207 foreach_transport_endpoint_fields \
208 _ (transport_endpoint_t, peer) \
209 _ (u32, next_node_index) \
210 _ (u32, next_node_opaque) \
212 _ (u8, transport_flags) \
217 #define _(type, name) type name;
222 #define foreach_transport_endpt_cfg_flags \
229 #define _(name) TRANSPORT_ENDPT_ATTR_F_BIT_##name,
237 TRANSPORT_ENDPT_ATTR_F_##name = 1 << TRANSPORT_ENDPT_ATTR_F_BIT_##name,
242 #define foreach_transport_attr_fields \
243 _ (u64, next_output_node, NEXT_OUTPUT_NODE) \
245 _ (u8, flags, FLAGS) \
246 _ (u8, cc_algo, CC_ALGO)
250 #define _(type, name, str) TRANSPORT_ENDPT_ATTR_##str,
260 #define _(type, name, str) type name;
292 #define ENDPOINT_INVALID_INDEX ((u32)~0)
transport_endpt_attr_flag_bit_
u8 transport_protocol_is_cl(transport_proto_t tp)
transport_service_type_t transport_protocol_service_type(transport_proto_t)
transport_endpt_attr_type_
transport_endpt_cfg_flags_
#define foreach_transport_proto
#define CLIB_CACHE_LINE_ALIGN_MARK(mark)
u8 * format_transport_proto(u8 *s, va_list *args)
enum transport_service_type_ transport_service_type_t
@ TRANSPORT_ENDPT_EXT_CFG_NONE
u8 * format_transport_proto_short(u8 *s, va_list *args)
struct _transport_connection transport_connection_t
u8 * format_transport_protos(u8 *s, va_list *args)
u8 hostname[256]
full domain len is 255 as per rfc 3986
transport_endpt_ext_cfg_type_
#define foreach_transport_endpoint_fields
fib table endpoint is associated with
enum transport_dequeue_type_ transport_tx_fn_type_t
#define STRUCT_OFFSET_OF(t, f)
@ TRANSPORT_CONNECTION_F_CLESS
Connection is "connection less".
static void elog_track(elog_main_t *em, elog_event_type_t *type, elog_track_t *track, u32 data)
Log a single-datum event to a specific track, non-inline version.
transport_endpt_attr_type_t type
transport_connection_flags_
#define foreach_transport_endpt_cfg_flags
static u8 transport_endpoint_fib_proto(transport_endpoint_t *tep)
struct transport_endpt_crypto_cfg_ transport_endpt_crypto_cfg_t
f64 end
end of the time range
#define foreach_transport_attr_fields
@ TRANSPORT_SERVICE_APP
app transport service
@ TRANSPORT_CONNECTION_F_IS_TX_PACED
@ TRANSPORT_CFG_F_CONNECTED
enum transport_connection_flags_ transport_connection_flags_t
transport_tx_fn_type_t transport_protocol_tx_fn_type(transport_proto_t tp)
u8 * format_transport_listen_connection(u8 *s, va_list *args)
clib_bihash_24_8_t transport_endpoint_table_t
@ TRANSPORT_TX_INTERNAL
apps acting as transports
uword unformat_transport_proto(unformat_input_t *input, va_list *args)
@ TRANSPORT_SERVICE_VC
virtual circuit service
@ TRANSPORT_TX_DGRAM
datagram mode
struct transport_endpt_ext_cfg_ transport_endpt_ext_cfg_t
@ TRANSPORT_CONNECTION_F_NO_LOOKUP
Don't register connection in lookup.
@ TRANSPORT_TX_PEEK
reliable transport protos
struct transport_endpoint_ transport_endpoint_t
u8 * format_transport_half_open_connection(u8 *s, va_list *args)
enum _transport_proto transport_proto_t
transport_endpt_attr_flag_
@ TRANSPORT_CFG_F_UNIDIRECTIONAL
@ TRANSPORT_SERVICE_CL
connectionless service
struct transport_endpoint_pair_ transport_endpoint_cfg_t
enum transport_endpt_attr_type_ transport_endpt_attr_type_t
enum transport_endpt_cfg_flags_ transport_endpt_cfg_flags_t
STATIC_ASSERT(sizeof(transport_connection_t)<=128, "moved into 3rd cache line")
#define foreach_transport_endpoint_cfg_fields
enum transport_endpt_ext_cfg_type_ transport_endpt_ext_cfg_type_t
enum transport_endpt_attr_flag_bit_ transport_endpt_attr_flag_bit_t
enum transport_endpt_attr_flag_ transport_endpt_attr_flag_t
u8 * format_transport_connection(u8 *s, va_list *args)
@ TRANSPORT_TX_DEQUEUE
unreliable transport protos
struct transport_endpt_attr_ transport_endpt_attr_t
@ TRANSPORT_CONNECTION_F_DESCHED
Connection descheduled by the session layer.
transport_endpt_crypto_cfg_t crypto
#define TRANSPORT_CONN_ID_LEN
@ TRANSPORT_ENDPT_EXT_CFG_CRYPTO
vl_api_wireguard_peer_flags_t flags
static u8 transport_connection_fib_proto(transport_connection_t *tc)