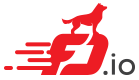 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
20 #define foreach_ipsec_protect_flags \
22 _ (ENCAPED, 2, "encapped") \
29 #define _(a,b,c) IPSEC_PROTECT_##a = b,
74 (*ip).as_u32 = k->
key >> 32;
105 #define ITP_MAX_N_SA_IN 4
130 #define FOR_EACH_IPSEC_PROTECT_INPUT_SAI(_itp, _sai, body) \
133 for (__ii = 0; __ii < _itp->itp_n_sa_in; __ii++) { \
134 _sai = itp->itp_in_sas[__ii]; \
138 #define FOR_EACH_IPSEC_PROTECT_INPUT_SA(_itp, _sa, body) \
141 for (__ii = 0; __ii < _itp->itp_n_sa_in; __ii++) { \
142 _sa = ipsec_sa_get(itp->itp_in_sas[__ii]); \
ipsec_tun_lkup_result_t value
void ipsec_tun_table_init(ip_address_family_t af, uword table_size, u32 n_buckets)
struct ipsec_ep_t_ ipsec_ep_t
static void ipsec4_tunnel_mk_key(ipsec4_tunnel_kv_t *k, const ip4_address_t *ip, u32 spi)
index_t * ipsec_tun_protect_sa_by_adj_index
Adj index to TX SA mapping.
u8 * format_ipsec_tun_protect(u8 *s, va_list *args)
ipsec_protect_flags_t itp_flags
STATIC_ASSERT_OFFSET_OF(ipsec4_tunnel_kv_t, value, STRUCT_OFFSET_OF(clib_bihash_kv_8_16_t, value))
static index_t ipsec_tun_protect_get_sa_out(adj_index_t ai)
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
int ipsec_tun_protect_update(u32 sw_if_index, const ip_address_t *nh, u32 sa_out, u32 *sa_ins)
STATIC_ASSERT_SIZEOF(ipsec4_tunnel_kv_t, sizeof(clib_bihash_kv_8_16_t))
void ipsec_tun_protect_walk(ipsec_tun_protect_walk_cb_t fn, void *cttx)
void ipsec_tun_register_nodes(ip_address_family_t af)
ipsec_tun_protect_t * ipsec_tun_protect_pool
Pool of tunnel protection objects.
void ipsec_tun_unregister_nodes(ip_address_family_t af)
CLIB_CACHE_LINE_ALIGN_MARK(cacheline0)
void ipsec_tun_protect_walk_itf(u32 sw_if_index, ipsec_tun_protect_walk_cb_t fn, void *cttx)
u8 * format_ipsec6_tunnel_kv(u8 *s, va_list *args)
#define foreach_ipsec_protect_flags
#define STRUCT_OFFSET_OF(t, f)
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
u8 * format_ipsec4_tunnel_kv(u8 *s, va_list *args)
struct ipsec_tun_protect_t_ ipsec_tun_protect_t
u32 index_t
A Data-Path Object is an object that represents actions that are applied to packets are they are swit...
walk_rc_t(* ipsec_tun_protect_walk_cb_t)(index_t itpi, void *arg)
int ipsec_tun_protect_del(u32 sw_if_index, const ip_address_t *nh)
result of a lookup in the protection bihash
static void ipsec4_tunnel_extract_key(const ipsec4_tunnel_kv_t *k, ip4_address_t *ip, u32 *spi)
struct ipsec_tun_lkup_result_t_ ipsec_tun_lkup_result_t
result of a lookup in the protection bihash
struct ipsec4_tunnel_kv_t ipsec4_tunnel_kv_t
struct ipsec6_tunnel_kv_t_ ipsec6_tunnel_kv_t
8 octet key, 8 octet key value pair
vl_api_address_family_t af
index_t itp_in_sas[ITP_MAX_N_SA_IN]
u32 adj_index_t
An index for adjacencies.
struct ipsec6_tunnel_kv_t_::@461 key
enum ipsec_protect_flags_t_ ipsec_protect_flags_t
static ipsec_tun_protect_t * ipsec_tun_protect_get(u32 index)
u8 * format_ipsec_tun_protect_index(u8 *s, va_list *args)
#define INDEX_INVALID
Invalid index - used when no index is known blazoned capitals INVALID speak volumes where ~0 does not...
vl_api_interface_index_t sw_if_index
ipsec_tun_lkup_result_t value
enum walk_rc_t_ walk_rc_t
Walk return code.
ipsec_protect_flags_t flags
u8 * format_ipsec_tun_protect_flags(u8 *s, va_list *args)
enum ip_address_family_t_ ip_address_family_t