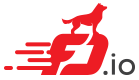 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
38 ctx->cb (session,
ctx->ctx);
40 return (BIHASH_WALK_CONTINUE);
66 return (BIHASH_WALK_CONTINUE);
101 s,
"session:[%U;%d -> %U;%d, %U] => %U;%d -> %U;%d %U lb:%d age:%f",
137 .path =
"show cnat session",
139 .short_help =
"show cnat session",
182 if (alloc_arena (
h) == 0)
185 for ( ;
i <
h->nbuckets;
i++)
191 if (
i < (
h->nbuckets - 3))
193 BVT (clib_bihash_bucket) *
b =
194 BV (clib_bihash_get_bucket) (
h,
i + 3);
196 b = BV (clib_bihash_get_bucket) (
h,
i + 1);
197 if (!BV (clib_bihash_bucket_is_empty) (
b))
205 BVT (clib_bihash_bucket) *
b = BV (clib_bihash_get_bucket) (
h,
i);
206 if (BV (clib_bihash_bucket_is_empty) (
b))
209 for (j = 0; j < (1 <<
b->log2_pages); j++)
213 if (v->kvp[k].key[0] == ~0ULL && v->kvp[k].value[0] == ~0ULL)
228 if (BV (clib_bihash_bucket_is_empty) (
b))
247 "CNat Session DB",
cm->session_hash_buckets,
248 cm->session_hash_memory);
273 .path =
"show cnat timestamp",
275 .short_help =
"show cnat timestamp",
clib_bihash_40_56_t cnat_bihash_t
ip46_address_t cs_ip[VLIB_N_DIR]
IP 4/6 address in the rx/tx direction.
void cnat_session_free(cnat_session_t *session)
Free a session & update refcounts.
u64 cnat_session_scan(vlib_main_t *vm, f64 start_time, int i)
Scan the session DB for expired sessions.
u8 * format_cnat_session_location(u8 *s, va_list *args)
#define BIHASH_KVP_PER_PAGE
static f64 cnat_timestamp_exp(u32 index)
index_t cs_lbi
The load balance object to use to forward.
@ CNAT_SESSION_FLAG_NO_CLIENT
This session doesn't have a client, do not attempt to free it.
cnat_session_walk_cb_t cb
#define clib_error_return(e, args...)
cnat_timestamp_t * cnat_timestamps
u8 * format_cnat_session(u8 *s, va_list *args)
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
int cnat_session_purge(void)
Purge all the sessions.
A session represents the memory of a translation.
struct cnat_session_t_::@645 key
this key sits in the same memory location a 'key' in the bihash kvp
static void clib_rwlock_reader_lock(clib_rwlock_t *p)
static void * clib_bihash_get_value(clib_bihash *h, uword offset)
Get pointer to value page given its clib mheap offset.
u8 cs_af
The address family describing the IP addresses.
static void clib_rwlock_reader_unlock(clib_rwlock_t *p)
#define pool_is_free_index(P, I)
Use free bitmap to query whether given index is free.
#define pool_foreach(VAR, POOL)
Iterate through pool.
struct cnat_session_walk_ctx_t_ cnat_session_walk_ctx_t
void clib_bihash_init(clib_bihash *h, char *name, u32 nbuckets, uword memory_size)
initialize a bounded index extensible hash table
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
void cnat_client_free_by_ip(ip46_address_t *ip, u8 af)
if(node->flags &VLIB_NODE_FLAG_TRACE) vnet_interface_output_trace(vm
BVT(clib_bihash)
The table of adjacencies indexed by the rewrite string.
static vlib_cli_command_t cnat_timestamp_show_cmd
(constructor) VLIB_CLI_COMMAND (cnat_timestamp_show_cmd)
vnet_feature_config_main_t * cm
#define VLIB_CLI_COMMAND(x,...)
void vlib_cli_output(vlib_main_t *vm, char *fmt,...)
u32 cs_ts_index
Timestamp index this session was last used.
ip_protocol_t cs_proto
The IP protocol TCP or UDP only supported.
static int cnat_session_purge_walk(BVT(clib_bihash_kv) *key, void *arg)
static clib_error_t * cnat_session_init(vlib_main_t *vm)
#define vec_free(V)
Free vector's memory (no header).
template key/value backing page structure
u8 cs_loc
input / output / fib session
description fragment has unexpected format
static int cnat_session_walk_cb(BVT(clib_bihash_kv) *kv, void *arg)
format_function_t format_ip46_address
walk_rc_t(* cnat_session_walk_cb_t)(const cnat_session_t *session, void *ctx)
Callback function invoked during a walk of all translations.
#define VLIB_INIT_FUNCTION(x)
@ CNAT_SESSION_FLAG_ALLOC_PORT
This session source port was allocated, free it on cleanup.
static_always_inline void clib_prefetch_load(void *p)
#define vec_foreach(var, vec)
Vector iterator.
static vlib_cli_command_t cnat_session_show_cmd_node
(constructor) VLIB_CLI_COMMAND (cnat_session_show_cmd_node)
static void cnat_timestamp_free(u32 index)
struct cnat_session_purge_walk_t_ cnat_session_purge_walk_ctx_t
cnat_bihash_t cnat_session_db
The DB of sessions.
enum ip_protocol ip_protocol_t
static clib_error_t * cnat_timestamp_show(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
void clib_bihash_foreach_key_value_pair(clib_bihash *h, clib_bihash_foreach_key_value_pair_cb *callback, void *arg)
Visit active (key,value) pairs in a bi-hash table.
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
struct cnat_session_t_::@646 value
this value sits in the same memory location a 'value' in the bihash kvp
static f64 vlib_time_now(vlib_main_t *vm)
void(* cnat_free_port_cb)(u16 port, ip_protocol_t iproto)
Port cleanup callback.
#define cnat_bihash_add_del
void cnat_session_walk(cnat_session_walk_cb_t cb, void *ctx)
Walk/visit each of the cnat session.
static clib_error_t * cnat_session_show(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
u16 cs_port[VLIB_N_DIR]
ports in rx/tx