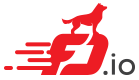 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
30 #define udp_error(n,s) s,
50 s =
format (s,
"UDP_INPUT: connection %d, disposition %d, thread %d",
55 #define foreach_udp_input_next \
56 _ (DROP, "error-drop")
60 #define _(s, n) UDP_INPUT_NEXT_##s,
75 #define udp_store_err_counters(vm, is_ip4, cnts) \
78 for (i = 0; i < UDP_N_ERROR; i++) \
80 udp_input_inc_counter(vm, is_ip4, i, cnts[i]); \
83 #define udp_inc_err_counter(cnts, err, val) \
114 uc->c_is_ip4 = hdr->
is_ip4;
115 uc->c_fib_index =
listener->c_fib_index;
117 uc->
flags |= UDP_CONN_F_CONNECTED;
126 (uc->c_lcl_port), uc->c_is_ip4);
137 if (!(uc0->
flags & UDP_CONN_F_CONNECTED))
143 *error0 = UDP_ERROR_FIFO_FULL;
167 if (!(uc0->
flags & UDP_CONN_F_CONNECTED))
199 &
ip4->src_address, udp->dst_port,
200 udp->src_port, TRANSPORT_PROTO_UDP);
213 udp->src_port, TRANSPORT_PROTO_UDP);
242 u32 error0 = UDP_ERROR_ENQUEUED;
250 error0 = UDP_ERROR_NO_LISTENER;
263 if (uc0->
flags & UDP_CONN_F_CONNECTED)
288 queue_event, &error0);
300 if (uc0->
flags & UDP_CONN_F_CONNECTED)
305 error0 = UDP_ERROR_CREATE_SESSION;
308 s0 =
session_get (uc0->c_s_index, uc0->c_thread_index);
309 error0 = UDP_ERROR_ACCEPT;
316 error0 = UDP_ERROR_NOT_READY;
333 err_counters[UDP_ERROR_MQ_FULL] = errors;
335 return frame->n_vectors;
349 .name =
"udp4-input",
350 .vector_size =
sizeof (
u32),
357 #define _(s, n) [UDP_INPUT_NEXT_##s] = n,
375 .name =
"udp6-input",
376 .vector_size =
sizeof (
u32),
383 #define _(s, n) [UDP_INPUT_NEXT_##s] = n,
static void vlib_buffer_free(vlib_main_t *vm, u32 *buffers, u32 n_buffers)
Free buffers Frees the entire buffer chain for each buffer.
void ip_set(ip46_address_t *dst, void *src, u8 is_ip4)
int session_enqueue_notify(session_t *s)
vlib_buffer_t * bufs[VLIB_FRAME_SIZE]
vlib_main_t vlib_node_runtime_t vlib_frame_t * frame
u32 session_index
Index in thread pool where session was allocated.
void udp_connection_free(udp_connection_t *uc)
vlib_get_buffers(vm, from, b, n_left_from)
@ VLIB_NODE_TYPE_INTERNAL
vlib_main_t vlib_node_runtime_t * node
u8 thread_index
Index of the thread that allocated the session.
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
int session_enqueue_dgram_connection(session_t *s, session_dgram_hdr_t *hdr, vlib_buffer_t *b, u8 proto, u8 queue_event)
session_t * session_lookup_safe6(u32 fib_index, ip6_address_t *lcl, ip6_address_t *rmt, u16 lcl_port, u16 rmt_port, u8 proto)
Lookup session with ip6 and transport layer information.
void udp_connection_share_port(u16 lcl_port, u8 is_ip4)
svm_fifo_t * rx_fifo
Pointers to rx/tx buffers.
transport_connection_t * session_get_transport(session_t *s)
#define VLIB_NODE_FLAG_TRACE
static void * vlib_frame_vector_args(vlib_frame_t *f)
Get pointer to frame vector data.
static_always_inline void clib_spinlock_lock(clib_spinlock_t *p)
int session_dgram_accept(transport_connection_t *tc, u32 listener_index, u32 thread_index)
session_t * session_lookup_safe4(u32 fib_index, ip4_address_t *lcl, ip4_address_t *rmt, u16 lcl_port, u16 rmt_port, u8 proto)
Lookup session with ip4 and transport layer information.
static void vlib_node_increment_counter(vlib_main_t *vm, u32 node_index, u32 counter_index, u64 increment)
int session_main_flush_enqueue_events(u8 transport_proto, u32 thread_index)
Flushes queue of sessions that are to be notified of new data enqueued events.
struct _vlib_node_registration vlib_node_registration_t
static session_t * session_get(u32 si, u32 thread_index)
u16 current_length
Nbytes between current data and the end of this buffer.
static u32 svm_fifo_max_enqueue_prod(svm_fifo_t *f)
Maximum number of bytes that can be enqueued into fifo.
description fragment has unexpected format
void ip_copy(ip46_address_t *dst, ip46_address_t *src, u8 is_ip4)
static_always_inline void clib_spinlock_unlock(clib_spinlock_t *p)
udp_connection_t * udp_connection_alloc(u32 thread_index)
void * vlib_add_trace(vlib_main_t *vm, vlib_node_runtime_t *r, vlib_buffer_t *b, u32 n_data_bytes)
static void * vlib_buffer_get_current(vlib_buffer_t *b)
Get pointer to current data to process.
clib_spinlock_t rx_lock
rx fifo lock
static udp_connection_t * udp_connection_clone_safe(u32 connection_index, u32 thread_index)
int session_dgram_connect_notify(transport_connection_t *tc, u32 old_thread_index, session_t **new_session)
Move dgram session to the right thread.
transport_connection_t connection
must be first
static void session_pool_remove_peeker(u32 thread_index)
u32 total_length_not_including_first_buffer
Only valid for first buffer in chain.
volatile u8 session_state
State in session layer state machine.
u32 connection_index
Index of the transport connection associated to the session.
static int svm_fifo_has_event(svm_fifo_t *f)
Check if fifo has io event.
vl_api_fib_path_type_t type
static udp_connection_t * udp_connection_from_transport(transport_connection_t *tc)
u32 flags
buffer flags: VLIB_BUFFER_FREE_LIST_INDEX_MASK: bits used to store free list index,...
VLIB buffer representation.
#define VLIB_REGISTER_NODE(x,...)