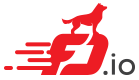 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
24 #include <vnet/feature/feature.api_enum.h>
25 #include <vnet/feature/feature.api_types.h>
27 #define REPLY_MSG_ID_BASE msg_id_base
35 vl_api_feature_enable_disable_reply_t *rmp;
48 (
const char *) feature_name);
50 rv = VNET_API_ERROR_INVALID_VALUE;
56 if (reg->enable_disable_cb)
60 (
const char *) feature_name,
65 rv = VNET_API_ERROR_CANNOT_ENABLE_DISABLE_FEATURE;
77 #include <vnet/feature/feature.api.c>
VLIB_API_INIT_FUNCTION(feature_api_hookup)
#define VALIDATE_SW_IF_INDEX(mp)
vnet_feature_registration_t * vnet_get_feature_reg(const char *arc_name, const char *node_name)
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
struct _vnet_feature_registration vnet_feature_registration_t
feature registration object
#define clib_error_report(e)
static void setup_message_id_table(api_main_t *am)
vl_api_interface_index_t sw_if_index
#define BAD_SW_IF_INDEX_LABEL
static void vl_api_feature_enable_disable_t_handler(vl_api_feature_enable_disable_t *mp)
#define vec_free(V)
Free vector's memory (no header).
description fragment has unexpected format
Feature path enable/disable request.
int vnet_feature_enable_disable(const char *arc_name, const char *node_name, u32 sw_if_index, int enable_disable, void *feature_config, u32 n_feature_config_bytes)
#define vec_terminate_c_string(V)
(If necessary) NULL terminate a vector containing a c-string.
vl_api_interface_index_t sw_if_index
static clib_error_t * feature_api_hookup(vlib_main_t *vm)