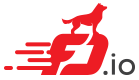 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
53 iproto =
ip4->protocol;
59 iproto =
ip6->protocol;
71 if (!session_not_found)
78 ip46_address_t ip46_dst_address;
118 CNAT_ERROR_EXHAUSTED_PORTS, 1);
124 if (iproto == IP_PROTOCOL_TCP || iproto == IP_PROTOCOL_UDP)
174 .name =
"cnat-snat-ip4",
175 .vector_size =
sizeof (
u32),
187 .name =
"cnat-snat-ip6",
188 .vector_size =
sizeof (
u32),
200 .arc_name =
"ip4-unicast",
201 .node_name =
"cnat-snat-ip4",
205 .arc_name =
"ip6-unicast",
206 .node_name =
"cnat-snat-ip6",
static uword cnat_snat_node_fn(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_buffer_t *b, cnat_node_ctx_t *ctx, int session_not_found, cnat_session_t *session)
static vlib_cli_command_t trace
(constructor) VLIB_CLI_COMMAND (trace)
static_always_inline void cnat_add_trace(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_buffer_t *b, cnat_session_t *session, const cnat_translation_t *ct, u8 flags)
ip46_address_t cs_ip[VLIB_N_DIR]
IP 4/6 address in the rx/tx direction.
vlib_main_t vlib_node_runtime_t vlib_frame_t * frame
static void ip46_address_set_ip4(ip46_address_t *ip46, const ip4_address_t *ip)
index_t cs_lbi
The load balance object to use to forward.
@ CNAT_SESSION_FLAG_NO_CLIENT
This session doesn't have a client, do not attempt to free it.
@ VLIB_NODE_TYPE_INTERNAL
static_always_inline void ip46_address_set_ip6(ip46_address_t *dst, const ip6_address_t *src)
vlib_main_t vlib_node_runtime_t * node
char * cnat_error_strings[]
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
static_always_inline void cnat_session_create(cnat_session_t *session, cnat_node_ctx_t *ctx, cnat_session_location_t rsession_location, u8 rsession_flags)
Create NAT sessions rsession_location is the location the (return) session will be matched at.
A session represents the memory of a translation.
struct cnat_session_t_::@645 key
this key sits in the same memory location a 'key' in the bihash kvp
@ CNAT_TRACE_SESSION_FOUND
#define VLIB_NODE_FN(node)
#define VLIB_NODE_FLAG_TRACE
static u8 * format_cnat_trace(u8 *s, va_list *args)
static_always_inline void vnet_feature_next(u32 *next0, vlib_buffer_t *b0)
@ CNAT_SESSION_FLAG_HAS_SNAT
Indicates a return path session that was source NATed on the way in.
static_always_inline void cnat_translation_ip6(const cnat_session_t *session, ip6_header_t *ip6, udp_header_t *udp)
static void cnat_timestamp_update(u32 index, f64 t)
static void vlib_node_increment_counter(vlib_main_t *vm, u32 node_index, u32 counter_index, u64 increment)
static_always_inline void cnat_translation_ip4(const cnat_session_t *session, ip4_header_t *ip4, udp_header_t *udp)
int cnat_allocate_port(u16 *port, ip_protocol_t iproto)
struct _vlib_node_registration vlib_node_registration_t
u32 cs_ts_index
Timestamp index this session was last used.
ip_protocol_t cs_proto
The IP protocol TCP or UDP only supported.
@ CNAT_SESSION_FLAG_ALLOC_PORT
This session source port was allocated, free it on cleanup.
static uword cnat_node_inline(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame, cnat_node_sub_t cnat_sub, ip_address_family_t af, cnat_session_location_t cs_loc, u8 do_trace)
cnat_snat_policy_t snat_policy
vlib_node_registration_t cnat_snat_ip4_node
(constructor) VLIB_REGISTER_NODE (cnat_snat_ip4_node)
enum ip_protocol ip_protocol_t
static void * vlib_buffer_get_current(vlib_buffer_t *b)
Get pointer to current data to process.
struct cnat_session_t_::@646 value
this value sits in the same memory location a 'value' in the bihash kvp
cnat_snat_policy_main_t cnat_snat_policy_main
#define INDEX_INVALID
Invalid index - used when no index is known blazoned capitals INVALID speak volumes where ~0 does not...
VNET_FEATURE_INIT(cnat_snat_ip4_node, static)
enum cnat_snat_next_ cnat_snat_next_t
vl_api_fib_path_type_t type
@ CNAT_TRACE_SESSION_CREATED
vlib_node_registration_t cnat_snat_ip6_node
(constructor) VLIB_REGISTER_NODE (cnat_snat_ip6_node)
u32 flags
buffer flags: VLIB_BUFFER_FREE_LIST_INDEX_MASK: bits used to store free list index,...
VLIB buffer representation.
u16 cs_port[VLIB_N_DIR]
ports in rx/tx
#define VLIB_REGISTER_NODE(x,...)