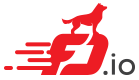 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
39 u32 *result = va_arg (*args,
u32 *);
59 u32 nsh_action = va_arg (*args,
u32);
70 return format (s,
"unknown %d", nsh_action);
80 s =
format (s,
"nsh entry nsp: %d nsi: %d ",
83 s =
format (s,
"maps to nsp: %d nsi: %d ",
89 switch (
map->next_node)
91 case NSH_NODE_NEXT_ENCAP_GRE4:
93 s =
format (s,
"encapped by GRE4 intf: %d",
map->sw_if_index);
96 case NSH_NODE_NEXT_ENCAP_GRE6:
98 s =
format (s,
"encapped by GRE6 intf: %d",
map->sw_if_index);
101 case NSH_NODE_NEXT_ENCAP_VXLANGPE:
103 s =
format (s,
"encapped by VXLAN GPE intf: %d",
map->sw_if_index);
106 case NSH_NODE_NEXT_ENCAP_VXLAN4:
108 s =
format (s,
"encapped by VXLAN4 intf: %d",
map->sw_if_index);
111 case NSH_NODE_NEXT_ENCAP_VXLAN6:
113 s =
format (s,
"encapped by VXLAN6 intf: %d",
map->sw_if_index);
116 case NSH_NODE_NEXT_DECAP_ETH_INPUT:
118 s =
format (s,
"encap-none");
121 case NSH_NODE_NEXT_ENCAP_LISP_GPE:
123 s =
format (s,
"encapped by LISP GPE intf: %d",
map->sw_if_index);
126 case NSH_NODE_NEXT_ENCAP_ETHERNET:
128 s =
format (s,
"encapped by Ethernet intf: %d",
map->sw_if_index);
132 s =
format (s,
"only GRE and VXLANGPE support in this rev");
167 u32 nsp, nsi, mapped_nsp, mapped_nsi, nsh_action;
168 int nsp_set = 0, nsi_set = 0, mapped_nsp_set = 0, mapped_nsi_set = 0;
169 int nsh_action_set = 0;
173 u32 rx_sw_if_index = ~0;
186 else if (
unformat (line_input,
"nsp %d", &nsp))
188 else if (
unformat (line_input,
"nsi %d", &nsi))
190 else if (
unformat (line_input,
"mapped-nsp %d", &mapped_nsp))
192 else if (
unformat (line_input,
"mapped-nsi %d", &mapped_nsi))
198 next_node = NSH_NODE_NEXT_ENCAP_GRE4;
200 next_node = NSH_NODE_NEXT_ENCAP_GRE6;
202 next_node = NSH_NODE_NEXT_ENCAP_VXLANGPE;
204 next_node = NSH_NODE_NEXT_ENCAP_LISP_GPE;
206 next_node = NSH_NODE_NEXT_ENCAP_VXLAN4;
208 next_node = NSH_NODE_NEXT_ENCAP_VXLAN6;
211 next_node = NSH_NODE_NEXT_ENCAP_ETHERNET;
216 (line_input,
"encap-none %d %d", &
sw_if_index, &rx_sw_if_index))
217 next_node = NSH_NODE_NEXT_DECAP_ETH_INPUT;
225 if (nsp_set == 0 || nsi_set == 0)
228 if (mapped_nsp_set == 0 || mapped_nsi_set == 0)
230 "mapped-nsp mapped-nsi pair required. Key: for NSH entry");
232 if (nsh_action_set == 0)
237 "must specific action: [encap-gre-intf <nn> | encap-vxlan-gpe-intf <nn> | encap-lisp-gpe-intf <nn> | encap-none <tx_sw_if_index> <rx_sw_if_index>]");
244 a->map.mapped_nsp_nsi = (mapped_nsp <<
NSH_NSP_SHIFT) | mapped_nsi;
245 a->map.nsh_action = nsh_action;
247 a->map.rx_sw_if_index = rx_sw_if_index;
248 a->map.next_node = next_node;
249 a->map.adj_index = adj_index;
259 "mapping already exists. Remove it first.");
268 if ((
a->map.next_node == NSH_NODE_NEXT_ENCAP_VXLAN4)
269 | (
a->map.next_node == NSH_NODE_NEXT_ENCAP_VXLAN6))
279 "nsh-proxy-session already exists. Remove it first.");
286 (0,
"nsh_add_del_proxy_session() returned %d",
rv);
295 .path =
"create nsh map",
297 "create nsh map nsp <nn> nsi <nn> [del] mapped-nsp <nn> mapped-nsi <nn> nsh_action [swap|push|pop] "
298 "[encap-gre4-intf <nn> | encap-gre4-intf <nn> | encap-vxlan-gpe-intf <nn> | encap-lisp-gpe-intf <nn> "
299 " encap-vxlan4-intf <nn> | encap-vxlan6-intf <nn>| encap-eth-intf <nn> | encap-none]\n",
327 .path =
"show nsh map",
346 u8 next_protocol = 1;
357 nsh_tlv_header_t tlv_header;
358 u8 cur_len = 0, tlvs_len = 0;
367 u8 has_ioam_trace_option = 0;
377 else if (
unformat (line_input,
"version %d", &
tmp))
378 ver_o_c |= (
tmp & 3) << 6;
380 ver_o_c |= (
tmp & 1) << 5;
382 ver_o_c |= (
tmp & 1) << 4;
385 else if (
unformat (line_input,
"md-type %d", &
tmp))
387 else if (
unformat (line_input,
"next-ip4"))
389 else if (
unformat (line_input,
"next-ip6"))
391 else if (
unformat (line_input,
"next-ethernet"))
393 else if (
unformat (line_input,
"c1 %d", &c1))
395 else if (
unformat (line_input,
"c2 %d", &c2))
397 else if (
unformat (line_input,
"c3 %d", &c3))
399 else if (
unformat (line_input,
"c4 %d", &c4))
401 else if (
unformat (line_input,
"nsp %d", &nsp))
403 else if (
unformat (line_input,
"nsi %d", &nsi))
405 else if (
unformat (line_input,
"tlv-ioam-trace"))
406 has_ioam_trace_option = 1;
420 if (md_type == 1 && has_ioam_trace_option == 1)
423 nsp_nsi = (nsp << 8) | nsi;
430 a->nsh_entry.md.md1_data.c1 = c1;
431 a->nsh_entry.md.md1_data.c2 = c2;
432 a->nsh_entry.md.md1_data.c3 = c3;
433 a->nsh_entry.md.md1_data.c4 = c4;
434 length = (
sizeof (nsh_base_header_t) +
sizeof (nsh_md1_data_t)) >> 2;
436 else if (md_type == 2)
438 length =
sizeof (nsh_base_header_t) >> 2;
443 a->nsh_entry.tlvs_data =
data;
446 if (has_ioam_trace_option)
453 if (nsh_option == NULL)
456 if (
nm->add_options[nsh_option->
option_id] != NULL)
458 if (0 !=
nm->add_options[nsh_option->
option_id] ((
u8 *) current,
465 nm->options_size[nsh_option->
option_id] = option_size;
467 option_size = (((option_size + 3) >> 2) << 2);
469 cur_len += option_size;
470 current =
data + option_size;
475 a->nsh_entry.tlvs_len = cur_len;
480 #define _(x) a->nsh_entry.nsh_base.x = x;
499 .path =
"create nsh entry",
501 "create nsh entry {nsp <nn> nsi <nn>} [ttl <nn>] [md-type <nn>]"
502 " [c1 <nn> c2 <nn> c3 <nn> c4 <nn>] [tlv-ioam-trace] [del]\n",
517 u8 *header = va_arg (*args,
u8 *);
518 nsh_base_header_t *nsh_base = (nsh_base_header_t *) header;
519 nsh_md1_data_t *nsh_md1 = (nsh_md1_data_t *) (nsh_base + 1);
524 -
sizeof (nsh_base_header_t)));
526 s =
format (s,
"nsh ver %d ", (nsh_base->ver_o_c >> 6));
536 s =
format (s,
"len %d (%d bytes) md_type %d next_protocol %d\n",
539 nsh_base->md_type, nsh_base->next_protocol);
541 s =
format (s,
" service path %d service index %d\n",
542 (clib_net_to_host_u32 (nsh_base->nsp_nsi) >>
NSH_NSP_SHIFT) &
544 clib_net_to_host_u32 (nsh_base->nsp_nsi) &
NSH_NSI_MASK);
546 if (nsh_base->md_type == 1)
548 s =
format (s,
" c1 %d c2 %d c3 %d c4 %d\n",
549 clib_net_to_host_u32 (nsh_md1->c1),
550 clib_net_to_host_u32 (nsh_md1->c2),
551 clib_net_to_host_u32 (nsh_md1->c3),
552 clib_net_to_host_u32 (nsh_md1->c4));
554 else if (nsh_base->md_type == 2)
556 s =
format (s,
" Supported TLVs: \n");
559 while (opt0 < limit0)
562 if (nsh_option != NULL)
571 format (s,
"\n untraced option %d length %d",
572 opt0->type, opt0->length);
578 format (s,
"\n unrecognized option %d length %d",
579 opt0->type, opt0->length);
583 option_len = ((opt0->length + 3) >> 2) << 2;
626 .path =
"show nsh entry",
static clib_error_t * show_nsh_entry_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
u8 * format_nsh_pop_node_map_trace(u8 *s, va_list *args)
ip_adjacency_t * adj_pool
The global adjacency pool.
static clib_error_t * nsh_add_del_map_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
CLI command for NSH map.
Note: rewrite and rewrite_size used to support varied nsh header.
u32 adj_get_sw_if_index(adj_index_t ai)
Return the sw interface index of the adjacency.
int nsh_add_del_entry(nsh_add_del_entry_args_t *a, u32 *entry_indexp)
Action function for adding an NSH entry nsh_add_del_entry_args_t *a: host order.
static vlib_cli_command_t create_nsh_map_command
(constructor) VLIB_CLI_COMMAND (create_nsh_map_command)
#define foreach_copy_nsh_base_hdr_field
u8 * format_nsh_header(u8 *s, va_list *args)
vlib_main_t vlib_node_runtime_t * node
static vlib_cli_command_t show_nsh_map_command
(constructor) VLIB_CLI_COMMAND (show_nsh_map_command)
#define clib_error_return(e, args...)
static clib_error_t * show_nsh_map_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
CLI command for showing the mapping between NSH entries.
static vlib_cli_command_t create_nsh_entry_command
(constructor) VLIB_CLI_COMMAND (create_nsh_entry_command)
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
nsh_option_map_t * nsh_md2_lookup_option(u16 class, u8 type)
u8 * format_nsh_map(u8 *s, va_list *args)
#define pool_foreach(VAR, POOL)
Iterate through pool.
nsh_tlv_header_t nsh_md2_data_t
#define NSH_MD2_IOAM_OPTION_TYPE_TRACE
#define vec_validate_aligned(V, I, A)
Make sure vector is long enough for given index (no header, specified alignment)
int nsh_add_del_map(nsh_add_del_map_args_t *a, u32 *map_indexp)
Action function to add or del an nsh map.
#define VLIB_CLI_COMMAND(x,...)
u8 * format_nsh_pop_header(u8 *s, va_list *args)
#define CLIB_CACHE_LINE_BYTES
void vlib_cli_output(vlib_main_t *vm, char *fmt,...)
static clib_error_t * nsh_add_del_entry_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
CLI command for adding NSH entry.
#define vec_free(V)
Free vector's memory (no header).
static vlib_cli_command_t show_nsh_entry_command
(constructor) VLIB_CLI_COMMAND (show_nsh_entry_command)
u8 * format_nsh_node_map_trace(u8 *s, va_list *args)
#define pool_foreach_index(i, v)
description fragment has unexpected format
int nsh_add_del_proxy_session(nsh_add_del_map_args_t *a)
Action function to add or del an nsh-proxy-session.
u8 * rewrite
Rewrite string.
static uword pool_elts(void *v)
Number of active elements in a pool.
u32 adj_index_t
An index for adjacencies.
#define NSH_MD2_IOAM_CLASS
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
static uword unformat_nsh_action(unformat_input_t *input, va_list *args)
static adj_index_t nsh_get_adj_by_sw_if_index(u32 sw_if_index)
static u8 * format_nsh_action(u8 *s, va_list *args)
vl_api_interface_index_t sw_if_index