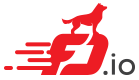 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
18 #ifndef __included_wg_noise_h__
19 #define __included_wg_noise_h__
26 #define NOISE_PUBLIC_KEY_LEN CURVE25519_KEY_SIZE
27 #define NOISE_SYMMETRIC_KEY_LEN 32 // CHACHA20POLY1305_KEY_SIZE
28 #define NOISE_TIMESTAMP_LEN (sizeof(uint64_t) + sizeof(uint32_t))
29 #define NOISE_AUTHTAG_LEN 16 //CHACHA20POLY1305_AUTHTAG_SIZE
30 #define NOISE_HASH_LEN BLAKE2S_HASH_SIZE
33 #define NOISE_HANDSHAKE_NAME "Noise_IKpsk2_25519_ChaChaPoly_BLAKE2s"
34 #define NOISE_IDENTIFIER_NAME "WireGuard v1 zx2c4 Jason@zx2c4.com"
37 #define COUNTER_BITS_TOTAL 8192
38 #define COUNTER_BITS (sizeof(unsigned long) * 8)
39 #define COUNTER_NUM (COUNTER_BITS_TOTAL / COUNTER_BITS)
40 #define COUNTER_WINDOW_SIZE (COUNTER_BITS_TOTAL - COUNTER_BITS)
43 #define REKEY_AFTER_MESSAGES (1ull << 60)
44 #define REJECT_AFTER_MESSAGES (UINT64_MAX - COUNTER_WINDOW_SIZE - 1)
45 #define REKEY_AFTER_TIME 120
46 #define REKEY_AFTER_TIME_RECV 165
47 #define REJECT_AFTER_TIME 180
48 #define REJECT_INTERVAL (0.02)
50 #define REJECT_INTERVAL_MASK (~((1ull<<24)-1))
189 uint8_t *
src,
size_t srclen, uint8_t *
dst);
194 uint8_t *
src,
size_t srclen, uint8_t *
dst);
void(* u_index_drop)(uint32_t)
noise_keypair_t * r_previous
uint8_t r_public[NOISE_PUBLIC_KEY_LEN]
bool noise_create_initiation(vlib_main_t *vm, noise_remote_t *, uint32_t *s_idx, uint8_t ue[NOISE_PUBLIC_KEY_LEN], uint8_t es[NOISE_PUBLIC_KEY_LEN+NOISE_AUTHTAG_LEN], uint8_t ets[NOISE_TIMESTAMP_LEN+NOISE_AUTHTAG_LEN])
void noise_remote_expire_current(noise_remote_t *r)
#define NOISE_SYMMETRIC_KEY_LEN
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
#define NOISE_AUTHTAG_LEN
uint8_t hs_hash[NOISE_HASH_LEN]
clib_rwlock_t r_keypair_lock
struct noise_keypair noise_keypair_t
struct noise_local::noise_upcall l_upcall
unsigned long c_backtrack[COUNTER_NUM]
noise_local_t * noise_local_pool
u32 vnet_crypto_key_index_t
bool noise_consume_response(vlib_main_t *vm, noise_remote_t *, uint32_t s_idx, uint32_t r_idx, uint8_t ue[NOISE_PUBLIC_KEY_LEN], uint8_t en[0+NOISE_AUTHTAG_LEN])
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
uint8_t r_timestamp[NOISE_TIMESTAMP_LEN]
enum noise_state_crypt noise_remote_decrypt(vlib_main_t *vm, noise_remote_t *, uint32_t r_idx, uint64_t nonce, uint8_t *src, size_t srclen, uint8_t *dst)
vnet_hw_if_output_node_runtime_t * r
uint8_t l_private[NOISE_PUBLIC_KEY_LEN]
struct noise_local noise_local_t
uint8_t hs_ck[NOISE_HASH_LEN]
struct noise_remote noise_remote_t
noise_keypair_t * r_current
noise_handshake_t r_handshake
bool noise_remote_ready(noise_remote_t *)
#define static_always_inline
uint8_t r_psk[NOISE_SYMMETRIC_KEY_LEN]
void noise_remote_precompute(noise_remote_t *)
enum noise_state_crypt noise_remote_encrypt(vlib_main_t *vm, noise_remote_t *, uint32_t *r_idx, uint64_t *nonce, uint8_t *src, size_t srclen, uint8_t *dst)
struct noise_counter noise_counter_t
enum noise_state_hs hs_state
bool noise_create_response(vlib_main_t *vm, noise_remote_t *, uint32_t *s_idx, uint32_t *r_idx, uint8_t ue[NOISE_PUBLIC_KEY_LEN], uint8_t en[0+NOISE_AUTHTAG_LEN])
static_always_inline noise_local_t * noise_local_get(uint32_t locali)
#define NOISE_PUBLIC_KEY_LEN
void noise_local_init(noise_local_t *, struct noise_upcall *)
void noise_remote_init(noise_remote_t *, uint32_t, const uint8_t[NOISE_PUBLIC_KEY_LEN], uint32_t)
uint8_t r_ss[NOISE_PUBLIC_KEY_LEN]
void noise_remote_clear(vlib_main_t *vm, noise_remote_t *r)
bool noise_remote_begin_session(vlib_main_t *vm, noise_remote_t *r)
bool noise_consume_initiation(vlib_main_t *vm, noise_local_t *, noise_remote_t **, uint32_t s_idx, uint8_t ue[NOISE_PUBLIC_KEY_LEN], uint8_t es[NOISE_PUBLIC_KEY_LEN+NOISE_AUTHTAG_LEN], uint8_t ets[NOISE_TIMESTAMP_LEN+NOISE_AUTHTAG_LEN])
uint8_t l_public[NOISE_PUBLIC_KEY_LEN]
uint8_t hs_e[NOISE_PUBLIC_KEY_LEN]
vnet_crypto_key_index_t kp_recv_index
#define NOISE_TIMESTAMP_LEN
struct noise_handshake noise_handshake_t
uint32_t(* u_index_set)(noise_remote_t *)
bool noise_local_set_private(noise_local_t *, const uint8_t[NOISE_PUBLIC_KEY_LEN])
vnet_crypto_key_index_t kp_send_index