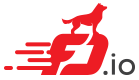 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
16 #ifndef __VOM_INTERFACE_H__
17 #define __VOM_INTERFACE_H__
33 namespace interface_cmds {
235 std::shared_ptr<interface>
singular()
const;
308 template <
typename MSG>
347 int sw_if_index = reply.get_response().get_payload().sw_if_index;
348 int retval = reply.get_response().get_payload().retval;
375 template <
typename MSG>
501 static std::shared_ptr<interface>
find(
const key_t& k);
506 static void dump(std::ostream& os);
545 virtual std::shared_ptr<interface>
singular_i()
const;
556 virtual std::queue<cmd*>&
mk_create_cmd(std::queue<cmd*>& cmds);
562 virtual std::queue<cmd*>&
mk_delete_cmd(std::queue<cmd*>& cmds);
567 virtual void sweep(
void);
602 void handle_replay();
607 void show(std::ostream& os);
625 void publish_stats();
635 void enable_stats_i(stat_listener* el,
const stats_type_t& st);
640 void disable_stats_i();
677 std::shared_ptr<route_domain> m_rd;
722 static std::map<handle_t, std::weak_ptr<interface>> m_hdl_db;
727 virtual void replay(
void);
733 template <
typename MSG>
740 template <
typename MSG>
743 static std::shared_ptr<interface_cmds::events_cmd> m_events_cmd;
const stats_t & get_stats(void) const
Get the interface stats.
const l2_address_t & l2_address() const
Return the L2 Address.
static const_iterator_t cend()
const static type_t BVI
A brideged Virtual interface (aka SVI or IRB)
static void remove(const HW::item< handle_t > &item)
remove an interface from the DB keyed on handle
static oper_state_t from_int(uint8_t val)
Convert VPP's numerical value to enum type.
delete_cmd(HW::item< handle_t > &item)
void enable_stats(stat_listener *el, const stats_type_t &st=stats_type_t::NORMAL)
Enable stats for this interface.
The VPP Object Model (VOM) library.
dependency_t
There needs to be a strict order in which object types are read from VPP (at boot time) and replayed ...
const static rc_t OK
The HW write was successfull.
static const rc_t & from_vpp_retval(int32_t rv)
Get the rc_t from the VPP API value.
The interface to writing objects into VPP OM.
static singular_db< key_t, interface > m_db
A map of all interfaces key against the interface's name.
HW::item< handle_t > m_hdl
The SW interface handle VPP has asigned to the interface.
inspect command handler Handler
const static type_t VXLAN
VXLAN interface.
A base class for all RPC commands to VPP.
static void dump(std::ostream &os)
Dump all interfaces into the stream provided.
singular_db< const std::string, interface >::const_iterator const_iterator_t
The iterator type.
static void add(const key_t &name, const HW::item< handle_t > &item)
Add an interface to the DB keyed on handle.
virtual bool operator==(const interface &i) const
Comparison operator - only used for UT.
A class that listens to interface Events.
vl_api_dhcp_client_state_t state
vl_api_ikev2_sa_stats_t stats
static void enable_events(interface::event_listener &el)
Enable the reception of events of all interfaces.
Class definition for listeners to OM events.
Base class for intterface Delete commands.
std::map< KEY, std::weak_ptr< OBJ > >::const_iterator const_iterator
Iterator.
const std::string m_name
The name of the interface to be created.
static type_t from_string(const std::string &str)
Convert VPP's name of the interface to a type.
const std::string & name() const
Return the interface type.
delete_cmd(HW::item< handle_t > &item, const std::string &name)
HW::item< handle_t > & m_hw_item
A reference to an object's HW::item that the command will update.
virtual bool operator==(const create_cmd &o) const
Comparison operator - only used for UT.
const static type_t LOCAL
Local interface type (specific to VPP)
static void disable_events()
disable the reception of events of all interfaces
HW::item< bool > & status()
Return the HW::item representing the status.
const type_t & type() const
Return the interface type.
void insert_interface()
add the created interface to the DB
virtual std::string to_string(void) const
convert to string format for debug purposes
const static type_t LOOPBACK
loopback interface type
virtual ~interface()
Destructor.
const static type_t BOND
bond interface type
virtual std::string to_string() const =0
convert to string format for debug purposes
A command class represents our desire to recieve interface events.
void disable_stats()
Disable stats for this interface.
HW::item< bool > m_status
The status of the subscription.
const static type_t PIPE
pipe-parent type
event_listener()
Default Constructor.
const static stats_type_t DETAILED
const static type_t ETHERNET
Ethernet interface type.
virtual void handle_interface_stat(const interface &)=0
Virtual function called on the listener when the command has data ready to process.
void succeeded()
Indicate the succeeded, when the HW Q is disabled.
const key_t & key() const
Return the interface type.
const admin_state_t & admin_state() const
Return the admin state.
virtual bool operator==(const delete_cmd &o) const
Comparison operator - only used for UT.
virtual void sweep(void)
Sweep/reap the object if still stale.
A template base class for all enum types.
const static oper_state_t UP
Operational UP state.
A representation of an interface in VPP.
const static type_t AFPACKET
AF-Packet interface type.
const typedef std::string key_t
In the opflex world each entity is known by a URI which can be converted into a string.
const static type_t VHOST
vhost-user interface type
void set(const admin_state_t &state)
Set the admin state of the interface.
std::shared_ptr< interface > singular() const
Return the matching'singular' of the interface.
A type declaration of an interface handle in VPP.
const static log_level_t DEBUG
A base class for interface Create commands.
std::ostream & operator<<(std::ostream &os, const std::pair< direction_t, interface::key_t > &key)
static admin_state_t from_int(uint8_t val)
Convert VPP's numerical value to enum type.
A class that listens to interface Stats.
interface(const std::string &name, type_t type, admin_state_t state, const std::string &tag="")
Construct a new object matching the desried state.
const handle_t & handle() const
Return VPP's handle to this object.
const static type_t TAPV2
TAPv2 interface type.
Type def of a L2 address as read from VPP.
HW::item< handle_t > & item()
return the HW item the command updates
const static admin_state_t DOWN
Admin DOWN state.
void remove_interface()
remove the deleted interface from the DB
HW::item< bool > & status()
Return the HW::item representing the status.
create_cmd(HW::item< handle_t > &item, const std::string &name)
virtual std::queue< cmd * > & mk_delete_cmd(std::queue< cmd * > &cmds)
Virtual functions to construct an interface delete commands.
virtual void handle_interface_event(std::vector< event > es)=0
Virtual function called on the listener when the command has data ready to process.
HW::item< bool > m_status
The status of the subscription.
virtual ~delete_cmd()=default
Destructor.
The oper state of the interface.
void succeeded()
Indicate the succeeded, when the HW Q is disabled.
virtual vapi_error_e operator()(MSG &reply)
call operator used as a callback by VAPI when the reply is available
virtual std::shared_ptr< interface > singular_i() const
Return the matching 'singular' of the interface.
interface::oper_state_t state
The admin state of the interface.
static const_iterator_t cbegin()
const static type_t UNKNOWN
Unknown type.
stat_listener()
Default Constructor.
const static handle_t INVALID
A value of an interface handle_t that means the itf does not exist.
A Database to store the unique 'singular' instances of a single object type.
event(const interface &itf, const interface::oper_state_t &state)
const std::string & m_name
The name of the interface to be created.
Error codes that VPP will return during a HW write.
std::string key_t
The key for interface's key.
virtual ~create_cmd()=default
Destructor.
void fulfill(const HW::item< handle_t > &d)
Fulfill the commands promise.
void release()
release/remove an interface form the singular store
void event_handler(void *tls_async)
const static stats_type_t NORMAL
A base class for all object_base in the VPP object_base-Model.
vl_api_interface_index_t sw_if_index
Stat reader: single interface to get stats.
const static type_t PIPE_END
pipe-end type
const static admin_state_t UP
Admin UP state.
virtual ~stat_listener()=default
virtual std::queue< cmd * > & mk_create_cmd(std::queue< cmd * > &cmds)
Virtual functions to construct an interface create commands.
static std::shared_ptr< interface > find(const handle_t &h)
The the singular instance of the interface in the DB by handle.
const static oper_state_t DOWN
Operational DOWN state.