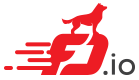 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
18 #include <picotls/openssl.h>
25 const void *, size_t);
32 ptls_cipher_context_t
super;
49 uint8_t
iv[PTLS_MAX_IV_SIZE];
59 ctx->op.key_index =
ctx->key_index;
70 const void *input,
size_t _len)
75 ctx->op.src = (
u8 *) input;
85 const EVP_CIPHER * cipher,
92 ctx->super.do_transform = do_transform;
96 if (!strcmp (
ctx->super.algo->name,
"AES128-CTR"))
98 algo = VNET_CRYPTO_ALG_AES_128_CTR;
99 ctx->id = is_enc ? VNET_CRYPTO_OP_AES_128_CTR_ENC :
100 VNET_CRYPTO_OP_AES_128_CTR_DEC;
102 else if (!strcmp (
ctx->super.algo->name,
"AES256-CTR"))
104 algo = VNET_CRYPTO_ALG_AES_256_CTR;
105 ctx->id = is_enc ? VNET_CRYPTO_OP_AES_256_CTR_ENC :
106 VNET_CRYPTO_OP_AES_256_CTR_DEC;
110 TLS_DBG (1,
"%s, Invalid crypto cipher : ", __FUNCTION__,
117 (
u8 *)
key, _ctx->algo->key_size);
125 const void *input,
size_t inlen, uint64_t seq,
126 const void *aad,
size_t aadlen)
130 int tag_size =
ctx->super.algo->tag_size;
133 ctx->op.aad = (
u8 *) aad;
134 ctx->op.aad_len = aadlen;
136 ptls_aead__build_iv (
ctx->super.algo,
ctx->op.iv,
ctx->static_iv, seq);
137 ctx->op.src = (
u8 *) input;
138 ctx->op.dst = _output;
139 ctx->op.key_index =
ctx->key_index;
140 ctx->op.len = inlen - tag_size;
141 ctx->op.tag_len = tag_size;
145 assert (
ctx->op.status == VNET_CRYPTO_OP_STATUS_COMPLETED);
152 const void *aad,
size_t aadlen)
157 ctx->op.aad = (
void *) aad;
158 ctx->op.aad_len = aadlen;
160 ptls_aead__build_iv (
ctx->super.algo,
ctx->op.iv,
ctx->static_iv, seq);
161 ctx->op.key_index =
ctx->key_index;
162 ctx->op.n_chunks = 2;
163 ctx->op.chunk_index = 0;
170 const void *input,
size_t inlen)
173 ctx->chunks[
ctx->chunk_index].dst = output;
174 ctx->chunks[
ctx->chunk_index].src = (
void *) input;
175 ctx->chunks[
ctx->chunk_index].len = inlen;
177 ctx->chunk_index =
ctx->chunk_index == 0 ? 1 : 0;
188 ctx->op.tag = _output;
189 ctx->op.tag_len =
ctx->super.algo->tag_size;
192 assert (
ctx->op.status == VNET_CRYPTO_OP_STATUS_COMPLETED);
194 return ctx->super.algo->tag_size;
205 const void *
key,
const void *
iv,
212 if (
alg == VNET_CRYPTO_ALG_AES_128_GCM)
214 ctx->id = is_enc ? VNET_CRYPTO_OP_AES_128_GCM_ENC :
215 VNET_CRYPTO_OP_AES_128_GCM_DEC;
217 else if (
alg == VNET_CRYPTO_ALG_AES_256_GCM)
219 ctx->id = is_enc ? VNET_CRYPTO_OP_AES_256_GCM_ENC :
220 VNET_CRYPTO_OP_AES_256_GCM_DEC;
224 TLS_DBG (1,
"%s, invalied aead cipher %s", __FUNCTION__,
230 ctx->chunk_index = 0;
254 int is_enc,
const void *
key)
262 int is_enc,
const void *
key)
270 int is_enc,
const void *
key,
274 VNET_CRYPTO_ALG_AES_128_GCM);
279 int is_enc,
const void *
key,
283 VNET_CRYPTO_ALG_AES_256_GCM);
288 PTLS_AES128_KEY_SIZE,
297 PTLS_AES256_KEY_SIZE,
306 PTLS_AESGCM_CONFIDENTIALITY_LIMIT,
307 PTLS_AESGCM_INTEGRITY_LIMIT,
310 PTLS_AES128_KEY_SIZE,
312 PTLS_AESGCM_TAG_SIZE,
319 PTLS_AESGCM_CONFIDENTIALITY_LIMIT,
320 PTLS_AESGCM_INTEGRITY_LIMIT,
323 PTLS_AES256_KEY_SIZE,
325 PTLS_AESGCM_TAG_SIZE,
331 { PTLS_CIPHER_SUITE_AES_128_GCM_SHA256,
337 { PTLS_CIPHER_SUITE_AES_256_GCM_SHA384,
static_always_inline void vnet_crypto_op_init(vnet_crypto_op_t *op, vnet_crypto_op_id_t type)
static int ptls_vpp_crypto_aead_aes256gcm_setup_crypto(ptls_aead_context_t *ctx, int is_enc, const void *key, const void *iv)
ptls_aead_algorithm_t ptls_vpp_crypto_aes256gcm
#define clib_memcpy(d, s, n)
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
picotls_main_t picotls_main
static size_t ptls_vpp_crypto_aead_encrypt_final(ptls_aead_context_t *_ctx, void *_output)
static void clib_rwlock_writer_unlock(clib_rwlock_t *p)
ptls_aead_algorithm_t ptls_vpp_crypto_aes128gcm
static void ptls_vpp_crypto_aead_encrypt_init(ptls_aead_context_t *_ctx, uint64_t seq, const void *aad, size_t aadlen)
ptls_cipher_suite_t * ptls_vpp_crypto_cipher_suites[]
vnet_crypto_op_chunk_t chunks[2]
static int ptls_vpp_crypto_aead_setup_crypto(ptls_aead_context_t *_ctx, int is_enc, const void *key, const void *iv, vnet_crypto_alg_t alg)
ptls_cipher_suite_t ptls_vpp_crypto_aes128gcmsha256
ptls_aead_context_t super
static void ptls_vpp_crypto_cipher_encrypt(ptls_cipher_context_t *_ctx, void *output, const void *input, size_t _len)
u32 vnet_crypto_process_ops(vlib_main_t *vm, vnet_crypto_op_t ops[], u32 n_ops)
#define TLS_DBG(_lvl, _fmt, _args...)
clib_rwlock_t crypto_keys_rw_lock
u32 vnet_crypto_process_chained_ops(vlib_main_t *vm, vnet_crypto_op_t ops[], vnet_crypto_op_chunk_t *chunks, u32 n_ops)
static size_t ptls_vpp_crypto_aead_encrypt_update(ptls_aead_context_t *_ctx, void *output, const void *input, size_t inlen)
#define VNET_CRYPTO_OP_FLAG_CHAINED_BUFFERS
static int ptls_vpp_crypto_aes128ctr_setup_crypto(ptls_cipher_context_t *ctx, int is_enc, const void *key)
static void clib_rwlock_writer_lock(clib_rwlock_t *p)
static int ptls_vpp_crypto_aead_aes128gcm_setup_crypto(ptls_aead_context_t *ctx, int is_enc, const void *key, const void *iv)
uint8_t static_iv[PTLS_MAX_IV_SIZE]
static void ptls_vpp_crypto_cipher_dispose(ptls_cipher_context_t *_ctx)
ptls_cipher_context_t super
vnet_crypto_main_t crypto_main
u32 vnet_crypto_key_add(vlib_main_t *vm, vnet_crypto_alg_t alg, u8 *data, u16 length)
size_t ptls_vpp_crypto_aead_decrypt(ptls_aead_context_t *_ctx, void *_output, const void *input, size_t inlen, uint64_t seq, const void *aad, size_t aadlen)
static int ptls_vpp_crypto_cipher_setup_crypto(ptls_cipher_context_t *_ctx, int is_enc, const void *key, const EVP_CIPHER *cipher, ptls_vpp_do_transform_fn do_transform)
ptls_cipher_algorithm_t ptls_vpp_crypto_aes256ctr
ptls_cipher_suite_t ptls_vpp_crypto_aes256gcmsha384
static vlib_main_t * vlib_get_main(void)
void(* ptls_vpp_do_transform_fn)(ptls_cipher_context_t *, void *, const void *, size_t)
static int ptls_vpp_crypto_aes256ctr_setup_crypto(ptls_cipher_context_t *ctx, int is_enc, const void *key)
uint8_t iv[PTLS_MAX_IV_SIZE]
ptls_cipher_algorithm_t ptls_vpp_crypto_aes128ctr
static void ptls_vpp_crypto_cipher_do_init(ptls_cipher_context_t *_ctx, const void *iv)
static void ptls_vpp_crypto_aead_dispose_crypto(ptls_aead_context_t *_ctx)