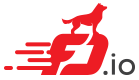 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
16 #ifndef __CNAT_SESSION_H__
17 #define __CNAT_SESSION_H__
clib_bihash_40_56_t cnat_bihash_t
ip46_address_t cs_ip[VLIB_N_DIR]
IP 4/6 address in the rx/tx direction.
index_t cs_lbi
The load balance object to use to forward.
@ CNAT_SESSION_FLAG_NO_CLIENT
This session doesn't have a client, do not attempt to free it.
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
struct cnat_session_t_ cnat_session_t
A session represents the memory of a translation.
u32 dpoi_next_node
Persist translation->ct_lb.dpoi_next_node.
A session represents the memory of a translation.
struct cnat_session_t_::@645 key
this key sits in the same memory location a 'key' in the bihash kvp
u8 cs_af
The address family describing the IP addresses.
void cnat_session_free(cnat_session_t *session)
Free a session & update refcounts.
#define STRUCT_OFFSET_OF(t, f)
u32 index_t
A Data-Path Object is an object that represents actions that are applied to packets are they are swit...
@ CNAT_SESSION_FLAG_HAS_SNAT
Indicates a return path session that was source NATed on the way in.
int cnat_session_purge(void)
Purge all the sessions.
cnat_bihash_t cnat_session_db
The DB of sessions.
STATIC_ASSERT(STRUCT_OFFSET_OF(cnat_session_t, key)==STRUCT_OFFSET_OF(cnat_bihash_kv_t, key), "key overlaps")
Ensure the session object correctly overlays the bihash key/value pair.
u32 cs_ts_index
Timestamp index this session was last used.
ip_protocol_t cs_proto
The IP protocol TCP or UDP only supported.
u8 * format_cnat_session(u8 *s, va_list *args)
enum cnat_session_location_t_ cnat_session_location_t
u8 cs_loc
input / output / fib session
u64 cnat_session_scan(vlib_main_t *vm, f64 start_time, int i)
Scan the session DB for expired sessions.
@ CNAT_SESSION_FLAG_NO_NAT
void(* cnat_free_port_cb)(u16 port, ip_protocol_t iproto)
Port cleanup callback.
walk_rc_t(* cnat_session_walk_cb_t)(const cnat_session_t *session, void *ctx)
Callback function invoked during a walk of all translations.
@ CNAT_SESSION_FLAG_ALLOC_PORT
This session source port was allocated, free it on cleanup.
enum cnat_session_flag_t_ cnat_session_flag_t
void cnat_session_walk(cnat_session_walk_cb_t cb, void *ctx)
Walk/visit each of the cnat session.
enum ip_protocol ip_protocol_t
struct cnat_session_t_::@646 value
this value sits in the same memory location a 'value' in the bihash kvp
enum walk_rc_t_ walk_rc_t
Walk return code.
u16 cs_port[VLIB_N_DIR]
ports in rx/tx