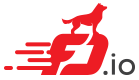 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
16 #ifndef included_vnet_crypto_crypto_h
17 #define included_vnet_crypto_crypto_h
21 #define VNET_CRYPTO_FRAME_SIZE 64
22 #define VNET_CRYPTO_FRAME_POOL_SIZE 1024
25 #define foreach_crypto_cipher_alg \
26 _(DES_CBC, "des-cbc", 7) \
27 _(3DES_CBC, "3des-cbc", 24) \
28 _(AES_128_CBC, "aes-128-cbc", 16) \
29 _(AES_192_CBC, "aes-192-cbc", 24) \
30 _(AES_256_CBC, "aes-256-cbc", 32) \
31 _(AES_128_CTR, "aes-128-ctr", 16) \
32 _(AES_192_CTR, "aes-192-ctr", 24) \
33 _(AES_256_CTR, "aes-256-ctr", 32)
36 #define foreach_crypto_aead_alg \
37 _(AES_128_GCM, "aes-128-gcm", 16) \
38 _(AES_192_GCM, "aes-192-gcm", 24) \
39 _(AES_256_GCM, "aes-256-gcm", 32) \
40 _(CHACHA20_POLY1305, "chacha20-poly1305", 32)
42 #define foreach_crypto_hash_alg \
44 _ (SHA224, "sha-224") \
45 _ (SHA256, "sha-256") \
46 _ (SHA384, "sha-384") \
49 #define foreach_crypto_hmac_alg \
52 _(SHA224, "sha-224") \
53 _(SHA256, "sha-256") \
54 _(SHA384, "sha-384") \
57 #define foreach_crypto_op_type \
58 _ (ENCRYPT, "encrypt") \
59 _ (DECRYPT, "decrypt") \
60 _ (AEAD_ENCRYPT, "aead-encrypt") \
61 _ (AEAD_DECRYPT, "aead-decrypt") \
67 #define _(n, s) VNET_CRYPTO_OP_TYPE_##n,
73 #define foreach_crypto_op_status \
75 _(PENDING, "pending") \
76 _(WORK_IN_PROGRESS, "work-in-progress") \
77 _(COMPLETED, "completed") \
78 _(FAIL_NO_HANDLER, "no-handler") \
79 _(FAIL_BAD_HMAC, "bad-hmac") \
80 _(FAIL_ENGINE_ERR, "engine-error")
85 #define foreach_crypto_aead_async_alg \
86 _(AES_128_GCM, "aes-128-gcm-aad8", 16, 16, 8) \
87 _(AES_128_GCM, "aes-128-gcm-aad12", 16, 16, 12) \
88 _(AES_192_GCM, "aes-192-gcm-aad8", 24, 16, 8) \
89 _(AES_192_GCM, "aes-192-gcm-aad12", 24, 16, 12) \
90 _(AES_256_GCM, "aes-256-gcm-aad8", 32, 16, 8) \
91 _(AES_256_GCM, "aes-256-gcm-aad12", 32, 16, 12) \
92 _(CHACHA20_POLY1305, "chacha20-poly1305-aad8", 32, 16, 8) \
93 _(CHACHA20_POLY1305, "chacha20-poly1305-aad12", 32, 16, 12)
96 #define foreach_crypto_link_async_alg \
97 _ (3DES_CBC, MD5, "3des-cbc-hmac-md5", 24, 12) \
98 _ (AES_128_CBC, MD5, "aes-128-cbc-hmac-md5", 16, 12) \
99 _ (AES_192_CBC, MD5, "aes-192-cbc-hmac-md5", 24, 12) \
100 _ (AES_256_CBC, MD5, "aes-256-cbc-hmac-md5", 32, 12) \
101 _ (3DES_CBC, SHA1, "3des-cbc-hmac-sha-1", 24, 12) \
102 _ (AES_128_CBC, SHA1, "aes-128-cbc-hmac-sha-1", 16, 12) \
103 _ (AES_192_CBC, SHA1, "aes-192-cbc-hmac-sha-1", 24, 12) \
104 _ (AES_256_CBC, SHA1, "aes-256-cbc-hmac-sha-1", 32, 12) \
105 _ (3DES_CBC, SHA224, "3des-cbc-hmac-sha-224", 24, 14) \
106 _ (AES_128_CBC, SHA224, "aes-128-cbc-hmac-sha-224", 16, 14) \
107 _ (AES_192_CBC, SHA224, "aes-192-cbc-hmac-sha-224", 24, 14) \
108 _ (AES_256_CBC, SHA224, "aes-256-cbc-hmac-sha-224", 32, 14) \
109 _ (3DES_CBC, SHA256, "3des-cbc-hmac-sha-256", 24, 16) \
110 _ (AES_128_CBC, SHA256, "aes-128-cbc-hmac-sha-256", 16, 16) \
111 _ (AES_192_CBC, SHA256, "aes-192-cbc-hmac-sha-256", 24, 16) \
112 _ (AES_256_CBC, SHA256, "aes-256-cbc-hmac-sha-256", 32, 16) \
113 _ (3DES_CBC, SHA384, "3des-cbc-hmac-sha-384", 24, 24) \
114 _ (AES_128_CBC, SHA384, "aes-128-cbc-hmac-sha-384", 16, 24) \
115 _ (AES_192_CBC, SHA384, "aes-192-cbc-hmac-sha-384", 24, 24) \
116 _ (AES_256_CBC, SHA384, "aes-256-cbc-hmac-sha-384", 32, 24) \
117 _ (3DES_CBC, SHA512, "3des-cbc-hmac-sha-512", 24, 32) \
118 _ (AES_128_CBC, SHA512, "aes-128-cbc-hmac-sha-512", 16, 32) \
119 _ (AES_192_CBC, SHA512, "aes-192-cbc-hmac-sha-512", 24, 32) \
120 _ (AES_256_CBC, SHA512, "aes-256-cbc-hmac-sha-512", 32, 32) \
121 _ (AES_128_CTR, SHA1, "aes-128-ctr-hmac-sha-1", 16, 12) \
122 _ (AES_192_CTR, SHA1, "aes-192-ctr-hmac-sha-1", 24, 12) \
123 _ (AES_256_CTR, SHA1, "aes-256-ctr-hmac-sha-1", 32, 12)
125 #define foreach_crypto_async_op_type \
126 _(ENCRYPT, "async-encrypt") \
127 _(DECRYPT, "async-decrypt")
138 #define _(n, s) VNET_CRYPTO_OP_STATUS_##n,
148 #define _(n, s, l) VNET_CRYPTO_ALG_##n,
151 #define _(n, s) VNET_CRYPTO_ALG_HMAC_##n,
154 #define _(n, s) VNET_CRYPTO_ALG_HASH_##n,
162 #define _(n, s) VNET_CRYPTO_ASYNC_OP_TYPE_##n,
171 #define _(n, s, k, t, a) \
172 VNET_CRYPTO_ALG_##n##_TAG##t##_AAD##a,
175 #define _(c, h, s, k ,d) \
176 VNET_CRYPTO_ALG_##c##_##h##_TAG##d,
185 #define _(n, s, k, t, a) \
186 VNET_CRYPTO_OP_##n##_TAG##t##_AAD##a##_ENC, \
187 VNET_CRYPTO_OP_##n##_TAG##t##_AAD##a##_DEC,
190 #define _(c, h, s, k ,d) \
191 VNET_CRYPTO_OP_##c##_##h##_TAG##d##_ENC, \
192 VNET_CRYPTO_OP_##c##_##h##_TAG##d##_DEC,
214 #define VNET_CRYPTO_KEY_TYPE_DATA 0
215 #define VNET_CRYPTO_KEY_TYPE_LINK 1
222 #define _(n, s, l) VNET_CRYPTO_OP_##n##_ENC, VNET_CRYPTO_OP_##n##_DEC,
225 #define _(n, s) VNET_CRYPTO_OP_##n##_HMAC,
228 #define _(n, s) VNET_CRYPTO_OP_##n##_HASH,
262 #define VNET_CRYPTO_OP_FLAG_INIT_IV (1 << 0)
263 #define VNET_CRYPTO_OP_FLAG_HMAC_CHECK (1 << 1)
264 #define VNET_CRYPTO_OP_FLAG_CHAINED_BUFFERS (1 << 2)
398 u32 * enqueue_thread_idx);
471 #define VNET_CRYPTO_ASYNC_DISPATCH_POLLING 0
472 #define VNET_CRYPTO_ASYNC_DISPATCH_INTERRUPT 1
609 cm->crypto_node_index);
622 u32 key_index,
u32 crypto_len,
623 i16 integ_len_adj,
i16 crypto_start_offset,
624 u16 integ_start_offset,
u32 buffer_index,
645 f->buffer_indices[
index] = buffer_index;
646 f->next_node_index[
index] = next_node;
vnet_crypto_async_frame_t *() vnet_crypto_frame_dequeue_t(vlib_main_t *vm, u32 *nb_elts_processed, u32 *enqueue_thread_idx)
static_always_inline void vnet_crypto_async_free_frame(vlib_main_t *vm, vnet_crypto_async_frame_t *frame)
static_always_inline void vnet_crypto_op_init(vnet_crypto_op_t *op, vnet_crypto_op_id_t type)
static u32 vlib_num_workers()
int vnet_crypto_set_handler2(char *ops_handler_name, char *engine, crypto_op_class_type_t oct)
unformat_function_t unformat_vnet_crypto_alg
vnet_crypto_frame_enqueue_t ** enqueue_handlers
vnet_crypto_async_frame_t * frame_pool
format_function_t format_vnet_crypto_async_op_type
#define foreach_crypto_op_status
vlib_main_t vlib_node_runtime_t vlib_frame_t * frame
@ VNET_CRYPTO_ASYNC_ALG_NONE
vnet_crypto_main_t crypto_main
#define foreach_crypto_aead_async_alg
async crypto
@ VNET_CRYPTO_KEY_OP_MODIFY
enum vnet_crypto_async_frame_state_t_ vnet_crypto_async_frame_state_t
#define foreach_crypto_link_async_alg
@ VNET_CRYPTO_ASYNC_OP_N_IDS
u32 vnet_crypto_register_engine(vlib_main_t *vm, char *name, int prio, char *desc)
@ VNET_CRYPTO_ASYNC_OP_N_TYPES
int vnet_crypto_set_async_handler2(char *alg_name, char *engine)
#define CLIB_CACHE_LINE_ALIGN_MARK(mark)
vnet_crypto_op_status_t status
#define foreach_crypto_hash_alg
u32 vnet_crypto_key_add_linked(vlib_main_t *vm, vnet_crypto_key_index_t index_crypto, vnet_crypto_key_index_t index_integ)
Use 2 created keys to generate new key for linked algs (cipher + integ) The returned key index is to ...
#define pool_get_aligned(P, E, A)
Allocate an object E from a pool P with alignment A.
vnet_crypto_alg_data_t * algs
int vnet_crypto_is_set_handler(vnet_crypto_alg_t alg)
#define VNET_CRYPTO_FRAME_SIZE
@ VNET_CRYPTO_N_ASYNC_ALGS
void() vnet_crypto_key_handler_t(vlib_main_t *vm, vnet_crypto_key_op_t kop, vnet_crypto_key_index_t idx)
uword * async_alg_index_by_name
u32 vnet_crypto_key_index_t
#define foreach_crypto_hmac_alg
vnet_crypto_thread_t * threads
vl_api_tunnel_mode_t mode
uword * alg_index_by_name
static_always_inline void vnet_crypto_async_add_to_frame(vlib_main_t *vm, vnet_crypto_async_frame_t *f, u32 key_index, u32 crypto_len, i16 integ_len_adj, i16 crypto_start_offset, u16 integ_start_offset, u32 buffer_index, u16 next_node, u8 *iv, u8 *tag, u8 *aad, u8 flags)
#define foreach_crypto_async_op_type
#define pool_put(P, E)
Free an object E in pool P.
vnet_crypto_async_alg_data_t * async_algs
vnet_crypto_chained_ops_handler_t ** chained_ops_handlers
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
vnet_crypto_async_frame_state_t state
#define VNET_CRYPTO_ASYNC_DISPATCH_INTERRUPT
vnet_crypto_async_next_node_t * next_nodes
static_always_inline int vnet_crypto_async_submit_open_frame(vlib_main_t *vm, vnet_crypto_async_frame_t *frame)
@ VNET_CRYPTO_FRAME_STATE_ELT_ERROR
void vnet_crypto_set_async_dispatch_mode(u8 mode)
void vnet_crypto_register_ops_handlers(vlib_main_t *vm, u32 engine_index, vnet_crypto_op_id_t opt, vnet_crypto_ops_handler_t *fn, vnet_crypto_chained_ops_handler_t *cfn)
vnet_crypto_async_op_id_t op
vnet_crypto_op_status_t status
int() vnet_crypto_frame_enqueue_t(vlib_main_t *vm, vnet_crypto_async_frame_t *frame)
async crypto function handlers
vnet_crypto_async_op_type_t type
void vnet_crypto_request_async_mode(int is_enable)
vnet_crypto_async_op_type_t
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
#define foreach_crypto_aead_alg
#define static_always_inline
vnet_crypto_async_alg_t vnet_crypto_link_algs(vnet_crypto_alg_t crypto_alg, vnet_crypto_alg_t integ_alg)
static_always_inline void vnet_crypto_async_reset_frame(vnet_crypto_async_frame_t *f)
u32 active_engine_index_simple
u32 vnet_crypto_register_post_node(vlib_main_t *vm, char *post_node_name)
async crypto register functions
vnet_feature_config_main_t * cm
u32() vnet_crypto_chained_ops_handler_t(vlib_main_t *vm, vnet_crypto_op_t *ops[], vnet_crypto_op_chunk_t *chunks, u32 n_ops)
static_always_inline vnet_crypto_key_t * vnet_crypto_get_key(vnet_crypto_key_index_t index)
u8 flags
share same VNET_CRYPTO_OP_FLAG_* values
static_always_inline u8 vnet_crypto_async_frame_is_full(const vnet_crypto_async_frame_t *f)
STATIC_ASSERT_SIZEOF(vnet_crypto_op_t, CLIB_CACHE_LINE_BYTES)
vnet_crypto_frame_dequeue_t ** dequeue_handlers
vnet_crypto_async_alg_t async_alg
#define CLIB_CACHE_LINE_BYTES
@ VNET_CRYPTO_OP_N_STATUS
format_function_t format_vnet_crypto_op
vnet_crypto_key_handler_t * key_op_handler
void vnet_crypto_register_key_handler(vlib_main_t *vm, u32 engine_index, vnet_crypto_key_handler_t *keyh)
u32 active_engine_index_chained
#define foreach_crypto_op_type
uword * engine_index_by_name
vnet_crypto_op_type_t type
u32 vnet_crypto_key_add(vlib_main_t *vm, vnet_crypto_alg_t alg, u8 *data, u16 length)
void vnet_crypto_register_ops_handler(vlib_main_t *vm, u32 engine_index, vnet_crypto_op_id_t opt, vnet_crypto_ops_handler_t *oph)
vnet_crypto_async_frame_state_t_
@ VNET_CRYPTO_FRAME_STATE_NOT_PROCESSED
static_always_inline vnet_crypto_op_type_t vnet_crypto_get_op_type(vnet_crypto_op_id_t id)
static void vlib_node_set_interrupt_pending(vlib_main_t *vm, u32 node_index)
@ VNET_CRYPTO_FRAME_STATE_SUCCESS
static_always_inline vnet_crypto_async_frame_t * vnet_crypto_async_get_frame(vlib_main_t *vm, vnet_crypto_async_op_id_t opt)
async crypto inline functions
static vlib_main_t * vlib_get_main_by_index(u32 thread_index)
static_always_inline int vnet_crypto_set_handler(char *alg_name, char *engine)
format_function_t format_vnet_crypto_async_alg
u32 vnet_crypto_process_ops(vlib_main_t *vm, vnet_crypto_op_t ops[], u32 n_ops)
int vnet_crypto_is_set_async_handler(vnet_crypto_async_op_id_t opt)
@ VNET_CRYPTO_FRAME_STATE_PENDING
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
clib_bitmap_t * async_active_ids
vnet_crypto_async_op_id_t
format_function_t format_vnet_crypto_engine
clib_error_t * crypto_dispatch_enable_disable(int is_enable)
u32() vnet_crypto_ops_handler_t(vlib_main_t *vm, vnet_crypto_op_t *ops[], u32 n_ops)
void vnet_crypto_register_chained_ops_handler(vlib_main_t *vm, u32 engine_index, vnet_crypto_op_id_t opt, vnet_crypto_chained_ops_handler_t *oph)
#define foreach_crypto_cipher_alg
@ VNET_CRYPTO_ASYNC_OP_NONE
vnet_crypto_ops_handler_t ** ops_handlers
vnet_crypto_engine_t * engines
format_function_t format_vnet_crypto_alg
u32 active_engine_index_async
static uword clib_bitmap_set_no_check(uword *a, uword i, uword new_value)
Sets the ith bit of a bitmap to new_value.
static vlib_thread_main_t * vlib_get_thread_main()
void vnet_crypto_key_del(vlib_main_t *vm, vnet_crypto_key_index_t index)
format_function_t format_vnet_crypto_async_op
void vnet_crypto_register_async_handler(vlib_main_t *vm, u32 engine_index, vnet_crypto_async_op_id_t opt, vnet_crypto_frame_enqueue_t *enq_fn, vnet_crypto_frame_dequeue_t *deq_fn)
format_function_t format_vnet_crypto_op_status
vl_api_fib_path_type_t type
u32 vnet_crypto_process_chained_ops(vlib_main_t *vm, vnet_crypto_op_t ops[], vnet_crypto_op_chunk_t *chunks, u32 n_ops)
@ VNET_CRYPTO_FRAME_STATE_WORK_IN_PROGRESS
format_function_t format_vnet_crypto_op_type
vnet_crypto_async_alg_t alg
vl_api_wireguard_peer_flags_t flags