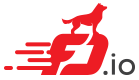 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
16 #include <vpp/app/version.h>
27 ip46_address_t
addr, *addrs;
28 u8 n_addrs4, n_addrs6;
38 n_addrs4 = n_addrs6 = 0;
61 else if (
unformat (input,
"no_preempt"))
63 else if (
unformat (input,
"accept_mode"))
65 else if (
unformat (input,
"unicast"))
113 case VNET_API_ERROR_ENTRY_ALREADY_EXISTS:
118 case VNET_API_ERROR_INVALID_SRC_ADDRESS:
123 case VNET_API_ERROR_ADDRESS_NOT_FOUND_FOR_INTERFACE:
125 "VR IP addresses not configured on interface");
130 case VNET_API_ERROR_NO_SUCH_ENTRY:
157 .path =
"vrrp vr add",
159 "vrrp vr add <interface> [vr_id <n>] [ipv6] [priority <value>] [interval <value>] [no_preempt] [accept_mode] [unicast] [<ip_addr> ...]",
174 .path =
"vrrp vr del",
175 .short_help =
"vrrp vr del <interface> [vr_id <n>] [ipv6]",
214 .path =
"show vrrp vr",
216 "show vrrp vr [(<intf_name>|sw_if_index <n>)]",
238 is_ipv6 = is_start = is_stop = 0;
258 if (is_start == is_stop)
275 case VNET_API_ERROR_INIT_FAILED:
296 ip46_address_t
addr, *addrs;
297 u8 n_addrs4, n_addrs6;
304 n_addrs4 = n_addrs6 = 0;
340 else if (n_addrs4 && (n_addrs6 ||
is_ipv6))
356 case VNET_API_ERROR_INVALID_ARGUMENT:
359 case VNET_API_ERROR_RSRC_IN_USE:
362 case VNET_API_ERROR_INVALID_DST_ADDRESS:
379 .path =
"vrrp proto",
381 "vrrp proto (start|stop) (<intf_name>|sw_if_index <n>) vr_id <n> [ipv6]",
389 .path =
"vrrp peers",
391 "vrrp peers (<intf_name>|sw_if_index <n>) vr_id <n> [ipv6] <peer1_addr> [<peer2_addr> ...]",
429 else if (
unformat (input,
"track-index %u priority %u", &track_if_index,
432 vec_add2 (track_intfs, track_intf, 1);;
433 track_intf->sw_if_index = track_if_index;
444 else if (is_add == is_del)
462 track_intf->sw_if_index);
465 if (!track_intf->priority)
473 "than VR priority (%u)",
493 .path =
"vrrp vr track-if",
495 "vrrp vr track-if (add|del) (<intf_name>|sw_if_index <n>) vr_id <n> [ipv6] track-index <n> priority <n> [ track-index <n> priority <n> ...]",
ip46_address_t * vr_addrs
static clib_error_t * vrrp_vr_del_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
#define clib_error_return(e, args...)
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
static vlib_cli_command_t vrrp_vr_track_if_command
(constructor) VLIB_CLI_COMMAND (vrrp_vr_track_if_command)
static uword vnet_sw_interface_is_valid(vnet_main_t *vnm, u32 sw_if_index)
#define pool_foreach(VAR, POOL)
Iterate through pool.
#define vec_add2(V, P, N)
Add N elements to end of vector V, return pointer to new elements in P.
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
vnet_main_t * vnet_get_main(void)
int vrrp_vr_set_peers(vrrp_vr_key_t *vr_key, ip46_address_t *peers)
static clib_error_t * vrrp_vr_track_if_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
static vlib_cli_command_t vrrp_proto_start_stop_command
(constructor) VLIB_CLI_COMMAND (vrrp_proto_start_stop_command)
static clib_error_t * vrrp_show_vr_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
static vlib_cli_command_t vrrp_peers_command
(constructor) VLIB_CLI_COMMAND (vrrp_peers_command)
#define VLIB_CLI_COMMAND(x,...)
static clib_error_t * vrrp_vr_add_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
u8 * format_vrrp_vr(u8 *s, va_list *args)
void vlib_cli_output(vlib_main_t *vm, char *fmt,...)
static vrrp_vr_t * vrrp_vr_lookup(u32 sw_if_index, u8 vr_id, u8 is_ipv6)
static clib_error_t * vrrp_vr_add_del_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd, u8 is_add)
#define vec_free(V)
Free vector's memory (no header).
static vlib_cli_command_t vrrp_show_vr_command
(constructor) VLIB_CLI_COMMAND (vrrp_show_vr_command)
unformat_function_t unformat_vnet_sw_interface
#define vec_foreach(var, vec)
Vector iterator.
int vrrp_vr_tracking_ifs_add_del(vrrp_vr_t *vr, vrrp_vr_tracking_if_t *track_ifs, u8 is_add)
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
int vrrp_vr_start_stop(u8 is_start, vrrp_vr_key_t *vr_key)
static vlib_cli_command_t vrrp_vr_del_command
(constructor) VLIB_CLI_COMMAND (vrrp_vr_del_command)
int vrrp_vr_add_del(u8 is_add, vrrp_vr_config_t *vr_conf)
static vlib_cli_command_t vrrp_vr_add_command
(constructor) VLIB_CLI_COMMAND (vrrp_vr_add_command)
vl_api_interface_index_t sw_if_index
static clib_error_t * vrrp_peers_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
static clib_error_t * vrrp_proto_start_stop_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)