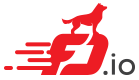 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
16 #ifndef SRC_VNET_UDP_UDP_INLINES_H_
17 #define SRC_VNET_UDP_UDP_INLINES_H_
37 uh->
length = clib_host_to_net_u16 (udp_len);
41 b->
flags |= VNET_BUFFER_F_L4_HDR_OFFSET_VALID;
136 ASSERT (_vec_len (ec0) == _vec_len (ec1));
148 u16 old_l0 = 0, old_l1 = 0;
u16 ip6_tcp_udp_icmp_compute_checksum(vlib_main_t *vm, vlib_buffer_t *p0, ip6_header_t *ip0, int *bogus_lengthp)
static void * vlib_buffer_push_udp(vlib_buffer_t *b, u16 sp, u16 dp, u8 offload_csum)
static_always_inline void vnet_buffer_offload_flags_set(vlib_buffer_t *b, vnet_buffer_oflags_t oflags)
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
static_always_inline void vnet_calc_checksums_inline(vlib_main_t *vm, vlib_buffer_t *b, int is_ip4, int is_ip6)
static uword vlib_buffer_length_in_chain(vlib_main_t *vm, vlib_buffer_t *b)
Get length in bytes of the buffer chain.
static_always_inline void * clib_memcpy_fast(void *restrict dst, const void *restrict src, size_t n)
static void ip_udp_encap_one(vlib_main_t *vm, vlib_buffer_t *b0, u8 *ec0, word ec_len, u8 is_ip4)
static void vlib_buffer_advance(vlib_buffer_t *b, word l)
Advance current data pointer by the supplied (signed!) amount.
static void ip_udp_encap_two(vlib_main_t *vm, vlib_buffer_t *b0, vlib_buffer_t *b1, u8 *ec0, u8 *ec1, word ec_len, u8 is_v4)
if(node->flags &VLIB_NODE_FLAG_TRACE) vnet_interface_output_trace(vm
u16 current_length
Nbytes between current data and the end of this buffer.
static void * vlib_buffer_push_uninit(vlib_buffer_t *b, u8 size)
Prepend uninitialized data to buffer.
static void ip_udp_fixup_one(vlib_main_t *vm, vlib_buffer_t *b0, u8 is_ip4)
#define ip_csum_update(sum, old, new, type, field)
static void * vlib_buffer_get_current(vlib_buffer_t *b)
Get pointer to current data to process.
u32 total_length_not_including_first_buffer
Only valid for first buffer in chain.
static u16 ip_csum_fold(ip_csum_t c)
u32 flags
buffer flags: VLIB_BUFFER_FREE_LIST_INDEX_MASK: bits used to store free list index,...
VLIB buffer representation.