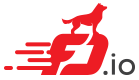 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
38 #ifndef included_format_h
39 #define included_format_h
61 #define stdin ((FILE *) 0)
62 #define stdout ((FILE *) 1)
63 #define stderr ((FILE *) 2)
90 #define _(f) __clib_export u8 * f (u8 * s, va_list * va)
119 typedef struct _unformat_input_t
133 uword (*fill_buffer) (
struct _unformat_input_t *
i);
137 #define UNFORMAT_END_OF_INPUT (~0)
138 #define UNFORMAT_MORE_INPUT 0
141 void *fill_buffer_arg;
147 void *fill_buffer_arg)
150 i->fill_buffer = fill_buffer;
151 i->fill_buffer_arg = fill_buffer_arg;
169 _unformat_fill_input (
i);
189 input->index =
i + 1;
190 i = input->buffer[
i];
246 char *
string,
int string_len);
261 #define unformat_parse_error(input) \
262 clib_error_return (0, "parse error `%U'", format_unformat_error, input)
#define vec_end(v)
End (last data address) of vector.
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
#define vec_free(V)
Free vector's memory (no header).
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)