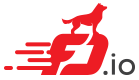 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
28 #include <vnet/span/span.api_enum.h>
29 #include <vnet/span/span.api_types.h>
31 #define REPLY_MSG_ID_BASE span_main.msg_id_base
38 vl_api_sw_interface_span_enable_disable_reply_t *rmp;
47 REPLY_MACRO (VL_API_SW_INTERFACE_SPAN_ENABLE_DISABLE_REPLY);
96 #include <vnet/span/span.api.c>
static vl_api_registration_t * vl_api_client_index_to_registration(u32 index)
Reply to SPAN dump request.
vl_api_interface_index_t sw_if_index_from
static void vl_api_send_msg(vl_api_registration_t *rp, u8 *elem)
vl_api_span_state_t state
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
VLIB_API_INIT_FUNCTION(span_api_hookup)
static void vl_api_sw_interface_span_enable_disable_t_handler(vl_api_sw_interface_span_enable_disable_t *mp)
static uword clib_bitmap_get(uword *ai, uword i)
Gets the ith bit value from a bitmap.
An API client registration, only in vpp/vlib.
static void setup_message_id_table(api_main_t *am)
clib_bitmap_t * mirror_ports
#define clib_bitmap_free(v)
Free a bitmap.
static clib_error_t * span_api_hookup(vlib_main_t *vm)
static void vl_api_sw_interface_span_dump_t_handler(vl_api_sw_interface_span_dump_t *mp)
vl_api_interface_index_t sw_if_index_to
vl_api_span_state_t state
int span_add_delete_entry(vlib_main_t *vm, u32 src_sw_if_index, u32 dst_sw_if_index, u8 state, span_feat_t sf)
#define vec_foreach(var, vec)
Vector iterator.
vl_api_interface_index_t sw_if_index_from
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
static vlib_main_t * vlib_get_main(void)
vl_api_interface_index_t sw_if_index_to
#define REPLY_MSG_ID_BASE
#define clib_bitmap_foreach(i, ai)
Macro to iterate across set bits in a bitmap.
span_interface_t * interfaces
static uword * clib_bitmap_dup_or(uword *ai, uword *bi)
Logical operator across two bitmaps which duplicates the first bitmap.
Enable/Disable span to mirror traffic from one interface to another.
void * vl_msg_api_alloc(int nbytes)