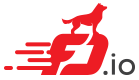 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
25 #define _(a, b, c) TUNNEL_ENCAP_DECAP_FLAG_##a |
30 #define _(a, b, c) TUNNEL_FLAG_##a |
42 #define _(n, v) case TUNNEL_MODE_##n: \
43 s = format (s, "%s", v); \
71 if (
f == TUNNEL_ENCAP_DECAP_FLAG_NONE)
75 else if (f & TUNNEL_ENCAP_DECAP_FLAG_##a) s = format (s, "%s ", b);
86 #define _(a,b,c) if (unformat(input, b)) {\
87 *f |= TUNNEL_ENCAP_DECAP_FLAG_##a;\
103 #define _(a, b, c) else if (f & TUNNEL_FLAG_##a) s = format (s, "%s ", c);
114 if (unformat (input, c)) \
116 *f |= TUNNEL_FLAG_##a; \
136 dst->t_encap_decap_flags =
src->t_encap_decap_flags;
137 dst->t_flags =
src->t_flags;
138 dst->t_mode =
src->t_mode;
139 dst->t_table_id =
src->t_table_id;
140 dst->t_dscp =
src->t_dscp;
141 dst->t_hop_limit =
src->t_hop_limit;
142 dst->t_fib_index =
src->t_fib_index;
144 dst->t_flags &= ~TUNNEL_FLAG_RESOLVED;
153 u32 indent = va_arg (*args,
u32);
155 s =
format (s,
"%Utable-ID:%d [%U->%U] hop-limit:%d %U %U [%U] [%U]",
161 if (t->
t_flags & TUNNEL_FLAG_RESOLVED)
198 return VNET_API_ERROR_NO_SUCH_FIB;
203 t->
t_flags |= TUNNEL_FLAG_RESOLVED;
211 if (t->
t_flags & TUNNEL_FLAG_RESOLVED)
214 t->
t_flags &= ~TUNNEL_FLAG_RESOLVED;
232 ip->ip_version_traffic_class_and_flow_label =
233 clib_host_to_net_u32 (0x60000000);
250 ip->ip_version_and_header_length = 0x45;
252 ip->src_address.as_u32 = t->
t_src.
ip.ip4.as_u32;
253 ip->dst_address.as_u32 = t->
t_dst.
ip.ip4.as_u32;
ip_address_family_t tunnel_get_af(const tunnel_t *t)
enum tunnel_encap_decap_flags_t_ tunnel_encap_decap_flags_t
u8 * format_tunnel_flags(u8 *s, va_list *args)
A representation of an IP tunnel config.
enum tunnel_flags_t_ tunnel_flags_t
void fib_entry_untrack(fib_node_index_t fei, u32 sibling)
Stop tracking a FIB entry.
u32 t_fib_index
derived data
const u8 TUNNEL_FLAG_MASK
#define FIB_NODE_INDEX_INVALID
vl_api_tunnel_mode_t mode
void ip_address_to_fib_prefix(const ip_address_t *addr, fib_prefix_t *prefix)
convert from a IP address to a FIB prefix
uword unformat_tunnel(unformat_input_t *input, va_list *args)
fib_node_index_t t_fib_entry_index
void tunnel_contribute_forwarding(const tunnel_t *t, dpo_id_t *dpo)
static_always_inline void ip6_set_dscp_network_order(ip6_header_t *ip6, ip_dscp_t dscp)
void tunnel_copy(const tunnel_t *src, tunnel_t *dst)
enum fib_node_type_t_ fib_node_type_t
The types of nodes in a FIB graph.
@ foreach_tunnel_encap_decap_flag
uword unformat_tunnel_mode(unformat_input_t *input, va_list *args)
void fib_entry_contribute_forwarding(fib_node_index_t fib_entry_index, fib_forward_chain_type_t fct, dpo_id_t *dpo)
u8 * format_tunnel_mode(u8 *s, va_list *args)
fib_node_index_t fib_entry_track(u32 fib_index, const fib_prefix_t *prefix, fib_node_type_t child_type, index_t child_index, u32 *sibling)
Trackers are used on FIB entries by objects that which to track the changing state of the entry.
void tunnel_build_v4_hdr(const tunnel_t *t, ip_protocol_t next_proto, ip4_header_t *ip)
#define ip_addr_version(_a)
uword unformat_ip_address(unformat_input_t *input, va_list *args)
static u32 ip6_compute_flow_hash(const ip6_header_t *ip, flow_hash_config_t flow_hash_config)
enum fib_forward_chain_type_t_ fib_forward_chain_type_t
FIB output chain type.
u32 index_t
A Data-Path Object is an object that represents actions that are applied to packets are they are swit...
#define IP_FLOW_HASH_DEFAULT
Default: 5-tuple + flowlabel without the "reverse" bit.
u8 * format_ip_address(u8 *s, va_list *args)
uword unformat_tunnel_encap_decap_flags(unformat_input_t *input, va_list *args)
static void ip6_address_copy(ip6_address_t *dst, const ip6_address_t *src)
static_always_inline void ip6_set_flow_label_network_order(ip6_header_t *ip6, u32 flow_label)
fib_protocol_t ip_address_family_to_fib_proto(ip_address_family_t af)
fib_forward_chain_type_t fib_forw_chain_type_from_fib_proto(fib_protocol_t proto)
Convert from a fib-protocol to a chain type.
description fragment has unexpected format
void tunnel_build_v6_hdr(const tunnel_t *t, ip_protocol_t next_proto, ip6_header_t *ip)
int tunnel_resolve(tunnel_t *t, fib_node_type_t child_type, index_t child_index)
uword unformat_tunnel_flags(unformat_input_t *input, va_list *args)
fib_protocol_t fp_proto
protocol type
vl_api_udp_decap_next_proto_t next_proto
u8 * format_tunnel_encap_decap_flags(u8 *s, va_list *args)
enum ip_protocol ip_protocol_t
enum tunnel_mode_t_ tunnel_mode_t
static u16 ip4_header_checksum(ip4_header_t *i)
const u8 TUNNEL_ENCAP_DECAP_FLAG_MASK
tunnel_encap_decap_flags_t t_encap_decap_flags
The identity of a DPO is a combination of its type and its instance number/index of objects of that t...
u8 * format_tunnel(u8 *s, va_list *args)
void ip_address_copy(ip_address_t *dst, const ip_address_t *src)
u32 fib_table_find(fib_protocol_t proto, u32 table_id)
Get the index of the FIB for a Table-ID.
unformat_function_t unformat_ip_dscp
u8 * format_ip_dscp(u8 *s, va_list *va)
Aggregate type for a prefix.
void tunnel_unresolve(tunnel_t *t)
enum ip_address_family_t_ ip_address_family_t