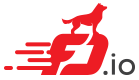 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
43 u32 session_index,
u8 is_failed)
45 static u32 client_index = 0;
51 "Bapi connect errored on session %u", session_index);
61 session->is_dgram = 1;
76 static u32 client_index = 0;
89 session->is_dgram = 1;
130 u32 session_index = session->session_index;
137 ECHO_FAIL (ECHO_FAIL_ACCEPTED_WAIT_FOR_SEG_ALLOC,
138 "accepted wait_for_segment_allocation errored");
144 sizeof (ip46_address_t));
145 session->transport.lcl_port = mp->
lcl_port;
154 session->is_dgram = 1;
static void udp_echo_accepted_cb(session_accepted_msg_t *mp, echo_session_t *session)
u32 *volatile data_thread_args
int echo_attach_session(uword segment_handle, uword rxf_offset, uword txf_offset, uword mq_offset, echo_session_t *s)
#define ECHO_FAIL(fail, _fmt, _args...)
echo_session_t * sessions
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
static void udp_echo_disconnected_cb(session_disconnected_msg_t *mp, echo_session_t *s)
volatile u32 n_clients_connected
volatile u64 accepted_session_count
@ ECHO_EVT_FIRST_QCONNECT
volatile connection_state_t state
#define SESSION_INVALID_INDEX
@ ECHO_SESSION_TYPE_STREAM
@ ECHO_SESSION_STATE_READY
static_always_inline void * clib_memcpy_fast(void *restrict dst, const void *restrict src, size_t n)
void echo_session_print_stats(echo_main_t *em, echo_session_t *session)
static void udp_echo_connected_cb(session_connected_bundled_msg_t *mp, u32 session_index, u8 is_failed)
ECHO_REGISTER_PROTO(TRANSPORT_PROTO_UDP, echo_udp_proto_cb_vft)
@ ECHO_SESSION_STATE_AWAIT_DATA
@ ECHO_SESSION_STATE_CLOSING
static void udp_echo_reset_cb(session_reset_msg_t *mp, echo_session_t *s)
@ ECHO_EVT_FIRST_SCONNECT
#define clib_atomic_sub_fetch(a, b)
#define clib_atomic_fetch_add(a, b)
static void udp_echo_sent_disconnect_cb(echo_session_t *s)
volatile u64 bytes_to_receive
static void udp_echo_bound_uri_cb(session_bound_msg_t *mp, echo_session_t *session)
@ ECHO_EVT_LAST_SCONNECTED
teardown_stat_t reset_count
teardown_stat_t clean_count
static void udp_echo_cleanup_cb(echo_session_t *s, u8 parent_died)
struct echo_main_t::@691 uri_elts
teardown_stat_t close_count
echo_proto_cb_vft_t echo_udp_proto_cb_vft
void(* disconnected_cb)(session_disconnected_msg_t *mp, echo_session_t *s)
@ ECHO_SESSION_STATE_CLOSED
void echo_notify_event(echo_main_t *em, echo_test_evt_t e)