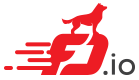 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
18 #ifndef included_vnet_l2_input_h
19 #define included_vnet_l2_input_h
44 u8 __force_u64_alignement[0] __attribute__ ((aligned (8)));
87 u32 feat_next_node_index[32];
127 #define L2INPUT_BVI ((u32) (~0-1))
132 #define foreach_l2input_feat \
133 _(DROP, "feature-bitmap-drop") \
134 _(XCONNECT, "l2-output") \
135 _(FLOOD, "l2-flood") \
136 _(ARP_UFWD, "l2-uu-fwd") \
137 _(ARP_TERM, "arp-term-l2bd") \
138 _(UU_FLOOD, "l2-flood") \
139 _(GBP_FWD, "gbp-fwd") \
140 _(UU_FWD, "l2-uu-fwd") \
143 _(LEARN, "l2-learn") \
144 _(L2_EMULATION, "l2-emulation") \
145 _(GBP_LEARN, "gbp-learn-l2") \
146 _(GBP_LPM_ANON_CLASSIFY, "l2-gbp-lpm-anon-classify") \
147 _(GBP_NULL_CLASSIFY, "gbp-null-classify") \
148 _(GBP_SRC_CLASSIFY, "gbp-src-classify") \
149 _(GBP_LPM_CLASSIFY, "l2-gbp-lpm-classify") \
150 _(VTR, "l2-input-vtr") \
151 _(L2_IP_QOS_RECORD, "l2-ip-qos-record") \
152 _(VPATH, "vpath-input-l2") \
153 _(ACL, "l2-input-acl") \
154 _(POLICER_CLAS, "l2-policer-classify") \
155 _(INPUT_FEAT_ARC, "l2-input-feat-arc") \
156 _(INPUT_CLASSIFY, "l2-input-classify") \
157 _(SPAN, "span-l2-input")
162 #define _(sym,str) L2INPUT_FEAT_##sym##_BIT,
174 #define _(sym,str) L2INPUT_FEAT_##sym = (1<<L2INPUT_FEAT_##sym##_BIT),
178 #define _(sym,str) L2INPUT_FEAT_##sym |
205 L2INPUT_FEAT_UU_FLOOD);
211 return ((bd_config->
feature_bitmap & L2INPUT_FEAT_FWD) == L2INPUT_FEAT_FWD);
225 L2INPUT_FEAT_ARP_TERM);
232 L2INPUT_FEAT_ARP_UFWD);
278 const u8 * old_address,
279 const u8 * new_address);
285 #define MODE_L2_BRIDGE 1
287 #define MODE_L2_CLASSIFY 3
289 #define MODE_ERROR_ETH 1
290 #define MODE_ERROR_BVI_DEF 2
316 ethertype = clib_net_to_host_u16 (eth->
type);
322 vlan = (
void *) (eth + 1);
323 ethertype = clib_net_to_host_u16 (vlan->
type);
324 if (ethertype == ETHERNET_TYPE_VLAN)
346 u16 ethertype = clib_net_to_host_u16 (*(
u16 *) (l3h - 2));
348 if (ethertype == ETHERNET_TYPE_IP4)
350 else if (ethertype == ETHERNET_TYPE_IP6)
static_always_inline int ethernet_frame_is_tagged(u16 type)
vl_api_tunnel_mode_t mode
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
#define hash_v3_finalize32(a, b, c)
static u32 ip6_compute_flow_hash(const ip6_header_t *ip, flow_hash_config_t flow_hash_config)
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
#define static_always_inline
#define IP_FLOW_HASH_DEFAULT
Default: 5-tuple + flowlabel without the "reverse" bit.
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
struct _vlib_node_registration vlib_node_registration_t
static u32 bd_is_valid(l2_bridge_domain_t *bd_config)
#define ethernet_buffer_set_vlan_count(b, v)
Sets the number of VLAN headers in the current Ethernet frame in the buffer.
static u32 ip4_compute_flow_hash(const ip4_header_t *ip, flow_hash_config_t flow_hash_config)
static void * vlib_buffer_get_current(vlib_buffer_t *b)
Get pointer to current data to process.
enum l2_bd_port_type_t_ l2_bd_port_type_t
l2_input_config_t * configs
vl_api_interface_index_t sw_if_index
enum walk_rc_t_ walk_rc_t
Walk return code.
l2_bridge_domain_t * bd_configs
#define hash_v3_mix32(a, b, c)
VLIB buffer representation.