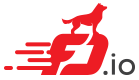 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
80 s =
format (s,
"page stats: page-size %U, total %lu, mapped %lu, "
89 s =
format (s,
"\n%Unuma %u: %lu pages, %U bytes",
__clib_export void * clib_mem_vm_map_shared(void *base, uword size, int fd, uword offset, char *fmt,...)
__clib_export void * clib_mem_vm_map(void *base, uword size, clib_mem_page_sz_t log2_page_sz, char *fmt,...)
vl_api_ikev2_sa_stats_t stats
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
void * clib_mem_vm_map_internal(void *base, clib_mem_page_sz_t log2_page_sz, uword size, int fd, uword offset, char *name)
__clib_export clib_mem_main_t clib_mem_main
#define vec_free(V)
Free vector's memory (no header).
template key/value backing page structure
description fragment has unexpected format
u8 * format_clib_mem_page_stats(u8 *s, va_list *va)
__clib_export void * clib_mem_vm_map_stack(uword size, clib_mem_page_sz_t log2_page_sz, char *fmt,...)