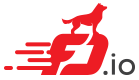 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
16 #ifndef SRC_VNET_TCP_TCP_TYPES_H_
17 #define SRC_VNET_TCP_TCP_TYPES_H_
24 #define TCP_TICK 0.000001
25 #define THZ (u32) (1/TCP_TICK)
27 #define TCP_TSTP_TICK 0.001
28 #define TCP_TSTP_HZ (u32) (1/TCP_TSTP_TICK)
29 #define TCP_PAWS_IDLE (24 * 86400 * TCP_TSTP_HZ)
30 #define TCP_TSTP_TO_HZ (u32) (TCP_TSTP_TICK * THZ)
32 #define TCP_FIB_RECHECK_PERIOD 1 * THZ
33 #define TCP_MAX_OPTION_SPACE 40
34 #define TCP_CC_DATA_SZ 24
35 #define TCP_RXT_MAX_BURST 10
37 #define TCP_DUPACK_THRESHOLD 3
38 #define TCP_IW_N_SEGMENTS 10
39 #define TCP_ALWAYS_ACK 1
40 #define TCP_USE_SACKS 1
43 #define foreach_tcp_fsm_state \
46 _(SYN_SENT, "SYN_SENT") \
47 _(SYN_RCVD, "SYN_RCVD") \
48 _(ESTABLISHED, "ESTABLISHED") \
49 _(CLOSE_WAIT, "CLOSE_WAIT") \
50 _(FIN_WAIT_1, "FIN_WAIT_1") \
51 _(LAST_ACK, "LAST_ACK") \
52 _(CLOSING, "CLOSING") \
53 _(FIN_WAIT_2, "FIN_WAIT_2") \
54 _(TIME_WAIT, "TIME_WAIT")
56 typedef enum _tcp_state
58 #define _(sym, str) TCP_STATE_##sym,
65 #define foreach_tcp_timer \
66 _(RETRANSMIT, "RETRANSMIT") \
67 _(PERSIST, "PERSIST") \
68 _(WAITCLOSE, "WAIT CLOSE") \
69 _(RETRANSMIT_SYN, "RETRANSMIT SYN") \
71 typedef enum _tcp_timers
73 #define _(sym, str) TCP_TIMER_##sym,
79 #define TCP_TIMER_HANDLE_INVALID ((u32) ~0)
81 #define TCP_TIMER_TICK 0.0001
82 #define TCP_TO_TIMER_TICK TCP_TICK*10000
85 #define TCP_RTO_MAX 60 * THZ
86 #define TCP_RTO_MIN 0.2 * THZ
87 #define TCP_RTT_MAX 30 * THZ
88 #define TCP_RTO_SYN_RETRIES 3
89 #define TCP_RTO_INIT 1 * THZ
90 #define TCP_RTO_BOFF_MAX 8
91 #define TCP_ESTABLISH_TIME (60 * THZ)
94 #define foreach_tcp_cfg_flag \
95 _(RATE_SAMPLE, "Rate sampling") \
96 _(NO_CSUM_OFFLOAD, "No csum offload") \
97 _(NO_TSO, "TSO off") \
99 _(NO_ENDPOINT,"No endpoint") \
103 #define _(sym, str) TCP_CFG_F_##sym##_BIT,
111 #define _(sym, str) TCP_CFG_F_##sym = 1 << TCP_CFG_F_##sym##_BIT,
118 #define foreach_tcp_connection_flag \
119 _(SNDACK, "Send ACK") \
120 _(FINSNT, "FIN sent") \
121 _(RECOVERY, "Recovery") \
122 _(FAST_RECOVERY, "Fast Recovery") \
123 _(DCNT_PENDING, "Disconnect pending") \
124 _(HALF_OPEN_DONE, "Half-open completed") \
125 _(FINPNDG, "FIN pending") \
126 _(RXT_PENDING, "Retransmit pending") \
127 _(FRXT_FIRST, "Retransmit first") \
128 _(DEQ_PENDING, "Dequeue pending ") \
129 _(PSH_PENDING, "PSH pending") \
130 _(FINRCVD, "FIN received") \
131 _(ZERO_RWND_SENT, "Zero RWND sent") \
135 #define _(sym, str) TCP_CONN_##sym##_BIT,
143 #define _(sym, str) TCP_CONN_##sym = 1 << TCP_CONN_##sym##_BIT,
149 #define TCP_SCOREBOARD_TRACE (0)
150 #define TCP_MAX_SACK_BLOCKS 255
151 #define TCP_INVALID_SACK_HOLE_INDEX ((u32)~0)
152 #define TCP_MAX_SACK_REORDER 300
154 typedef struct _scoreboard_trace_elt
163 typedef struct _sack_scoreboard_hole
172 typedef struct _sack_scoreboard
178 u32 last_sacked_bytes;
179 u32 last_bytes_delivered;
190 #if TCP_SCOREBOARD_TRACE
196 #define TCP_BTS_INVALID_INDEX ((u32)~0)
246 typedef enum _tcp_cc_algorithm_type
255 typedef enum _tcp_cc_ack_t
278 typedef struct _tcp_connection
317 u32 tsval_recent_age;
368 u32 next_node_opaque;
369 u32 limited_transmit;
389 #define rst_state snd_wl1
393 struct _tcp_cc_algorithm
411 #define tcp_fastrecovery_on(tc) (tc)->flags |= TCP_CONN_FAST_RECOVERY
412 #define tcp_fastrecovery_off(tc) (tc)->flags &= ~TCP_CONN_FAST_RECOVERY
413 #define tcp_recovery_on(tc) (tc)->flags |= TCP_CONN_RECOVERY
414 #define tcp_recovery_off(tc) (tc)->flags &= ~TCP_CONN_RECOVERY
415 #define tcp_in_fastrecovery(tc) ((tc)->flags & TCP_CONN_FAST_RECOVERY)
416 #define tcp_in_recovery(tc) ((tc)->flags & (TCP_CONN_RECOVERY))
417 #define tcp_in_slowstart(tc) (tc->cwnd < tc->ssthresh)
418 #define tcp_disconnect_pending(tc) ((tc)->flags & TCP_CONN_DCNT_PENDING)
419 #define tcp_disconnect_pending_on(tc) ((tc)->flags |= TCP_CONN_DCNT_PENDING)
420 #define tcp_disconnect_pending_off(tc) ((tc)->flags &= ~TCP_CONN_DCNT_PENDING)
421 #define tcp_fastrecovery_first(tc) ((tc)->flags & TCP_CONN_FRXT_FIRST)
422 #define tcp_fastrecovery_first_on(tc) ((tc)->flags |= TCP_CONN_FRXT_FIRST)
423 #define tcp_fastrecovery_first_off(tc) ((tc)->flags &= ~TCP_CONN_FRXT_FIRST)
425 #define tcp_in_cong_recovery(tc) ((tc)->flags & \
426 (TCP_CONN_FAST_RECOVERY | TCP_CONN_RECOVERY))
431 tc->flags &= ~(TCP_CONN_FAST_RECOVERY | TCP_CONN_RECOVERY);
435 #define tcp_csum_offload(tc) (!((tc)->cfg_flags & TCP_CFG_F_NO_CSUM_OFFLOAD))
437 #define tcp_zero_rwnd_sent(tc) ((tc)->flags & TCP_CONN_ZERO_RWND_SENT)
438 #define tcp_zero_rwnd_sent_on(tc) (tc)->flags |= TCP_CONN_ZERO_RWND_SENT
439 #define tcp_zero_rwnd_sent_off(tc) (tc)->flags &= ~TCP_CONN_ZERO_RWND_SENT
451 #undef TW_TIMER_WHEELS
452 #undef TW_SLOTS_PER_RING
455 #undef TW_TIMERS_PER_OBJECT
456 #undef LOG2_TW_TIMERS_PER_OBJECT
458 #undef TW_OVERFLOW_VECTOR
459 #undef TW_FAST_WHEEL_BITMAP
460 #undef TW_TIMER_ALLOW_DUPLICATE_STOP
461 #undef TW_START_STOP_TRACE_SIZE
463 #define TW_TIMER_WHEELS 2
464 #define TW_SLOTS_PER_RING 1024
465 #define TW_RING_SHIFT 10
466 #define TW_RING_MASK (TW_SLOTS_PER_RING -1)
467 #define TW_TIMERS_PER_OBJECT 16
468 #define LOG2_TW_TIMERS_PER_OBJECT 4
469 #define TW_SUFFIX _tcp_twsl
470 #define TW_FAST_WHEEL_BITMAP 0
471 #define TW_TIMER_ALLOW_DUPLICATE_STOP 1
#define foreach_tcp_connection_flag
TCP connection flags.
u32 min_seq
Min seq number in sample.
enum tcp_connection_flag_bits_ tcp_connection_flag_bits_e
static vlib_cli_command_t trace
(constructor) VLIB_CLI_COMMAND (trace)
rb_tree_t sample_lookup
Rbtree for sample lookup by min_seq.
struct tcp_bt_sample_ tcp_bt_sample_t
f64 tx_time
Transmit time for the burst.
#define foreach_tcp_fsm_state
TCP FSM state definitions as per RFC793.
struct tcp_rate_sample_ tcp_rate_sample_t
#define CLIB_CACHE_LINE_ALIGN_MARK(mark)
tcp_connection_flag_bits_
u64 prior_delivered
Delivered of sample used for rate, i.e., total bytes delivered at prior_time.
u32 below_data_wnd
All data in seg is below snd_una.
struct tcp_errors_ tcp_errors_t
struct _tcp_connection tcp_connection_t
tcp_bts_flags_t flags
Rate sample flags from bt sample.
tcp_bts_flags_t flags
Sample flag.
vl_api_dhcp_client_state_t state
struct _transport_connection transport_connection_t
u32 lost
Number of bytes lost over interval.
enum tcp_connection_flag_ tcp_connection_flags_e
static void tcp_cong_recovery_off(tcp_connection_t *tc)
f64 prior_time
Delivered time of sample used for rate.
struct tcp_byte_tracker_ tcp_byte_tracker_t
enum _tcp_timers tcp_timers_e
enum _tcp_state tcp_state_t
u32 next
Next sample index in list.
u32 tail
Tail of samples linked list.
u32 last_ooo
Cached last ooo sample.
static u32 * pending_timers
Vector of pending timers.
u64 tx_lost
Lost at tx time.
struct _sack_scoreboard sack_scoreboard_t
#define foreach_tcp_cfg_flag
Connection configuration flags.
f64 end
end of the time range
TW timer template header file, do not compile directly.
enum _tcp_cc_algorithm_type tcp_cc_algorithm_type_e
u32 above_data_wnd
Some data in segment is above snd_wnd.
f64 interval_time
Time to ack the bytes delivered.
u64 tx_in_flight
In flight at tx time.
u32 max_seq
Max seq number.
u32 prev
Previous sample index in list.
u32 delivered
Bytes delivered in interval_time.
struct _sack_block sack_block_t
enum tcp_bts_flags_ tcp_bts_flags_t
struct _sack_scoreboard_hole sack_scoreboard_hole_t
enum tcp_cfg_flag_bits_ tcp_cfg_flag_bits_e
f64 first_tx_time
Connection first tx time at tx.
f64 rtt_time
RTT for sample.
f64 delivered_time
Delivered time when sample taken.
u32 last_lost
Bytes lost now.
u32 above_ack_wnd
Acks for data not sent.
u64 tx_lost
Lost over interval.
static tcp_connection_t * tcp_get_connection_from_transport(transport_connection_t *tconn)
struct _tcp_cc_algorithm tcp_cc_algorithm_t
enum tcp_cfg_flag_ tcp_cfg_flags_e
#define tcp_fastrecovery_first_off(tc)
u32 head
Head of samples linked list.
u32 acked_and_sacked
Bytes acked + sacked now.
static void cleanup(void)
enum _tcp_cc_ack_t tcp_cc_ack_t
tw_timer_wheel_tcp_twsl_t tcp_timer_wheel_t
struct _scoreboard_trace_elt scoreboard_trace_elt_t
u64 tx_in_flight
In flight at (re)transmit time.
u32 below_ack_wnd
Acks for data below snd_una.
enum tcp_cc_event_ tcp_cc_event_t
vl_api_interface_index_t sw_if_index
#define foreach_tcp_timer
TCP timers.
u64 delivered
Total delivered bytes for sample.
tcp_bt_sample_t * samples
Pool of samples.
vl_api_wireguard_peer_flags_t flags