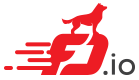 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
16 #ifndef included_tcp_packet_h
17 #define included_tcp_packet_h
22 #define foreach_tcp_flag \
34 #define _(f) TCP_FLAG_BIT_##f,
42 #define _(f) TCP_FLAG_##f = 1 << TCP_FLAG_BIT_##f,
47 typedef struct _tcp_header
69 u8 data_offset_and_reserved;
78 #define tcp_doff(_th) ((_th)->data_offset_and_reserved >> 4)
79 #define tcp_fin(_th) ((_th)->flags & TCP_FLAG_FIN)
80 #define tcp_syn(_th) ((_th)->flags & TCP_FLAG_SYN)
81 #define tcp_rst(_th) ((_th)->flags & TCP_FLAG_RST)
82 #define tcp_psh(_th) ((_th)->flags & TCP_FLAG_PSH)
83 #define tcp_ack(_th) ((_th)->flags & TCP_FLAG_ACK)
84 #define tcp_urg(_th) ((_th)->flags & TCP_FLAG_URG)
85 #define tcp_ece(_th) ((_th)->flags & TCP_FLAG_ECE)
86 #define tcp_cwr(_th) ((_th)->flags & TCP_FLAG_CWR)
89 #define tcp_is_syn(_th) !!((_th)->flags & TCP_FLAG_SYN)
90 #define tcp_is_fin(_th) !!((_th)->flags & TCP_FLAG_FIN)
115 #define foreach_tcp_options_flag \
124 #define _(f) TCP_OPTS_FLAG_BIT_##f,
132 #define _(f) TCP_OPTS_FLAG_##f = 1 << TCP_OPTS_FLAG_BIT_##f,
137 typedef struct _sack_block
155 #define tcp_opts_mss(_to) ((_to)->flags & TCP_OPTS_FLAG_MSS)
156 #define tcp_opts_tstamp(_to) ((_to)->flags & TCP_OPTS_FLAG_TSTAMP)
157 #define tcp_opts_wscale(_to) ((_to)->flags & TCP_OPTS_FLAG_WSCALE)
158 #define tcp_opts_sack(_to) ((_to)->flags & TCP_OPTS_FLAG_SACK)
159 #define tcp_opts_sack_permitted(_to) ((_to)->flags & TCP_OPTS_FLAG_SACK_PERMITTED)
162 #define TCP_OPTION_LEN_EOL 1
163 #define TCP_OPTION_LEN_NOOP 1
164 #define TCP_OPTION_LEN_MSS 4
165 #define TCP_OPTION_LEN_WINDOW_SCALE 3
166 #define TCP_OPTION_LEN_SACK_PERMITTED 2
167 #define TCP_OPTION_LEN_TIMESTAMP 10
168 #define TCP_OPTION_LEN_SACK_BLOCK 8
170 #define TCP_HDR_LEN_MAX 60
171 #define TCP_WND_MAX 65535U
172 #define TCP_MAX_WND_SCALE 14
173 #define TCP_OPTS_ALIGN 4
174 #define TCP_OPTS_MAX_SACK_BLOCKS 3
175 #define TCP_MAX_GSO_SZ 65536
178 #define seq_lt(_s1, _s2) ((i32)((_s1)-(_s2)) < 0)
179 #define seq_leq(_s1, _s2) ((i32)((_s1)-(_s2)) <= 0)
180 #define seq_gt(_s1, _s2) ((i32)((_s1)-(_s2)) > 0)
181 #define seq_geq(_s1, _s2) ((i32)((_s1)-(_s2)) >= 0)
182 #define seq_max(_s1, _s2) (seq_gt((_s1), (_s2)) ? (_s1) : (_s2))
185 #define timestamp_lt(_t1, _t2) ((i32)((_t1)-(_t2)) < 0)
186 #define timestamp_leq(_t1, _t2) ((i32)((_t1)-(_t2)) <= 0)
200 u8 opt_len, opts_len, kind;
205 data = (
const u8 *) (th + 1);
208 to->flags &= (TCP_OPTS_FLAG_SACK_PERMITTED | TCP_OPTS_FLAG_WSCALE
209 | TCP_OPTS_FLAG_TSTAMP | TCP_OPTS_FLAG_MSS);
211 for (; opts_len > 0; opts_len -= opt_len,
data += opt_len)
231 if (opt_len < 2 || opt_len > opts_len)
243 to->flags |= TCP_OPTS_FLAG_MSS;
244 to->mss = clib_net_to_host_u16 (*(
u16 *) (
data + 2));
252 to->flags |= TCP_OPTS_FLAG_WSCALE;
260 to->flags |= TCP_OPTS_FLAG_TSTAMP;
261 if ((
to->flags & TCP_OPTS_FLAG_TSTAMP)
264 to->tsval = clib_net_to_host_u32 (*(
u32 *) (
data + 2));
265 to->tsecr = clib_net_to_host_u32 (*(
u32 *) (
data + 6));
272 to->flags |= TCP_OPTS_FLAG_SACK_PERMITTED;
276 if ((
to->flags & TCP_OPTS_FLAG_SACK_PERMITTED) == 0 ||
tcp_syn (th))
283 to->flags |= TCP_OPTS_FLAG_SACK;
286 for (j = 0; j <
to->n_sack_blocks; j++)
288 b.start = clib_net_to_host_u32 (*(
u32 *) (
data + 2 + 8 * j));
289 b.end = clib_net_to_host_u32 (*(
u32 *) (
data + 6 + 8 * j));
318 buf = clib_host_to_net_u16 (opts->
mss);
343 buf = clib_host_to_net_u32 (opts->
tsval);
346 buf = clib_host_to_net_u32 (opts->
tsecr);
362 buf = clib_host_to_net_u32 (opts->
sacks[
i].start);
365 buf = clib_host_to_net_u32 (opts->
sacks[
i].end);
#define vec_reset_length(v)
Reset vector length to zero NULL-pointer tolerant.
u32 tsval
Timestamp value.
static int tcp_header_bytes(tcp_header_t *t)
@ TCP_OPTION_EOL
End of options.
#define tcp_opts_tstamp(_to)
vl_api_ip_port_and_mask_t dst_port
u8 wscale
Window scale advertised.
@ TCP_OPTIONS_N_FLAG_BITS
#define TCP_OPTION_LEN_WINDOW_SCALE
struct _tcp_header tcp_header_t
@ TCP_OPTION_WINDOW_SCALE
Window scale.
sack_block_t * sacks
SACK blocks.
@ TCP_OPTION_SACK_PERMITTED
Selective Ack permitted.
@ TCP_OPTION_NOOP
No operation.
u32 tsecr
Echoed/reflected time stamp.
u16 mss
Maximum segment size advertised.
u8 n_sack_blocks
Number of SACKs blocks.
static_always_inline void * clib_memcpy_fast(void *restrict dst, const void *restrict src, size_t n)
@ TCP_OPTION_TIMESTAMP
Timestamps.
u8 flags
Option flags, see above.
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
#define tcp_opts_wscale(_to)
@ TCP_OPTION_AO
Authentication Option.
@ TCP_OPTION_SACK_BLOCK
Selective Ack block.
#define foreach_tcp_flag
Sender reduced congestion window.
f64 end
end of the time range
#define tcp_opts_mss(_to)
vl_api_ip_port_and_mask_t src_port
#define tcp_opts_sack_permitted(_to)
#define foreach_tcp_options_flag
SACK present.
static u32 tcp_options_write(u8 *data, tcp_options_t *opts)
Write TCP options to segment.
struct _sack_block sack_block_t
#define tcp_opts_sack(_to)
#define TCP_MAX_WND_SCALE
enum tcp_option_type tcp_option_type_t
@ TCP_OPTION_UTO
User timeout.
#define TCP_OPTION_LEN_TIMESTAMP
#define TCP_OPTION_LEN_SACK_PERMITTED
@ TCP_OPTION_MSS
Limit MSS.
#define TCP_OPTION_LEN_NOOP
#define TCP_OPTION_LEN_MSS
static int tcp_options_parse(tcp_header_t *th, tcp_options_t *to, u8 is_syn)
Parse TCP header options.
#define TCP_OPTION_LEN_SACK_BLOCK
vl_api_wireguard_peer_flags_t flags