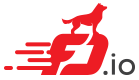 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
23 static vl_api_mfib_itf_flags_t
54 vl_api_mfib_path_t *out)
65 in = clib_net_to_host_u32(in);
85 in = clib_net_to_host_u32(in);
117 return VNET_API_ERROR_NO_SUCH_FIB;
@ MFIB_API_ITF_FLAG_FORWARD
u32 frp_mitf_flags
MFIB interface flags.
mfib_entry_flags_t mfib_api_path_entry_flags_decode(vl_api_mfib_entry_flags_t in)
int mfib_api_path_decode(vl_api_mfib_path_t *in, fib_route_path_t *out)
@ MFIB_API_ENTRY_FLAG_ACCEPT_ALL_ITF
enum mfib_entry_flags_t_ mfib_entry_flags_t
static void mfib_api_path_itf_flags_decode(vl_api_mfib_itf_flags_t in, mfib_itf_flags_t *out)
@ MFIB_API_ITF_FLAG_SIGNAL_PRESENT
void fib_api_path_encode(const fib_route_path_t *rpath, vl_api_fib_path_t *out)
Encode and decode functions from the API types to internal types.
int mfib_api_table_id_decode(fib_protocol_t fproto, u32 table_id, u32 *fib_index)
enum mfib_itf_flags_t_ mfib_itf_flags_t
enum fib_protocol_t_ fib_protocol_t
Protocol Type.
@ MFIB_API_ITF_FLAG_ACCEPT
@ MFIB_ENTRY_FLAG_ACCEPT_ALL_ITF
void mfib_api_path_encode(const fib_route_path_t *in, vl_api_mfib_path_t *out)
Encode and decode functions from the API types to internal types.
@ MFIB_ITF_FLAG_DONT_PRESERVE
@ MFIB_API_ENTRY_FLAG_CONNECTED
u32 mfib_table_find(fib_protocol_t proto, u32 table_id)
Get the index of the FIB for a Table-ID.
@ MFIB_API_ENTRY_FLAG_DROP
A representation of a path as described by a route producer.
@ MFIB_ITF_FLAG_SIGNAL_PRESENT
@ MFIB_API_ITF_FLAG_NEGATE_SIGNAL
@ MFIB_API_ENTRY_FLAG_SIGNAL
@ MFIB_API_ITF_FLAG_DONT_PRESERVE
#define INDEX_INVALID
Invalid index - used when no index is known blazoned capitals INVALID speak volumes where ~0 does not...
int fib_api_path_decode(vl_api_fib_path_t *in, fib_route_path_t *out)
static vl_api_mfib_itf_flags_t mfib_api_path_itf_flags_encode(mfib_itf_flags_t flags)
@ MFIB_ENTRY_FLAG_CONNECTED
@ MFIB_ITF_FLAG_NEGATE_SIGNAL
vl_api_wireguard_peer_flags_t flags