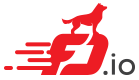 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
21 #ifndef __included_crypto_blake2s_h__
22 #define __included_crypto_blake2s_h__
27 #define BLAKE2_PACKED(x) __pragma(pack(push, 1)) x __pragma(pack(pop))
29 #define BLAKE2_PACKED(x) x __attribute__((packed))
55 uint8_t digest_length;
79 int blake2s (
void *out,
size_t outlen,
const void *in,
size_t inlen,
80 const void *
key,
size_t keylen);
int blake2s_init_key(blake2s_state_t *S, size_t outlen, const void *key, size_t keylen)
int blake2s_final(blake2s_state_t *S, void *out, size_t outlen)
uint8_t buf[BLAKE2S_BLOCK_BYTES]
int blake2s_update(blake2s_state_t *S, const void *in, size_t inlen)
int blake2s(void *out, size_t outlen, const void *in, size_t inlen, const void *key, size_t keylen)
int blake2s_init(blake2s_state_t *S, size_t outlen)
int blake2s_init_param(blake2s_state_t *S, const blake2s_param_t *P)
struct blake2s_param blake2s_param_t
struct blake2s_state blake2s_state_t