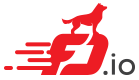 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
16 #ifndef included_clib_interrupt_h
17 #define included_clib_interrupt_h
86 __atomic_fetch_or (bmp,
mask, __ATOMIC_RELAXED);
99 bmp[
off] |= __atomic_exchange_n (abm +
off, 0, __ATOMIC_SEQ_CST);
116 bmp[
off] |= __atomic_exchange_n (abm +
off, 0, __ATOMIC_SEQ_CST);
128 bmp[
off] |= __atomic_exchange_n (abm +
off, 0, __ATOMIC_SEQ_CST);
129 bmp_uword = bmp[
off];
static_always_inline void clib_interrupt_free(void **data)
#define count_trailing_zeros(x)
static uword pow2_mask(uword x)
#define CLIB_CACHE_LINE_ALIGN_MARK(mark)
static void clib_mem_free(void *p)
static_always_inline int clib_interrupt_get_next(void *in, int last)
static_always_inline void clib_interrupt_set_atomic(void *in, int int_num)
static_always_inline uword * clib_interrupt_get_atomic_bitmap(void *d)
static_always_inline void clib_interrupt_clear(void *in, int int_num)
#define static_always_inline
static heap_elt_t * last(heap_header_t *h)
static_always_inline void clib_interrupt_set(void *in, int int_num)
static_always_inline int clib_interrupt_get_n_int(void *d)
void clib_interrupt_resize(void **data, uword n_interrupts)
void clib_interrupt_init(void **data, uword n_interrupts)
static_always_inline uword * clib_interrupt_get_bitmap(void *d)