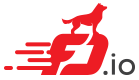 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
38 #ifndef included_clib_vec_bootstrap_h
39 #define included_clib_vec_bootstrap_h
63 #define VEC_NUMA_UNSPECIFIED (0xFF)
73 #define _vec_find(v) ((vec_header_t *) (v) - 1)
75 #define _vec_round_size(s) \
76 (((s) + sizeof (uword) - 1) &~ (sizeof (uword) - 1))
134 #define _vec_len(v) (_vec_find(v)->len)
142 #define vec_len(v) ((v) ? _vec_len(v) : 0)
151 #define _vec_numa(v) (_vec_find(v)->numa_id)
156 #define vec_numa(v) ((v) ? _vec_numa(v) : 0)
161 #define vec_bytes(v) (vec_len (v) * sizeof (v[0]))
165 #define vec_capacity(v,b) \
167 void * _vec_capacity_v = (void *) (v); \
168 uword _vec_capacity_b = (b); \
169 _vec_capacity_b = sizeof (vec_header_t) + _vec_round_size (_vec_capacity_b); \
170 _vec_capacity_v ? clib_mem_size (_vec_capacity_v - _vec_capacity_b) : 0; \
174 #define vec_max_len(v) \
175 ((v) ? (vec_capacity (v,0) - vec_header_bytes (0)) / sizeof (v[0]) : 0)
179 #define vec_set_len(v, l) do { \
181 ASSERT((l) <= vec_max_len(v)); \
182 CLIB_MEM_POISON_LEN((void *)(v), _vec_len(v) * sizeof((v)[0]), (l) * sizeof((v)[0])); \
186 #define vec_set_len(v, l) do { \
194 #define vec_reset_length(v) do { if (v) vec_set_len (v, 0); } while (0)
197 #define vec_end(v) ((v) + vec_len (v))
200 #define vec_is_member(v,e) ((e) >= (v) && (e) < vec_end (v))
203 #define vec_elt_at_index(v,i) \
205 ASSERT ((i) < vec_len (v)); \
210 #define vec_elt(v,i) (vec_elt_at_index(v,i))[0]
213 #define vec_foreach(var,vec) for (var = (vec); var < vec_end (vec); var++)
216 #define vec_foreach_backwards(var,vec) \
217 for (var = vec_end (vec) - 1; var >= (vec); var--)
220 #define vec_foreach_index(var,v) for ((var) = 0; (var) < vec_len (v); (var)++)
223 #define vec_foreach_index_backwards(var,v) \
224 for ((var) = vec_len((v)) - 1; (var) >= 0; (var)--)
static void * vec_aligned_header(void *v, uword header_bytes, uword align)
static uword vec_aligned_header_bytes(uword header_bytes, uword align)
static uword round_pow2(uword x, uword pow2)
static void * vec_header_end(void *v, uword header_bytes)
Find the end of user vector header.
static void * vec_header(void *v, uword header_bytes)
Find a user vector header.
u32 vec_len_not_inline(void *v)
static uword vec_get_numa(void *v)
return the NUMA index for a vector
static void * vec_aligned_header_end(void *v, uword header_bytes, uword align)
static uword vec_header_bytes(uword header_bytes)