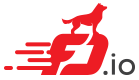 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
20 #include <linux/if_ether.h>
21 #include <linux/if_packet.h>
22 #include <sys/ioctl.h>
26 #include <sys/types.h>
41 #define AF_PACKET_TX_FRAMES_PER_BLOCK 1024
42 #define AF_PACKET_TX_FRAME_SIZE (2048 * 5)
43 #define AF_PACKET_TX_BLOCK_NR 1
44 #define AF_PACKET_TX_FRAME_NR (AF_PACKET_TX_BLOCK_NR * \
45 AF_PACKET_TX_FRAMES_PER_BLOCK)
46 #define AF_PACKET_TX_BLOCK_SIZE (AF_PACKET_TX_FRAME_SIZE * \
47 AF_PACKET_TX_FRAMES_PER_BLOCK)
49 #define AF_PACKET_RX_FRAMES_PER_BLOCK 1024
50 #define AF_PACKET_RX_FRAME_SIZE (2048 * 5)
51 #define AF_PACKET_RX_BLOCK_NR 1
52 #define AF_PACKET_RX_FRAME_NR (AF_PACKET_RX_BLOCK_NR * \
53 AF_PACKET_RX_FRAMES_PER_BLOCK)
54 #define AF_PACKET_RX_BLOCK_SIZE (AF_PACKET_RX_FRAME_SIZE * \
55 AF_PACKET_RX_FRAMES_PER_BLOCK)
81 return VNET_API_ERROR_SYSCALL_ERROR_1;
101 return VNET_API_ERROR_SYSCALL_ERROR_1;
128 s =
format (0,
"/sys/class/net/%s/bridge%c", host_if_name, 0);
129 dir = opendir ((
char *) s);
147 struct sockaddr_ll sll;
148 int ver = TPACKET_V2;
149 socklen_t req_sz =
sizeof (
struct tpacket_req);
150 u32 ring_sz = rx_req->tp_block_size * rx_req->tp_block_nr +
151 tx_req->tp_block_size * tx_req->tp_block_nr;
153 if ((*fd = socket (AF_PACKET, SOCK_RAW, htons (ETH_P_ALL))) < 0)
156 "Failed to create AF_PACKET socket: %s (errno %d)",
157 strerror (errno), errno);
158 ret = VNET_API_ERROR_SYSCALL_ERROR_1;
164 sll.sll_family = PF_PACKET;
165 sll.sll_protocol = htons (ETH_P_ALL);
166 sll.sll_ifindex = host_if_index;
167 if (bind (*fd, (
struct sockaddr *) &sll,
sizeof (sll)) < 0)
170 "Failed to bind rx packet socket: %s (errno %d)",
171 strerror (errno), errno);
172 ret = VNET_API_ERROR_SYSCALL_ERROR_1;
176 if (setsockopt (*fd, SOL_PACKET, PACKET_VERSION, &ver,
sizeof (ver)) < 0)
179 "Failed to set rx packet interface version: %s (errno %d)",
180 strerror (errno), errno);
181 ret = VNET_API_ERROR_SYSCALL_ERROR_1;
186 if (setsockopt (*fd, SOL_PACKET, PACKET_LOSS, &opt,
sizeof (opt)) < 0)
189 "Failed to set packet tx ring error handling option: %s (errno %d)",
190 strerror (errno), errno);
191 ret = VNET_API_ERROR_SYSCALL_ERROR_1;
195 if (setsockopt (*fd, SOL_PACKET, PACKET_QDISC_BYPASS, &opt,
sizeof (opt)) <
199 "Failed to set qdisc bypass error "
200 "handling option: %s (errno %d)",
201 strerror (errno), errno);
204 if (setsockopt (*fd, SOL_PACKET, PACKET_RX_RING, rx_req, req_sz) < 0)
207 "Failed to set packet rx ring options: %s (errno %d)",
208 strerror (errno), errno);
209 ret = VNET_API_ERROR_SYSCALL_ERROR_1;
213 if (setsockopt (*fd, SOL_PACKET, PACKET_TX_RING, tx_req, req_sz) < 0)
216 "Failed to set packet tx ring options: %s (errno %d)",
217 strerror (errno), errno);
218 ret = VNET_API_ERROR_SYSCALL_ERROR_1;
223 mmap (NULL, ring_sz, PROT_READ | PROT_WRITE, MAP_SHARED | MAP_LOCKED, *fd,
225 if (*ring == MAP_FAILED)
228 strerror (errno), errno);
229 ret = VNET_API_ERROR_SYSCALL_ERROR_1;
248 int ret, fd = -1, fd2 = -1;
249 struct tpacket_req *rx_req = 0;
250 struct tpacket_req *tx_req = 0;
262 u8 *host_if_name_dup = 0;
263 int host_if_index = -1;
270 return VNET_API_ERROR_IF_ALREADY_EXISTS;
273 host_if_name_dup =
vec_dup (host_if_name);
291 if ((fd2 = socket (AF_UNIX, SOCK_DGRAM, 0)) < 0)
294 "Failed to create AF_UNIX socket: %s (errno %d)",
295 strerror (errno), errno);
296 ret = VNET_API_ERROR_SYSCALL_ERROR_1;
300 clib_memcpy (ifr.ifr_name, (
const char *) host_if_name,
302 if (ioctl (fd2, SIOCGIFINDEX, &ifr) < 0)
305 "Failed to retrieve the interface (%s) index: %s (errno %d)",
306 host_if_name, strerror (errno), errno);
307 ret = VNET_API_ERROR_INVALID_INTERFACE;
311 host_if_index = ifr.ifr_ifindex;
312 if (ioctl (fd2, SIOCGIFFLAGS, &ifr) < 0)
315 "Failed to get the active flag: %s (errno %d)",
316 strerror (errno), errno);
317 ret = VNET_API_ERROR_SYSCALL_ERROR_1;
321 if (!(ifr.ifr_flags & IFF_UP))
323 ifr.ifr_flags |= IFF_UP;
324 if (ioctl (fd2, SIOCSIFFLAGS, &ifr) < 0)
327 "Failed to set the active flag: %s (errno %d)",
328 strerror (errno), errno);
329 ret = VNET_API_ERROR_SYSCALL_ERROR_1;
357 apif->
tx_ring = ring + rx_req->tp_block_size * rx_req->tp_block_nr;
398 ret = VNET_API_ERROR_SYSCALL_ERROR_1;
420 template.file_descriptor = fd;
421 template.private_data = if_index;
423 template.description =
464 return VNET_API_ERROR_SYSCALL_ERROR_1;
481 ring_sz = apif->
rx_req->tp_block_size * apif->
rx_req->tp_block_nr +
482 apif->
tx_req->tp_block_size * apif->
tx_req->tp_block_nr;
483 if (munmap (apif->
rx_ring, ring_sz))
485 "Host interface %s could not free rx/tx ring",
518 return VNET_API_ERROR_INVALID_INTERFACE;
544 vec_add2 (r_af_packet_ifs, af_packet_if, 1);
555 *out_af_packet_ifs = r_af_packet_ifs;
static void clib_spinlock_init(clib_spinlock_t *p)
#define VNET_HW_IF_RXQ_THREAD_ANY
#define AF_PACKET_TX_FRAME_NR
clib_file_main_t file_main
#define AF_PACKET_RX_BLOCK_NR
struct tpacket_req * tx_req
vlib_node_registration_t af_packet_input_node
(constructor) VLIB_REGISTER_NODE (af_packet_input_node)
vnet_hw_interface_capabilities_t caps
af_packet_main_t af_packet_main
#define clib_memcpy(d, s, n)
int vnet_hw_if_set_rx_queue_mode(vnet_main_t *vnm, u32 queue_index, vnet_hw_if_rx_mode mode)
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
vlib_log_class_t vlib_log_register_class(char *class, char *subclass)
clib_file_function_t * read_function
#define vlib_log_warn(...)
unsigned int if_nametoindex(const char *ifname)
static int is_bridge(const u8 *host_if_name)
#define pool_put(P, E)
Free an object E in pool P.
static uword * mhash_get(mhash_t *h, const void *key)
int af_packet_create_if(vlib_main_t *vm, u8 *host_if_name, u8 *hw_addr_set, u32 *sw_if_index)
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
@ VNET_HW_INTERFACE_FLAG_LINK_UP
#define AF_PACKET_TX_BLOCK_SIZE
vnet_device_class_t af_packet_device_class
#define vlib_log_err(...)
__clib_export u8 * format_clib_error(u8 *s, va_list *va)
static u32 random_u32(u32 *seed)
32-bit random number generator
@ VNET_HW_INTERFACE_CAP_SUPPORTS_TX_TCP_CKSUM
uword * pending_input_bitmap
#define pool_foreach(VAR, POOL)
Iterate through pool.
struct tpacket_req tpacket_req_t
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
#define UNIX_FILE_EVENT_EDGE_TRIGGERED
struct tpacket_req * rx_req
#define vec_add2(V, P, N)
Add N elements to end of vector V, return pointer to new elements in P.
int af_packet_delete_if(vlib_main_t *vm, u8 *host_if_name)
clib_error_t * vnet_netlink_set_link_mtu(int ifindex, int mtu)
#define vec_dup(V)
Return copy of vector (no header, no alignment)
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
static vnet_hw_interface_t * vnet_get_hw_interface(vnet_main_t *vnm, u32 hw_if_index)
vnet_main_t * vnet_get_main(void)
#define AF_PACKET_TX_FRAME_SIZE
#define AF_PACKET_RX_FRAME_NR
#define vec_validate_aligned(V, I, A)
Make sure vector is long enough for given index (no header, specified alignment)
vlib_log_class_t log_class
log class
af_packet_if_t * interfaces
static void mhash_init_vec_string(mhash_t *h, uword n_value_bytes)
void vnet_hw_if_update_runtime_data(vnet_main_t *vnm, u32 hw_if_index)
@ VNET_HW_IF_RX_MODE_INTERRUPT
#define ETHERNET_INTERFACE_FLAG_MTU
#define pool_get(P, E)
Allocate an object E from a pool P (unspecified alignment).
#define vlib_log_debug(...)
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
static_always_inline void vnet_hw_if_rx_queue_set_int_pending(vnet_main_t *vnm, u32 queue_index)
clib_error_t * vnet_netlink_get_link_mtu(int ifindex, u32 *mtu)
static clib_error_t * af_packet_fd_read_ready(clib_file_t *uf)
static uword * clib_bitmap_set(uword *ai, uword i, uword value)
Sets the ith bit of a bitmap to new_value Removes trailing zeros from the bitmap.
int af_packet_set_l4_cksum_offload(vlib_main_t *vm, u32 sw_if_index, u8 set)
#define CLIB_CACHE_LINE_BYTES
static u32 af_packet_eth_flag_change(vnet_main_t *vnm, vnet_hw_interface_t *hi, u32 flags)
void vnet_hw_if_set_rx_queue_file_index(vnet_main_t *vnm, u32 queue_index, u32 file_index)
#define AF_PACKET_TX_BLOCK_NR
format_function_t format_af_packet_device_name
int af_packet_dump_ifs(af_packet_if_detail_t **out_af_packet_ifs)
#define vec_free(V)
Free vector's memory (no header).
description fragment has unexpected format
static void clib_file_del(clib_file_main_t *um, clib_file_t *f)
static vnet_sw_interface_t * vnet_get_hw_sw_interface(vnet_main_t *vnm, u32 hw_if_index)
#define VLIB_INIT_FUNCTION(x)
static vnet_hw_interface_t * vnet_get_sup_hw_interface(vnet_main_t *vnm, u32 sw_if_index)
void ethernet_delete_interface(vnet_main_t *vnm, u32 hw_if_index)
#define AF_PACKET_RX_BLOCK_SIZE
static uword clib_file_add(clib_file_main_t *um, clib_file_t *template)
static int af_packet_read_mtu(af_packet_if_t *apif)
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
u32 vnet_hw_if_register_rx_queue(vnet_main_t *vnm, u32 hw_if_index, u32 queue_id, u32 thread_index)
clib_error_t * vnet_hw_interface_set_flags(vnet_main_t *vnm, u32 hw_if_index, vnet_hw_interface_flags_t flags)
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
@ VNET_HW_INTERFACE_CAP_SUPPORTS_TX_UDP_CKSUM
@ VNET_HW_INTERFACE_CAP_SUPPORTS_INT_MODE
#define clib_error_free(e)
static f64 vlib_time_now(vlib_main_t *vm)
static vlib_thread_main_t * vlib_get_thread_main()
#define AF_PACKET_RX_FRAME_SIZE
vl_api_interface_index_t sw_if_index
__clib_export uword mhash_set_mem(mhash_t *h, void *key, uword *new_value, uword *old_value)
__clib_export uword mhash_unset(mhash_t *h, void *key, uword *old_value)
u32 per_interface_next_index
clib_error_t * ethernet_register_interface(vnet_main_t *vnm, u32 dev_class_index, u32 dev_instance, const u8 *address, u32 *hw_if_index_return, ethernet_flag_change_function_t flag_change)
void vnet_hw_if_set_input_node(vnet_main_t *vnm, u32 hw_if_index, u32 node_index)
static clib_error_t * af_packet_init(vlib_main_t *vm)
mhash_t if_index_by_host_if_name
static int create_packet_v2_sock(int host_if_index, tpacket_req_t *rx_req, tpacket_req_t *tx_req, int *fd, u8 **ring)
vl_api_wireguard_peer_flags_t flags