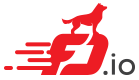 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
20 #include <vat/plugin.h>
28 #define PLUGIN_LOG_DBG(...) \
29 do {vlib_log_debug (vat_builtin_logger, __VA_ARGS__);} while(0)
30 #define PLUGIN_LOG_ERR(...) \
31 do {vlib_log_err (vat_builtin_logger, __VA_ARGS__);} while(0)
32 #define PLUGIN_LOG_NOTICE(...) \
33 do {vlib_log_notice (vat_builtin_logger, __VA_ARGS__);} while(0)
38 void *handle, *register_handle;
42 handle = dlopen ((
char *) pi->
filename, RTLD_LAZY);
57 register_handle = dlsym (pi->
handle,
"vat_plugin_register");
58 if (register_handle == 0)
67 error = (*fp) (pm->vat_main);
115 struct dirent *entry;
126 dp = opendir ((
char *) plugin_path[
i]);
131 while ((entry = readdir (dp)))
144 file_name =
format (0,
"%s/%s%c", plugin_path[
i], entry->d_name, 0);
145 plugin_name =
format (0,
"%s%c", entry->d_name, 0);
148 if (stat ((
char *) file_name, &statb) < 0)
157 if (!S_ISREG (statb.st_mode))
164 pi->
name = plugin_name;
190 #define QUOTE(x) QUOTE_(x)
203 u8 *plugin_name_filter;
215 if (plugin_name_filter)
static int load_one_vat_plugin(plugin_main_t *pm, plugin_info_t *pi)
plugin_main_t vat_plugin_main
plugin_info_t * plugin_info
static vlib_log_class_t vat_builtin_logger
#define hash_create_string(elts, value_bytes)
#define hash_set_mem(h, key, value)
uword * plugin_by_name_hash
static u8 ** split_plugin_path(plugin_main_t *pm)
__clib_export u8 * format_clib_error(u8 *s, va_list *va)
description IKE_SA_INIT ignore(IKE SA already auth)"
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
#define vec_add2(V, P, N)
Add N elements to end of vector V, return pointer to new elements in P.
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
int vat_load_new_plugins(plugin_main_t *pm)
vlib_log_class_t vlib_log_register_class_rate_limit(char *class, char *subclass, u32 limit)
int vat_plugin_init(vat_main_t *vam)
u8 * vlib_get_vat_plugin_name_filter(void)
char * vat_plugin_name_filter
#define PLUGIN_LOG_ERR(...)
u8 * vlib_get_vat_plugin_path(void)
#define hash_get_mem(h, key)
#define vec_free(V)
Free vector's memory (no header).
description fragment has unexpected format
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
#define clib_error_free(e)
#define PLUGIN_LOG_NOTICE(...)