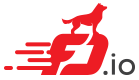 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
35 [IP_PROTOCOL_ICMP] = 16, [IP_PROTOCOL_IGMP] = 8,
36 [IP_PROTOCOL_TCP] = 32, [IP_PROTOCOL_UDP] = 32,
37 [IP_PROTOCOL_IPSEC_ESP] = 32, [IP_PROTOCOL_IPSEC_AH] = 32,
38 [IP_PROTOCOL_ICMP6] = 16,
70 if ((((
u8 *) p)[0] & 0xf0) == 0x40)
72 else if ((((
u8 *) p)[0] & 0xf0) == 0x60)
95 hash[0] = clib_crc32c (
key[0].as_u8,
sizeof (
key[0]));
96 hash[1] = clib_crc32c (
key[1].as_u8,
sizeof (
key[1]));
97 hash[2] = clib_crc32c (
key[2].as_u8,
sizeof (
key[2]));
98 hash[3] = clib_crc32c (
key[3].as_u8,
sizeof (
key[3]));
111 hash[0] = clib_crc32c (
key.as_u8, sizeof (
key));
122 u16 ethertype = 0, l2hdr_sz = 0;
125 ethertype = clib_net_to_host_u16 (eh->
type);
132 ethertype = clib_net_to_host_u16 (vlan->
type);
133 l2hdr_sz +=
sizeof (*vlan);
137 ethertype = clib_net_to_host_u16 (vlan->
type);
138 l2hdr_sz +=
sizeof (*vlan);
142 if (ethertype == ETHERNET_TYPE_IP4)
147 else if (ethertype == ETHERNET_TYPE_IP6)
173 hash[0] = clib_crc32c (
key[0].as_u8,
sizeof (
key[0]));
174 hash[1] = clib_crc32c (
key[1].as_u8,
sizeof (
key[1]));
175 hash[2] = clib_crc32c (
key[2].as_u8,
sizeof (
key[2]));
176 hash[3] = clib_crc32c (
key[3].as_u8,
sizeof (
key[3]));
189 hash[0] = clib_crc32c (
key.as_u8, sizeof (
key));
198 .name =
"crc32c-5tuple",
199 .description =
"IPv4/IPv6 header and TCP/UDP ports",
static uword pow2_mask(uword x)
static_always_inline int ethernet_frame_is_tagged(u16 type)
ip46_address_t src_address
void vnet_crc32c_5tuple_ip_func(void **p, u32 *hash, u32 n_packets)
ip46_address_t dst_address
static void * ip6_next_header(ip6_header_t *i)
static_always_inline void * clib_memcpy_fast(void *restrict dst, const void *restrict src, size_t n)
static_always_inline void compute_ethernet_key(void *p, crc32c_5tuple_key_t *key)
#define static_always_inline
static const u8 l4_mask_bits[256]
static_always_inline void compute_ip6_key(ip6_header_t *ip, crc32c_5tuple_key_t *k)
VNET_REGISTER_HASH_FUNCTION(crc32c_5tuple, static)
static_always_inline void compute_ip_key(void *p, crc32c_5tuple_key_t *key)
void vnet_crc32c_5tuple_ethernet_func(void **p, u32 *hash, u32 n_packets)
static_always_inline void clib_prefetch_load(void *p)
static_always_inline void compute_ip4_key(ip4_header_t *ip, crc32c_5tuple_key_t *k)
static void * ip4_next_header(ip4_header_t *i)