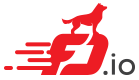 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
20 #include <sys/types.h>
21 #include <sys/socket.h>
24 #include <arpa/inet.h>
55 struct sockaddr_storage server_addr;
60 #define vtc_min(a, b) (a < b ? a : b)
61 #define vtc_max(a, b) (a > b ? a : b)
69 int rx_bytes, tx_bytes;
74 vtinf (
"(fd %d): Sending config to server.", ts->
fd);
77 tx_bytes = ts->
write (ts, &ts->
cfg, sizeof (ts->
cfg));
80 vtwrn (
"(fd %d): write test cfg failed (%d)!", ts->
fd, tx_bytes);
90 vtwrn (
"(fd %d): Bad server reply cfg -- aborting!", ts->
fd);
96 vtwrn (
"(fd %d): Invalid config received from server!", ts->
fd);
99 vtinf (
"\tRx bytes %d != cfg size %lu", rx_bytes,
105 vtinf (
"(fd %d): Valid config sent to server.", ts->
fd);
112 vtinf (
"(fd %d): Got config back from server.", ts->
fd);
126 uint32_t n_test_sessions;
129 n_test_sessions =
wrk->cfg.num_test_sessions;
130 if (n_test_sessions < 1)
136 if (
wrk->n_sessions >= n_test_sessions)
147 vterr (
"failed to alloc sessions", -errno);
153 for (
i = 0;
i < n_test_sessions;
i++)
156 memset (ts, 0,
sizeof (*ts));
164 wrk->n_sessions = n_test_sessions;
167 vtinf (
"All test sessions (%d) connected!", n_test_sessions);
180 FD_ZERO (&
wrk->wr_fdset);
181 FD_ZERO (&
wrk->rd_fdset);
183 clock_gettime (CLOCK_REALTIME, &
now);
195 ts->
txbuf[j] = j & 0xff;
201 FD_SET (vppcom_session_index (ts->
fd), &
wrk->wr_fdset);
202 FD_SET (vppcom_session_index (ts->
fd), &
wrk->rd_fdset);
203 sidx = vppcom_session_index (ts->
fd);
206 wrk->max_fd_index += 1;
217 __wrk_index =
wrk->wrk_index;
219 vtinf (
"Initializing worker %u ...",
wrk->wrk_index);
223 if (vppcom_worker_register ())
225 vtwrn (
"failed to register worker");
233 vterr (
"vtc_connect_test_sessions ()",
rv);
253 while (__sync_lock_test_and_set (&
stats_lock, 1))
260 for (
i = 0;
i <
wrk->cfg.num_test_sessions;
i++)
267 snprintf (
buf,
sizeof (
buf),
"CLIENT (fd %d) RESULTS", ts->
fd);
286 for (
i = 0;
i <
wrk->cfg.num_test_sessions;
i++)
289 vppcom_session_close (ts->
fd);
303 clock_gettime (CLOCK_REALTIME, &ts->
stats.
stop);
317 uint32_t n_active_sessions;
318 fd_set _wfdset, *wfdset = &_wfdset;
319 fd_set _rfdset, *rfdset = &_rfdset;
321 int i,
rv, check_rx = 0;
326 vterr (
"vtc_worker_init()",
rv);
330 vtinf (
"Starting test ...");
332 if (
wrk->wrk_index == 0)
333 clock_gettime (CLOCK_REALTIME, &ctrl->
stats.
start);
336 n_active_sessions =
wrk->cfg.num_test_sessions;
339 _wfdset =
wrk->wr_fdset;
340 _rfdset =
wrk->rd_fdset;
342 rv = vppcom_select (
wrk->max_fd_index, (
unsigned long *) rfdset,
343 (
unsigned long *) wfdset, NULL, 0);
352 for (
i = 0;
i <
wrk->cfg.num_test_sessions;
i++)
358 if (FD_ISSET (vppcom_session_index (ts->
fd), rfdset)
366 if (FD_ISSET (vppcom_session_index (ts->
fd), wfdset)
372 vtwrn (
"vppcom_test_write (%d) failed -- aborting test",
382 clock_gettime (CLOCK_REALTIME, &ts->
stats.
stop);
389 vtinf (
"Worker %d done ...",
wrk->wrk_index);
416 vtinf (
" ctrl session info\n"
420 " rxbuf size: %u (0x%08x)\n"
422 " txbuf size: %u (0x%08x)\n"
424 ctrl->
fd, (uint32_t) ctrl->
fd,
430 snprintf (
buf,
sizeof (
buf),
"Echo");
432 snprintf (
buf,
sizeof (
buf),
"%s-directional Stream",
444 memset (&ctrl->
stats, 0, sizeof (ctrl->
stats));
449 vtwrn (
"vppcom_test_write (%d) failed ", ctrl->
fd);
462 uint32_t
i, n_conn, n_conn_per_wrk;
464 vtinf (
"%s-directional Stream Test Starting!",
472 n_conn_per_wrk = n_conn / vcm->
n_workers;
478 wrk->cfg.num_test_sessions =
vtc_min (n_conn_per_wrk, n_conn);
479 n_conn -=
wrk->cfg.num_test_sessions;
486 vtwrn (
"test cfg sync failed -- aborting!");
501 vtinf (
"Sending config on ctrl session (fd %d) for stats...", ctrl->
fd);
505 vtwrn (
"test cfg sync failed -- aborting!");
515 vtwrn (
"post-test cfg sync failed!");
524 uint64_t txbuf_size = strtoull ((
const char *) p, NULL, 10);
535 vtwrn (
"Invalid txbuf size (%lu) < minimum buf size (%u)!",
545 uint32_t num_writes = strtoul ((
const char *) p, NULL, 10);
555 vtwrn (
"invalid num writes: %u", num_writes);
565 uint32_t num_test_sessions = strtoul ((
const char *) p, NULL, 10);
567 if ((num_test_sessions > 0) &&
575 vtwrn (
"invalid num test sessions: %u, (%d max)",
586 uint64_t rxbuf_size = strtoull ((
const char *) p, NULL, 10);
596 vtwrn (
"Invalid rxbuf size (%lu) < minimum buf size (%u)!",
669 "vcl_test_client [OPTIONS] <ipaddr> <port>\n"
671 " -h Print this message and exit.\n"
673 " -c Print test config before test.\n"
674 " -w <dir> Write test results to <dir>.\n"
675 " -X Exit after running test.\n"
676 " -p <proto> Use <proto> transport layer\n"
677 " -D Use UDP transport layer\n"
678 " -L Use TLS transport layer\n"
679 " -E Run Echo test.\n"
680 " -N <num-writes> Test Cfg: number of writes.\n"
681 " -R <rxbuf-size> Test Cfg: rx buffer size.\n"
682 " -T <txbuf-size> Test Cfg: tx buffer size.\n"
683 " -U Run Uni-directional test.\n"
684 " -B Run Bi-directional test.\n"
685 " -V Verbose mode.\n"
686 " -I <N> Use N sessions.\n"
687 " -s <N> Use N sessions.\n"
688 " -S Print incremental stats per session.\n"
689 " -q <n> QUIC : use N Ssessions on top of n Qsessions\n");
700 while ((
c = getopt (argc, argv,
"chnp:w:XE:I:N:R:T:UBV6DLs:q:S")) != -1)
712 vtwrn (
"Invalid value for option -%c!",
c);
718 vtwrn (
"Invalid number of sessions (%d) specified for option -%c!"
719 "\n Valid range is 1 - %d",
730 vtwrn (
"Invalid value for option -%c!",
c);
736 vtwrn (
"Invalid number of Stream sessions (%d) per Qsession"
737 "for option -%c!\nValid range is 1 - %d",
745 if (sscanf (optarg,
"%d", &v) != 1)
747 vtwrn (
"Invalid value for option -%c!",
c);
761 vtwrn (
"Option -%c value larger than txbuf size (%d)!",
773 vtwrn (
"Invalid value for option -%c!",
c);
783 vtwrn (
"Invalid value for option -%c!",
c);
790 (uint8_t **) & ctrl->
rxbuf,
795 vtwrn (
"rxbuf size (%lu) less than minumum (%u)",
806 vtwrn (
"Invalid value for option -%c!",
c);
813 (uint8_t **) & ctrl->
txbuf,
820 vtwrn (
"txbuf size (%lu) less than minumum (%u)!",
843 if (vppcom_unformat_proto (&vcm->
proto, optarg))
844 vtwrn (
"Invalid vppcom protocol %s, defaulting to TCP", optarg);
848 vcm->
proto = VPPCOM_PROTO_UDP;
852 vcm->
proto = VPPCOM_PROTO_TLS;
870 vtwrn (
"Option -%c requires an argument.", optopt);
874 if (isprint (optopt))
875 vtwrn (
"Unknown option `-%c'.", optopt);
877 vtwrn (
"Unknown option character `\\x%x'.", optopt);
885 if (argc < (optind + 2))
887 vtwrn (
"Insufficient number of arguments!");
898 struct sockaddr_in6 *sddr6 = (
struct sockaddr_in6 *) &vcm->
server_addr;
899 sddr6->sin6_family = AF_INET6;
900 inet_pton (AF_INET6, argv[optind++], &(sddr6->sin6_addr));
901 sddr6->sin6_port = htons (atoi (argv[optind]));
909 struct sockaddr_in *saddr4 = (
struct sockaddr_in *) &vcm->
server_addr;
910 saddr4->sin_family = AF_INET;
911 inet_pton (AF_INET, argv[optind++], &(saddr4->sin_addr));
912 saddr4->sin_port = htons (atoi (argv[optind]));
923 printf (
"\nType some characters and hit <return>\n"
928 if (strlen (ctrl->
txbuf) == 1)
930 printf (
"\nNothing to send! Please try again...\n");
948 vtinf (
"(fd %d): Sending exit cfg to server...", ctrl->
fd);
960 ctrl->
fd = vppcom_session_create (VPPCOM_PROTO_TCP, 0 );
963 vterr (
"vppcom_session_create()", ctrl->
fd);
967 vtinf (
"Connecting to server...");
971 vterr (
"vppcom_session_connect()",
rv);
974 vtinf (
"Control session (fd %d) connected.", ctrl->
fd);
988 memset (&ctrl->
stats, 0, sizeof (ctrl->
stats));
1000 clock_gettime (CLOCK_REALTIME, &ctrl->
stats.
stop);
1006 struct sigaction sa;
1008 memset (&sa, 0,
sizeof (sa));
1010 sa.sa_flags = SA_SIGINFO;
1011 if (sigaction (SIGINT, &sa, 0))
1013 vtwrn (
"couldn't intercept sigint");
1035 rv = vppcom_app_create (
"vcl_test_client");
1037 vtfail (
"vppcom_app_create()",
rv);
1044 vtfail (
"vppcom_session_create() ctrl session",
rv);
1108 vppcom_app_destroy ();
static int vtc_cfg_sync(vcl_test_session_t *ts)
vcl_test_stats_t old_stats
vcl_test_session_t ctrl_session
void * realloc(void *p, size_t size)
uint8_t incremental_stats
int main(int argc, char **argv)
static void vtc_print_stats(vcl_test_session_t *ctrl)
static int vtc_worker_init(vcl_test_client_worker_t *wrk)
static void vtc_echo_client(vcl_test_client_main_t *vcm)
void print_usage_and_exit(void)
static void vtc_inc_stats_check(vcl_test_session_t *ts)
static void cfg_rxbuf_size_set(void)
vcl_test_client_main_t vcl_client_main
static double vcl_test_time_diff(struct timespec *old, struct timespec *new)
int(* open)(vcl_test_session_t *ts, vppcom_endpt_t *endpt)
#define VCL_TEST_TOKEN_NUM_WRITES
static void cfg_num_writes_set(void)
static void vcl_test_cfg_dump(vcl_test_cfg_t *cfg, uint8_t is_client)
static void * vtc_worker_loop(void *arg)
#define VCL_TEST_TOKEN_TXBUF_SIZE
#define VCL_TEST_TOKEN_RUN_BI
static void dump_help(void)
#define VCL_TEST_TOKEN_HELP
static vcl_test_t parse_input()
uint32_t num_test_qsessions
#define VCL_TEST_TOKEN_SHOW_CFG
static int vtc_worker_test_setup(vcl_test_client_worker_t *wrk)
static void cfg_num_test_sessions_set(void)
static int vtc_connect_test_sessions(vcl_test_client_worker_t *wrk)
static void vcl_test_session_buf_alloc(vcl_test_session_t *ts)
static int vtc_ctrl_session_init(vcl_test_client_main_t *vcm, vcl_test_session_t *ctrl)
int(* write)(struct vcl_test_session *ts, void *buf, uint32_t buflen)
static void vcl_test_buf_alloc(vcl_test_cfg_t *cfg, uint8_t is_rxbuf, uint8_t **buf, uint32_t *bufsize)
vcl_test_session_t * qsessions
static void vcl_test_session_buf_free(vcl_test_session_t *ts)
static void vtc_stream_client(vcl_test_client_main_t *vcm)
uint32_t num_test_sessions_perq
volatile int active_workers
static void vcl_test_stats_dump(char *header, vcl_test_stats_t *stats, uint8_t show_rx, uint8_t show_tx, uint8_t verbose)
vcl_test_session_t * sessions
volatile int test_running
int(* read)(struct vcl_test_session *ts, void *buf, uint32_t buflen)
vppcom_endpt_t server_endpt
#define VCL_TEST_CFG_BUF_SIZE_MIN
const vcl_test_proto_vft_t * protos[VPPCOM_PROTO_SRTP+1]
uint32_t num_test_sessions
static void vt_incercept_sigs(void)
static void vtc_worker_sessions_exit(vcl_test_client_worker_t *wrk)
#define VCL_TEST_SEPARATOR_STRING
#define VCL_TEST_CFG_MAX_TEST_SESS
static void vcl_test_stats_dump_inc(vcl_test_session_t *ts, int is_rx)
static void vt_sigs_handler(int signum, siginfo_t *si, void *uc)
struct sockaddr_storage server_addr
#define vt_atomic_add(_ptr, _val)
vcl_test_main_t vcl_test_main
static void cfg_txbuf_size_set(void)
static void vcl_test_stats_accumulate(vcl_test_stats_t *accum, vcl_test_stats_t *incr)
static int vcl_test_cfg_verify(vcl_test_cfg_t *cfg, vcl_test_cfg_t *valid_cfg)
static void vtc_accumulate_stats(vcl_test_client_worker_t *wrk, vcl_test_session_t *ctrl)
#define vtwrn(_fmt, _args...)
#define VCL_TEST_TOKEN_EXIT
static void vtc_read_user_input(vcl_test_session_t *ctrl)
#define VCL_TEST_TOKEN_VERBOSE
session_t * sessions
Worker session pool.
vcl_test_client_worker_t * workers
void * calloc(size_t nmemb, size_t size)
#define VCL_TEST_TOKEN_RXBUF_SIZE
#define vtinf(_fmt, _args...)
static void vtc_process_opts(vcl_test_client_main_t *vcm, int argc, char **argv)
#define VCL_TEST_CFG_CTRL_MAGIC
static void vcl_test_cfg_init(vcl_test_cfg_t *cfg)
#define VCL_TEST_TOKEN_RUN_UNI
static int vcl_test_write(vcl_test_session_t *ts, void *buf, uint32_t nbytes)
static void cfg_verbose_toggle(void)
static int vcl_comp_tspec(struct timespec *a, struct timespec *b)
int(* init)(vcl_test_cfg_t *cfg)
static void vtc_ctrl_session_exit(void)
#define VCL_TEST_TOKEN_NUM_TEST_SESS
static int vcl_test_read(vcl_test_session_t *ts, void *buf, uint32_t nbytes)
#define VCL_TEST_DELAY_DISCONNECT