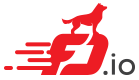 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
16 #ifndef __included_vpp_echo_common_h__
17 #define __included_vpp_echo_common_h__
21 #include <vnet/session/session.api_enum.h>
22 #include <vnet/session/session.api_types.h>
25 #define LOGGING_BATCH (100)
26 #define LOG_EVERY_N_IDLE_CYCLES (1e8)
27 #define ECHO_MQ_SEG_HANDLE ((u64) ~0 - 1)
29 #define foreach_echo_fail_code \
30 _(ECHO_FAIL_NONE, "ECHO_FAIL_NONE") \
31 _(ECHO_FAIL_USAGE, "ECHO_FAIL_USAGE") \
32 _(ECHO_FAIL_SEND_IO_EVT, "ECHO_FAIL_SEND_IO_EVT") \
33 _(ECHO_FAIL_SOCKET_CONNECT, "ECHO_FAIL_SOCKET_CONNECT") \
34 _(ECHO_FAIL_INIT_SHM_API, "ECHO_FAIL_INIT_SHM_API") \
35 _(ECHO_FAIL_SHMEM_CONNECT, "ECHO_FAIL_SHMEM_CONNECT") \
36 _(ECHO_FAIL_TEST_BYTES_ERR, "ECHO_FAIL_TEST_BYTES_ERR") \
37 _(ECHO_FAIL_BIND, "ECHO_FAIL_BIND") \
38 _(ECHO_FAIL_SESSION_ACCEPTED_BAD_LISTENER, \
39 "ECHO_FAIL_SESSION_ACCEPTED_BAD_LISTENER") \
40 _(ECHO_FAIL_ACCEPTED_WAIT_FOR_SEG_ALLOC, \
41 "ECHO_FAIL_ACCEPTED_WAIT_FOR_SEG_ALLOC") \
42 _(ECHO_FAIL_SESSION_CONNECT, "ECHO_FAIL_SESSION_CONNECT") \
43 _(ECHO_FAIL_CONNECTED_WAIT_FOR_SEG_ALLOC, \
44 "ECHO_FAIL_CONNECTED_WAIT_FOR_SEG_ALLOC") \
45 _(ECHO_FAIL_APP_ATTACH, "ECHO_FAIL_APP_ATTACH") \
46 _(ECHO_FAIL_SERVER_DISCONNECT_TIMEOUT, \
47 "ECHO_FAIL_SERVER_DISCONNECT_TIMEOUT") \
48 _(ECHO_FAIL_INVALID_URI, "ECHO_FAIL_INVALID_URI") \
49 _(ECHO_FAIL_PROTOCOL_NOT_SUPPORTED, \
50 "ECHO_FAIL_PROTOCOL_NOT_SUPPORTED") \
51 _(ECHO_FAIL_CONNECT_TO_VPP, "ECHO_FAIL_CONNECT_TO_VPP") \
52 _(ECHO_FAIL_ATTACH_TO_VPP, "ECHO_FAIL_ATTACH_TO_VPP") \
53 _(ECHO_FAIL_1ST_PTHREAD_CREATE, "ECHO_FAIL_1ST_PTHREAD_CREATE") \
54 _(ECHO_FAIL_PTHREAD_CREATE, "ECHO_FAIL_PTHREAD_CREATE") \
55 _(ECHO_FAIL_DETACH, "ECHO_FAIL_DETACH") \
56 _(ECHO_FAIL_DEL_CERT_KEY, "ECHO_FAIL_DEL_CERT_KEY") \
57 _(ECHO_FAIL_MQ_PTHREAD, "ECHO_FAIL_MQ_PTHREAD") \
58 _(ECHO_FAIL_VL_API_APP_ATTACH, "ECHO_FAIL_VL_API_APP_ATTACH") \
59 _(ECHO_FAIL_VL_API_MISSING_SEGMENT_NAME, \
60 "ECHO_FAIL_VL_API_MISSING_SEGMENT_NAME") \
61 _(ECHO_FAIL_VL_API_NULL_APP_MQ, "ECHO_FAIL_VL_API_NULL_APP_MQ") \
62 _(ECHO_FAIL_VL_API_RECV_FD_MSG, "ECHO_FAIL_VL_API_RECV_FD_MSG") \
63 _(ECHO_FAIL_VL_API_SVM_FIFO_SEG_ATTACH, \
64 "ECHO_FAIL_VL_API_SVM_FIFO_SEG_ATTACH") \
65 _(ECHO_FAIL_VL_API_FIFO_SEG_ATTACH, \
66 "ECHO_FAIL_VL_API_FIFO_SEG_ATTACH") \
67 _(ECHO_FAIL_VL_API_DETACH_REPLY, "ECHO_FAIL_VL_API_DETACH_REPLY") \
68 _(ECHO_FAIL_VL_API_BIND_URI_REPLY, "ECHO_FAIL_VL_API_BIND_URI_REPLY") \
69 _(ECHO_FAIL_VL_API_UNBIND_REPLY, "ECHO_FAIL_VL_API_UNBIND_REPLY") \
70 _(ECHO_FAIL_SESSION_DISCONNECT, "ECHO_FAIL_SESSION_DISCONNECT") \
71 _(ECHO_FAIL_SESSION_RESET, "ECHO_FAIL_SESSION_RESET") \
72 _(ECHO_FAIL_VL_API_CERT_KEY_ADD_REPLY, \
73 "ECHO_FAIL_VL_API_CERT_KEY_ADD_REPLY") \
74 _(ECHO_FAIL_VL_API_CERT_KEY_DEL_REPLY, \
75 "ECHO_FAIL_VL_API_CERT_KEY_DEL_REPLY") \
76 _(ECHO_FAIL_GET_SESSION_FROM_HANDLE, \
77 "ECHO_FAIL_GET_SESSION_FROM_HANDLE") \
78 _(ECHO_FAIL_QUIC_WRONG_CONNECT, "ECHO_FAIL_QUIC_WRONG_CONNECT") \
79 _(ECHO_FAIL_QUIC_WRONG_ACCEPT, "ECHO_FAIL_QUIC_WRONG_ACCEPT") \
80 _(ECHO_FAIL_TCP_BAPI_CONNECT, "ECHO_FAIL_TCP_BAPI_CONNECT") \
81 _(ECHO_FAIL_UDP_BAPI_CONNECT, "ECHO_FAIL_UDP_BAPI_CONNECT") \
82 _(ECHO_FAIL_MISSING_START_EVENT, "ECHO_FAIL_MISSING_START_EVENT") \
83 _(ECHO_FAIL_MISSING_END_EVENT, "ECHO_FAIL_MISSING_END_EVENT") \
84 _(ECHO_FAIL_TEST_ASSERT_RX_TOTAL, "ECHO_FAIL_TEST_ASSERT_RX_TOTAL") \
85 _(ECHO_FAIL_UNIDIRECTIONAL, "ECHO_FAIL_UNIDIRECTIONAL") \
86 _(ECHO_FAIL_TEST_ASSERT_TX_TOTAL, "ECHO_FAIL_TEST_ASSERT_TX_TOTAL") \
87 _(ECHO_FAIL_TEST_ASSERT_ALL_SESSIONS_CLOSED, \
88 "ECHO_FAIL_TEST_ASSERT_ALL_SESSIONS_CLOSED") \
89 _(ECHO_FAIL_RPC_SIZE, "ECHO_FAIL_RPC_SIZE")
93 #define _(sym, str) sym,
100 #define CHECK_SAME(fail, expected, result, _fmt, _args...) \
102 if ((expected) != (result)) \
103 ECHO_FAIL ((fail), "expected same (%lld, got %lld) : "_fmt, \
104 (u64)(expected), (u64)(result), ##_args); \
107 #define CHECK_DIFF(fail, expected, result, _fmt, _args...) \
109 if ((expected) == (result)) \
110 ECHO_FAIL ((fail), "expected different (both %lld) : "_fmt, \
111 (u64)(expected), ##_args); \
114 #define ECHO_FAIL(fail, _fmt, _args...) \
116 echo_main_t *em = &echo_main; \
117 em->has_failed = (fail); \
118 if (vec_len(em->fail_descr)) \
119 em->fail_descr = format(em->fail_descr, " | %s (%u): "_fmt, \
120 echo_fail_code_str[fail], fail, ##_args); \
122 em->fail_descr = format(0, "%s (%u): "_fmt, \
123 echo_fail_code_str[fail], fail, ##_args); \
124 em->time_to_stop = 1; \
125 if (em->log_lvl > 0) \
126 clib_warning ("%v", em->fail_descr); \
129 #define ECHO_LOG(lvl, _fmt,_args...) \
131 echo_main_t *em = &echo_main; \
132 if (em->log_lvl > lvl) \
133 clib_warning (_fmt, ##_args); \
136 #define ECHO_REGISTER_PROTO(proto, vft) \
137 static void __clib_constructor \
138 vpp_echo_init_##proto () \
140 echo_main_t *em = &echo_main; \
141 vec_validate (em->available_proto_cb_vft, proto); \
142 em->available_proto_cb_vft[proto] = &vft; \
148 #define _(type, name) type name;
session_connected_msg_t * mp
uword unformat_ip4_address(unformat_input_t *input, va_list *args)
uword echo_unformat_close(unformat_input_t *input, va_list *args)
void echo_segment_detach(u64 segment_handle)
u32 *volatile data_thread_args
void echo_session_handle_add_del(echo_main_t *em, u64 handle, u32 sid)
void echo_send_connect(echo_main_t *em, void *args)
echo_session_t * sessions
u8 * echo_format_app_state(u8 *s, va_list *args)
pthread_t mq_thread_handle
#define CLIB_CACHE_LINE_ALIGN_MARK(mark)
void(* reset_cb)(session_reset_msg_t *mp, echo_session_t *s)
volatile u32 n_clients_connected
echo_proto_cb_vft_t ** available_proto_cb_vft
int echo_attach_session(uword segment_handle, uword rxf_offset, uword mq_offset, uword txf_offset, echo_session_t *s)
u8 * echo_format_session_type(u8 *s, va_list *args)
void echo_send_add_cert_key(echo_main_t *em)
echo_disconnect_args_t disconnect
volatile u64 accepted_session_count
struct _svm_queue svm_queue_t
@ ECHO_EVT_FIRST_QCONNECT
volatile connection_state_t state
@ ECHO_SESSION_TYPE_STREAM
vl_api_dhcp_client_state_t state
void echo_session_print_stats(echo_main_t *em, echo_session_t *session)
@ ECHO_SESSION_STATE_READY
int echo_segment_attach(u64 segment_handle, char *name, ssvm_segment_type_t type, int fd)
struct echo_connect_args_ echo_connect_args_t
int echo_segment_attach_mq(uword segment_handle, uword mq_offset, u32 mq_index, svm_msg_q_t **mq)
volatile u64 bytes_received
u8 * format_ip6_address(u8 *s, va_list *args)
void(* connected_cb)(session_connected_bundled_msg_t *mp, u32 session_index, u8 is_failed)
@ ECHO_SESSION_TYPE_LISTEN
u8 * echo_format_session_state(u8 *s, va_list *args)
enum ssvm_segment_type_ ssvm_segment_type_t
u8 * echo_format_session(u8 *s, va_list *args)
echo_connect_args_t connect
fifo_segment_main_t segment_main
foreach_app_session_field u64 vpp_session_handle
uword * session_index_by_vpp_handles
data_source_t data_source
echo_stats_t last_stat_sampling
@ ECHO_SESSION_STATE_AWAIT_CLOSING
void echo_send_detach(echo_main_t *em)
uword * error_string_by_error_number
void(* accepted_cb)(session_accepted_msg_t *mp, echo_session_t *session)
echo_proto_cb_vft_t * proto_cb_vft
u8 send_stream_disconnects
int echo_send_rpc(echo_main_t *em, void *fp, echo_rpc_args_t *args)
u8 * echo_format_bytes_per_sec(u8 *s, va_list *args)
u32 echo_segment_lookup(u64 segment_handle)
echo_session_t * echo_session_new(echo_main_t *em)
void echo_send_disconnect_session(echo_main_t *em, void *args)
uword unformat_ip6_address(unformat_input_t *input, va_list *args)
void(* bound_uri_cb)(session_bound_msg_t *mp, echo_session_t *session)
#define foreach_echo_fail_code
volatile u32 listen_session_cnt
void(* cleanup_cb)(echo_session_t *s, u8 parent_died)
echo_session_t * echo_get_session_from_handle(echo_main_t *em, u64 handle)
f64 last_stat_sampling_ts
void echo_send_attach(echo_main_t *em)
struct echo_disconnect_args_ echo_disconnect_args_t
@ ECHO_SESSION_STATE_AWAIT_DATA
@ ECHO_SESSION_STATE_CLOSING
svm_msg_q_t rpc_msq_queue
@ ECHO_INVALID_DATA_SOURCE
struct echo_stats_ echo_stats_t
clib_spinlock_t segment_handles_lock
@ ECHO_EVT_LAST_QCONNECTED
@ ECHO_EVT_FIRST_SCONNECT
teardown_stat_t active_count
uword * shared_segment_handles
@ ECHO_SESSION_STATE_INITIAL
void echo_api_hookup(echo_main_t *em)
volatile int max_sim_connects
template key/value backing page structure
teardown_stat_t accepted_count
volatile u64 bytes_to_receive
union session_connected_bundled_msg_ session_connected_bundled_msg_t
clib_spinlock_t sid_vpp_handles_lock
teardown_stat_t connected_count
struct echo_proto_cb_vft_ echo_proto_cb_vft_t
u8 * echo_format_crypto_engine(u8 *s, va_list *args)
void(* echo_rpc_t)(echo_main_t *em, echo_rpc_args_t *arg)
@ ECHO_EVT_LAST_SCONNECTED
void(* set_defaults_before_opts_cb)(void)
svm_fifo_chunk_t * echo_segment_alloc_chunk(uword segment_handle, u32 slice_index, u32 size, uword *offset)
pthread_t * data_thread_handles
teardown_stat_t reset_count
u8 * echo_format_timing_event(u8 *s, va_list *args)
u8 * format_transport_proto(u8 *s, va_list *args)
u8 * format_ip46_address(u8 *s, va_list *args)
svm_queue_t * vl_input_queue
uword unformat_transport_proto(unformat_input_t *input, va_list *args)
#define foreach_app_session_field
flag for dgram mode
u32 * listen_session_indexes
u8 use_sock_api
Flag that decides if socket, instead of svm, api is used to connect to vpp.
teardown_stat_t clean_count
u8 * format_ip4_address(u8 *s, va_list *args)
volatile u32 nxt_available_sidx
void(* print_usage_cb)(void)
char * echo_fail_code_str[]
enum echo_test_evt_ echo_test_evt_t
void echo_send_listen(echo_main_t *em, ip46_address_t *ip)
void(* sent_disconnect_cb)(echo_session_t *s)
void echo_send_del_cert_key(echo_main_t *em)
int(* process_opts_cb)(unformat_input_t *a)
u8 * format_api_error(u8 *s, va_list *args)
@ RETURN_PACKETS_LOG_WRONG
teardown_stat_t close_count
void(* set_defaults_after_opts_cb)(void)
void(* disconnected_cb)(session_disconnected_msg_t *mp, echo_session_t *s)
@ ECHO_SESSION_STATE_CLOSED
u64 parent_session_handle
uword echo_unformat_timing_event(unformat_input_t *input, va_list *args)
void echo_send_unbind(echo_main_t *em, echo_session_t *s)
void init_error_string_table()
struct teardown_stat_ teardown_stat_t
int wait_for_state_change(echo_main_t *em, connection_state_t state, f64 timeout)
uword echo_unformat_crypto_engine(unformat_input_t *input, va_list *args)
vl_api_fib_path_type_t type
void echo_notify_event(echo_main_t *em, echo_test_evt_t e)