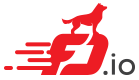 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
59 u32 next0 = NSH_NODE_NEXT_DROP, next1 = NSH_NODE_NEXT_DROP;
60 uword * entry0, *entry1;
61 nsh_base_header_t * hdr0 = 0, *hdr1 = 0;
62 u32 header_len0 = 0, header_len1 = 0;
63 u32 nsp_nsi0, nsp_nsi1;
96 nsp_nsi0 = hdr0->nsp_nsi;
97 header_len0 = hdr0->length * 4;
100 nsp_nsi1 = hdr1->nsp_nsi;
101 header_len1 = hdr1->length * 4;
107 error0 = NSH_NODE_ERROR_NO_MAPPING;
125 error0 = NSH_NODE_ERROR_INVALID_OPTIONS;
139 error0 = NSH_NODE_ERROR_NO_ENTRY;
143 trace0: b0->
error = error0 ?
node->errors[error0] : 0;
155 error1 = NSH_NODE_ERROR_NO_MAPPING;
163 next1 = map1->next_node;
173 error1 = NSH_NODE_ERROR_INVALID_OPTIONS;
184 entry1 =
hash_get_mem(
nm->nsh_entry_by_key, &map1->mapped_nsp_nsi);
187 error1 = NSH_NODE_ERROR_NO_ENTRY;
192 trace1: b1->
error = error1 ?
node->errors[error1] : 0;
201 n_left_to_next, bi0, bi1, next0, next1);
209 u32 next0 = NSH_NODE_NEXT_DROP;
211 nsh_base_header_t * hdr0 = 0;
228 nsp_nsi0 = hdr0->nsp_nsi;
229 header_len0 = hdr0->length * 4;
235 error0 = NSH_NODE_ERROR_NO_MAPPING;
254 error0 = NSH_NODE_ERROR_INVALID_OPTIONS;
268 error0 = NSH_NODE_ERROR_NO_ENTRY;
272 trace00: b0->
error = error0 ?
node->errors[error0] : 0;
281 n_left_to_next, bi0, next0);
309 #define _(sym,string) string,
317 .vector_size =
sizeof (
u32),
328 #define _(s,n) [NSH_NODE_NEXT_##s] = n,
#define vlib_prefetch_buffer_header(b, type)
Prefetch buffer metadata.
nat44_ei_hairpin_src_next_t next_index
static vlib_buffer_t * vlib_get_buffer(vlib_main_t *vm, u32 buffer_index)
Translate buffer index into buffer pointer.
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
@ VLIB_NODE_TYPE_INTERNAL
vlib_main_t vlib_node_runtime_t * node
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
vlib_main_t vlib_node_runtime_t vlib_frame_t * from_frame
u8 * format_nsh_node_map_trace(u8 *s, va_list *args)
u8 * format_nsh_pop_header(u8 *s, va_list *args)
static_always_inline void * clib_memcpy_fast(void *restrict dst, const void *restrict src, size_t n)
#define CLIB_PREFETCH(addr, size, type)
static void vlib_buffer_advance(vlib_buffer_t *b, word l)
Advance current data pointer by the supplied (signed!) amount.
vlib_error_t error
Error code for buffers to be enqueued to error handler.
#define VLIB_NODE_FN(node)
nsh_option_map_t * nsh_md2_lookup_option(u16 class, u8 type)
static void * vlib_frame_vector_args(vlib_frame_t *f)
Get pointer to frame vector data.
vlib_node_registration_t nsh_pop_node
(constructor) VLIB_REGISTER_NODE (nsh_pop_node)
static char * nsh_pop_node_error_strings[]
#define CLIB_CACHE_LINE_BYTES
struct _vlib_node_registration vlib_node_registration_t
#define hash_get_mem(h, key)
#define vlib_validate_buffer_enqueue_x1(vm, node, next_index, to_next, n_left_to_next, bi0, next0)
Finish enqueueing one buffer forward in the graph.
static uword nsh_pop_inline(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *from_frame)
vlib_put_next_frame(vm, node, next_index, 0)
void * vlib_add_trace(vlib_main_t *vm, vlib_node_runtime_t *r, vlib_buffer_t *b, u32 n_data_bytes)
static void * vlib_buffer_get_current(vlib_buffer_t *b)
Get pointer to current data to process.
u8 * format_nsh_header(u8 *s, va_list *args)
u32 mapped_nsp_nsi
Key for nsh_header_t entry to map to.
#define foreach_nsh_node_next
#define foreach_nsh_node_error
#define vlib_validate_buffer_enqueue_x2(vm, node, next_index, to_next, n_left_to_next, bi0, bi1, next0, next1)
Finish enqueueing two buffers forward in the graph.
u8 * format_nsh_pop_node_map_trace(u8 *s, va_list *args)
#define vlib_get_next_frame(vm, node, next_index, vectors, n_vectors_left)
Get pointer to next frame vector data by (vlib_node_runtime_t, next_index).
vl_api_fib_path_type_t type
u32 flags
buffer flags: VLIB_BUFFER_FREE_LIST_INDEX_MASK: bits used to store free list index,...
VLIB buffer representation.
#define VLIB_REGISTER_NODE(x,...)