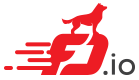 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
40 #ifndef included_vnet_buffer_h
41 #define included_vnet_buffer_h
49 #define foreach_vnet_buffer_flag \
50 _ (1, L4_CHECKSUM_COMPUTED, "l4-cksum-computed", 1) \
51 _ (2, L4_CHECKSUM_CORRECT, "l4-cksum-correct", 1) \
52 _ (3, VLAN_2_DEEP, "vlan-2-deep", 1) \
53 _ (4, VLAN_1_DEEP, "vlan-1-deep", 1) \
54 _ (5, SPAN_CLONE, "span-clone", 1) \
55 _ (6, LOOP_COUNTER_VALID, "loop-counter-valid", 0) \
56 _ (7, LOCALLY_ORIGINATED, "local", 1) \
57 _ (8, IS_IP4, "ip4", 1) \
58 _ (9, IS_IP6, "ip6", 1) \
59 _ (10, OFFLOAD, "offload", 0) \
60 _ (11, IS_NATED, "natted", 1) \
61 _ (12, L2_HDR_OFFSET_VALID, "l2_hdr_offset_valid", 0) \
62 _ (13, L3_HDR_OFFSET_VALID, "l3_hdr_offset_valid", 0) \
63 _ (14, L4_HDR_OFFSET_VALID, "l4_hdr_offset_valid", 0) \
64 _ (15, FLOW_REPORT, "flow-report", 1) \
65 _ (16, IS_DVR, "dvr", 1) \
66 _ (17, QOS_DATA_VALID, "qos-data-valid", 0) \
67 _ (18, GSO, "gso", 0) \
68 _ (19, AVAIL1, "avail1", 1) \
69 _ (20, AVAIL2, "avail2", 1) \
70 _ (21, AVAIL3, "avail3", 1) \
71 _ (22, AVAIL4, "avail4", 1) \
72 _ (23, AVAIL5, "avail5", 1) \
73 _ (24, AVAIL6, "avail6", 1) \
74 _ (25, AVAIL7, "avail7", 1) \
75 _ (26, AVAIL8, "avail8", 1) \
76 _ (27, AVAIL9, "avail9", 1)
84 #define VNET_BUFFER_FLAGS_ALL_AVAIL \
85 (VNET_BUFFER_F_AVAIL1 | VNET_BUFFER_F_AVAIL2 | VNET_BUFFER_F_AVAIL3 | \
86 VNET_BUFFER_F_AVAIL4 | VNET_BUFFER_F_AVAIL5 | VNET_BUFFER_F_AVAIL6 | \
87 VNET_BUFFER_F_AVAIL7 | VNET_BUFFER_F_AVAIL8 | VNET_BUFFER_F_AVAIL9)
89 #define VNET_BUFFER_FLAGS_VLAN_BITS \
90 (VNET_BUFFER_F_VLAN_1_DEEP | VNET_BUFFER_F_VLAN_2_DEEP)
94 #define _(bit, name, s, v) VNET_BUFFER_F_##name = (1 << LOG2_VLIB_BUFFER_FLAG_USER(bit)),
101 #define _(bit, name, s, v) VNET_BUFFER_F_LOG2_##name = LOG2_VLIB_BUFFER_FLAG_USER(bit),
108 "VLIB / VNET buffer flags overlap");
110 #define foreach_vnet_buffer_offload_flag \
111 _ (0, IP_CKSUM, "offload-ip-cksum", 1) \
112 _ (1, TCP_CKSUM, "offload-tcp-cksum", 1) \
113 _ (2, UDP_CKSUM, "offload-udp-cksum", 1) \
114 _ (3, OUTER_IP_CKSUM, "offload-outer-ip-cksum", 1) \
115 _ (4, OUTER_UDP_CKSUM, "offload-outer-udp-cksum", 1) \
116 _ (5, TNL_VXLAN, "offload-vxlan-tunnel", 1) \
117 _ (6, TNL_IPIP, "offload-ipip-tunnel", 1)
121 #define _(bit, name, s, v) VNET_BUFFER_OFFLOAD_F_##name = (1 << bit),
126 #define VNET_BUFFER_OFFLOAD_F_TNL_MASK \
127 (VNET_BUFFER_OFFLOAD_F_TNL_VXLAN | VNET_BUFFER_OFFLOAD_F_TNL_IPIP)
129 #define foreach_buffer_opaque_union_subtype \
235 u8 save_rewrite_length;
277 u8 save_rewrite_length;
411 #define VNET_REWRITE_TOTAL_BYTES (VLIB_BUFFER_PRE_DATA_SIZE)
415 ip.reass.save_rewrite_length)
417 ip.reass.save_rewrite_length) ==
422 "save_rewrite_length member must be able to hold the max value of rewrite length");
426 ip.reass.save_rewrite_length)
430 ip.reass.save_rewrite_length),
431 "save_rewrite_length must be aligned so that reass doesn't overwrite it");
439 "VNET buffer meta-data too large for vlib_buffer");
441 #define vnet_buffer(b) ((vnet_buffer_opaque_t *) (b)->opaque)
505 #define vnet_buffer2(b) ((vnet_buffer_opaque2_t *) (b)->opaque2)
513 "VNET buffer opaque2 meta-data too large for vlib_buffer");
515 #define gso_mtu_sz(b) (vnet_buffer2(b)->gso_size + \
516 vnet_buffer2(b)->gso_l4_hdr_sz + \
517 vnet_buffer(b)->l4_hdr_offset - \
518 vnet_buffer (b)->l3_hdr_offset)
530 if (
b->
flags & VNET_BUFFER_F_OFFLOAD)
539 b->
flags |= VNET_BUFFER_F_OFFLOAD;
548 b->
flags &= ~VNET_BUFFER_F_OFFLOAD;
#define VNET_BUFFER_FLAGS_ALL_AVAIL
nat44_ei_hairpin_src_next_t next_index
format_function_t format_vnet_buffer_offload
format_function_t format_vnet_buffer_opaque
static_always_inline void vnet_buffer_offload_flags_set(vlib_buffer_t *b, vnet_buffer_oflags_t oflags)
#define VLIB_BUFFER_FLAGS_ALL
#define STRUCT_OFFSET_OF(t, f)
#define VNET_REWRITE_TOTAL_BYTES
#define foreach_vnet_buffer_offload_flag
static_always_inline void vnet_buffer_offload_flags_clear(vlib_buffer_t *b, vnet_buffer_oflags_t oflags)
STATIC_ASSERT(((VNET_BUFFER_FLAGS_ALL_AVAIL &VLIB_BUFFER_FLAGS_ALL)==0), "VLIB / VNET buffer flags overlap")
#define static_always_inline
format_function_t format_vnet_buffer_no_chain
u32 cached_dst_nat_session_index
vnet_buffer_oflags_t oflags
u32 required_thread_index
#define foreach_vnet_buffer_flag
Flags that are set in the high order bits of ((vlib_buffer*)b)->flags.
u16 hdr_offset
offset relative to ip hdr
u16 data_offset
offset relative to ip hdr
format_function_t format_vnet_buffer_flags
u8 icmp_type_or_tcp_flags
u8 pad[3]
log2 (size of the packing page block)
vl_api_interface_index_t sw_if_index
format_function_t format_vnet_buffer_opaque2
#define STRUCT_SIZE_OF(t, f)
format_function_t format_vnet_buffer
u32 flags
buffer flags: VLIB_BUFFER_FREE_LIST_INDEX_MASK: bits used to store free list index,...
VLIB buffer representation.
vl_api_wireguard_peer_flags_t flags