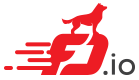 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
107 .name =
"ip4-lookup",
108 .vector_size =
sizeof (
u32),
135 u32 lbi0, hc0, lbi1, hc1;
266 return frame->n_vectors;
272 .name =
"ip4-load-balance",
273 .vector_size =
sizeof (
u32),
274 .sibling_of =
"ip4-lookup",
279 #ifndef CLIB_MARCH_VARIANT
301 *result_ia = result ? ia : 0;
357 .fp_len =
a->address_length,
359 .fp_addr.ip4.as_u32 =
address->as_u32 &
im->fib_masks[
a->address_length],
384 pfx_special.
fp_len =
a->address_length;
404 if (
a->address_length <= 30)
408 pfx_special.
fp_addr.ip4.as_u32 =
409 address->as_u32 &
im->fib_masks[
a->address_length];
419 pfx_special.
fp_addr.ip4.as_u32 =
420 address->as_u32 | ~
im->fib_masks[
a->address_length];
427 else if (
a->address_length == 31)
430 pfx_special.
fp_addr.ip4.as_u32 =
431 address->as_u32 ^ clib_host_to_net_u32(1);
509 .fp_len = address_length,
511 .fp_addr.ip4.as_u32 =
address->as_u32 &
im->fib_masks[address_length],
540 if (address_length <= 30)
545 pfx_special.
fp_addr.ip4.as_u32 =
546 address->as_u32 &
im->fib_masks[address_length];
555 pfx_special.
fp_addr.ip4.as_u32 =
556 address->as_u32 | ~
im->fib_masks[address_length];
564 else if (address_length == 31)
567 pfx_special.
fp_addr.ip4.as_u32 =
568 address->as_u32 ^ clib_host_to_net_u32(1);
575 pfx_special.
fp_len = address_length;
600 #ifndef CLIB_MARCH_VARIANT
633 else if (
hi->l3_if_count)
647 u32 address_length,
u32 is_del)
653 u32 if_address_index;
687 ip_interface_address_get_address
688 (&im->lookup_main, ia);
690 if (ip4_destination_matches_route
691 (im, address, x, ia->address_length) ||
692 ip4_destination_matches_route (im,
698 if ((sw_if_index == sif->sw_if_index) &&
699 (ia->address_length == address_length) &&
700 (x->as_u32 != address->as_u32))
703 if (ia->flags & IP_INTERFACE_ADDRESS_FLAG_STALE)
712 vnm->api_errno = VNET_API_ERROR_ADDRESS_IN_USE;
714 error = clib_error_create
715 (
"failed to add %U on %U which conflicts with %U for interface %U",
716 format_ip4_address_and_length, address,
718 format_vnet_sw_if_index_name, vnm,
720 format_ip4_address_and_length, x,
722 format_vnet_sw_if_index_name, vnm,
736 if (~0 == if_address_index)
738 vnm->api_errno = VNET_API_ERROR_ADDRESS_NOT_FOUND_FOR_INTERFACE;
740 lm->format_address_and_length,
741 addr_fib, address_length,
754 if (~0 != if_address_index)
780 addr_fib, address_length,
786 vnm->api_errno = VNET_API_ERROR_DUPLICATE_IF_ADDRESS;
788 (
"Prefix %U already found on interface %U",
789 lm->format_address_and_length, addr_fib, address_length,
795 addr_fib, address_length,
814 im, ip4_af.fib_index,
816 (lm->if_address_pool, if_address_index));
822 address, address_length, if_address_index, is_del);
833 u32 address_length,
u32 is_del)
855 if (ia->address_length <= 30)
859 ipa = ip_interface_address_get_address (&im->lookup_main, ia);
863 .fp_proto = FIB_PROTOCOL_IP4,
865 .ip4.as_u32 = (ipa->as_u32 | ~im->fib_masks[ia->address_length]),
869 ip4_add_subnet_bcast_route
870 (fib_table_get_index_for_sw_if_index(FIB_PROTOCOL_IP4,
885 u32 is_admin_up, fib_index;
888 lookup_main.if_address_pool_index_by_sw_if_index,
899 a = ip_interface_address_get_address (&im->lookup_main, ia);
901 ip4_add_interface_routes (sw_if_index,
905 ip4_del_interface_routes (sw_if_index,
907 a, ia->address_length);
920 .arc_name =
"ip4-unicast",
921 .start_nodes =
VNET_FEATURES (
"ip4-input",
"ip4-input-no-checksum"),
922 .last_in_arc =
"ip4-lookup",
928 .arc_name =
"ip4-unicast",
929 .node_name =
"ip4-flow-classify",
935 .arc_name =
"ip4-unicast",
936 .node_name =
"ip4-inacl",
942 .arc_name =
"ip4-unicast",
943 .node_name =
"ip4-source-and-port-range-check-rx",
949 .arc_name =
"ip4-unicast",
950 .node_name =
"ip4-policer-classify",
956 .arc_name =
"ip4-unicast",
957 .node_name =
"ipsec4-input-feature",
963 .arc_name =
"ip4-unicast",
964 .node_name =
"vpath-input-ip4",
970 .arc_name =
"ip4-unicast",
971 .node_name =
"ip4-vxlan-bypass",
977 .arc_name =
"ip4-unicast",
978 .node_name =
"ip4-not-enabled",
984 .arc_name =
"ip4-unicast",
985 .node_name =
"ip4-lookup",
992 .arc_name =
"ip4-multicast",
993 .start_nodes =
VNET_FEATURES (
"ip4-input",
"ip4-input-no-checksum"),
994 .last_in_arc =
"ip4-mfib-forward-lookup",
1000 .arc_name =
"ip4-multicast",
1001 .node_name =
"vpath-input-ip4",
1007 .arc_name =
"ip4-multicast",
1008 .node_name =
"ip4-not-enabled",
1014 .arc_name =
"ip4-multicast",
1015 .node_name =
"ip4-mfib-forward-lookup",
1022 .arc_name =
"ip4-output",
1023 .start_nodes =
VNET_FEATURES (
"ip4-rewrite",
"ip4-midchain",
"ip4-dvr-dpo"),
1024 .last_in_arc =
"interface-output",
1030 .arc_name =
"ip4-output",
1031 .node_name =
"ip4-source-and-port-range-check-tx",
1037 .arc_name =
"ip4-output",
1038 .node_name =
"ip4-outacl",
1044 .arc_name =
"ip4-output",
1045 .node_name =
"ipsec4-output-feature",
1052 .arc_name =
"ip4-output",
1053 .node_name =
"interface-output",
1103 #ifndef CLIB_MARCH_VARIANT
1131 im->fib_masks[
i] = clib_host_to_net_u32 (m);
1153 #define _16(f,v) h.f = clib_host_to_net_u16 (v);
1154 #define _8(f,v) h.f = v;
1155 _16 (l2_type, ETHERNET_ARP_HARDWARE_TYPE_ethernet);
1156 _16 (l3_type, ETHERNET_TYPE_IP4);
1157 _8 (n_l2_address_bytes, 6);
1158 _8 (n_l3_address_bytes, 4);
1159 _16 (opcode, ETHERNET_ARP_OPCODE_request);
1187 #ifndef CLIB_MARCH_VARIANT
1210 s =
format (s,
"fib %d dpo-idx %d flow hash: 0x%08x",
1226 s =
format (s,
"tx_sw_if_index %d dpo-idx %d : %U flow hash: 0x%08x",
1236 #ifndef CLIB_MARCH_VARIANT
1265 if (b0->
flags & VLIB_BUFFER_IS_TRACED)
1280 if (b1->
flags & VLIB_BUFFER_IS_TRACED)
1307 if (b0->
flags & VLIB_BUFFER_IS_TRACED)
1331 u32 ip_header_length, payload_length_host_byte_order;
1335 payload_length_host_byte_order =
1336 clib_net_to_host_u16 (ip0->
length) - ip_header_length;
1338 clib_host_to_net_u32 (payload_length_host_byte_order +
1355 payload_length_host_byte_order, (
u8 *) ip0,
1356 ip_header_length, NULL);
1367 || ip0->
protocol == IP_PROTOCOL_UDP);
1369 udp0 = (
void *) (ip0 + 1);
1372 p0->
flags |= (VNET_BUFFER_F_L4_CHECKSUM_COMPUTED
1373 | VNET_BUFFER_F_L4_CHECKSUM_CORRECT);
1379 p0->
flags |= (VNET_BUFFER_F_L4_CHECKSUM_COMPUTED
1380 | ((sum16 == 0) << VNET_BUFFER_F_LOG2_L4_CHECKSUM_CORRECT));
1389 .arc_name =
"ip4-local",
1391 .last_in_arc =
"ip4-local-end-of-arc",
1402 *good_tcp_udp = (flags0 & VNET_BUFFER_F_L4_CHECKSUM_CORRECT) != 0;
1406 u32 ip_len, udp_len;
1410 ip_len = clib_net_to_host_u16 (
ip->length);
1411 udp_len = clib_net_to_host_u16 (udp->
length);
1413 len_diff = ip_len - udp_len;
1414 *good_tcp_udp &= len_diff >= 0;
1415 *
error = len_diff < 0 ? IP4_ERROR_UDP_LENGTH : *
error;
1419 #define ip4_local_csum_is_offloaded(_b) \
1420 ((_b->flags & VNET_BUFFER_F_OFFLOAD) && \
1421 (vnet_buffer (_b)->oflags & \
1422 (VNET_BUFFER_OFFLOAD_F_TCP_CKSUM | VNET_BUFFER_OFFLOAD_F_UDP_CKSUM)))
1424 #define ip4_local_need_csum_check(is_tcp_udp, _b) \
1425 (is_tcp_udp && !(_b->flags & VNET_BUFFER_F_L4_CHECKSUM_COMPUTED \
1426 || ip4_local_csum_is_offloaded (_b)))
1428 #define ip4_local_csum_is_valid(_b) \
1429 (_b->flags & VNET_BUFFER_F_L4_CHECKSUM_CORRECT \
1430 || (ip4_local_csum_is_offloaded (_b))) != 0
1436 u8 is_udp, is_tcp_udp, good_tcp_udp;
1438 is_udp = ih->
protocol == IP_PROTOCOL_UDP;
1439 is_tcp_udp = is_udp || ih->
protocol == IP_PROTOCOL_TCP;
1446 ASSERT (IP4_ERROR_TCP_CHECKSUM + 1 == IP4_ERROR_UDP_CHECKSUM);
1447 *
error = (is_tcp_udp && !good_tcp_udp
1448 ? IP4_ERROR_TCP_CHECKSUM + is_udp : *
error);
1455 u8 is_udp[2], is_tcp_udp[2], good_tcp_udp[2];
1457 is_udp[0] = ih[0]->
protocol == IP_PROTOCOL_UDP;
1458 is_udp[1] = ih[1]->
protocol == IP_PROTOCOL_UDP;
1460 is_tcp_udp[0] = is_udp[0] || ih[0]->
protocol == IP_PROTOCOL_TCP;
1461 is_tcp_udp[1] = is_udp[1] || ih[1]->
protocol == IP_PROTOCOL_TCP;
1477 error[0] = (is_tcp_udp[0] && !good_tcp_udp[0] ?
1478 IP4_ERROR_TCP_CHECKSUM + is_udp[0] :
error[0]);
1479 error[1] = (is_tcp_udp[1] && !good_tcp_udp[1] ?
1480 IP4_ERROR_TCP_CHECKSUM + is_udp[1] :
error[1]);
1486 u8 head_of_feature_arc)
1493 if (head_of_feature_arc)
1556 *error0 = ((*error0 == IP4_ERROR_UNKNOWN_PROTOCOL
1558 IP4_ERROR_SPOOFED_LOCAL_PACKETS : *error0);
1559 *error0 = ((*error0 == IP4_ERROR_UNKNOWN_PROTOCOL
1562 IP4_ERROR_SRC_LOOKUP_MISS : *error0);
1565 last_check->
lbi = lbi0;
1566 last_check->
error = *error0;
1567 last_check->
first = 0;
1574 *error0 = last_check->
error;
1587 not_last_hit = last_check->
first;
1588 not_last_hit |=
ip[0]->src_address.as_u32 ^ last_check->
src.
as_u32;
1589 not_last_hit |=
ip[1]->src_address.as_u32 ^ last_check->
src.
as_u32;
1611 &
ip[0]->src_address, &
ip[1]->src_address, &lbi[0], &lbi[1]);
1627 error[0] = ((
error[0] == IP4_ERROR_UNKNOWN_PROTOCOL &&
1629 IP4_ERROR_SPOOFED_LOCAL_PACKETS :
error[0]);
1630 error[0] = ((
error[0] == IP4_ERROR_UNKNOWN_PROTOCOL &&
1632 ip[0]->dst_address.as_u32 != 0xFFFFFFFF)
1633 ? IP4_ERROR_SRC_LOOKUP_MISS :
error[0]);
1635 error[1] = ((
error[1] == IP4_ERROR_UNKNOWN_PROTOCOL &&
1637 IP4_ERROR_SPOOFED_LOCAL_PACKETS :
error[1]);
1638 error[1] = ((
error[1] == IP4_ERROR_UNKNOWN_PROTOCOL &&
1640 ip[1]->dst_address.as_u32 != 0xFFFFFFFF)
1641 ? IP4_ERROR_SRC_LOOKUP_MISS :
error[1]);
1643 last_check->
src.
as_u32 =
ip[1]->src_address.as_u32;
1644 last_check->
lbi = lbi[1];
1646 last_check->
first = 0;
1718 .error = IP4_ERROR_UNKNOWN_PROTOCOL,
1745 error[0] =
error[1] = IP4_ERROR_UNKNOWN_PROTOCOL;
1756 not_batch = pt[0] ^ pt[1];
1758 if (head_of_feature_arc == 0 || (pt[0] && not_batch == 0))
1783 head_of_feature_arc);
1785 head_of_feature_arc);
1794 error[0] = IP4_ERROR_UNKNOWN_PROTOCOL;
1800 if (head_of_feature_arc == 0 || pt[0])
1809 head_of_feature_arc);
1817 return frame->n_vectors;
1829 .name =
"ip4-local",
1830 .vector_size =
sizeof (
u32),
1856 .name =
"ip4-local-end-of-arc",
1857 .vector_size =
sizeof (
u32),
1860 .sibling_of =
"ip4-local",
1864 .arc_name =
"ip4-local",
1865 .node_name =
"ip4-local-end-of-arc",
1870 #ifndef CLIB_MARCH_VARIANT
1934 .path =
"show ip local",
1936 .short_help =
"show ip local",
1952 #if CLIB_ARCH_IS_BIG_ENDIAN
1953 #define IP4_MCAST_ADDR_MASK 0x007fffff
1955 #define IP4_MCAST_ADDR_MASK 0xffff7f00
1963 if (packet_len > adj_packet_bytes)
1965 *
error = IP4_ERROR_MTU_EXCEEDED;
1969 (
b, ICMP4_destination_unreachable,
1970 ICMP4_destination_unreachable_fragmentation_needed_and_dont_fragment_set,
1998 checksum =
ip->checksum - clib_host_to_net_u16 (0x0100);
1999 checksum += checksum >= 0xffff;
2001 ip->checksum = checksum;
2024 checksum =
ip->checksum + clib_host_to_net_u16 (0x0100);
2025 checksum += checksum >= 0xffff;
2027 ip->checksum = checksum;
2037 *
error = IP4_ERROR_TIME_EXPIRED;
2040 ICMP4_time_exceeded_ttl_exceeded_in_transit,
2047 (
vnet_buffer (
b)->oflags & VNET_BUFFER_OFFLOAD_F_IP_CKSUM) ||
2048 (
vnet_buffer (
b)->oflags & VNET_BUFFER_OFFLOAD_F_OUTER_IP_CKSUM));
2070 #if (CLIB_N_PREFETCHES >= 8)
2074 for (
i = 2;
i < 6;
i++)
2084 u32 rw_len0, error0, adj_index0;
2085 u32 rw_len1, error1, adj_index1;
2086 u32 tx_sw_if_index0, tx_sw_if_index1;
2112 error0 = error1 = IP4_ERROR_NONE;
2122 rw_len0 = adj0[0].rewrite_header.data_bytes;
2123 rw_len1 = adj1[0].rewrite_header.data_bytes;
2136 u16 ip0_len = clib_net_to_host_u16 (ip0->
length);
2137 u16 ip1_len = clib_net_to_host_u16 (ip1->
length);
2139 if (
b[0]->
flags & VNET_BUFFER_F_GSO)
2141 if (
b[1]->
flags & VNET_BUFFER_F_GSO)
2145 adj0[0].rewrite_header.max_l3_packet_bytes,
2148 next + 0, is_midchain, &error0);
2150 adj1[0].rewrite_header.max_l3_packet_bytes,
2153 next + 1, is_midchain, &error1);
2157 error0 = ((adj0[0].rewrite_header.sw_if_index ==
2159 IP4_ERROR_SAME_INTERFACE : error0);
2160 error1 = ((adj1[0].rewrite_header.sw_if_index ==
2162 IP4_ERROR_SAME_INTERFACE : error1);
2172 tx_sw_if_index0 = adj0[0].rewrite_header.sw_if_index;
2190 if (error0 == IP4_ERROR_MTU_EXCEEDED)
2198 tx_sw_if_index1 = adj1[0].rewrite_header.sw_if_index;
2215 if (error1 == IP4_ERROR_MTU_EXCEEDED)
2230 if (error0 == IP4_ERROR_NONE)
2237 if (error1 == IP4_ERROR_NONE)
2247 if (error0 == IP4_ERROR_NONE)
2249 if (error1 == IP4_ERROR_NONE)
2256 if (error0 == IP4_ERROR_NONE)
2258 adj0->rewrite_header.dst_mcast_offset,
2260 if (error1 == IP4_ERROR_NONE)
2262 adj1->rewrite_header.dst_mcast_offset,
2270 #elif (CLIB_N_PREFETCHES >= 4)
2277 u32 rw_len0, error0, adj_index0;
2278 u32 tx_sw_if_index0;
2311 error0 = IP4_ERROR_NONE;
2319 rw_len0 = adj0[0].rewrite_header.data_bytes;
2323 u16 ip0_len = clib_net_to_host_u16 (ip0->
length);
2325 if (
b[0]->
flags & VNET_BUFFER_F_GSO)
2329 adj0[0].rewrite_header.max_l3_packet_bytes,
2332 next + 0, is_midchain, &error0);
2336 error0 = ((adj0[0].rewrite_header.sw_if_index ==
2338 IP4_ERROR_SAME_INTERFACE : error0);
2347 tx_sw_if_index0 = adj0[0].rewrite_header.sw_if_index;
2387 adj0->rewrite_header.dst_mcast_offset,
2393 if (error0 == IP4_ERROR_MTU_EXCEEDED)
2407 u32 rw_len0, adj_index0, error0;
2408 u32 tx_sw_if_index0;
2420 error0 = IP4_ERROR_NONE;
2426 rw_len0 = adj0[0].rewrite_header.data_bytes;
2430 u16 ip0_len = clib_net_to_host_u16 (ip0->
length);
2431 if (
b[0]->
flags & VNET_BUFFER_F_GSO)
2435 adj0[0].rewrite_header.max_l3_packet_bytes,
2438 next + 0, is_midchain, &error0);
2442 error0 = ((adj0[0].rewrite_header.sw_if_index ==
2444 IP4_ERROR_SAME_INTERFACE : error0);
2453 tx_sw_if_index0 = adj0[0].rewrite_header.sw_if_index;
2490 adj0->rewrite_header.dst_mcast_offset,
2497 if (error0 == IP4_ERROR_MTU_EXCEEDED)
2512 return frame->n_vectors;
2598 .name =
"ip4-rewrite",
2599 .vector_size =
sizeof (
u32),
2612 .name =
"ip4-rewrite-bcast",
2613 .vector_size =
sizeof (
u32),
2616 .sibling_of =
"ip4-rewrite",
2620 .name =
"ip4-rewrite-mcast",
2621 .vector_size =
sizeof (
u32),
2624 .sibling_of =
"ip4-rewrite",
2628 .name =
"ip4-mcast-midchain",
2629 .vector_size =
sizeof (
u32),
2632 .sibling_of =
"ip4-rewrite",
2636 .name =
"ip4-midchain",
2637 .vector_size =
sizeof (
u32),
2639 .sibling_of =
"ip4-rewrite",
2650 u32 flow_hash_config = 0;
2657 #define _(a, b, v) \
2658 else if (unformat (input, #a)) \
2660 flow_hash_config |= v; \
2679 case VNET_API_ERROR_NO_SUCH_FIB:
2683 clib_warning (
"BUG: illegal flow hash config 0x%x", flow_hash_config);
2776 .path =
"set ip flow-hash",
2778 "set ip flow-hash table <table-id> [src] [dst] [sport] [dport] [proto] [reverse]",
2783 #ifndef CLIB_MARCH_VARIANT
2796 return VNET_API_ERROR_NO_MATCHING_INTERFACE;
2799 return VNET_API_ERROR_NO_SUCH_ENTRY;
2806 if (NULL != if_addr)
2811 .fp_addr.ip4 = *if_addr,
2819 if (table_index != (
u32) ~ 0)
2850 u32 table_index = ~0;
2851 int table_index_set = 0;
2857 if (
unformat (input,
"table-index %d", &table_index))
2858 table_index_set = 1;
2866 if (table_index_set == 0)
2879 case VNET_API_ERROR_NO_MATCHING_INTERFACE:
2882 case VNET_API_ERROR_NO_SUCH_ENTRY:
2901 .path =
"set ip classify",
2903 "set ip classify intfc <interface> table-index <classify-idx>",
@ IP_INTERFACE_ADDRESS_FLAG_STALE
static void ip4_local_check_l4_csum(vlib_main_t *vm, vlib_buffer_t *b, ip4_header_t *ih, u8 *error)
static clib_error_t * mfib_module_init(vlib_main_t *vm)
static int adj_are_counters_enabled(void)
Get the global configuration option for enabling per-adj counters.
u16 dpoi_next_node
The next VLIB node to follow.
vnet_interface_main_t * im
u16 ip4_tcp_udp_compute_checksum(vlib_main_t *vm, vlib_buffer_t *p0, ip4_header_t *ip0)
ip_interface_prefix_t * if_prefix_pool
Pool of prefixes containing addresses assigned to interfaces.
mhash_t prefix_to_if_prefix_index
Hash table mapping prefix to index in interface prefix pool.
u32 ip4_tcp_udp_validate_checksum(vlib_main_t *vm, vlib_buffer_t *p0)
u16 lb_n_buckets
number of buckets in the load-balance.
ip_interface_prefix_key_t key
static void * ip_interface_address_get_address(ip_lookup_main_t *lm, ip_interface_address_t *a)
index_t dpoi_index
the index of objects of that type
void ip4_mfib_interface_enable_disable(u32 sw_if_index, int is_enable)
Add/remove the interface from the accepting list of the special MFIB entries.
#define DPO_INVALID
An initialiser for DPOs declared on the stack.
fib_node_index_t fib_table_entry_special_dpo_add(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source, fib_entry_flag_t flags, const dpo_id_t *dpo)
Add a 'special' entry to the FIB that links to the DPO passed A special entry is an entry that the FI...
#define IP4_HEADER_FLAG_DONT_FRAGMENT
vlib_buffer_t * bufs[VLIB_FRAME_SIZE]
#define foreach_ip_interface_address(lm, a, sw_if_index, loop, body)
#define vlib_prefetch_buffer_header(b, type)
Prefetch buffer metadata.
vlib_main_t vlib_node_runtime_t vlib_frame_t * frame
ip_lookup_main_t lookup_main
static uword vlib_node_add_next(vlib_main_t *vm, uword node, uword next_node)
u8 ucast_feature_arc_index
#define clib_memcpy(d, s, n)
@ IP4_REWRITE_NEXT_ICMP_ERROR
void fib_table_entry_delete(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source)
Delete a FIB entry.
nat44_ei_hairpin_src_next_t next_index
void ip4_sw_interface_enable_disable(u32 sw_if_index, u32 is_enable)
static void ip4_add_subnet_bcast_route(u32 fib_index, fib_prefix_t *pfx, u32 sw_if_index)
@ IP_LOCAL_PACKET_TYPE_NAT
vlib_node_registration_t ip4_rewrite_bcast_node
(constructor) VLIB_REGISTER_NODE (ip4_rewrite_bcast_node)
fib_node_index_t fib_table_entry_update_one_path(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source, fib_entry_flag_t flags, dpo_proto_t next_hop_proto, const ip46_address_t *next_hop, u32 next_hop_sw_if_index, u32 next_hop_fib_index, u32 next_hop_weight, fib_mpls_label_t *next_hop_labels, fib_route_path_flags_t path_flags)
Update the entry to have just one path.
static vlib_buffer_t * vlib_get_buffer(vlib_main_t *vm, u32 buffer_index)
Translate buffer index into buffer pointer.
dpo_type_t dpoi_type
the type
static uword pow2_mask(uword x)
#define IP4_LOOKUP_NEXT_NODES
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
static int fib_urpf_check_size(index_t ui)
Data-Plane function to check the size of an uRPF list, (i.e.
static vlib_cli_command_t set_ip_flow_hash_command
(constructor) VLIB_CLI_COMMAND (set_ip_flow_hash_command)
static_always_inline void clib_memset_u16(void *p, u16 val, uword count)
enum vnet_sw_interface_flags_t_ vnet_sw_interface_flags_t
static_always_inline void ip4_ttl_inc(vlib_buffer_t *b, ip4_header_t *ip)
void ip4_directed_broadcast(u32 sw_if_index, u8 enable)
static void ip4_mtu_check(vlib_buffer_t *b, u16 packet_len, u16 adj_packet_bytes, bool df, u16 *next, u8 is_midchain, u32 *error)
static uword ip4_header_checksum_is_valid(ip4_header_t *i)
vlib_get_buffers(vm, from, b, n_left_from)
VNET_FEATURE_INIT(ip4_flow_classify, static)
@ IP_LOCAL_PACKET_TYPE_FRAG
vlib_main_t vlib_node_runtime_t * node
flow_hash_config_t lb_hash_config
the hash config to use when selecting a bucket.
vnet_classify_main_t vnet_classify_main
@ FIB_ENTRY_FLAG_ATTACHED
u32 mfib_table_find_or_create_and_lock(fib_protocol_t proto, u32 table_id, mfib_source_t src)
Get the index of the FIB for a Table-ID.
#define clib_error_return(e, args...)
const ip46_address_t ADJ_BCAST_ADDR
The special broadcast address (to construct a broadcast adjacency.
vlib_combined_counter_main_t lbm_via_counters
ip4_main_t ip4_main
Global ip4 main structure.
u16 fp_len
The mask length.
@ VNET_REWRITE_HAS_FEATURES
This adjacency/interface has output features configured.
#define vlib_call_init_function(vm, x)
@ VNET_SW_INTERFACE_FLAG_ADMIN_UP
#define pool_put(P, E)
Free an object E in pool P.
u32 * classify_table_index_by_sw_if_index
First table index to use for this interface, ~0 => none.
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
vlib_buffer_enqueue_to_next(vm, node, from,(u16 *) nexts, frame->n_vectors)
@ VNET_SW_INTERFACE_FLAG_DIRECTED_BCAST
static_always_inline void vnet_calc_checksums_inline(vlib_main_t *vm, vlib_buffer_t *b, int is_ip4, int is_ip6)
index_t classify_dpo_create(dpo_proto_t proto, u32 classify_table_index)
clib_error_t * ip_interface_address_add(ip_lookup_main_t *lm, u32 sw_if_index, void *addr_fib, u32 address_length, u32 *result_if_address_index)
static u16 ip_calculate_l4_checksum(vlib_main_t *vm, vlib_buffer_t *p0, ip_csum_t sum0, u32 payload_length, u8 *iph, u32 ip_header_size, u8 *l4h)
static uword vlib_buffer_length_in_chain(vlib_main_t *vm, vlib_buffer_t *b)
Get length in bytes of the buffer chain.
static_always_inline void * clib_memcpy_fast(void *restrict dst, const void *restrict src, size_t n)
static u8 * format_ip4_lookup_trace(u8 *s, va_list *args)
static u8 * format_ip4_rewrite_trace(u8 *s, va_list *args)
static void ip4_local_l4_csum_validate(vlib_main_t *vm, vlib_buffer_t *p, ip4_header_t *ip, u8 is_udp, u8 *error, u8 *good_tcp_udp)
static vlib_cli_command_t set_ip_classify_command
(constructor) VLIB_CLI_COMMAND (set_ip_classify_command)
static_always_inline void * vnet_feature_arc_start_w_cfg_index(u8 arc, u32 sw_if_index, u32 *next, vlib_buffer_t *b, u32 cfg_index)
void ip_frag_set_vnet_buffer(vlib_buffer_t *b, u16 mtu, u8 next_index, u8 flags)
#define pool_is_free_index(P, I)
Use free bitmap to query whether given index is free.
#define vec_elt(v, i)
Get vector value at index i.
@ FIB_ENTRY_FLAG_LOOSE_URPF_EXEMPT
#define CLIB_PREFETCH(addr, size, type)
i16 current_data
signed offset in data[], pre_data[] that we are currently processing.
static clib_error_t * ip4_add_del_interface_address_internal(vlib_main_t *vm, u32 sw_if_index, ip4_address_t *address, u32 address_length, u32 is_del)
void fib_table_entry_special_remove(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source)
Remove a 'special' entry from the FIB.
vlib_node_registration_t ip4_local_end_of_arc_node
(constructor) VLIB_REGISTER_NODE (ip4_local_end_of_arc_node)
vlib_error_t * errors
Vector of errors for this node.
#define pool_foreach(VAR, POOL)
Iterate through pool.
load_balance_main_t load_balance_main
The one instance of load-balance main.
static void vlib_buffer_advance(vlib_buffer_t *b, word l)
Advance current data pointer by the supplied (signed!) amount.
static const dpo_id_t * load_balance_get_fwd_bucket(const load_balance_t *lb, u16 bucket)
#define clib_error_create(args...)
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
vlib_error_t error
Error code for buffers to be enqueued to error handler.
static clib_error_t * ip4_sw_interface_add_del(vnet_main_t *vnm, u32 sw_if_index, u32 is_add)
#define VLIB_NODE_FN(node)
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
static clib_error_t * ip4_mtrie_module_init(vlib_main_t *vm)
int vnet_sw_interface_update_unnumbered(u32 unnumbered_sw_if_index, u32 ip_sw_if_index, u8 enable)
void ip_lookup_init(ip_lookup_main_t *lm, u32 is_ip6)
@ FIB_SOURCE_INTERFACE
Route added as a result of interface configuration.
unformat_function_t * unformat_edit
static int ip4_is_fragment(const ip4_header_t *i)
static vnet_sw_interface_flags_t vnet_sw_interface_get_flags(vnet_main_t *vnm, u32 sw_if_index)
vnet_main_t * vnet_get_main(void)
u8 mcast_feature_arc_index
Feature arc indices.
#define VLIB_NODE_FLAG_TRACE
@ IP_LOCAL_NEXT_REASSEMBLY
static void ip4_addr_fib_init(ip4_address_fib_t *addr_fib, const ip4_address_t *address, u32 fib_index)
static_always_inline void ip4_ttl_and_checksum_check(vlib_buffer_t *b, ip4_header_t *ip, u16 *next, u32 *error)
u32 fib_table_find_or_create_and_lock(fib_protocol_t proto, u32 table_id, fib_source_t src)
Get the index of the FIB for a Table-ID.
static uword ip4_lookup_inline(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame)
static vlib_cli_command_t show_ip_local
(constructor) VLIB_CLI_COMMAND (show_ip_local)
static void * vlib_frame_vector_args(vlib_frame_t *f)
Get pointer to frame vector data.
static void vlib_prefetch_combined_counter(const vlib_combined_counter_main_t *cm, u32 thread_index, u32 index)
Pre-fetch a per-thread combined counter for the given object index.
int ip_flow_hash_set(ip_address_family_t af, u32 table_id, u32 flow_hash_config)
#define static_always_inline
#define vlib_prefetch_buffer_with_index(vm, bi, type)
Prefetch buffer metadata by buffer index The first 64 bytes of buffer contains most header informatio...
static clib_error_t * ip4_lookup_init(vlib_main_t *vm)
u8 * format_ip4_forward_next_trace(u8 *s, va_list *args)
static pg_node_t * pg_get_node(uword node_index)
@ MFIB_SOURCE_DEFAULT_ROUTE
static u8 ip4_local_classify(vlib_buffer_t *b, ip4_header_t *ip, u16 *next)
Determine packet type and next node.
vlib_node_registration_t ip4_rewrite_mcast_node
(constructor) VLIB_REGISTER_NODE (ip4_rewrite_mcast_node)
#define clib_mem_unaligned(pointer, type)
static clib_error_t * ip4_sw_interface_admin_up_down(vnet_main_t *vnm, u32 sw_if_index, u32 flags)
static vlib_node_t * vlib_get_node(vlib_main_t *vm, u32 i)
Get vlib node by index.
static const dpo_id_t * load_balance_get_bucket_i(const load_balance_t *lb, u32 bucket)
static ip_interface_prefix_t * ip_get_interface_prefix(ip_lookup_main_t *lm, ip_interface_prefix_key_t *k)
vnet_feature_config_main_t * cm
#define pool_get(P, E)
Allocate an object E from a pool P (unspecified alignment).
@ FIB_SOURCE_CLASSIFY
Classify.
@ IP_FRAG_NEXT_IP_REWRITE
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
@ IP4_REWRITE_NEXT_FRAGMENT
manual_print typedef address
#define VLIB_CLI_COMMAND(x,...)
int vnet_set_ip4_classify_intfc(vlib_main_t *vm, u32 sw_if_index, u32 table_index)
static clib_error_t * vnet_feature_init(vlib_main_t *vm)
VNET_SW_INTERFACE_ADD_DEL_FUNCTION(ip4_sw_interface_add_del)
static clib_error_t * show_ip_local_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
static_always_inline void ip4_fib_forwarding_lookup_x2(u32 fib_index0, u32 fib_index1, const ip4_address_t *addr0, const ip4_address_t *addr1, index_t *lb0, index_t *lb1)
#define CLIB_CACHE_LINE_BYTES
struct _vlib_node_registration vlib_node_registration_t
void vlib_cli_output(vlib_main_t *vm, char *fmt,...)
vnet_sw_interface_t * sw_interfaces
#define ip4_local_csum_is_valid(_b)
ip46_address_t fp_addr
The address type is not deriveable from the fp_addr member.
static uword vnet_sw_interface_is_admin_up(vnet_main_t *vnm, u32 sw_if_index)
VNET_FEATURE_ARC_INIT(ip4_unicast, static)
VNET_SW_INTERFACE_ADMIN_UP_DOWN_FUNCTION(ip4_sw_interface_admin_up_down)
static_always_inline void icmp4_error_set_vnet_buffer(vlib_buffer_t *b, u8 type, u8 code, u32 data)
static void ip4_del_interface_routes(u32 sw_if_index, ip4_main_t *im, u32 fib_index, ip4_address_t *address, u32 address_length)
#define vec_free(V)
Free vector's memory (no header).
clib_error_t * vnet_sw_interface_supports_addressing(vnet_main_t *vnm, u32 sw_if_index)
vlib_node_registration_t ip4_mcast_midchain_node
(constructor) VLIB_REGISTER_NODE (ip4_mcast_midchain_node)
vlib_node_registration_t ip4_rewrite_node
(constructor) VLIB_REGISTER_NODE (ip4_rewrite_node)
ip4_enable_disable_interface_function_t * function
format_function_t format_vlib_node_name
static void ip4_add_interface_routes(u32 sw_if_index, ip4_main_t *im, u32 fib_index, ip_interface_address_t *a)
@ FIB_ENTRY_FLAG_CONNECTED
format_function_t format_vnet_sw_if_index_name
unformat_function_t unformat_vnet_sw_interface
description fragment has unexpected format
vlib_combined_counter_main_t adjacency_counters
Adjacency packet counters.
A collection of combined counters.
@ FIB_SOURCE_DEFAULT_ROUTE
The default route source.
#define vec_validate_init_empty(V, I, INIT)
Make sure vector is long enough for given index and initialize empty space (no header,...
static ip_csum_t ip_csum_with_carry(ip_csum_t sum, ip_csum_t x)
u32 fib_table_get_index_for_sw_if_index(fib_protocol_t proto, u32 sw_if_index)
Get the index of the FIB bound to the interface.
@ FIB_ROUTE_PATH_FLAG_NONE
static clib_error_t * fib_module_init(vlib_main_t *vm)
void ip4_register_protocol(u32 protocol, u32 node_index)
#define VLIB_INIT_FUNCTION(x)
static vnet_hw_interface_t * vnet_get_sup_hw_interface(vnet_main_t *vnm, u32 sw_if_index)
ip4_address_t * ip4_interface_first_address(ip4_main_t *im, u32 sw_if_index, ip_interface_address_t **result_ia)
static uword ip4_local_inline(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame, int head_of_feature_arc)
vlib_node_registration_t ip4_local_node
(constructor) VLIB_REGISTER_NODE (ip4_local_node)
vl_api_ip_proto_t protocol
static_always_inline void clib_prefetch_load(void *p)
static void ip4_local_check_src_x2(vlib_buffer_t **b, ip4_header_t **ip, ip4_local_last_check_t *last_check, u8 *error)
u32 ia_cfg_index
feature [arc] config index
#define vec_foreach(var, vec)
Vector iterator.
static load_balance_t * load_balance_get(index_t lbi)
static uword ip4_rewrite_inline(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame, int do_counters, int is_midchain, int is_mcast)
char * ip4_error_strings[]
struct _vnet_classify_main vnet_classify_main_t
@ IP_LOCAL_NEXT_UDP_LOOKUP
static vlib_node_runtime_t * vlib_node_get_runtime(vlib_main_t *vm, u32 node_index)
Get node runtime by node index.
fib_protocol_t fp_proto
protocol type
static u32 ip4_compute_flow_hash(const ip4_header_t *ip, flow_hash_config_t flow_hash_config)
vlib_node_registration_t ip4_lookup_node
(constructor) VLIB_REGISTER_NODE (ip4_lookup_node)
#define ip4_local_need_csum_check(is_tcp_udp, _b)
int vnet_feature_enable_disable(const char *arc_name, const char *node_name, u32 sw_if_index, int enable_disable, void *feature_config, u32 n_feature_config_bytes)
ip_interface_address_t * if_address_pool
Pool of addresses that are assigned to interfaces.
clib_error_t * ip4_add_del_interface_address(vlib_main_t *vm, u32 sw_if_index, ip4_address_t *address, u32 address_length, u32 is_del)
#define vnet_rewrite_two_headers(rw0, rw1, p0, p1, most_likely_size)
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
clib_error_t * ip_interface_address_del(ip_lookup_main_t *lm, vnet_main_t *vnm, u32 address_index, void *addr_fib, u32 address_length, u32 sw_if_index)
u8 local_next_by_ip_protocol[256]
Table mapping ip protocol to ip[46]-local node next index.
void * vlib_add_trace(vlib_main_t *vm, vlib_node_runtime_t *r, vlib_buffer_t *b, u32 n_data_bytes)
static vlib_main_t * vlib_get_main(void)
#define VNET_FEATURES(...)
static void * vlib_buffer_get_current(vlib_buffer_t *b)
Get pointer to current data to process.
static void ip4_del_interface_prefix_routes(ip4_main_t *im, u32 sw_if_index, u32 fib_index, ip4_address_t *address, u32 address_length)
u32 ip_interface_address_find(ip_lookup_main_t *lm, void *addr_fib, u32 address_length)
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
void ip4_forward_next_trace(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame, vlib_rx_or_tx_t which_adj_index)
static void vnet_ip_mcast_fixup_header(u32 dst_mcast_mask, u32 dst_mcast_offset, u32 *addr, u8 *packet0)
@ IP_LOCAL_PACKET_TYPE_L4
vlib_node_registration_t ip4_input_node
Global ip4 input node.
#define vnet_rewrite_one_header(rw0, p0, most_likely_size)
static uword ip4_source_and_port_range_check_rx(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame)
void ip4_unregister_protocol(u32 protocol)
static_always_inline void vnet_feature_arc_start(u8 arc, u32 sw_if_index, u32 *next0, vlib_buffer_t *b0)
#define clib_warning(format, args...)
The identity of a DPO is a combination of its type and its instance number/index of objects of that t...
u16 nexts[VLIB_FRAME_SIZE]
static int ip4_header_bytes(const ip4_header_t *i)
static void ip4_local_check_src(vlib_buffer_t *b, ip4_header_t *ip0, ip4_local_last_check_t *last_check, u8 *error0)
u16 lb_n_buckets_minus_1
number of buckets in the load-balance - 1.
void vlib_packet_template_init(vlib_main_t *vm, vlib_packet_template_t *t, void *packet_data, uword n_packet_data_bytes, uword min_n_buffers_each_alloc, char *fmt,...)
static index_t ip4_fib_forwarding_lookup(u32 fib_index, const ip4_address_t *addr)
static_always_inline void clib_prefetch_store(void *p)
void dpo_set(dpo_id_t *dpo, dpo_type_t type, dpo_proto_t proto, index_t index)
Set/create a DPO ID The DPO will be locked.
index_t lb_urpf
This is the index of the uRPF list for this LB.
static uword ip4_source_and_port_range_check_tx(vlib_main_t *vm, vlib_node_runtime_t *node, vlib_frame_t *frame)
fib_node_index_t fib_table_entry_special_add(u32 fib_index, const fib_prefix_t *prefix, fib_source_t source, fib_entry_flag_t flags)
Add a 'special' entry to the FIB.
static void ip4_add_interface_prefix_routes(ip4_main_t *im, u32 sw_if_index, u32 fib_index, ip_interface_address_t *a)
static_always_inline void adj_midchain_fixup(vlib_main_t *vm, const ip_adjacency_t *adj, vlib_buffer_t *b, vnet_link_t lt)
static clib_error_t * set_ip_classify_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
vl_api_interface_index_t sw_if_index
static void ip4_local_set_next_and_error(vlib_node_runtime_t *error_node, vlib_buffer_t *b, u16 *next, u8 error, u8 head_of_feature_arc)
u8 packet_data[64 - 1 *sizeof(u32)]
ip_interface_address_flags_t flags
vlib_node_registration_t ip4_load_balance_node
(constructor) VLIB_REGISTER_NODE (ip4_load_balance_node)
static u32 ip4_lookup(gid_ip4_table_t *db, u32 vni, ip_prefix_t *key)
void dpo_reset(dpo_id_t *dpo)
reset a DPO ID The DPO will be unlocked.
static ip_adjacency_t * adj_get(adj_index_t adj_index)
Get a pointer to an adjacency object from its index.
__clib_export uword mhash_unset(mhash_t *h, void *key, uword *old_value)
#define IP4_MCAST_ADDR_MASK
This bits of an IPv4 address to mask to construct a multicast MAC address.
Aggregate type for a prefix.
u8 output_feature_arc_index
vnet_interface_main_t interface_main
vlib_increment_combined_counter(ccm, ti, sw_if_index, n_buffers, n_bytes)
#define vlib_prefetch_buffer_data(b, type)
@ IP_FRAG_NEXT_IP_REWRITE_MIDCHAIN
static clib_error_t * set_ip_flow_hash_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
static void * ip4_next_header(ip4_header_t *i)
static uword mhash_set(mhash_t *h, void *key, uword new_value, uword *old_value)
u32 flags
buffer flags: VLIB_BUFFER_FREE_LIST_INDEX_MASK: bits used to store free list index,...
vlib_node_registration_t ip4_midchain_node
(constructor) VLIB_REGISTER_NODE (ip4_midchain_node)
VLIB buffer representation.
static void ip4_local_check_l4_csum_x2(vlib_main_t *vm, vlib_buffer_t **b, ip4_header_t **ih, u8 *error)
#define VLIB_REGISTER_NODE(x,...)
vnet_feature_arc_registration_t vnet_feat_arc_ip4_local
vl_api_wireguard_peer_flags_t flags