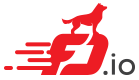 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
25 u8 *
a = va_arg (*args,
u8 *);
28 else if (
unformat (input,
"include-v6"))
44 s =
format (s,
"Included v4");
47 s =
format (s,
"Included v6");
53 s =
format (s,
"(unknown)");
82 int dst_address_length = 128 -
i;
95 return VNET_API_ERROR_INVALID_VALUE;
118 else if (
unformat (input,
"table %U",
141 .path =
"set cnat snat-policy if",
142 .short_help =
"set cnat snat-policy if [del]"
143 "[table [include-v4 include-v6 k8s]] [interface]",
169 clib_bihash_add_del_24_8 (&table->
ip_hash, &kv, 1 );
200 if (clib_bihash_search_24_8 (&table->
ip_hash, &kv, &val))
204 clib_bihash_add_del_24_8 (&table->
ip_hash, &kv, 0 );
243 int dst_address_length =
245 ip6_address_t *
mask = &table->
ip_masks[dst_address_length];
247 ASSERT (dst_address_length >= 0 && dst_address_length <= 128);
251 kv.
key[2] = ((
u64)
af << 32) | dst_address_length;
252 rv = clib_bihash_search_inline_2_24_8 (&table->
ip_hash, &kv, &val);
359 ip6_address_t
ip6 = { { 0 } };
395 .path =
"set cnat snat-policy addr",
397 "set cnat snat-policy addr [<ip4-address>][<ip6-address>][sw_if_index]",
433 .path =
"set cnat snat-policy prefix",
434 .short_help =
"set cnat snat-policy prefix [del] [prefix]",
467 .path =
"show cnat snat-policy",
468 .short_help =
"show cnat snat-policy",
502 else if (
unformat (input,
"if-pfx"))
516 .path =
"set cnat snat-policy",
517 .short_help =
"set cnat snat-policy [none][if-pfx][k8s]",
563 for (j = 0; j < i0; j++)
564 excluded_pfx->
ip_masks[
i].as_u32[j] = ~0;
568 clib_host_to_net_u32 (
pow2_mask (i1) << (32 - i1));
570 clib_bihash_init_24_8 (&excluded_pfx->
ip_hash,
"snat prefixes",
571 cm->snat_hash_buckets,
cm->snat_hash_memory);
572 clib_bihash_set_kvp_format_fn_24_8 (&excluded_pfx->
ip_hash,
#define vec_reset_length(v)
Reset vector length to zero NULL-pointer tolerant.
int cnat_snat_policy_add_pfx(ip_prefix_t *pfx)
static uword ip6_address_is_equal(const ip6_address_t *a, const ip6_address_t *b)
static_always_inline int cnat_snat_policy_interface_enabled(u32 sw_if_index, ip_address_family_t af)
ip46_address_t cs_ip[VLIB_N_DIR]
IP 4/6 address in the rx/tx direction.
uword unformat_ip_prefix(unformat_input_t *input, va_list *args)
static clib_error_t * cnat_snat_policy_add_del_pfx_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
u8 cnat_resolve_ep(cnat_endpoint_t *ep)
Resolve endpoint address.
u32 sw_if_index
The interface index to resolve.
static uword pow2_mask(uword x)
vl_api_ipsec_spd_action_t policy
static clib_error_t * cnat_snat_policy_set_cmd_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
u8 * format_cnat_snat_prefix(u8 *s, va_list *args)
void cnat_translation_register_addr_add_cb(cnat_addr_resol_type_t typ, cnat_if_addr_add_cb_t fn)
void cnat_translation_watch_addr(index_t cti, u64 opaque, cnat_endpoint_t *ep, cnat_addr_resol_type_t type)
Add an address resolution request.
#define clib_error_return(e, args...)
static void cnat_compute_prefix_lengths_in_search_order(cnat_snat_exclude_pfx_table_t *table, ip_address_family_t af)
void cnat_translation_unwatch_addr(u32 cti, cnat_addr_resol_type_t type)
Cleanup matching addr resolution requests.
static_always_inline int ip4_address_is_equal(const ip4_address_t *ip4_1, const ip4_address_t *ip4_2)
cnat_snat_exclude_pfx_table_t excluded_pfx
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
cnat_snat_pfx_table_meta_t meta[2]
A session represents the memory of a translation.
@ CNAT_SNAT_IF_MAP_INCLUDE_V4
struct cnat_session_t_::@645 key
this key sits in the same memory location a 'key' in the bihash kvp
u8 cs_af
The address family describing the IP addresses.
enum cnat_snat_interface_map_type_t_ cnat_snat_interface_map_type_t
static vlib_cli_command_t cnat_show_snat_command
(constructor) VLIB_CLI_COMMAND (cnat_show_snat_command)
int cnat_snat_policy_add_del_if(u32 sw_if_index, u8 is_add, cnat_snat_interface_map_type_t table)
void cnat_set_snat(ip4_address_t *ip4, ip6_address_t *ip6, u32 sw_if_index)
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
clib_bitmap_t * interface_maps[CNAT_N_SNAT_IF_MAP]
static u8 ip46_address_is_equal(const ip46_address_t *ip46_1, const ip46_address_t *ip46_2)
static uword clib_bitmap_get(uword *ai, uword i)
Gets the ith bit value from a bitmap.
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
static void cnat_if_addr_add_del_snat_cb(addr_resolution_t *ar, ip_address_t *address, u8 is_del)
vnet_main_t * vnet_get_main(void)
vl_api_mac_address_t src_addr
int cnat_snat_policy_none(vlib_buffer_t *b, cnat_session_t *session)
uword unformat_cnat_snat_interface_map_type(unformat_input_t *input, va_list *args)
#define static_always_inline
ip6_address_t ip_masks[129]
if(node->flags &VLIB_NODE_FLAG_TRACE) vnet_interface_output_trace(vm
@ CNAT_SNAT_IF_MAP_INCLUDE_V6
vl_api_mac_address_t dst_addr
static clib_error_t * cnat_snat_init(vlib_main_t *vm)
vnet_feature_config_main_t * cm
manual_print typedef address
#define VLIB_CLI_COMMAND(x,...)
void ip_address_set(ip_address_t *dst, const void *src, ip_address_family_t af)
static uword * clib_bitmap_set(uword *ai, uword i, uword value)
Sets the ith bit of a bitmap to new_value Removes trailing zeros from the bitmap.
int cnat_set_snat_policy(cnat_snat_policy_type_t policy)
int cnat_snat_policy_k8s(vlib_buffer_t *b, cnat_session_t *session)
void vlib_cli_output(vlib_main_t *vm, char *fmt,...)
int cnat_snat_policy_if_pfx(vlib_buffer_t *b, cnat_session_t *session)
static vlib_cli_command_t cnat_snat_policy_add_del_if_command
(constructor) VLIB_CLI_COMMAND (cnat_snat_policy_add_del_if_command)
#define clib_bitmap_validate(v, n_bits)
int cnat_snat_policy_del_pfx(ip_prefix_t *pfx)
format_function_t format_vnet_sw_if_index_name
unformat_function_t unformat_vnet_sw_interface
description fragment has unexpected format
#define VLIB_INIT_FUNCTION(x)
vl_api_address_family_t af
void cnat_lazy_init()
Lazy initialization when first adding a translation or using snat.
cnat_snat_policy_t snat_policy
ip_address_family_t af
ip4 or ip6 resolution
@ CNAT_SNAT_POLICY_IF_PFX
u8 * format_cnat_endpoint(u8 *s, va_list *args)
#define ip_prefix_version(_a)
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
int cnat_search_snat_prefix(ip46_address_t *addr, ip_address_family_t af)
static clib_error_t * cnat_set_snat_cli(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
u8 * format_cnat_snat_interface_map_type(u8 *s, va_list *args)
clib_bihash_24_8_t ip_hash
u8 cnat_resolve_addr(u32 sw_if_index, ip_address_family_t af, ip_address_t *addr)
enum cnat_snat_policy_type_t_ cnat_snat_policy_type_t
@ CNAT_SNAT_IF_MAP_INCLUDE_POD
#define clib_bitmap_foreach(i, ai)
Macro to iterate across set bits in a bitmap.
static vlib_cli_command_t cnat_snat_policy_set_cmd
(constructor) VLIB_CLI_COMMAND (cnat_snat_policy_set_cmd)
cnat_snat_policy_main_t cnat_snat_policy_main
static vlib_cli_command_t cnat_snat_policy_add_del_pfx_command
(constructor) VLIB_CLI_COMMAND (cnat_snat_policy_add_del_pfx_command)
#define INDEX_INVALID
Invalid index - used when no index is known blazoned capitals INVALID speak volumes where ~0 does not...
void ip_address_copy(ip_address_t *dst, const ip_address_t *src)
static vlib_cli_command_t cnat_set_snat_command
(constructor) VLIB_CLI_COMMAND (cnat_set_snat_command)
vl_api_interface_index_t sw_if_index
static clib_error_t * cnat_show_snat(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
Entry used to account for a translation's backend waiting for address resolution.
static clib_error_t * cnat_snat_policy_add_del_if_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
enum ip_address_family_t_ ip_address_family_t
VLIB buffer representation.