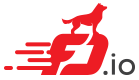 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
20 #include <vpp/app/version.h>
21 #include <linux/limits.h>
22 #include <sys/ioctl.h>
24 #include <perfmon/perfmon.h>
26 #include <linux/perf_event.h>
28 #define foreach_perf_sw_counter \
29 _ (CONTEXT_SWITCHES, "context-switches") \
30 _ (PAGE_FAULTS_MIN, "page-faults-minor") \
31 _ (PAGE_FAULTS_MAJ, "page-faults-major")
42 [n] = { .type = PERF_TYPE_SOFTWARE, .config = PERF_COUNT_SW_##n, .name = s },
49 .description =
"Linux kernel performance counters",
58 int row = va_arg (*args,
int);
59 f64 t = (
f64)
r->time_running * 1e-9;
64 s =
format (s,
"%9.2f", t);
68 s =
format (s,
"%9.2f", (
f64)
r->value[0] / t);
75 .name =
"context-switches",
76 .description =
"per-thread context switches",
79 .events[0] = CONTEXT_SWITCHES,
89 int row = va_arg (*args,
int);
90 f64 t = (
f64)
r->time_running * 1e-9;
95 s =
format (s,
"%9.2f", t);
99 s =
format (s,
"%9.2f", (
f64)
r->value[0] / t);
103 s =
format (s,
"%9.2f", (
f64)
r->value[1] / t);
110 .name =
"page-faults",
111 .description =
"per-thread page faults",
114 .events[0] = PAGE_FAULTS_MIN,
115 .events[1] = PAGE_FAULTS_MAJ,
119 "MajorPageFaults/Sec"),
#define foreach_perf_sw_counter
static u8 * format_page_faults(u8 *s, va_list *args)
vnet_hw_if_output_node_runtime_t * r
@ PERFMON_BUNDLE_TYPE_THREAD
#define PERFMON_STRINGS(...)
PERFMON_REGISTER_BUNDLE(context_switches)
static perfmon_event_t events[]
static u8 * format_context_switches(u8 *s, va_list *args)
description fragment has unexpected format
PERFMON_REGISTER_SOURCE(linux)