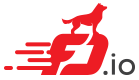 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
16 #ifndef __FIB_ENTRY_H__
17 #define __FIB_ENTRY_H__
92 #define FIB_ENTRY_ATTRIBUTES { \
93 [FIB_ENTRY_ATTRIBUTE_CONNECTED] = "connected", \
94 [FIB_ENTRY_ATTRIBUTE_ATTACHED] = "attached", \
95 [FIB_ENTRY_ATTRIBUTE_IMPORT] = "import", \
96 [FIB_ENTRY_ATTRIBUTE_DROP] = "drop", \
97 [FIB_ENTRY_ATTRIBUTE_EXCLUSIVE] = "exclusive", \
98 [FIB_ENTRY_ATTRIBUTE_LOCAL] = "local", \
99 [FIB_ENTRY_ATTRIBUTE_URPF_EXEMPT] = "uRPF-exempt", \
100 [FIB_ENTRY_ATTRIBUTE_MULTICAST] = "multicast", \
101 [FIB_ENTRY_ATTRIBUTE_NO_ATTACHED_EXPORT] = "no-attached-export", \
102 [FIB_ENTRY_ATTRIBUTE_COVERED_INHERIT] = "covered-inherit", \
103 [FIB_ENTRY_ATTRIBUTE_INTERPOSE] = "interpose", \
106 #define FOR_EACH_FIB_ATTRIBUTE(_item) \
107 for (_item = FIB_ENTRY_ATTRIBUTE_FIRST; \
108 _item <= FIB_ENTRY_ATTRIBUTE_LAST; \
163 #define FIB_ENTRY_SRC_ATTRIBUTES { \
164 [FIB_ENTRY_SRC_ATTRIBUTE_ADDED] = "added", \
165 [FIB_ENTRY_SRC_ATTRIBUTE_CONTRIBUTING] = "contributing", \
166 [FIB_ENTRY_SRC_ATTRIBUTE_ACTIVE] = "active", \
167 [FIB_ENTRY_SRC_ATTRIBUTE_STALE] = "stale", \
168 [FIB_ENTRY_SRC_ATTRIBUTE_INHERITED] = "inherited", \
171 #define FOR_EACH_FIB_SRC_ATTRIBUTE(_item) \
172 for (_item = FIB_ENTRY_SRC_ATTRIBUTE_FIRST; \
173 _item <= FIB_ENTRY_SRC_ATTRIBUTE_LAST; \
192 "FIB entry flags field size too big");
356 #define FOR_EACH_FIB_ENTRY_FLAG(_item) \
357 for (_item = FIB_ENTRY_FLAG_FIRST; _item < FIB_ENTRY_FLAG_MAX; _item++)
359 #define FIB_ENTRY_FORMAT_BRIEF (0x0)
360 #define FIB_ENTRY_FORMAT_DETAIL (0x1)
361 #define FIB_ENTRY_FORMAT_DETAIL2 (0x2)
void fib_entry_mark(fib_node_index_t fib_entry_index, fib_source_t source)
union fib_entry_src_t_::@292 u
Source specific info.
void fib_entry_child_remove(fib_node_index_t fib_entry_index, u32 sibling_index)
void fib_entry_module_init(void)
u8 * format_fib_entry_src_flags(u8 *s, va_list *args)
u32 fesa_sibling
This source's index in the cover's list.
int fib_entry_cmp_for_sort(void *i1, void *i2)
void fib_entry_path_add(fib_node_index_t fib_entry_index, fib_source_t source, fib_entry_flag_t flags, const fib_route_path_t *rpaths)
struct fib_entry_src_t_::@292::@296 interface
void fib_entry_cover_updated(fib_node_index_t fib_entry)
enum fib_entry_src_attribute_t_ fib_entry_src_attribute_t
Flags for the source data.
fib_source_t fib_entry_get_best_source(fib_node_index_t fib_entry_index)
void fib_entry_special_add(fib_node_index_t fib_entry_index, fib_source_t source, fib_entry_flag_t flags, const dpo_id_t *dpo)
@ FIB_ENTRY_ATTRIBUTE_LOCAL
The prefix/address is local to this device.
@ FIB_ENTRY_ATTRIBUTE_URPF_EXEMPT
The prefix/address exempted from loose uRPF check To be used with caution.
enum fib_entry_attribute_t_ fib_entry_attribute_t
The different sources that can create a route.
@ FIB_ENTRY_SRC_FLAG_ACTIVE
@ FIB_ENTRY_SRC_FLAG_STALE
@ FIB_ENTRY_FLAG_ATTACHED
u32 fib_entry_pool_size(void)
@ FIB_ENTRY_ATTRIBUTE_COVERED_INHERIT
This FIB entry imposes its source information on all prefixes that is covers.
Information related to the source of a FIB entry.
fib_node_index_t fe_parent
the path-list for which this entry is a child.
int fib_entry_is_host(fib_node_index_t fib_entry_index)
Return !0 is the entry represents a host prefix.
@ FIB_ENTRY_SRC_ATTRIBUTE_LAST
Marker.
void fib_entry_set_flow_hash_config(fib_node_index_t fib_entry_index, flow_hash_config_t hash_config)
@ FIB_ENTRY_FLAG_EXCLUSIVE
struct fib_entry_src_t_::@292::@294 interpose
@ FIB_ENTRY_FLAG_INTERPOSE
enum fib_node_type_t_ fib_node_type_t
The types of nodes in a FIB graph.
@ FIB_ENTRY_ATTRIBUTE_LAST
Marker.
u32 fib_entry_child_add(fib_node_index_t fib_entry_index, fib_node_type_t type, fib_node_index_t child_index)
@ FIB_ENTRY_SRC_FLAG_INHERITED
fib_path_ext_list_t fes_path_exts
A vector of path extensions.
dpo_id_t fesi_dpo
DPO type to interpose.
@ FIB_ENTRY_ATTRIBUTE_CONNECTED
Connected.
@ FIB_ENTRY_FLAG_LOOSE_URPF_EXEMPT
fib_node_index_t fesm_lfes[2]
the indicies of the LFIB entries created
u32 fe_sibling
index of this entry in the parent's child list.
STATIC_ASSERT(sizeof(fib_entry_src_flag_t)<=2, "FIB entry flags field size too big")
enum fib_entry_flag_t_ fib_entry_flag_t
@ FIB_ENTRY_FLAG_MULTICAST
fib_node_index_t fib_entry_get_path_list(fib_node_index_t fib_entry_index)
u32 fe_fib_index
The index of the FIB table this entry is in.
@ FIB_ENTRY_ATTRIBUTE_IMPORT
The route is attached cross tables and thus imports covered prefixes from the other table.
fib_entry_src_t * fe_srcs
Vector of source infos.
enum fib_forward_chain_type_t_ fib_forward_chain_type_t
FIB output chain type.
void fib_entry_inherit(fib_node_index_t cover, fib_node_index_t covered)
fib_entry_inherit
adj_index_t fib_entry_get_adj(fib_node_index_t fib_entry_index)
const int fib_entry_get_dpo_for_source(fib_node_index_t fib_entry_index, fib_source_t source, dpo_id_t *dpo)
fib_node_index_t fesa_cover
the index of the FIB entry that is the covering entry
@ FIB_ENTRY_SRC_FLAG_NONE
void fib_entry_unlock(fib_node_index_t fib_entry_index)
enum flow_hash_config_t_ flow_hash_config_t
A flow hash configuration is a mask of the flow hash options.
int fib_entry_is_marked(fib_node_index_t fib_entry_index, fib_source_t source)
A list of path-extensions.
u32 index_t
A Data-Path Object is an object that represents actions that are applied to packets are they are swit...
u8 * format_fib_source(u8 *s, va_list *args)
u32 fib_node_index_t
A typedef of a node index.
u8 fes_ref_count
1 bytes ref count.
void fib_entry_update(fib_node_index_t fib_entry_index, fib_source_t source, fib_entry_flag_t flags, const fib_route_path_t *paths)
fib_entry_update
index_t * fe_delegates
A vector of delegate indices.
void fib_entry_contribute_forwarding(fib_node_index_t fib_entry_index, fib_forward_chain_type_t type, dpo_id_t *dpo)
fib_node_index_t fib_entry_create_special(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source, fib_entry_flag_t flags, const dpo_id_t *dpo)
fib_node_index_t fesl_fib_index
The source FIB index.
const void * fib_entry_get_source_data(fib_node_index_t fib_entry_index, fib_source_t source)
enum fib_entry_src_flag_t_ fib_entry_src_flag_t
struct fib_entry_src_t_::@292::@297 mpls
u32 fib_entry_get_resolving_interface(fib_node_index_t fib_entry_index)
fib_entry_flag_t fib_entry_get_flags_for_source(fib_node_index_t fib_entry_index, fib_source_t source)
void fib_entry_cover_changed(fib_node_index_t fib_entry)
@ FIB_ENTRY_SRC_ATTRIBUTE_STALE
the source is stale
u32 fesi_sibling
This source's index in the cover's list.
fib_route_path_t * fib_entry_encode(fib_node_index_t fib_entry_index)
@ FIB_ENTRY_ATTRIBUTE_NO_ATTACHED_EXPORT
The prefix/address exempted from attached export.
@ FIB_ENTRY_SRC_ATTRIBUTE_INHERITED
the source is inherited from its cover
struct fib_entry_src_t_::@292::@298 lisp
@ FIB_ENTRY_ATTRIBUTE_FIRST
Marker.
fib_node_index_t fib_entry_create(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source, fib_entry_flag_t flags, const fib_route_path_t *paths)
u32 fib_entry_get_stats_index(fib_node_index_t fib_entry_index)
fib_node_index_t fib_entry_get_index(const fib_entry_t *fib_entry)
fib_entry_attribute_t_
The different sources that can create a route.
struct fib_entry_src_t_::@292::@293 rr
void fib_entry_lock(fib_node_index_t fib_entry_index)
const dpo_id_t * fib_entry_contribute_ip_forwarding(fib_node_index_t fib_entry_index)
@ FIB_ENTRY_SRC_ATTRIBUTE_CONTRIBUTING
the source is contributing forwarding
mpls_label_t fesm_label
This MPLS local label associated with the prefix.
@ FIB_ENTRY_FLAG_COVERED_INHERIT
@ FIB_ENTRY_SRC_ATTRIBUTE_ADDED
the source has been added to the entry
@ FIB_ENTRY_FLAG_CONNECTED
int fib_entry_recursive_loop_detect(fib_node_index_t entry_index, fib_node_index_t **entry_indicies)
int fib_entry_is_sourced(fib_node_index_t fib_entry_index, fib_source_t source)
dpo_id_t fe_lb
The load-balance used for forwarding.
fib_entry_flag_t fib_entry_get_flags(fib_node_index_t fib_entry_index)
@ FIB_ENTRY_ATTRIBUTE_ATTACHED
Attached.
u32 fesr_sibling
This source's index in the cover's list.
fib_source_t fes_src
Which source this info block is for.
u32 fib_entry_get_fib_index(fib_node_index_t fib_entry_index)
A representation of a path as described by a route producer.
void fib_entry_recalculate_forwarding(fib_node_index_t fib_entry_index)
fib_node_t fe_node
Base class.
int fib_entry_is_resolved(fib_node_index_t fib_entry_index)
Return !0 is the entry is resolved, i.e.
fib_node_index_t fesi_cover
the index of the FIB entry that is the covering entry
fib_node_index_t fesr_cover
the index of the FIB entry that is the covering entry
struct fib_entry_src_t_::@292::@295 adj
@ FIB_ENTRY_ATTRIBUTE_EXCLUSIVE
The route is exclusive.
An node in the FIB graph.
u32 adj_index_t
An index for adjacencies.
void fib_entry_contribute_urpf(fib_node_index_t path_index, index_t urpf)
Contribute the set of Adjacencies that this entry forwards with to build the uRPF list of its childre...
adj_index_t fib_entry_get_adj_for_source(fib_node_index_t fib_entry_index, fib_source_t source)
@ FIB_ENTRY_SRC_ATTRIBUTE_FIRST
Marker.
const fib_prefix_t fe_prefix
The prefix of the route.
fib_entry_flag_t fes_entry_flags
Flags the source contributes to the entry.
void fib_entry_special_update(fib_node_index_t fib_entry_index, fib_source_t source, fib_entry_flag_t flags, const dpo_id_t *dpo)
fib_entry_src_attribute_t_
Flags for the source data.
fib_entry_src_flag_t fes_flags
Flags on the source.
u8 * format_fib_entry_flags(u8 *s, va_list *args)
@ FIB_ENTRY_ATTRIBUTE_DROP
The route is an explicit drop.
The identity of a DPO is a combination of its type and its instance number/index of objects of that t...
enum fib_source_t_ fib_source_t
The different sources that can create a route.
u32 mpls_label_t
A label value only, i.e.
u8 * format_fib_entry(u8 *s, va_list *args)
fib_entry_src_flag_t fib_entry_delete(fib_node_index_t fib_entry_index, fib_source_t source)
fib_entry_delete
struct fib_entry_t_ fib_entry_t
An entry in a FIB table.
@ FIB_ENTRY_SRC_FLAG_ADDED
fib_entry_t * fib_entry_get(fib_node_index_t fib_entry_index)
struct fib_entry_src_t_ fib_entry_src_t
Information related to the source of a FIB entry.
@ FIB_ENTRY_ATTRIBUTE_INTERPOSE
The interpose attribute.
fib_entry_src_flag_t fib_entry_path_remove(fib_node_index_t fib_entry_index, fib_source_t source, const fib_route_path_t *rpaths)
fib_node_index_t fes_pl
The path-list created by the source.
@ FIB_ENTRY_ATTRIBUTE_MULTICAST
The prefix/address is a multicast prefix.
const fib_prefix_t * fib_entry_get_prefix(fib_node_index_t fib_entry_index)
u32 fib_entry_get_resolving_interface_for_source(fib_node_index_t fib_entry_index, fib_source_t source)
Aggregate type for a prefix.
vl_api_fib_path_type_t type
@ FIB_ENTRY_SRC_FLAG_CONTRIBUTING
void fib_entry_set_source_data(fib_node_index_t fib_entry_index, fib_source_t source, const void *data)
@ FIB_ENTRY_SRC_ATTRIBUTE_ACTIVE
the source is active/best
u32 fib_entry_get_any_resolving_interface(fib_node_index_t fib_entry_index)
fib_entry_src_flag_t fib_entry_special_remove(fib_node_index_t fib_entry_index, fib_source_t source)
vl_api_wireguard_peer_flags_t flags
@ FIB_ENTRY_FLAG_NO_ATTACHED_EXPORT