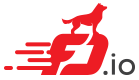 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
16 #ifndef SRC_VNET_SESSION_SESSION_TYPES_H_
17 #define SRC_VNET_SESSION_SESSION_TYPES_H_
22 #define SESSION_INVALID_INDEX ((u32)~0)
23 #define SESSION_INVALID_HANDLE ((u64)~0)
24 #define SESSION_CTRL_MSG_MAX_SIZE 86
25 #define SESSION_NODE_FRAME_SIZE 128
27 #define foreach_session_endpoint_fields \
28 foreach_transport_endpoint_cfg_fields \
29 _(u8, transport_proto) \
31 typedef struct _session_endpoint
33 #define _(type, name) type name;
38 typedef struct _session_endpoint_cfg
40 #define _(type, name) type name;
52 #define SESSION_IP46_ZERO \
59 #define TRANSPORT_ENDPOINT_NULL \
61 .sw_if_index = ENDPOINT_INVALID_INDEX, \
62 .ip = SESSION_IP46_ZERO, \
63 .fib_index = ENDPOINT_INVALID_INDEX, \
67 #define SESSION_ENDPOINT_NULL \
69 .sw_if_index = ENDPOINT_INVALID_INDEX, \
70 .ip = SESSION_IP46_ZERO, \
71 .fib_index = ENDPOINT_INVALID_INDEX, \
74 .peer = TRANSPORT_ENDPOINT_NULL, \
75 .transport_proto = 0, \
77 #define SESSION_ENDPOINT_CFG_NULL \
79 .sw_if_index = ENDPOINT_INVALID_INDEX, .ip = SESSION_IP46_ZERO, \
80 .fib_index = ENDPOINT_INVALID_INDEX, .is_ip4 = 0, .port = 0, \
81 .peer = TRANSPORT_ENDPOINT_NULL, .transport_proto = 0, \
82 .app_wrk_index = ENDPOINT_INVALID_INDEX, \
83 .opaque = ENDPOINT_INVALID_INDEX, \
84 .parent_handle = SESSION_INVALID_HANDLE, .ext_cfg = 0, \
87 #define session_endpoint_to_transport(_sep) ((transport_endpoint_t *)_sep)
88 #define session_endpoint_to_transport_cfg(_sep) \
89 ((transport_endpoint_cfg_t *)_sep)
129 #define foreach_session_state \
130 _(CREATED, "created") \
131 _(LISTENING, "listening") \
132 _(CONNECTING, "connecting") \
133 _(ACCEPTING, "accepting") \
135 _(OPENED, "opened") \
136 _(TRANSPORT_CLOSING, "transport-closing") \
137 _(CLOSING, "closing") \
138 _(APP_CLOSED, "app-closed") \
139 _(TRANSPORT_CLOSED, "transport-closed") \
140 _(CLOSED, "closed") \
141 _(TRANSPORT_DELETED, "transport-deleted") \
145 #define _(sym, str) SESSION_STATE_ ## sym,
151 #define foreach_session_flag \
152 _ (RX_EVT, "rx-event") \
154 _ (CUSTOM_TX, "custom-tx") \
155 _ (IS_MIGRATING, "migrating") \
156 _ (UNIDIRECTIONAL, "unidirectional") \
157 _ (CUSTOM_FIFO_TUNING, "custom-fifo-tuning") \
158 _ (HALF_OPEN, "half-open")
162 #define _(sym, str) SESSION_F_BIT_ ## sym,
170 #define _(sym, str) SESSION_F_ ## sym = 1 << SESSION_F_BIT_ ## sym,
226 return (
proto << 1 | is_ip4);
291 return handle & 0xFFFFFFFF;
311 return (((
u64)
data << 32) | (
u64) session_index);
317 return (ho_handle & 0xffffffff);
323 return (ho_handle >> 32);
365 #define foreach_session_ctrl_evt \
367 _ (LISTEN_URI, listen_uri) \
369 _ (UNLISTEN, unlisten) \
370 _ (UNLISTEN_REPLY, unlisten_reply) \
371 _ (ACCEPTED, accepted) \
372 _ (ACCEPTED_REPLY, accepted_reply) \
373 _ (CONNECT, connect) \
374 _ (CONNECT_URI, connect_uri) \
375 _ (CONNECTED, connected) \
376 _ (SHUTDOWN, shutdown) \
377 _ (DISCONNECT, disconnect) \
378 _ (DISCONNECTED, disconnected) \
379 _ (DISCONNECTED_REPLY, disconnected_reply) \
380 _ (RESET_REPLY, reset_reply) \
381 _ (REQ_WORKER_UPDATE, req_worker_update) \
382 _ (WORKER_UPDATE, worker_update) \
383 _ (WORKER_UPDATE_REPLY, worker_update_reply) \
384 _ (APP_DETACH, app_detach) \
385 _ (APP_ADD_SEGMENT, app_add_segment) \
386 _ (APP_DEL_SEGMENT, app_del_segment) \
387 _ (MIGRATED, migrated) \
388 _ (CLEANUP, cleanup) \
389 _ (APP_WRK_RPC, app_wrk_rpc) \
390 _ (TRANSPORT_ATTR, transport_attr) \
391 _ (TRANSPORT_ATTR_REPLY, transport_attr_reply) \
393 #define FIFO_EVENT_APP_RX SESSION_IO_EVT_RX
394 #define FIFO_EVENT_APP_TX SESSION_IO_EVT_TX
395 #define FIFO_EVENT_DISCONNECT SESSION_CTRL_EVT_CLOSE
396 #define FIFO_EVENT_BUILTIN_RX SESSION_IO_EVT_BUILTIN_RX
397 #define FIFO_EVENT_BUILTIN_TX SESSION_IO_EVT_BUILTIN_TX
427 } __clib_packed session_event_t;
429 #define SESSION_MSG_NULL { }
448 #define SESSION_CONN_ID_LEN 37
449 #define SESSION_CONN_HDR_LEN 45
452 "session conn id wrong length");
454 #define foreach_session_error \
455 _ (NONE, "no error") \
456 _ (UNKNOWN, "generic/unknown error") \
457 _ (REFUSED, "refused") \
458 _ (TIMEDOUT, "timedout") \
459 _ (ALLOC, "obj/memory allocation error") \
460 _ (OWNER, "object not owned by application") \
461 _ (NOROUTE, "no route") \
462 _ (NOINTF, "no resolving interface") \
463 _ (NOIP, "no ip for lcl interface") \
464 _ (NOPORT, "no lcl port") \
465 _ (NOSUPPORT, "not supported") \
466 _ (NOLISTEN, "not listening") \
467 _ (NOSESSION, "session does not exist") \
468 _ (NOAPP, "app not attached") \
469 _ (PORTINUSE, "lcl port in use") \
470 _ (IPINUSE, "ip in use") \
471 _ (ALREADY_LISTENING, "ip port pair already listened on") \
472 _ (INVALID, "invalid value") \
473 _ (INVALID_RMT_IP, "invalid remote ip") \
474 _ (INVALID_APPWRK, "invalid app worker") \
475 _ (INVALID_NS, "invalid namespace") \
476 _ (SEG_NO_SPACE, "Couldn't allocate a fifo pair") \
477 _ (SEG_NO_SPACE2, "Created segment, couldn't allocate a fifo pair") \
478 _ (SEG_CREATE, "Couldn't create a new segment") \
479 _ (FILTERED, "session filtered") \
480 _ (SCOPE, "scope not supported") \
481 _ (BAPI_NO_FD, "bapi doesn't have a socket fd") \
482 _ (BAPI_SEND_FD, "couldn't send fd over bapi socket fd") \
483 _ (BAPI_NO_REG, "app bapi registration not found") \
484 _ (MQ_MSG_ALLOC, "failed to alloc mq msg") \
485 _ (TLS_HANDSHAKE, "failed tls handshake") \
486 _ (EVENTFD_ALLOC, "failed to alloc eventfd") \
487 _ (NOEXTCFG, "no extended transport config") \
488 _ (NOCRYPTOENG, "no crypto engine") \
489 _ (NOCRYPTOCKP, "cert key pair not found ") \
490 _ (LOCAL_CONNECT, "could not connect with local scope")
494 #define _(sym, str) SESSION_EP_##sym,
502 #define _(sym, str) SESSION_E_##sym = -SESSION_EP_##sym,
507 #define SESSION_CLI_ID_LEN "60"
508 #define SESSION_CLI_STATE_LEN "15"
511 #define SESSION_ERROR_SEG_CREATE SESSION_E_SEG_CREATE
512 #define SESSION_ERROR_NO_SPACE SESSION_E_SEG_NO_SPACE
513 #define SESSION_ERROR_NEW_SEG_NO_SPACE SESSION_E_SEG_NO_SPACE2
@ SESSION_CTRL_EVT_SHUTDOWN
enum session_error_ session_error_t
transport_tx_fn_type_t transport_protocol_tx_fn_type(transport_proto_t tp)
#define foreach_session_state
CLIB_CACHE_LINE_ALIGN_MARK(pad)
u32 session_index
Index in thread pool where session was allocated.
@ SESSION_FT_ACTION_ENQUEUED
@ SESSION_CTRL_EVT_WORKER_UPDATE
static void session_parse_handle(session_handle_t handle, u32 *index, u32 *thread_index)
@ SESSION_CTRL_EVT_CONNECTED
@ SESSION_CTRL_EVT_DISCONNECTED
struct session_dgram_header_ session_dgram_hdr_t
@ SESSION_CTRL_EVT_CONNECT
struct _session_endpoint_cfg session_endpoint_cfg_t
enum transport_service_type_ transport_service_type_t
enum session_error_p_ session_error_p_t
static session_handle_t session_make_handle(u32 session_index, u32 data)
@ SESSION_FT_ACTION_DEQUEUED
u8 thread_index
Index of the thread that allocated the session.
session_type_t session_type
Type built from transport and network protocol types.
@ SESSION_IO_EVT_BUILTIN_TX
static u8 session_tx_is_dgram(session_t *s)
static u8 session_endpoint_is_local(session_endpoint_t *sep)
static u32 session_thread_from_handle(session_handle_t handle)
static transport_proto_t session_type_transport_proto(session_type_t st)
struct session_ session_t
#define foreach_session_error
#define SESSION_CONN_ID_LEN
struct _svm_fifo svm_fifo_t
@ SESSION_CLEANUP_TRANSPORT
enum transport_dequeue_type_ transport_tx_fn_type_t
u32 app_wrk_index
Index of the app worker that owns the session.
enum session_ft_action_ session_ft_action_t
@ SESSION_CTRL_EVT_TRANSPORT_ATTR_REPLY
svm_fifo_t * rx_fifo
Pointers to rx/tx buffers.
@ SESSION_CTRL_EVT_UNLISTEN
@ SESSION_IO_EVT_BUILTIN_RX
@ SESSION_CTRL_EVT_UNLISTEN_REPLY
u32 app_index
Index of application that owns the listener.
@ SESSION_CTRL_EVT_HALF_CLOSE
STATIC_ASSERT(sizeof(session_dgram_hdr_t)==(SESSION_CONN_ID_LEN+8), "session conn id wrong length")
@ SESSION_CTRL_EVT_LISTEN_URI
static u8 session_endpoint_is_zero(session_endpoint_t *sep)
@ SESSION_CTRL_EVT_CLEANUP
static u8 session_endpoint_fib_proto(session_endpoint_t *sep)
@ SESSION_CTRL_EVT_LISTEN
u8 ip_is_local_host(ip46_address_t *ip46_address, u8 is_ip4)
@ SESSION_IO_EVT_TX_FLUSH
static u8 session_type_is_ip4(session_type_t st)
static fib_protocol_t session_get_fib_proto(session_t *s)
@ SESSION_CTRL_EVT_DISCONNECTED_REPLY
static u32 session_index_from_handle(session_handle_t handle)
static session_handle_t session_handle(session_t *s)
@ SESSION_FT_ACTION_N_ACTIONS
enum fib_protocol_t_ fib_protocol_t
Protocol Type.
@ SESSION_CTRL_EVT_APP_DETACH
@ SESSION_CTRL_EVT_REQ_WORKER_UPDATE
session_handle_t listener_handle
Parent listener session index if the result of an accept.
static session_type_t session_type_from_proto_and_ip(transport_proto_t proto, u8 is_ip4)
@ SESSION_CTRL_EVT_RESET_REPLY
@ TRANSPORT_TX_DGRAM
datagram mode
@ SESSION_CTRL_EVT_ACCEPTED
u32 opaque
Opaque, for general use.
enum session_flags_bits_ session_flag_bits_t
@ SESSION_CLEANUP_SESSION
enum _transport_proto transport_proto_t
transport_service_type_t transport_protocol_service_type(transport_proto_t tp)
struct _session_endpoint session_endpoint_t
@ SESSION_CTRL_EVT_APP_WRK_RPC
static transport_proto_t session_get_transport_proto(session_t *s)
@ SESSION_CTRL_EVT_CONNECT_URI
static transport_service_type_t session_transport_service_type(session_t *s)
@ SESSION_CTRL_EVT_TRANSPORT_ATTR
enum session_flags_ session_flags_t
@ SESSION_CTRL_EVT_DISCONNECT
static u32 session_handle_data(session_handle_t ho_handle)
@ SESSION_CTRL_EVT_ACCEPTED_REPLY
static u32 session_handle_index(session_handle_t ho_handle)
@ SESSION_CTRL_EVT_MIGRATED
u32 ho_index
Index in app worker's half-open table if a half-open.
u8 pad[3]
log2 (size of the packing page block)
static transport_tx_fn_type_t session_transport_tx_fn_type(session_t *s)
u32 al_index
App listener index in app's listener pool if a listener.
static u8 session_has_transport(session_t *s)
@ SESSION_MQ_CTRL_EVT_RING
#define foreach_session_flag
@ SESSION_CTRL_EVT_WORKER_UPDATE_REPLY
#define foreach_session_endpoint_fields
volatile u8 session_state
State in session layer state machine.
struct session_dgram_pre_hdr_ session_dgram_pre_hdr_t
u32 connection_index
Index of the transport connection associated to the session.
@ SESSION_CTRL_EVT_APP_ADD_SEGMENT
u8 ip_is_zero(ip46_address_t *ip46_address, u8 is_ip4)
@ SESSION_CTRL_EVT_APP_DEL_SEGMENT
vl_api_wireguard_peer_flags_t flags