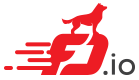 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
40 #ifndef included_vnet_interface_h
41 #define included_vnet_interface_h
51 union ip46_address_t_;
75 const u8 * old_address,
const u8 * new_address);
108 uword * private_data);
115 #define VNET_ITF_FUNC_N_PRIO ((vnet_interface_function_priority_t)VNET_ITF_FUNC_PRIORITY_HIGH+1)
117 typedef struct _vnet_interface_function_list_elt
119 struct _vnet_interface_function_list_elt *next_interface_function;
121 } _vnet_interface_function_list_elt_t;
123 #ifndef CLIB_MARCH_VARIANT
124 #define _VNET_INTERFACE_FUNCTION_DECL_PRIO(f,tag,p) \
126 static void __vnet_interface_function_init_##tag##_##f (void) \
127 __attribute__((__constructor__)) ; \
129 static void __vnet_interface_function_init_##tag##_##f (void) \
131 vnet_main_t * vnm = vnet_get_main(); \
132 static _vnet_interface_function_list_elt_t init_function; \
133 init_function.next_interface_function = vnm->tag##_functions[p]; \
134 vnm->tag##_functions[p] = &init_function; \
135 init_function.fp = (void *) &f; \
137 static void __vnet_interface_function_deinit_##tag##_##f (void) \
138 __attribute__((__destructor__)) ; \
140 static void __vnet_interface_function_deinit_##tag##_##f (void) \
142 vnet_main_t * vnm = vnet_get_main(); \
143 _vnet_interface_function_list_elt_t *next; \
144 if (vnm->tag##_functions[p]->fp == f) \
146 vnm->tag##_functions[p] = \
147 vnm->tag##_functions[p]->next_interface_function; \
150 next = vnm->tag##_functions[p]; \
151 while (next->next_interface_function) \
153 if (next->next_interface_function->fp == f) \
155 next->next_interface_function = \
156 next->next_interface_function->next_interface_function; \
159 next = next->next_interface_function; \
165 #define _VNET_INTERFACE_FUNCTION_DECL_PRIO(f,tag,p) \
166 static __clib_unused void * __clib_unused_##f = f;
169 #define _VNET_INTERFACE_FUNCTION_DECL(f,tag) \
170 _VNET_INTERFACE_FUNCTION_DECL_PRIO(f,tag,VNET_ITF_FUNC_PRIORITY_LOW)
172 #define VNET_HW_INTERFACE_ADD_DEL_FUNCTION(f) \
173 _VNET_INTERFACE_FUNCTION_DECL(f,hw_interface_add_del)
174 #define VNET_HW_INTERFACE_LINK_UP_DOWN_FUNCTION(f) \
175 _VNET_INTERFACE_FUNCTION_DECL(f,hw_interface_link_up_down)
176 #define VNET_HW_INTERFACE_LINK_UP_DOWN_FUNCTION_PRIO(f,p) \
177 _VNET_INTERFACE_FUNCTION_DECL_PRIO(f,hw_interface_link_up_down,p)
178 #define VNET_SW_INTERFACE_MTU_CHANGE_FUNCTION(f) \
179 _VNET_INTERFACE_FUNCTION_DECL(f,sw_interface_mtu_change)
180 #define VNET_SW_INTERFACE_ADD_DEL_FUNCTION(f) \
181 _VNET_INTERFACE_FUNCTION_DECL(f,sw_interface_add_del)
182 #define VNET_SW_INTERFACE_ADD_DEL_FUNCTION_PRIO(f,p) \
183 _VNET_INTERFACE_FUNCTION_DECL_PRIO(f,sw_interface_add_del,p)
184 #define VNET_SW_INTERFACE_ADMIN_UP_DOWN_FUNCTION(f) \
185 _VNET_INTERFACE_FUNCTION_DECL(f,sw_interface_admin_up_down)
186 #define VNET_SW_INTERFACE_ADMIN_UP_DOWN_FUNCTION_PRIO(f,p) \
187 _VNET_INTERFACE_FUNCTION_DECL_PRIO(f,sw_interface_admin_up_down, p)
193 union ip46_address_t_ *
src,
194 union ip46_address_t_ *
dst,
198 typedef struct _vnet_device_class
231 char **tx_function_error_strings;
235 u32 tx_function_n_errors;
239 u32 new_dev_instance);
270 u32 hw_if_index,
u32 new_hw_class_index);
273 void (*rx_redirect_to_node) (
struct vnet_main_t * vnm,
277 struct _vnet_device_class *next_class_registration;
290 #ifndef CLIB_MARCH_VARIANT
291 #define VNET_DEVICE_CLASS(x,...) \
292 __VA_ARGS__ vnet_device_class_t x; \
293 static void __vnet_add_device_class_registration_##x (void) \
294 __attribute__((__constructor__)) ; \
295 static void __vnet_add_device_class_registration_##x (void) \
297 vnet_main_t * vnm = vnet_get_main(); \
298 x.next_class_registration = vnm->device_class_registrations; \
299 vnm->device_class_registrations = &x; \
301 static void __vnet_rm_device_class_registration_##x (void) \
302 __attribute__((__destructor__)) ; \
303 static void __vnet_rm_device_class_registration_##x (void) \
305 vnet_main_t * vnm = vnet_get_main(); \
306 VLIB_REMOVE_FROM_LINKED_LIST (vnm->device_class_registrations, \
307 &x, next_class_registration); \
309 __VA_ARGS__ vnet_device_class_t x
313 #define VNET_DEVICE_CLASS(x,...) \
314 static __clib_unused vnet_device_class_t __clib_unused_##x
317 #define VNET_DEVICE_CLASS_TX_FN(devclass) \
318 uword CLIB_MARCH_SFX (devclass##_tx_fn) (); \
319 static vlib_node_fn_registration_t CLIB_MARCH_SFX ( \
320 devclass##_tx_fn_registration) = { \
321 .function = &CLIB_MARCH_SFX (devclass##_tx_fn), \
324 static void __clib_constructor CLIB_MARCH_SFX ( \
325 devclass##_tx_fn_multiarch_register) (void) \
327 extern vnet_device_class_t devclass; \
328 vlib_node_fn_registration_t *r; \
329 r = &CLIB_MARCH_SFX (devclass##_tx_fn_registration); \
330 r->march_variant = CLIB_MARCH_SFX (CLIB_MARCH_VARIANT_TYPE); \
331 r->next_registration = devclass.tx_fn_registrations; \
332 devclass.tx_fn_registrations = r; \
334 uword CLIB_MARCH_SFX (devclass##_tx_fn)
355 #define VNET_LINKS { \
356 [VNET_LINK_ETHERNET] = "ethernet", \
357 [VNET_LINK_IP4] = "ipv4", \
358 [VNET_LINK_IP6] = "ipv6", \
359 [VNET_LINK_MPLS] = "mpls", \
360 [VNET_LINK_ARP] = "arp", \
361 [VNET_LINK_NSH] = "nsh", \
364 #define FOR_EACH_VNET_LINK(_link) \
365 for (_link = VNET_LINK_IP4; \
366 _link <= VNET_LINK_NSH; \
369 #define FOR_EACH_VNET_IP_LINK(_link) \
370 for (_link = VNET_LINK_IP4; \
371 _link <= VNET_LINK_IP6; \
378 #define VNET_LINK_NUM (VNET_LINK_NSH+1)
379 #define VNET_N_LINKS VNET_LINK_NUM
402 typedef struct _vnet_hw_interface_class
450 vnet_link_t link_type,
const void *dst_hw_address);
454 void (*update_adjacency) (
struct vnet_main_t * vnm,
463 void (*hw_class_change) (
struct vnet_main_t * vnm,
u32 hw_if_index,
464 u32 old_class_index,
u32 new_class_index);
467 struct _vnet_hw_interface_class *next_class_registration;
477 const void *dst_hw_address);
485 #define VNET_HW_INTERFACE_CLASS(x,...) \
486 __VA_ARGS__ vnet_hw_interface_class_t x; \
487 static void __vnet_add_hw_interface_class_registration_##x (void) \
488 __attribute__((__constructor__)) ; \
489 static void __vnet_add_hw_interface_class_registration_##x (void) \
491 vnet_main_t * vnm = vnet_get_main(); \
492 x.next_class_registration = vnm->hw_interface_class_registrations; \
493 vnm->hw_interface_class_registrations = &x; \
495 static void __vnet_rm_hw_interface_class_registration_##x (void) \
496 __attribute__((__destructor__)) ; \
497 static void __vnet_rm_hw_interface_class_registration_##x (void) \
499 vnet_main_t * vnm = vnet_get_main(); \
500 VLIB_REMOVE_FROM_LINKED_LIST (vnm->hw_interface_class_registrations,\
501 &x, next_class_registration); \
503 __VA_ARGS__ vnet_hw_interface_class_t x
555 #define VNET_HW_INTERFACE_CAP_SUPPORTS_L4_TX_CKSUM \
556 (VNET_HW_INTERFACE_CAP_SUPPORTS_TX_TCP_CKSUM | \
557 VNET_HW_INTERFACE_CAP_SUPPORTS_TX_UDP_CKSUM)
559 #define VNET_HW_INTERFACE_CAP_SUPPORTS_TX_CKSUM \
560 (VNET_HW_INTERFACE_CAP_SUPPORTS_TX_IP4_CKSUM | \
561 VNET_HW_INTERFACE_CAP_SUPPORTS_TX_TCP_CKSUM | \
562 VNET_HW_INTERFACE_CAP_SUPPORTS_TX_UDP_CKSUM)
564 #define VNET_HW_INTERFACE_CAP_SUPPORTS_L4_RX_CKSUM \
565 (VNET_HW_INTERFACE_CAP_SUPPORTS_RX_TCP_CKSUM | \
566 VNET_HW_INTERFACE_CAP_SUPPORTS_RX_UDP_CKSUM)
568 #define VNET_HW_INTERFACE_CAP_SUPPORTS_RX_CKSUM \
569 (VNET_HW_INTERFACE_CAP_SUPPORTS_RX_IP4_CKSUM | \
570 VNET_HW_INTERFACE_CAP_SUPPORTS_RX_TCP_CKSUM | \
571 VNET_HW_INTERFACE_CAP_SUPPORTS_RX_UDP_CKSUM)
573 #define VNET_HW_INTERFACE_FLAG_DUPLEX_SHIFT 1
574 #define VNET_HW_INTERFACE_FLAG_SPEED_SHIFT 3
575 #define VNET_HW_INTERFACE_FLAG_DUPLEX_MASK \
576 (VNET_HW_INTERFACE_FLAG_HALF_DUPLEX | \
577 VNET_HW_INTERFACE_FLAG_FULL_DUPLEX)
598 #define VNET_HW_IF_RXQ_THREAD_ANY ~0
599 #define VNET_HW_IF_RXQ_NO_RX_INTERRUPT ~0
714 #define VNET_HW_INTERFACE_BOND_INFO_NONE ((uword *) 0)
715 #define VNET_HW_INTERFACE_BOND_INFO_SLAVE ((uword *) ~0)
849 __VNET_SW_INTERFACE_FLAG_UNSUED = (1 << 2),
853 __VNET_SW_INTERFACE_FLAG_UNUSED2 = (1 << 4),
926 #define foreach_rx_combined_interface_counter(_x) \
927 for (_x = VNET_INTERFACE_COUNTER_RX; \
928 _x <= VNET_INTERFACE_COUNTER_RX_BROADCAST; \
931 #define foreach_tx_combined_interface_counter(_x) \
932 for (_x = VNET_INTERFACE_COUNTER_TX; \
933 _x <= VNET_INTERFACE_COUNTER_TX_BROADCAST; \
936 #define foreach_simple_interface_counter_name \
941 _(RX_NO_BUF, rx-no-buf, if) \
942 _(RX_MISS, rx-miss, if) \
943 _(RX_ERROR, rx-error, if) \
944 _(TX_ERROR, tx-error, if) \
947 #define foreach_combined_interface_counter_name \
949 _(RX_UNICAST, rx-unicast, if) \
950 _(RX_MULTICAST, rx-multicast, if) \
951 _(RX_BROADCAST, rx-broadcast, if) \
953 _(TX_UNICAST, tx-unicast, if) \
954 _(TX_MULTICAST, tx-multicast, if) \
955 _(TX_BROADCAST, tx-broadcast, if)
@ VNET_SW_INTERFACE_FLAG_NONE
@ VNET_N_SIMPLE_INTERFACE_COUNTER
vnet_interface_main_t * im
uword * sub_interface_sw_if_index_by_id
vnet_hw_if_output_node_runtime_t * output_node_thread_runtimes
struct _vlib_node_fn_registration vlib_node_fn_registration_t
vlib_node_registration_t vnet_interface_output_arc_end_node
(constructor) VLIB_REGISTER_NODE (vnet_interface_output_arc_end_node)
@ VNET_HW_INTERFACE_CAP_SUPPORTS_UDP_TNL_GSO
@ VNET_SW_INTERFACE_FLAG_ERROR
uword * hw_interface_class_by_name
@ VNET_SW_INTERFACE_TYPE_PIPE
enum vnet_interface_function_priority_t_ vnet_interface_function_priority_t
vnet_sw_interface_type_t type
void vnet_register_format_buffer_opaque_helper(vnet_buffer_opquae_formatter_t fn)
enum vnet_hw_interface_class_flags_t_ vnet_hw_interface_class_flags_t
Attributes assignable to a HW interface Class.
u32 if_out_arc_end_node_next_index
@ VNET_HW_IF_RX_MODE_ADAPTIVE
struct _vnet_device_class vnet_device_class_t
vnet_hw_interface_capabilities_t caps
int(* vnet_dev_class_ip_tunnel_desc_t)(u32 sw_if_index, union ip46_address_t_ *src, union ip46_address_t_ *dst, u8 *is_l2)
Tunnel description parameters.
void vnet_register_format_buffer_opaque2_helper(vnet_buffer_opquae_formatter_t fn)
@ VNET_INTERFACE_COUNTER_IP6
@ VNET_INTERFACE_COUNTER_TX
@ VNET_HW_INTERFACE_CAP_SUPPORTS_MAC_FILTER
enum vnet_hw_interface_flags_t_ vnet_hw_interface_flags_t
int collect_detailed_interface_stats_flag
@ VNET_INTERFACE_COUNTER_MPLS
@ VNET_INTERFACE_COUNTER_RX_MULTICAST
vnet_hw_if_tx_frame_hint_t
clib_error_t *() vnet_interface_set_mac_address_function_t(struct vnet_hw_interface_t *hi, const u8 *old_address, const u8 *new_address)
#define CLIB_CACHE_LINE_ALIGN_MARK(mark)
enum vnet_sw_interface_flags_t_ vnet_sw_interface_flags_t
static clib_error_t * admin_up_down_function(vnet_main_t *vm, u32 hw_if_index, u32 flags)
vnet_hw_interface_flags_t flags
vnet_p2p_sub_interface_t p2p
@ VNET_SW_INTERFACE_TYPE_SUB
vnet_hw_interface_flags_t_
uword * device_class_by_name
u8 * default_build_rewrite(struct vnet_main_t *vnm, u32 sw_if_index, vnet_link_t link_type, const void *dst_hw_address)
Return a complete, zero-length (aka placeholder) rewrite.
@ VNET_FLOOD_CLASS_NORMAL
vnet_buffer_opquae_formatter_t * buffer_opaque_format_helpers
@ VNET_ITF_FUNC_PRIORITY_HIGH
@ VNET_HW_INTERFACE_CAP_SUPPORTS_RX_IP4_OUTER_CKSUM
vl_api_tunnel_mode_t mode
vnet_interface_stats_collection_mode_e
@ VNET_SW_INTERFACE_FLAG_ADMIN_UP
@ VNET_HW_IF_RX_MODE_POLLING
clib_error_t *() vnet_interface_rss_queues_set_t(struct vnet_main_t *vnm, struct vnet_hw_interface_t *hi, clib_bitmap_t *bitmap)
vnet_sw_interface_flags_t_
@ VNET_HW_INTERFACE_FLAG_LINK_UP
@ VNET_HW_INTERFACE_CAP_SUPPORTS_RX_UDP_CKSUM
vlib_simple_counter_main_t * sw_if_counters
@ VNET_HW_IF_NUM_RX_MODES
vnet_hw_interface_t * hw_interfaces
@ VNET_SW_INTERFACE_FLAG_DIRECTED_BCAST
@ VNET_HW_INTERFACE_CAP_SUPPORTS_TCP_LRO
vnet_mtu_t vnet_link_to_mtu(vnet_link_t link)
vnet_interface_function_priority_t_
uword() vlib_node_function_t(struct vlib_main_t *vm, struct vlib_node_runtime_t *node, struct vlib_frame_t *frame)
vnet_hw_if_rx_queue_t * hw_if_rx_queues
@ VNET_HW_INTERFACE_FLAG_NONE
@ VNET_FLOW_DEV_OP_GET_COUNTER
f64 max_rate_bits_per_sec
@ VNET_HW_INTERFACE_CAP_SUPPORTS_IP_TNL_GSO
vnet_buffer_opquae_formatter_t * buffer_opaque2_format_helpers
vnet_hw_interface_class_flags_t_
Attributes assignable to a HW interface Class.
@ VNET_HW_INTERFACE_CAP_SUPPORTS_TX_UDP_OUTER_CKSUM
vnet_interface_counter_type_t
clib_spinlock_t sw_if_counter_lock
@ VNET_INTERFACE_COUNTER_TX_BROADCAST
vnet_sw_interface_flags_t flags
clib_error_t *() vnet_interface_add_del_mac_address_function_t(struct vnet_hw_interface_t *hi, const u8 *address, u8 is_add)
@ VNET_FLOOD_CLASS_TUNNEL_NORMAL
@ VNET_HW_INTERFACE_CAP_SUPPORTS_TX_TCP_CKSUM
@ VNET_HW_INTERFACE_FLAG_NBMA
static int collect_detailed_interface_stats(void)
@ VNET_INTERFACE_COUNTER_RX_MISS
vnet_l3_packet_type_t vnet_link_to_l3_proto(vnet_link_t link)
Convert a link to to an Ethertype.
vnet_hw_if_rxq_poll_vector_t * rxq_vector_int
@ VNET_HW_INTERFACE_FLAG_FULL_DUPLEX
@ VNET_INTERFACE_COUNTER_RX_BROADCAST
static void clear_counters(perfmon_main_t *pm)
void default_update_adjacency(struct vnet_main_t *vnm, u32 sw_if_index, u32 adj_index)
Default adjacency update function.
@ VNET_HW_IF_TX_FRAME_HINT_NO_CKSUM_OFFLOAD
static clib_error_t * link_up_down_function(vnet_main_t *vm, u32 hw_if_index, u32 flags)
@ VNET_HW_INTERFACE_CAP_SUPPORTS_IPIP_TNL_GSO
@ VNET_HW_INTERFACE_CAP_SUPPORTS_TCP_GSO
u32 * hw_if_index_by_sw_if_index
@ VNET_HW_INTERFACE_CAP_SUPPORTS_UDP_GSO
clib_error_t *() vnet_interface_set_l2_mode_function_t(struct vnet_main_t *vnm, struct vnet_hw_interface_t *hi, i32 l2_if_adjust)
@ VNET_HW_INTERFACE_CAP_NONE
static_always_inline void clib_spinlock_lock(clib_spinlock_t *p)
void vnet_pcap_drop_trace_filter_add_del(u32 error_index, int is_add)
static format_function_t format_flow
vnet_flood_class_t flood_class
@ VNET_HW_IF_TX_FRAME_HINT_NOT_CHAINED
@ VNET_HW_INTERFACE_CAP_SUPPORTS_VXLAN_TNL_GSO
@ VNET_HW_INTERFACE_CAP_SUPPORTS_GENEVE_TNL_GSO
int vnet_pcap_dispatch_trace_configure(vnet_pcap_dispatch_trace_args_t *)
clib_error_t *() vnet_interface_set_rx_mode_function_t(struct vnet_main_t *vnm, u32 if_index, u32 queue_id, vnet_hw_if_rx_mode mode)
@ VNET_HW_INTERFACE_CAP_SUPPORTS_RX_UDP_OUTER_CKSUM
vnet_hw_if_rx_mode default_rx_mode
vnet_device_class_t vnet_local_interface_device_class
struct vnet_hw_interface_t vnet_hw_interface_t
vnet_hw_interface_class_t * hw_interface_classes
vlib_node_registration_t vnet_interface_output_node
(constructor) VLIB_REGISTER_NODE (vnet_interface_output_node)
@ VNET_HW_IF_RX_MODE_INTERRUPT
PCAP utility definitions.
enum vnet_hw_interface_capabilities_t_ vnet_hw_interface_capabilities_t
@ VNET_ITF_FUNC_PRIORITY_LOW
manual_print typedef address
@ VNET_SW_INTERFACE_FLAG_UNNUMBERED
@ VNET_FLOW_DEV_OP_DEL_FLOW
vnet_device_class_t * device_classes
@ VNET_HW_IF_RX_MODE_DEFAULT
@ VNET_INTERFACE_COUNTER_RX
static u8 * format_device(u8 *s, va_list *args)
#define CLIB_CACHE_LINE_BYTES
STATIC_ASSERT_OFFSET_OF(vnet_hw_interface_t, cacheline1, CLIB_CACHE_LINE_BYTES)
u32 max_supported_packet_bytes
struct _vlib_node_registration vlib_node_registration_t
vlib_combined_counter_main_t * combined_sw_if_counters
void collect_detailed_interface_stats_flag_set(void)
vnet_sw_interface_t * sw_interfaces
@ VNET_HW_INTERFACE_CAP_SUPPORTS_GRE_TNL_GSO
vnet_hw_interface_capabilities_t_
vnet_hw_if_tx_frame_hint_t hints
@ VNET_FLOW_DEV_OP_ADD_FLOW
u32 output_node_next_index
uword * txq_index_by_hw_if_index_and_queue_id
u32 unnumbered_sw_if_index
u16 * if_out_arc_end_next_index_by_sw_if_index
@ VNET_INTERFACE_COUNTER_PUNT
@ VNET_HW_IF_TX_FRAME_HINT_NO_GSO
vnet_hw_if_tx_frame_t frame
static void vnet_interface_counter_unlock(vnet_interface_main_t *im)
int() vnet_flow_dev_ops_function_t(struct vnet_main_t *vnm, vnet_flow_dev_op_t op, u32 hw_if_index, u32 index, uword *private_data)
clib_error_t *() vnet_subif_add_del_function_t(struct vnet_main_t *vnm, u32 if_index, struct vnet_sw_interface_t *template, int is_add)
@ VNET_INTERFACE_COUNTER_TX_ERROR
A collection of combined counters.
@ VNET_FLOOD_CLASS_NO_FLOOD
@ VNET_FLOW_DEV_OP_RESET_COUNTER
@ VNET_INTERFACE_COUNTER_RX_NO_BUF
int vnet_interface_name_renumber(u32 sw_if_index, u32 new_show_dev_instance)
clib_error_t *() vnet_interface_function_t(struct vnet_main_t *vnm, u32 if_index, u32 flags)
u32 min_supported_packet_bytes
CLIB_CACHE_LINE_ALIGN_MARK(cacheline0)
vnet_interface_per_thread_data_t * per_thread_data
u32 trace_classify_table_index
vnet_hw_interface_nodes_t * deleted_hw_interface_nodes
uword * pcap_drop_filter_hash
@ VNET_SW_INTERFACE_FLAG_PUNT
static_always_inline void clib_spinlock_unlock(clib_spinlock_t *p)
@ VNET_INTERFACE_COUNTER_RX_ERROR
vnet_hw_if_tx_queue_t * hw_if_tx_queues
uword * hw_interface_by_name
vnet_hw_if_rxq_poll_vector_t * rxq_vector_poll
@ VNET_HW_INTERFACE_CAP_SUPPORTS_RX_IP4_CKSUM
@ VNET_SW_INTERFACE_FLAG_HIDDEN
enum vnet_link_t_ vnet_link_t
Link Type: A description of the protocol of packets on the link.
A collection of simple counters.
@ VNET_HW_INTERFACE_CAP_SUPPORTS_TX_IP4_CKSUM
uword * sw_if_index_by_sup_and_sub
@ VNET_SW_INTERFACE_TYPE_P2P
@ VNET_HW_INTERFACE_CAP_SUPPORTS_TX_UDP_CKSUM
clib_bitmap_t * rss_queues
@ VNET_FLOOD_CLASS_TUNNEL_MASTER
@ VNET_INTERFACE_COUNTER_TX_MULTICAST
@ VNET_HW_INTERFACE_CLASS_FLAG_P2P
a point 2 point interface
@ VNET_HW_INTERFACE_CAP_SUPPORTS_INT_MODE
@ VNET_N_COMBINED_INTERFACE_COUNTER
u8 *(* vnet_buffer_opquae_formatter_t)(const vlib_buffer_t *b, u8 *s)
uword * rxq_index_by_hw_if_index_and_queue_id
@ VNET_HW_INTERFACE_CLASS_FLAG_NBMA
a non-broadcast multiple access interface
@ VNET_INTERFACE_COUNTER_IP4
@ VNET_HW_INTERFACE_FLAG_HALF_DUPLEX
@ VNET_SW_INTERFACE_TYPE_HARDWARE
vnet_link_t_
Link Type: A description of the protocol of packets on the link.
void collect_detailed_interface_stats_flag_clear(void)
vl_api_interface_index_t sw_if_index
u8 output_feature_arc_index
@ VNET_INTERFACE_COUNTER_TX_UNICAST
static void vnet_interface_counter_lock(vnet_interface_main_t *im)
struct _vnet_hw_interface_class vnet_hw_interface_class_t
@ VNET_INTERFACE_COUNTER_DROP
@ VNET_HW_INTERFACE_CAP_SUPPORTS_TX_IP4_OUTER_CKSUM
@ VNET_HW_IF_RX_MODE_UNKNOWN
@ VNET_HW_INTERFACE_CAP_SUPPORTS_RX_TCP_CKSUM
VLIB buffer representation.
@ VNET_INTERFACE_COUNTER_RX_UNICAST
u32 * input_node_thread_index_by_queue
vl_api_wireguard_peer_flags_t flags