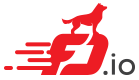 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
61 #ifndef CLIB_MARCH_VARIANT
124 if (b0->
flags & VLIB_BUFFER_IS_TRACED)
132 if (b1->
flags & VLIB_BUFFER_IS_TRACED)
154 if (b0->
flags & VLIB_BUFFER_IS_TRACED)
171 b->
flags & VNET_BUFFER_F_IS_IP6);
182 u32 n_bytes0, n_bytes1, n_bytes2, n_bytes3;
200 ASSERT (
b[0]->current_length > 0);
201 ASSERT (
b[1]->current_length > 0);
202 ASSERT (
b[2]->current_length > 0);
203 ASSERT (
b[3]->current_length > 0);
212 u32 tx_swif0, tx_swif1, tx_swif2, tx_swif3;
260 ASSERT (
b[0]->current_length > 0);
300 if (in_interface_ouput)
326 if (in_interface_ouput)
511 .name =
"interface-output-template",
512 .vector_size =
sizeof (
u32),
522 u32 n_left_to_next, *
from, *to_next;
538 u32 bi0, bi1, next0, next1;
571 n_left_to_next, bi0, bi1, next0,
598 n_left_to_next, bi0, next0);
604 return frame->n_vectors;
639 s =
format (s,
"IP4: %U -> %U",
643 s =
format (s,
"IP6: %U -> %U",
675 if (b0->
flags & VLIB_BUFFER_IS_TRACED)
682 if (b1->
flags & VLIB_BUFFER_IS_TRACED)
703 if (b0->
flags & VLIB_BUFFER_IS_TRACED)
727 if (
b->
flags & VNET_BUFFER_F_L2_HDR_OFFSET_VALID)
745 case ETHERNET_TYPE_IP4:
746 ip4 = (
void *) (eh + 1);
749 t->
src.ip4.as_u32 =
ip4->src_address.as_u32;
750 t->
dst.ip4.as_u32 =
ip4->dst_address.as_u32;
753 case ETHERNET_TYPE_IP6:
754 ip6 = (
void *) (eh + 1);
758 sizeof (ip6_address_t));
760 sizeof (ip6_address_t));
897 return frame->n_vectors;
909 i16 save_current_data;
910 u16 save_current_length;
947 if (b0->
flags & VNET_BUFFER_F_L2_HDR_OFFSET_VALID)
960 u32 error_node_index;
964 int error_string_len =
972 drop_string_len = error_string_len +
vec_len (n->
name) + 2;
975 while (
last->flags & VLIB_BUFFER_NEXT_PRESENT)
986 last->current_length,
995 last->current_length += drop_string_len;
996 b0->
flags &= ~(VLIB_BUFFER_TOTAL_LENGTH_VALID);
998 last->current_length -= drop_string_len;
1016 #ifndef CLIB_MARCH_VARIANT
1055 .name =
"error-drop",
1056 .vector_size =
sizeof (
u32),
1068 .name =
"error-punt",
1069 .vector_size =
sizeof (
u32),
1080 .name =
"interface-output",
1081 .vector_size =
sizeof (
u32),
1221 .name =
"interface-output-arc-end",
1222 .vector_size =
sizeof (
u32),
1230 .arc_name =
"interface-output",
1232 .last_in_arc =
"interface-output-arc-end",
1237 .arc_name =
"interface-output",
1238 .node_name =
"span-output",
1243 .arc_name =
"interface-output",
1244 .node_name =
"ipsec-if-output",
1249 .arc_name =
"interface-output",
1250 .node_name =
"interface-output-arc-end",
1254 #ifndef CLIB_MARCH_VARIANT
void vnet_pcap_drop_trace_filter_add_del(u32 error_index, int is_add)
static u8 * format_vnet_error_trace(u8 *s, va_list *va)
@ VNET_ERROR_DISPOSITION_PUNT
vnet_interface_main_t * im
uword * sub_interface_sw_if_index_by_id
vnet_hw_if_output_node_runtime_t * output_node_thread_runtimes
u8 * format_vnet_interface_output_trace(u8 *s, va_list *va)
vnet_config_main_t config_main
#define hash_set(h, key, value)
static_always_inline int vnet_have_features(u8 arc, u32 sw_if_index)
#define count_trailing_zeros(x)
static void drop_catchup_trace(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_buffer_t *b)
#define vlib_prefetch_buffer_header(b, type)
Prefetch buffer metadata.
vnet_hw_interface_capabilities_t caps
static uword vlib_node_add_next(vlib_main_t *vm, uword node, uword next_node)
@ VNET_INTERFACE_COUNTER_TX
vlib_error_desc_t * counters_heap
vlib_buffer_copy_indices(to, tmp, n_free)
static vlib_buffer_t * vlib_get_buffer(vlib_main_t *vm, u32 buffer_index)
Translate buffer index into buffer pointer.
static_always_inline uword interface_drop_punt(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame, vnet_error_disposition_t disposition)
VNET_INTERFACE_OUTPUT_NEXT_DROP
vnet_hw_interface_flags_t flags
@ VNET_INTERFACE_OUTPUT_NEXT_TX
vlib_node_registration_t vnet_interface_output_node
(constructor) VLIB_REGISTER_NODE (vnet_interface_output_node)
vlib_error_main_t error_main
static uword hash_elts(void *v)
vlib_node_main_t node_main
@ VNET_SW_INTERFACE_FLAG_ADMIN_UP
vlib_main_t vlib_node_runtime_t vlib_frame_t * frame
static_always_inline uword vnet_interface_output_node_inline(vlib_main_t *vm, u32 sw_if_index, vlib_combined_counter_main_t *ccm, vlib_buffer_t **b, u32 config_index, u8 arc, u32 n_left, int do_tx_offloads, int arc_or_subif)
static vnet_sw_interface_t * vnet_get_sw_interface(vnet_main_t *vnm, u32 sw_if_index)
@ VNET_HW_INTERFACE_FLAG_LINK_UP
static u32 vlib_get_trace_count(vlib_main_t *vm, vlib_node_runtime_t *rt)
vlib_simple_counter_main_t * sw_if_counters
vlib_buffer_enqueue_to_next(vm, node, from,(u16 *) nexts, frame->n_vectors)
vlib_buffer_t * bufs[VLIB_FRAME_SIZE]
#define VLIB_BUFFER_DEFAULT_DATA_SIZE
vlib_combined_counter_main_t * ccm
static void pcap_add_buffer(pcap_main_t *pm, struct vlib_main_t *vm, u32 buffer_index, u32 n_bytes_in_trace)
Add buffer (vlib_buffer_t) to the trace.
static_always_inline void vnet_calc_checksums_inline(vlib_main_t *vm, vlib_buffer_t *b, int is_ip4, int is_ip6)
vnet_hw_if_output_node_runtime_t * r
static uword vlib_buffer_length_in_chain(vlib_main_t *vm, vlib_buffer_t *b)
Get length in bytes of the buffer chain.
static_always_inline void * clib_memcpy_fast(void *restrict dst, const void *restrict src, size_t n)
static void interface_trace_buffers(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame)
#define hash_unset(h, key)
vnet_sw_interface_flags_t flags
#define pool_is_free_index(P, I)
Use free bitmap to query whether given index is free.
#define vec_elt(v, i)
Get vector value at index i.
static_always_inline void enqueu_to_tx_node(vlib_main_t *vm, vlib_node_runtime_t *node, vnet_hw_interface_t *hi, u32 *from, u32 n_vectors)
#define STRUCT_OFFSET_OF(t, f)
i16 current_data
signed offset in data[], pre_data[] that we are currently processing.
static void vlib_increment_simple_counter(vlib_simple_counter_main_t *cm, u32 thread_index, u32 index, u64 increment)
Increment a simple counter.
static_always_inline void vlib_buffer_enqueue_to_single_next(vlib_main_t *vm, vlib_node_runtime_t *node, u32 *buffers, u16 next_index, u32 count)
static void vlib_buffer_advance(vlib_buffer_t *b, word l)
Advance current data pointer by the supplied (signed!) amount.
static_always_inline void vnet_interface_pcap_tx_trace(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame, int in_interface_ouput)
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
VNET_HW_INTERFACE_ADD_DEL_FUNCTION(vnet_per_buffer_interface_output_hw_interface_add_del)
vlib_error_t error
Error code for buffers to be enqueued to error handler.
#define VLIB_NODE_FN(node)
@ VNET_ERROR_DISPOSITION_DROP
clib_error_t * vnet_per_buffer_interface_output_hw_interface_add_del(vnet_main_t *vnm, u32 hw_if_index, u32 is_create)
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
static vnet_hw_interface_t * vnet_get_hw_interface(vnet_main_t *vnm, u32 hw_if_index)
vnet_main_t * vnet_get_main(void)
vlib_node_registration_t interface_drop
(constructor) VLIB_REGISTER_NODE (interface_drop)
#define VLIB_NODE_FLAG_TRACE
static void * vlib_frame_vector_args(vlib_frame_t *f)
Get pointer to frame vector data.
static_always_inline void store_tx_frame_scalar_data(vnet_hw_if_output_node_runtime_t *r, vnet_hw_if_tx_frame_t *tf)
#define static_always_inline
VNET_FEATURE_ARC_INIT(interface_output, static)
#define vlib_prefetch_buffer_with_index(vm, bi, type)
Prefetch buffer metadata by buffer index The first 64 bytes of buffer contains most header informatio...
static void pcap_drop_trace(vlib_main_t *vm, vnet_interface_main_t *im, vnet_pcap_t *pp, vlib_frame_t *f)
static heap_elt_t * last(heap_header_t *h)
uword vlib_error_drop_buffers(vlib_main_t *vm, vlib_node_runtime_t *node, u32 *buffers, u32 next_buffer_stride, u32 n_buffers, u32 next_index, u32 drop_error_node, u32 drop_error_code)
if(node->flags &VLIB_NODE_FLAG_TRACE) vnet_interface_output_trace(vm
vlib_node_registration_t interface_punt
(constructor) VLIB_REGISTER_NODE (interface_punt)
static vlib_node_t * vlib_get_node(vlib_main_t *vm, u32 i)
Get vlib node by index.
@ VNET_INTERFACE_OUTPUT_ERROR_INTERFACE_DOWN
#define VLIB_NODE_FLAG_TRACE_SUPPORTED
vnet_feature_config_main_t * cm
static_always_inline uword clib_count_equal_u32(u32 *data, uword max_count)
static_always_inline u32 clib_compress_u32(u32 *dst, u32 *src, u64 *mask, u32 n_elts)
Compress array of 32-bit elemments into destination array based on mask.
static void vlib_set_trace_count(vlib_main_t *vm, vlib_node_runtime_t *rt, u32 count)
struct _vlib_node_registration vlib_node_registration_t
vlib_combined_counter_main_t * combined_sw_if_counters
vnet_sw_interface_t * sw_interfaces
u16 current_length
Nbytes between current data and the end of this buffer.
static void * vlib_frame_scalar_args(vlib_frame_t *f)
Get pointer to frame scalar data.
u32 output_node_next_index
u16 * if_out_arc_end_next_index_by_sw_if_index
static_always_inline void vnet_interface_output_handle_offload(vlib_main_t *vm, vlib_buffer_t *b)
vnet_hw_if_tx_frame_t * tf
vlib_node_registration_t vnet_interface_output_arc_end_node
(constructor) VLIB_REGISTER_NODE (vnet_interface_output_arc_end_node)
u32 current_config_index
Used by feature subgraph arcs to visit enabled feature nodes.
vlib_node_registration_t vnet_per_buffer_interface_output_node
(constructor) VLIB_REGISTER_NODE (vnet_per_buffer_interface_output_node)
#define clib_strnlen(s, m)
vlib_get_buffers(vm, from, bufs, n_buffers)
@ VNET_INTERFACE_COUNTER_PUNT
VNET_FEATURE_INIT(span_tx, static)
#define vlib_validate_buffer_enqueue_x1(vm, node, next_index, to_next, n_left_to_next, bi0, next0)
Finish enqueueing one buffer forward in the graph.
vnet_hw_if_tx_frame_t frame
format_function_t format_vnet_sw_if_index_name
@ VNET_INTERFACE_COUNTER_TX_ERROR
description fragment has unexpected format
A collection of combined counters.
vlib_put_next_frame(vm, node, next_index, 0)
static_always_inline int vnet_is_packet_pcaped(vnet_pcap_t *pp, vlib_buffer_t *b, u32 sw_if_index)
vnet_is_packet_pcaped
static vnet_hw_interface_t * vnet_get_sup_hw_interface(vnet_main_t *vnm, u32 sw_if_index)
u64 mask[VLIB_FRAME_SIZE/64]
static void vnet_interface_output_trace(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame, uword n_buffers)
struct vnet_error_trace_t_ vnet_error_trace_t
uword * pcap_drop_filter_hash
u8 data[128 - 2 *sizeof(u32)]
static_always_inline u32 vnet_get_feature_config_index(u8 arc, u32 sw_if_index)
vlib_main_t vlib_node_runtime_t * node
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
static_always_inline vnet_feature_config_main_t * vnet_feature_get_config_main(u16 arc)
A collection of simple counters.
void * vlib_add_trace(vlib_main_t *vm, vlib_node_runtime_t *r, vlib_buffer_t *b, u32 n_data_bytes)
#define VNET_FEATURES(...)
static void * vlib_buffer_get_current(vlib_buffer_t *b)
Get pointer to current data to process.
vnet_interface_output_runtime_t * rt
static void * vnet_get_config_data(vnet_config_main_t *cm, u32 *config_index, u32 *next_index, u32 n_data_bytes)
void vnet_set_interface_output_node(vnet_main_t *vnm, u32 hw_if_index, u32 node_index)
Set interface output node - for interface registered without its output/tx nodes created because its ...
u16 nexts[VLIB_FRAME_SIZE]
#define vlib_validate_buffer_enqueue_x2(vm, node, next_index, to_next, n_left_to_next, bi0, bi1, next0, next1)
Finish enqueueing two buffers forward in the graph.
u8 pad[3]
log2 (size of the packing page block)
clib_mask_compare_u32(swif, sw_if_indices, mask, frame->n_vectors)
#define VNET_HW_INTERFACE_CAP_SUPPORTS_TX_CKSUM
static __clib_warn_unused_result int vlib_trace_buffer(vlib_main_t *vm, vlib_node_runtime_t *r, u32 next_index, vlib_buffer_t *b, int follow_chain)
#define vlib_get_next_frame(vm, node, next_index, vectors, n_vectors_left)
Get pointer to next frame vector data by (vlib_node_runtime_t, next_index).
#define hash_create(elts, value_bytes)
u8 output_feature_arc_index
u32 sw_if_indices[VLIB_FRAME_SIZE]
node VNET_INTERFACE_OUTPUT_ERROR_INTERFACE_DELETED
@ VNET_INTERFACE_COUNTER_DROP
u64 used_elts[VLIB_FRAME_SIZE/64]
vnet_interface_main_t interface_main
vlib_increment_combined_counter(ccm, ti, sw_if_index, n_buffers, n_bytes)
vlib_frame_t * vlib_get_next_frame_internal(vlib_main_t *vm, vlib_node_runtime_t *node, u32 next_index, u32 allocate_new_next_frame)
u32 flags
buffer flags: VLIB_BUFFER_FREE_LIST_INDEX_MASK: bits used to store free list index,...
format_function_t format_vnet_sw_interface_name
@ VNET_ERROR_N_DISPOSITION
VLIB buffer representation.
#define VLIB_REGISTER_NODE(x,...)
vl_api_wireguard_peer_flags_t flags