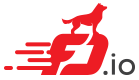 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
30 uword * hash, * result;
78 uword * hash, * result;
82 for (mask_len =
len; mask_len >= 0; mask_len--)
102 uword * hash, * result;
109 if (NULL == result) {
133 uword * hash, * result;
204 const fib_prefix_t *sub_tree;
210 vec_foreach(sub_tree, sub_trees)
212 if (ip4_destination_matches_route(&ip4_main,
214 &sub_tree->fp_addr.ip4,
226 case FIB_TABLE_WALK_CONTINUE:
228 case FIB_TABLE_WALK_SUB_TREE_STOP: {
230 .fp_proto = FIB_PROTOCOL_IP4,
234 vec_add1(sub_trees, pfx);
index_t dpoi_index
the index of objects of that type
#define hash_set(h, key, value)
index_t ip4_fib_hash_table_lookup_lb(const ip4_fib_hash_t *fib, const ip4_address_t *addr)
void ip4_fib_hash_table_entry_remove(ip4_fib_hash_t *fib, const ip4_address_t *addr, u32 len)
ip4_main_t ip4_main
Global ip4 main structure.
#define HASH_FLAG_NO_AUTO_SHRINK
#define hash_foreach_pair(p, v, body)
Iterate over hash pairs.
#define FIB_NODE_INDEX_INVALID
u16 fp_len
The mask length.
void ip4_fib_hash_table_entry_insert(ip4_fib_hash_t *fib, const ip4_address_t *addr, u32 len, fib_node_index_t fib_entry_index)
void ip4_fib_hash_table_sub_tree_walk(ip4_fib_hash_t *fib, const fib_prefix_t *root, fib_table_walk_fn_t fn, void *ctx)
Walk all entries in a sub-tree of the FIB table N.B: This is NOT safe to deletes.
fib_node_index_t ip4_fib_hash_table_lookup_exact_match(const ip4_fib_hash_t *fib, const ip4_address_t *addr, u32 len)
#define hash_unset(h, key)
const dpo_id_t * fib_entry_contribute_ip_forwarding(fib_node_index_t fib_entry_index)
u32 index_t
A Data-Path Object is an object that represents actions that are applied to packets are they are swit...
u32 fib_node_index_t
A typedef of a node index.
if(node->flags &VLIB_NODE_FLAG_TRACE) vnet_interface_output_trace(vm
ip46_address_t fp_addr
The address type is not deriveable from the fp_addr member.
fib_table_walk_rc_t(* fib_table_walk_fn_t)(fib_node_index_t fei, void *ctx)
Call back function when walking entries in a FIB table.
static uword ip4_destination_matches_route(const ip4_main_t *im, const ip4_address_t *key, const ip4_address_t *dest, uword dest_length)
#define vec_free(V)
Free vector's memory (no header).
fib_node_index_t ip4_fib_hash_table_lookup(const ip4_fib_hash_t *fib, const ip4_address_t *addr, u32 len)
@ FIB_TABLE_WALK_STOP
Stop the walk completely.
uword * fib_entry_by_dst_address[33]
fib_protocol_t fp_proto
protocol type
static void hash_set_flags(void *v, uword flags)
The identity of a DPO is a combination of its type and its instance number/index of objects of that t...
#define INDEX_INVALID
Invalid index - used when no index is known blazoned capitals INVALID speak volumes where ~0 does not...
#define hash_create(elts, value_bytes)
Aggregate type for a prefix.
void ip4_fib_hash_table_walk(ip4_fib_hash_t *fib, fib_table_walk_fn_t fn, void *ctx)
Walk all entries in a FIB table N.B: This is NOT safe to deletes.