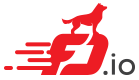 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
21 #define foreach_ipsec_handoff_error \
22 _(CONGESTION_DROP, "congestion drop")
26 #define _(sym,str) IPSEC_HANDOFF_ERROR_##sym,
33 #define _(sym,string) string,
136 thread_indices,
frame->n_vectors, 1);
140 IPSEC_HANDOFF_ERROR_CONGESTION_DROP,
141 frame->n_vectors - n_enq);
264 .name =
"esp4-encrypt-handoff",
265 .vector_size =
sizeof (
u32),
276 .name =
"esp6-encrypt-handoff",
277 .vector_size =
sizeof (
u32),
288 .name =
"esp4-encrypt-tun-handoff",
289 .vector_size =
sizeof (
u32),
300 .name =
"esp6-encrypt-tun-handoff",
301 .vector_size =
sizeof (
u32),
312 .name =
"esp-mpls-encrypt-tun-handoff",
313 .vector_size =
sizeof (
u32),
324 .name =
"esp4-decrypt-handoff",
325 .vector_size =
sizeof (
u32),
336 .name =
"esp6-decrypt-handoff",
337 .vector_size =
sizeof (
u32),
348 .name =
"esp4-decrypt-tun-handoff",
349 .vector_size =
sizeof (
u32),
360 .name =
"esp6-decrypt-tun-handoff",
361 .vector_size =
sizeof (
u32),
372 .name =
"ah4-encrypt-handoff",
373 .vector_size =
sizeof (
u32),
384 .name =
"ah6-encrypt-handoff",
385 .vector_size =
sizeof (
u32),
396 .name =
"ah4-decrypt-handoff",
397 .vector_size =
sizeof (
u32),
408 .name =
"ah6-decrypt-handoff",
409 .vector_size =
sizeof (
u32),
vnet_interface_main_t * im
vlib_buffer_t * bufs[VLIB_FRAME_SIZE]
static_always_inline u32 vlib_buffer_enqueue_to_thread(vlib_main_t *vm, vlib_node_runtime_t *node, u32 frame_queue_index, u32 *buffer_indices, u16 *thread_indices, u32 n_packets, int drop_on_congestion)
#define vlib_prefetch_buffer_header(b, type)
Prefetch buffer metadata.
vlib_main_t vlib_node_runtime_t vlib_frame_t * frame
vlib_main_t vlib_node_runtime_t vlib_frame_t * from_frame
static u8 * format_ipsec_handoff_trace(u8 *s, va_list *args)
vlib_get_buffers(vm, from, b, n_left_from)
@ VLIB_NODE_TYPE_INTERNAL
vlib_node_registration_t esp4_decrypt_tun_handoff
(constructor) VLIB_REGISTER_NODE (esp4_decrypt_tun_handoff)
vlib_node_registration_t ah4_encrypt_handoff
(constructor) VLIB_REGISTER_NODE (ah4_encrypt_handoff)
vlib_node_registration_t ah4_decrypt_handoff
(constructor) VLIB_REGISTER_NODE (ah4_decrypt_handoff)
#define foreach_ipsec_handoff_error
#define VLIB_NODE_FN(node)
static_always_inline uword ipsec_handoff(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame, u32 fq_index)
vlib_node_registration_t esp4_encrypt_tun_handoff
(constructor) VLIB_REGISTER_NODE (esp4_encrypt_tun_handoff)
#define VLIB_NODE_FLAG_TRACE
vlib_node_registration_t esp6_decrypt_handoff
(constructor) VLIB_REGISTER_NODE (esp6_decrypt_handoff)
static void * vlib_frame_vector_args(vlib_frame_t *f)
Get pointer to frame vector data.
#define static_always_inline
vlib_node_registration_t esp6_decrypt_tun_handoff
(constructor) VLIB_REGISTER_NODE (esp6_decrypt_tun_handoff)
static void vlib_node_increment_counter(vlib_main_t *vm, u32 node_index, u32 counter_index, u64 increment)
struct ipsec_handoff_trace_t_ ipsec_handoff_trace_t
struct _vlib_node_registration vlib_node_registration_t
vlib_main_t vlib_node_runtime_t * node
vlib_node_registration_t ah6_encrypt_handoff
(constructor) VLIB_REGISTER_NODE (ah6_encrypt_handoff)
vlib_node_registration_t esp4_encrypt_handoff
(constructor) VLIB_REGISTER_NODE (esp4_encrypt_handoff)
description fragment has unexpected format
vlib_node_registration_t esp6_encrypt_tun_handoff
(constructor) VLIB_REGISTER_NODE (esp6_encrypt_tun_handoff)
vlib_node_registration_t esp_mpls_encrypt_tun_handoff
(constructor) VLIB_REGISTER_NODE (esp_mpls_encrypt_tun_handoff)
vlib_node_registration_t esp6_encrypt_handoff
(constructor) VLIB_REGISTER_NODE (esp6_encrypt_handoff)
vlib_node_registration_t ah6_decrypt_handoff
(constructor) VLIB_REGISTER_NODE (ah6_decrypt_handoff)
static char * ipsec_handoff_error_strings[]
void * vlib_add_trace(vlib_main_t *vm, vlib_node_runtime_t *r, vlib_buffer_t *b, u32 n_data_bytes)
vlib_node_registration_t esp4_decrypt_handoff
(constructor) VLIB_REGISTER_NODE (esp4_decrypt_handoff)
vl_api_fib_path_type_t type
VLIB buffer representation.
#define VLIB_REGISTER_NODE(x,...)
vl_api_wireguard_peer_flags_t flags