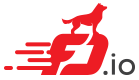 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
65 a->fg_color =
c->attr.fg_color;
71 a->bg_color =
c->attr.bg_color;
99 s =
format (s,
"%s%u",
i ?
";" :
"", codes[
i]);
142 if (c < t->n_header_cols)
258 va_start (arg, n_strings);
259 for (
r = 0;
r < n_rows;
r++)
263 va_arg (arg,
char *));
276 va_start (arg, n_strings);
#define clib_memcpy(d, s, n)
table_text_attr_align_t align
table_text_attr_color_t fg_color
vnet_hw_if_output_node_runtime_t * r
table_text_attr_t default_header_col
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
if(node->flags &VLIB_NODE_FLAG_TRACE) vnet_interface_output_trace(vm
table_text_attr_t default_title
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
#define vec_free(V)
Free vector's memory (no header).
table_text_attr_t default_header_row
description fragment has unexpected format
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
table_text_attr_color_t bg_color
table_text_attr_t default_body
table_text_attr_flags_t flags
#define vec_insert(V, N, M)
Insert N vector elements starting at element M, initialize new elements to zero (no header,...