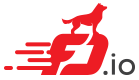 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
21 #include <mss_clamp/mss_clamp.api_enum.h>
22 #include <mss_clamp/mss_clamp.api_types.h>
25 #include <vpp/app/version.h>
27 #define REPLY_MSG_ID_BASE cm->msg_id_base
36 vl_api_mss_clamp_enable_disable_reply_t *rmp;
49 REPLY_MACRO (VL_API_MSS_CLAMP_ENABLE_DISABLE_REPLY);
63 dir4 = dir6 = MSS_CLAMP_DIR_NONE;
65 if (
rv == VNET_API_ERROR_FEATURE_DISABLED)
99 VL_API_MSS_CLAMP_GET_REPLY,
cm->dir_enabled4, mp, rmp,
rv,
100 ({ send_mss_clamp_details (cursor, reg, mp->context); }));
114 #include <mss_clamp/mss_clamp.api.c>
129 .version = VPP_BUILD_VER,
130 .description =
"TCP MSS clamping plugin",
#define VALIDATE_SW_IF_INDEX(mp)
static vl_api_registration_t * vl_api_client_index_to_registration(u32 index)
#define REPLY_MACRO2(t, body)
vl_api_interface_index_t sw_if_index
Configured MSS values on an interface.
static void vl_api_mss_clamp_get_t_handler(vl_api_mss_clamp_get_t *mp)
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
vl_api_mss_clamp_dir_t ipv6_direction
#define REPLY_AND_DETAILS_VEC_MACRO(t, v, mp, rmp, rv, body)
vl_api_interface_index_t sw_if_index
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
Reply for get TCP MSS Clamping feature settings request.
An API client registration, only in vpp/vlib.
int mssc_get_mss(u32 sw_if_index, u8 *dir4, u8 *dir6, u16 *mss4, u16 *mss6)
static void setup_message_id_table(api_main_t *am)
#define REPLY_MACRO_DETAILS4(t, rp, context, body)
int mssc_enable_disable(u32 sw_if_index, u8 dir4, u8 dir6, u16 mss4, u16 mss6)
vnet_feature_config_main_t * cm
vl_api_mss_clamp_dir_t ipv4_direction
static clib_error_t * mssc_api_hookup(vlib_main_t *vm)
vl_api_mss_clamp_dir_t ipv6_direction
#define BAD_SW_IF_INDEX_LABEL
VLIB_API_INIT_FUNCTION(mssc_api_hookup)
vl_api_mss_clamp_dir_t ipv4_direction
Get the TCP MSS Clamping feature settings.
vl_api_interface_index_t sw_if_index
static void vl_api_mss_clamp_enable_disable_t_handler(vl_api_mss_clamp_enable_disable_t *mp)
static void send_mss_clamp_details(u32 sw_if_index, vl_api_registration_t *rp, u32 context)
vl_api_interface_index_t sw_if_index
Enable/Disable TCP MSS Clamping feature on an interface.