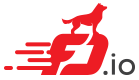 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
192 if (fib_entry_cover_index != fib_entry_index)
359 return (fib_entry_index);
401 return (fib_entry_index);
420 return (fib_entry_index);
510 (~0 ==
path->frp_sw_if_index) &&
519 path->frp_sw_if_index != ~0 &&
555 if (
path->frp_sw_if_index != ~0)
568 const ip46_address_t *next_hop,
569 u32 next_hop_sw_if_index,
570 u32 next_hop_fib_index,
576 .frp_proto = next_hop_proto,
577 .frp_addr = (NULL == next_hop?
zero_addr : *next_hop),
578 .frp_sw_if_index = next_hop_sw_if_index,
579 .frp_fib_index = next_hop_fib_index,
580 .frp_weight = next_hop_weight,
581 .frp_flags = path_flags,
583 .frp_label_stack = next_hop_labels,
594 return (fib_entry_index);
618 for (ii = 0; ii <
vec_len(rpaths); ii++)
650 return (fib_entry_index);
740 const ip46_address_t *next_hop,
741 u32 next_hop_sw_if_index,
742 u32 next_hop_fib_index,
753 .frp_proto = next_hop_proto,
754 .frp_addr = (NULL == next_hop?
zero_addr : *next_hop),
755 .frp_sw_if_index = next_hop_sw_if_index,
756 .frp_fib_index = next_hop_fib_index,
757 .frp_weight = next_hop_weight,
758 .frp_flags = path_flags,
815 return (fib_entry_index);
824 const ip46_address_t *next_hop,
825 u32 next_hop_sw_if_index,
826 u32 next_hop_fib_index,
833 .frp_proto = next_hop_proto,
834 .frp_addr = (NULL == next_hop?
zero_addr : *next_hop),
835 .frp_sw_if_index = next_hop_sw_if_index,
836 .frp_fib_index = next_hop_fib_index,
837 .frp_weight = next_hop_weight,
838 .frp_flags = path_flags,
839 .frp_label_stack = next_hop_labels,
850 return (fib_entry_index);
932 fib_entry_index,
prefix, source);
967 return (fib_entry_index);
1074 .hash_config = hash_config,
1096 return ((NULL != fib_table ? fib_table->
ft_table_id : ~0));
1107 return ((NULL != fib_table ? fib_table->
ft_table_id : ~0));
1152 if (NULL == fib_table->
ft_desc)
1191 const char *
const fmt,
1278 return (
ctx->fn(fei,
ctx->data));
1406 return format(s,
"none");
1410 if (1 << attr &
flags) {
1455 .ftf_entries = NULL,
1456 .ftf_source = source,
1490 .ftf_source = source,
1523 .ftf_source = source,
void fib_entry_mark(fib_node_index_t fib_entry_index, fib_source_t source)
static void fib_table_entry_remove(fib_table_t *fib_table, const fib_prefix_t *prefix, fib_node_index_t fib_entry_index)
void fib_entry_unlock(fib_node_index_t fib_entry_index)
fib_node_index_t fib_table_entry_path_add(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source, fib_entry_flag_t flags, dpo_proto_t next_hop_proto, const ip46_address_t *next_hop, u32 next_hop_sw_if_index, u32 next_hop_fib_index, u32 next_hop_weight, fib_mpls_label_t *next_hop_labels, fib_route_path_flags_t path_flags)
Add one path to an entry (aka route) in the FIB.
u32 ip4_fib_table_find_or_create_and_lock(u32 table_id, fib_source_t src)
Get or create an IPv4 fib.
int fib_route_path_cmp(const fib_route_path_t *rpath1, const fib_route_path_t *rpath2)
void ip6_fib_table_fwding_dpo_remove(u32 fib_index, const ip6_address_t *addr, u32 len, const dpo_id_t *dpo)
#define DPO_INVALID
An initialiser for DPOs declared on the stack.
fib_node_index_t fib_table_entry_special_dpo_add(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source, fib_entry_flag_t flags, const dpo_id_t *dpo)
Add a 'special' entry to the FIB that links to the DPO passed A special entry is an entry that the FI...
u32 ip6_fib_table_find_or_create_and_lock(u32 table_id, fib_source_t src)
Get or create an IPv6 fib.
static u32 fib_table_find_or_create_and_lock_i(fib_protocol_t proto, u32 table_id, fib_source_t src, const u8 *name)
void fib_entry_special_add(fib_node_index_t fib_entry_index, fib_source_t source, fib_entry_flag_t flags, const dpo_id_t *dpo)
const fib_prefix_t * fib_entry_get_prefix(fib_node_index_t fib_entry_index)
void fib_table_sweep(u32 fib_index, fib_protocol_t proto, fib_source_t source)
Signal that the table has converged, i.e.
void mpls_fib_table_entry_remove(mpls_fib_t *mf, mpls_label_t label, mpls_eos_bit_t eos)
u32 fib_table_get_table_id(u32 fib_index, fib_protocol_t proto)
Get the Table-ID of the FIB from protocol and index.
const ip46_address_t zero_addr
#include <vnet/feature/feature.h>
fib_node_index_t * ftf_entries
The list of entries to flush.
void fib_table_entry_delete(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source)
Delete a FIB entry.
void fib_table_mark(u32 fib_index, fib_protocol_t proto, fib_source_t source)
Resync all entries from a table for the source this is the mark part of the mark and sweep algorithm.
ip4_main_t ip4_main
Global ip4 main structure.
enum dpo_proto_t_ dpo_proto_t
Data path protocol.
fib_node_index_t fib_table_entry_update_one_path(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source, fib_entry_flag_t flags, dpo_proto_t next_hop_proto, const ip46_address_t *next_hop, u32 next_hop_sw_if_index, u32 next_hop_fib_index, u32 next_hop_weight, fib_mpls_label_t *next_hop_labels, fib_route_path_flags_t path_flags)
Update the entry to have just one path.
static fib_node_index_t fib_table_get_less_specific_i(fib_table_t *fib_table, const fib_prefix_t *prefix)
u32 fib_table_find_or_create_and_lock_w_name(fib_protocol_t proto, u32 table_id, fib_source_t src, const u8 *name)
Get the index of the FIB for a Table-ID.
void fib_entry_set_flow_hash_config(fib_node_index_t fib_entry_index, flow_hash_config_t hash_config)
static void fib_table_entry_insert(fib_table_t *fib_table, const fib_prefix_t *prefix, fib_node_index_t fib_entry_index)
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
void fib_table_sub_tree_walk(u32 fib_index, fib_protocol_t proto, const fib_prefix_t *root, fib_table_walk_fn_t fn, void *ctx)
Walk all entries in a sub-tree FIB table.
fib_source_t ftf_source
The source we are flushing.
u32 ft_epoch
Epoch - number of resyncs performed.
fib_node_index_t ip6_fib_table_lookup_exact_match(u32 fib_index, const ip6_address_t *addr, u32 len)
void fib_table_set_flow_hash_config(u32 fib_index, fib_protocol_t proto, flow_hash_config_t hash_config)
Set the flow hash configured used by the table.
flow_hash_config_t fib_table_get_default_flow_hash_config(fib_protocol_t proto)
Get the flow hash configured used by the protocol.
static void fib_table_entry_delete_i(u32 fib_index, fib_node_index_t fib_entry_index, const fib_prefix_t *prefix, fib_source_t source)
static int ip46_address_cmp(const ip46_address_t *ip46_1, const ip46_address_t *ip46_2)
u8 * format_fib_table_name(u8 *s, va_list *ap)
Format the description/name of the table.
#define FIB_NODE_INDEX_INVALID
u32 fib_table_get_num_entries(u32 fib_index, fib_protocol_t proto, fib_source_t source)
Return the number of entries in the FIB added by a given source.
u32 ip4_fib_table_get_index_for_sw_if_index(u32 sw_if_index)
#define ip4_fib_table_fwding_dpo_update
u16 fp_len
The mask length.
static void vlib_smp_unsafe_warning(void)
int fib_route_path_is_attached(const fib_route_path_t *rpath)
u32 ip4_fib_table_create_and_lock(fib_source_t src)
#define ip4_fib_table_entry_remove
fib_node_index_t fib_table_lookup(u32 fib_index, const fib_prefix_t *prefix)
Perfom a longest prefix match in the non-forwarding table.
void fib_table_walk(u32 fib_index, fib_protocol_t proto, fib_table_walk_fn_t fn, void *ctx)
Walk all entries in a FIB table N.B: This is NOT safe to deletes.
#define ip4_fib_table_walk
u32 mpls_fib_index_from_table_id(u32 table_id)
void ip6_fib_table_walk(u32 fib_index, fib_table_walk_fn_t fn, void *arg)
Walk all entries in a FIB table N.B: This is NOT safe to deletes.
@ FIB_ENTRY_FLAG_EXCLUSIVE
static fib_table_walk_rc_t fib_table_sweep_cb(fib_node_index_t fib_entry_index, void *arg)
u8 * format_fib_table_memory(u8 *s, va_list *args)
format (display) the memory used by the FIB tables
void fib_entry_inherit(fib_node_index_t cover, fib_node_index_t covered)
fib_entry_inherit
struct fib_table_flush_ctx_t_ fib_table_flush_ctx_t
Table flush context.
static u32 ip6_fib_index_from_table_id(u32 table_id)
enum fib_table_flags_t_ fib_table_flags_t
static fib_table_walk_rc_t fib_table_flush_cb(fib_node_index_t fib_entry_index, void *arg)
#define FOR_EACH_FIB_TABLE_ATTRIBUTE(_item)
fib_node_index_t fib_table_entry_special_dpo_update(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source, fib_entry_flag_t flags, const dpo_id_t *dpo)
Update a 'special' entry to the FIB that links to the DPO passed A special entry is an entry that the...
void fib_table_fwding_dpo_update(u32 fib_index, const fib_prefix_t *prefix, const dpo_id_t *dpo)
Add or update an entry in the FIB's forwarding table.
static void fib_table_lock_dec(fib_table_t *fib_table, fib_source_t source)
#define MPLS_FLOW_HASH_DEFAULT
There are no options for controlling the MPLS flow hash, but since it mostly entails using IP data to...
void ip6_fib_table_fwding_dpo_update(u32 fib_index, const ip6_address_t *addr, u32 len, const dpo_id_t *dpo)
u8 * format_ip6_fib_table_memory(u8 *s, va_list *args)
A protocol Independent FIB table.
void mpls_fib_table_destroy(u32 fib_index)
void fib_table_entry_special_remove(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source)
Remove a 'special' entry from the FIB.
static void fib_table_source_count_inc(fib_table_t *fib_table, fib_source_t source)
static u32 mpls_fib_table_get_index_for_sw_if_index(u32 sw_if_index)
u32 mpls_fib_table_find_or_create_and_lock(u32 table_id, fib_source_t src)
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
enum fib_entry_flag_t_ fib_entry_flag_t
u32 fib_table_get_table_id_for_sw_if_index(fib_protocol_t proto, u32 sw_if_index)
Get the Table-ID of the FIB bound to the interface.
fib_node_index_t fib_entry_create_special(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source, fib_entry_flag_t flags, const dpo_id_t *dpo)
static void fib_table_source_count_dec(fib_table_t *fib_table, fib_source_t source)
static void fib_table_lock_inc(fib_table_t *fib_table, fib_source_t source)
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
void fib_table_entry_path_remove(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source, dpo_proto_t next_hop_proto, const ip46_address_t *next_hop, u32 next_hop_sw_if_index, u32 next_hop_fib_index, u32 next_hop_weight, fib_route_path_flags_t path_flags)
remove one path to an entry (aka route) in the FIB.
fib_node_index_t ft_index
Index into FIB vector.
void fib_entry_lock(fib_node_index_t fib_entry_index)
u32 ft_table_id
Table ID (hash key) for this FIB.
void ip6_fib_table_entry_insert(u32 fib_index, const ip6_address_t *addr, u32 len, fib_node_index_t fib_entry_index)
void fib_entry_special_update(fib_node_index_t fib_entry_index, fib_source_t source, fib_entry_flag_t flags, const dpo_id_t *dpo)
static fib_table_walk_rc_t fib_table_set_flow_hash_config_cb(fib_node_index_t fib_entry_index, void *arg)
u32 ft_total_route_counts
Total route counters.
u32 fib_table_find_or_create_and_lock(fib_protocol_t proto, u32 table_id, fib_source_t src)
Get the index of the FIB for a Table-ID.
u8 * ft_desc
Table description.
static void fib_table_post_insert_actions(fib_table_t *fib_table, const fib_prefix_t *prefix, fib_node_index_t fib_entry_index)
enum flow_hash_config_t_ flow_hash_config_t
A flow hash configuration is a mask of the flow hash options.
int fib_entry_is_marked(fib_node_index_t fib_entry_index, fib_source_t source)
u32 * ft_locks
per-source number of locks on the table
static void fib_table_route_path_fixup(const fib_prefix_t *prefix, fib_entry_flag_t *eflags, fib_route_path_t *path)
fib_table_route_path_fixup
int fib_entry_is_host(fib_node_index_t fib_entry_index)
Return !0 is the entry represents a host prefix.
#define IP_FLOW_HASH_DEFAULT
Default: 5-tuple + flowlabel without the "reverse" bit.
u32 fib_node_index_t
A typedef of a node index.
void fib_table_flush(u32 fib_index, fib_protocol_t proto, fib_source_t source)
Flush all entries from a table for the source.
static int fib_route_path_cmp_for_sort(void *v1, void *v2)
void fib_entry_path_add(fib_node_index_t fib_entry_index, fib_source_t source, fib_entry_flag_t flags, const fib_route_path_t *rpaths)
static ip4_fib_t * ip4_fib_get(u32 index)
Get the FIB at the given index.
#define ip4_fib_table_entry_insert
const void * fib_entry_get_source_data(fib_node_index_t fib_entry_index, fib_source_t source)
fib_table_flags_t ft_flags
Table flags.
enum fib_entry_src_flag_t_ fib_entry_src_flag_t
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
fib_entry_flag_t fib_entry_get_flags_for_source(fib_node_index_t fib_entry_index, fib_source_t source)
struct fib_table_t_ * fibs
u32 ip6_fib_table_get_index_for_sw_if_index(u32 sw_if_index)
enum fib_protocol_t_ fib_protocol_t
Protocol Type.
#define ip4_fib_table_sub_tree_walk
u8 * format_mpls_fib_table_memory(u8 *s, va_list *args)
void fib_prefix_normalize(const fib_prefix_t *p, fib_prefix_t *out)
normalise a prefix (i.e.
struct fib_table_set_flow_hash_config_ctx_t_ fib_table_set_flow_hash_config_ctx_t
Table set flow hash config context.
dpo_proto_t fib_proto_to_dpo(fib_protocol_t fib_proto)
void ip4_fib_table_destroy(u32 fib_index)
static void fib_table_destroy(fib_table_t *fib_table)
fib_table_walk_rc_t(* fib_table_walk_fn_t)(fib_node_index_t fei, void *ctx)
Call back function when walking entries in a FIB table.
void fib_table_entry_local_label_remove(u32 fib_index, const fib_prefix_t *prefix, mpls_label_t label)
remove a MPLS local label for the prefix/route.
void mpls_fib_forwarding_table_update(mpls_fib_t *mf, mpls_label_t label, mpls_eos_bit_t eos, const dpo_id_t *dpo)
void mpls_fib_table_entry_insert(mpls_fib_t *mf, mpls_label_t label, mpls_eos_bit_t eos, fib_node_index_t lfei)
static u8 ip46_address_is_zero(const ip46_address_t *ip46)
flow_hash_config_t fib_table_get_flow_hash_config(u32 fib_index, fib_protocol_t proto)
Get the flow hash configured used by the table.
fib_node_index_t fib_table_lookup_exact_match(u32 fib_index, const fib_prefix_t *prefix)
Perfom an exact match in the non-forwarding table.
void mpls_fib_forwarding_table_reset(mpls_fib_t *mf, mpls_label_t label, mpls_eos_bit_t eos)
#define vec_free(V)
Free vector's memory (no header).
u8 * format_ip4_fib_table_memory(u8 *s, va_list *args)
fib_node_index_t fib_entry_create(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source, fib_entry_flag_t flags, const fib_route_path_t *paths)
u8 * format_fib_protocol(u8 *s, va_list *ap)
u32 ip6_fib_table_create_and_lock(fib_source_t src, fib_table_flags_t flags, u8 *desc)
void dpo_copy(dpo_id_t *dst, const dpo_id_t *src)
atomic copy a data-plane object.
fib_entry_src_flag_t fib_entry_delete(fib_node_index_t fib_entry_index, fib_source_t source)
fib_entry_delete
static mpls_fib_t * mpls_fib_get(fib_node_index_t index)
u32 ft_flow_hash_config
flow hash configuration
@ FIB_ENTRY_FLAG_CONNECTED
fib_node_index_t fib_table_entry_path_add2(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source, fib_entry_flag_t flags, fib_route_path_t *rpaths)
Add n paths to an entry (aka route) in the FIB.
enum fib_table_attribute_t_ fib_table_attribute_t
Flags for the source data.
int fib_entry_is_sourced(fib_node_index_t fib_entry_index, fib_source_t source)
description fragment has unexpected format
void fib_entry_cover_change_notify(fib_node_index_t cover_index, fib_node_index_t covered)
fib_table_t * fib_table_get(fib_node_index_t index, fib_protocol_t proto)
Get a pointer to a FIB table.
u32 fib_table_get_index_for_sw_if_index(fib_protocol_t proto, u32 sw_if_index)
Get the index of the FIB bound to the interface.
fib_node_index_t mpls_fib_table_lookup(const mpls_fib_t *mf, mpls_label_t label, mpls_eos_bit_t eos)
void fib_table_lock(u32 fib_index, fib_protocol_t proto, fib_source_t source)
Release a reference counting lock on the table.
u32 fib_table_create_and_lock(fib_protocol_t proto, fib_source_t src, const char *const fmt,...)
Create a new table with no table ID.
enum fib_table_walk_rc_t_ fib_table_walk_rc_t
return code controlling how a table walk proceeds
struct fib_table_t_ * fibs
Vector of FIBs.
#define ip4_fib_table_lookup
void fib_table_entry_path_remove2(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source, fib_route_path_t *rpaths)
Remove n paths to an entry (aka route) in the FIB.
A representation of a path as described by a route producer.
u8 * format_fib_prefix(u8 *s, va_list *args)
#define vec_foreach(var, vec)
Vector iterator.
#define ip4_fib_table_lookup_exact_match
void ip6_fib_table_sub_tree_walk(u32 fib_index, const fib_prefix_t *root, fib_table_walk_fn_t fn, void *arg)
Walk all entries in a sub-tree of the FIB table N.B: This is NOT safe to deletes.
const static char * fib_table_flags_strings[]
@ FIB_ROUTE_PATH_ATTACHED
Attached path.
fib_protocol_t fp_proto
protocol type
static fib_node_index_t fib_table_lookup_exact_match_i(const fib_table_t *fib_table, const fib_prefix_t *prefix)
u32 fib_entry_get_fib_index(fib_node_index_t fib_entry_index)
void dpo_unlock(dpo_id_t *dpo)
Release a reference counting lock on the DPO.
#define vec_sort_with_function(vec, f)
Sort a vector using the supplied element comparison function.
static fib_table_walk_rc_t fib_table_mark_cb(fib_node_index_t fib_entry_index, void *arg)
static u32 ip4_fib_index_from_table_id(u32 table_id)
@ FIB_TABLE_WALK_CONTINUE
Continue on to the next entry.
u8 * format_fib_table_flags(u8 *s, va_list *args)
Configuration for each label value in the output-stack.
const dpo_id_t * drop_dpo_get(dpo_proto_t proto)
fib_node_index_t ip6_fib_table_lookup(u32 fib_index, const ip6_address_t *addr, u32 len)
@ FIB_ROUTE_PATH_GLEAN
A path that resolves via a glean adjacency.
u32 fib_entry_get_stats_index(fib_node_index_t fib_entry_index)
@ FIB_SOURCE_MPLS
MPLS label.
void fib_table_unlock(u32 fib_index, fib_protocol_t proto, fib_source_t source)
Take a reference counting lock on the table.
enum fib_route_path_flags_t_ fib_route_path_flags_t
Path flags from the control plane.
void mpls_fib_table_walk(mpls_fib_t *mpls_fib, fib_table_walk_fn_t fn, void *ctx)
Walk all entries in a FIB table N.B: This is NOT safe to deletes.
@ FIB_ROUTE_PATH_DROP
A Drop path - resolve the path on the drop DPO.
fib_node_index_t fib_table_get_less_specific(u32 fib_index, const fib_prefix_t *prefix)
Get the less specific (covering) prefix.
#define clib_warning(format, args...)
static fib_node_index_t fib_table_lookup_i(fib_table_t *fib_table, const fib_prefix_t *prefix)
int fib_prefix_is_host(const fib_prefix_t *prefix)
Return true is the prefix is a host prefix.
fib_entry_src_flag_t fib_entry_special_remove(fib_node_index_t fib_entry_index, fib_source_t source)
enum fib_source_t_ fib_source_t
The different sources that can create a route.
The identity of a DPO is a combination of its type and its instance number/index of objects of that t...
u32 mpls_label_t
A label value only, i.e.
struct fib_table_t_ * fibs
A pool of all the MPLS FIBs.
void fib_table_entry_delete_index(fib_node_index_t fib_entry_index, fib_source_t source)
Delete a FIB entry.
struct fib_table_walk_w_src_ctx_t_ fib_table_walk_w_src_cxt_t
@ FIB_ENTRY_SRC_FLAG_ADDED
fib_entry_src_flag_t fib_entry_path_remove(fib_node_index_t fib_entry_index, fib_source_t source, const fib_route_path_t *rpaths)
@ FIB_ROUTE_PATH_EXCLUSIVE
Don't resolve the path, use the DPO the client provides.
fib_node_index_t fib_table_entry_local_label_add(u32 fib_index, const fib_prefix_t *prefix, mpls_label_t label)
Add a MPLS local label for the prefix/route.
fib_protocol_t ft_proto
Which protocol this table serves.
#define ip4_fib_table_fwding_dpo_remove
void fib_entry_update(fib_node_index_t fib_entry_index, fib_source_t source, fib_entry_flag_t flags, const fib_route_path_t *paths)
fib_entry_update
fib_node_index_t fib_table_entry_special_add(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source, fib_entry_flag_t flags)
Add a 'special' entry to the FIB.
#define FIB_TABLE_ATTRIBUTES
u32 fib_table_entry_get_stats_index(u32 fib_index, const fib_prefix_t *prefix)
Return the stats index for a FIB entry.
#define MPLS_LABEL_INVALID
#define INDEX_INVALID
Invalid index - used when no index is known blazoned capitals INVALID speak volumes where ~0 does not...
u32 fib_table_find(fib_protocol_t proto, u32 table_id)
Get the index of the FIB for a Table-ID.
u32 * ft_src_route_counts
Per-source route counters.
vl_api_interface_index_t sw_if_index
void ip6_fib_table_entry_remove(u32 fib_index, const ip6_address_t *addr, u32 len)
void fib_table_fwding_dpo_remove(u32 fib_index, const fib_prefix_t *prefix, const dpo_id_t *dpo)
remove an entry in the FIB's forwarding table
Aggregate type for a prefix.
fib_source_t fib_entry_get_best_source(fib_node_index_t entry_index)
@ FIB_ROUTE_PATH_LOCAL
A for-us/local path.
u32 mpls_fib_table_create_and_lock(fib_source_t src)
void fib_table_walk_w_src(u32 fib_index, fib_protocol_t proto, fib_source_t src, fib_table_walk_fn_t fn, void *data)
Walk all entries in a FIB table N.B: This is NOT safe to deletes.
flow_hash_config_t hash_config
the flow hash config to set
fib_node_index_t fib_table_entry_update(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source, fib_entry_flag_t flags, fib_route_path_t *paths)
Update an entry to have a new set of paths.
void fib_entry_set_source_data(fib_node_index_t fib_entry_index, fib_source_t source, const void *data)
Table set flow hash config context.
void ip6_fib_table_destroy(u32 fib_index)
static fib_table_walk_rc_t fib_table_walk_w_src_cb(fib_node_index_t fei, void *arg)
vl_api_wireguard_peer_flags_t flags