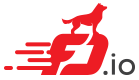 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
22 #include <sys/types.h>
23 #include <sys/socket.h>
31 #include <stdatomic.h>
55 struct msghdr msg = { 0 };
59 struct iovec io = {.iov_base = iobuf,.iov_len =
sizeof (iobuf) };
62 char buf[CMSG_SPACE (
sizeof (fd))];
67 msg.msg_control = u.buf;
68 msg.msg_controllen =
sizeof (u.buf);
71 if ((
size = recvmsg (sock, &msg, 0)) < 0)
73 perror (
"recvmsg failed");
76 cmsg = CMSG_FIRSTHDR (&msg);
77 if (cmsg && cmsg->cmsg_level == SOL_SOCKET && cmsg->cmsg_type == SCM_RIGHTS)
79 memmove (&fd, CMSG_DATA (cmsg),
sizeof (fd));
99 if ((sock = socket (AF_UNIX, SOCK_SEQPACKET, 0)) < 0)
101 perror (
"Stat client couldn't open socket");
105 struct sockaddr_un
un = { 0 };
106 un.sun_family = AF_UNIX;
107 strncpy ((
char *)
un.sun_path, socket_name, sizeof (
un.sun_path) - 1);
108 if (connect (sock, (
struct sockaddr *) &
un,
sizeof (
struct sockaddr_un)) <
115 if ((mfd =
recv_fd (sock)) < 0)
118 fprintf (stderr,
"Receiving file descriptor failed\n");
125 struct stat st = { 0 };
127 if (fstat (mfd, &st) == -1)
130 perror (
"mmap fstat failed");
134 mmap (NULL, st.st_size, PROT_READ, MAP_SHARED, mfd, 0)) == MAP_FAILED)
137 perror (
"mmap map failed");
195 #define stat_vec_dup(S,V) \
197 __typeof__ ((V)[0]) * _v(v) = 0; \
198 if (V && ((void *)V > (void *)S->shared_header) && \
199 (((void*)V + vec_bytes(V)) < \
200 ((void *)S->shared_header + S->memory_size))) \
201 _v(v) = vec_dup(V); \
233 uint64_t *error_vector;
309 fprintf (stderr,
"Unknown type: %d\n", ep->
type);
323 for (j = 0; j <
vec_len (res[
i].simple_counter_vec); j++)
328 for (j = 0; j <
vec_len (res[
i].combined_counter_vec); j++)
329 vec_free (res[
i].combined_counter_vec[j]);
333 for (j = 0; j <
vec_len (res[
i].name_vector); j++)
356 regex_t regex[
vec_len (patterns)];
361 int rv = regcomp (®ex[
i], (
const char *) patterns[
i], 0);
364 fprintf (stderr,
"Could not compile regex %s\n", patterns[
i]);
373 for (j = 0; j <
vec_len (counter_vec); j++)
377 int rv = regexec (®ex[
i], counter_vec[j].
name, 0, NULL, 0);
436 fprintf (stderr,
"Epoch changed while reading, invalid results\n");
466 size_t len = strlen (
string);
470 return string_vector;
519 return strdup (ep->
name);
stat_segment_directory_entry_t * directory_vector
@ STAT_DIR_TYPE_COUNTER_VECTOR_COMBINED
void stat_segment_vec_free(void *vec)
counter_t ** simple_counter_vec
static vlib_counter_t * stat_vec_combined_init(vlib_counter_t c)
uint8_t ** stat_segment_string_vector(uint8_t **string_vector, const char *string)
@ STAT_DIR_TYPE_NAME_VECTOR
int stat_segment_connect_r(const char *socket_name, stat_client_main_t *sm)
void stat_segment_disconnect_r(stat_client_main_t *sm)
@ STAT_DIR_TYPE_COUNTER_VECTOR_SIMPLE
char * stat_segment_index_to_name(uint32_t index)
vl_api_ikev2_sa_stats_t stats
static int stat_segment_access_start(stat_segment_access_t *sa, stat_client_main_t *sm)
uint32_t * stat_segment_ls(uint8_t **patterns)
static bool stat_segment_access_end(stat_segment_access_t *sa, stat_client_main_t *sm)
uint64_t stat_segment_version(void)
void stat_segment_disconnect(void)
char * stat_segment_index_to_name_r(uint32_t index, stat_client_main_t *sm)
uint64_t stat_segment_version_r(stat_client_main_t *sm)
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
void stat_segment_data_free(stat_segment_data_t *res)
static int recv_fd(int sock)
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
int stat_segment_connect(const char *socket_name)
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
int stat_segment_vec_len(void *vec)
Combined counter to hold both packets and byte differences.
stat_client_main_t * stat_client_get(void)
uint64_t counter_t
64bit counters
stat_segment_shared_header_t * shared_header
uint32_t * stat_segment_ls_r(uint8_t **patterns, stat_client_main_t *sm)
static stat_segment_directory_entry_t * get_stat_vector_r(stat_client_main_t *sm)
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
static counter_t * stat_vec_simple_init(counter_t c)
static stat_segment_data_t copy_data(stat_segment_directory_entry_t *ep, u32 index2, char *name, stat_client_main_t *sm)
#define vec_validate_init_c_string(V, S, L)
Make a vector containing a NULL terminated c-string.
#define vec_free(V)
Free vector's memory (no header).
double stat_segment_heartbeat_r(stat_client_main_t *sm)
vlib_counter_t ** combined_counter_vec
stat_directory_type_t type
@ STAT_DIR_TYPE_SCALAR_INDEX
stat_client_main_t stat_client_main
#define stat_vec_dup(S, V)
static void * stat_segment_adjust(stat_client_main_t *sm, void *data)
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
stat_segment_data_t * stat_segment_dump_entry_r(uint32_t index, stat_client_main_t *sm)
double stat_segment_heartbeat(void)
void * malloc(size_t size)
stat_segment_data_t * stat_segment_dump_r(uint32_t *stats, stat_client_main_t *sm)
stat_segment_data_t * stat_segment_dump(uint32_t *stats)
stat_segment_data_t * stat_segment_dump_entry(uint32_t index)
void stat_client_free(stat_client_main_t *sm)
stat_directory_type_t type
@ STAT_DIR_TYPE_ERROR_INDEX
vl_api_fib_path_type_t type
vl_api_address_union_t un