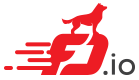 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
19 #ifndef __IP4_FIB_HASH_H__
20 #define __IP4_FIB_HASH_H__
void ip4_fib_hash_table_entry_insert(ip4_fib_hash_t *fib, const ip4_address_t *addr, u32 len, fib_node_index_t fib_entry_index)
void ip4_fib_hash_table_walk(ip4_fib_hash_t *fib, fib_table_walk_fn_t fn, void *ctx)
Walk all entries in a FIB table N.B: This is NOT safe to deletes.
index_t ip4_fib_hash_table_lookup_lb(const ip4_fib_hash_t *fib, const ip4_address_t *addr)
void ip4_fib_hash_table_sub_tree_walk(ip4_fib_hash_t *fib, const fib_prefix_t *root, fib_table_walk_fn_t fn, void *ctx)
Walk all entries in a sub-tree of the FIB table N.B: This is NOT safe to deletes.
void ip4_fib_hash_table_init(ip4_fib_hash_t *fib)
fib_node_index_t ip4_fib_hash_table_lookup_exact_match(const ip4_fib_hash_t *fib, const ip4_address_t *addr, u32 len)
void ip4_fib_hash_table_entry_remove(ip4_fib_hash_t *fib, const ip4_address_t *addr, u32 len)
fib_node_index_t ip4_fib_hash_table_lookup(const ip4_fib_hash_t *fib, const ip4_address_t *addr, u32 len)
struct ip4_fib_hash_t_ ip4_fib_hash_t
The IPv4 FIB Hash table.
u32 index_t
A Data-Path Object is an object that represents actions that are applied to packets are they are swit...
u32 fib_node_index_t
A typedef of a node index.
void ip4_fib_hash_table_destroy(ip4_fib_hash_t *fib)
fib_table_walk_rc_t(* fib_table_walk_fn_t)(fib_node_index_t fei, void *ctx)
Call back function when walking entries in a FIB table.
uword * fib_entry_by_dst_address[33]
Aggregate type for a prefix.