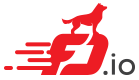 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
26 .class_name =
"interface",
27 .subclass_name =
"runtime",
30 #define log_debug(fmt, ...) vlib_log_debug (if_rxq_log.class, fmt, __VA_ARGS__)
31 #define log_err(fmt, ...) vlib_log_err (if_rxq_log.class, fmt, __VA_ARGS__)
34 [VLIB_NODE_STATE_DISABLED] =
"disabled",
35 [VLIB_NODE_STATE_POLLING] =
"polling",
36 [VLIB_NODE_STATE_INTERRUPT] =
"interrupt",
69 u16 *per_thread_node_adaptive = 0;
70 int something_changed_on_rx = 0;
71 int something_changed_on_tx = 0;
75 log_debug (
"update node '%U' triggered by interface %v",
81 VLIB_NODE_STATE_DISABLED);
100 per_thread_node_state[
ti] = VLIB_NODE_STATE_POLLING;
101 per_thread_node_adaptive[
ti] = 0;
104 if (per_thread_node_state[
ti] == VLIB_NODE_STATE_POLLING)
109 per_thread_node_state[
ti] = VLIB_NODE_STATE_INTERRUPT;
112 per_thread_node_adaptive[
ti] = 1;
130 if (per_thread_node_adaptive[
ti])
137 if (per_thread_node_state[
ti] != VLIB_NODE_STATE_POLLING)
147 for (
int i = 0;
i < n_threads;
i++)
154 if (per_thread_node_state[
i] != old_state)
156 something_changed_on_rx = 1;
157 log_debug (
"state changed for node %U on thread %u from %s to %s",
164 if (something_changed_on_rx == 0)
169 something_changed_on_rx = 1;
170 else if (memcmp (d[
i],
rt->rxq_vector_int,
172 something_changed_on_rx = 1;
174 something_changed_on_rx = 1;
176 if (something_changed_on_rx == 0 && per_thread_node_adaptive[
i])
179 something_changed_on_rx = 1;
180 else if (memcmp (
a[
i],
rt->rxq_vector_poll,
182 something_changed_on_rx = 1;
192 if (
vec_len (
hi->output_node_thread_runtimes) !=
vec_len (new_out_runtimes))
193 something_changed_on_tx = 1;
195 for (
int i = 0;
i <
vec_len (
hi->tx_queue_indices);
i++)
198 u32 queue_index =
hi->tx_queue_indices[
i];
207 (
rt->n_threads != n_threads))
209 log_debug (
"tx queue data changed for interface %v, thread %u "
210 "(queue_id %u -> %u, n_threads %u -> %u)",
213 something_changed_on_tx = 1;
216 rt->n_threads = n_threads;
221 if (something_changed_on_rx || something_changed_on_tx)
228 log_debug (
"%s",
"already running under the barrier");
236 if (something_changed_on_rx)
238 for (
int i = 0;
i < n_threads;
i++)
243 pv =
rt->rxq_vector_int;
244 rt->rxq_vector_int = d[
i];
247 if (per_thread_node_adaptive[
i])
249 pv =
rt->rxq_vector_poll;
250 rt->rxq_vector_poll =
a[
i];
254 if (
rt->rxq_interrupts)
256 void *in =
rt->rxq_interrupts;
263 last_int =
clib_max (last_int, int_num);
269 per_thread_node_adaptive[
i]);
277 if (something_changed_on_tx)
280 t =
hi->output_node_thread_runtimes;
281 hi->output_node_thread_runtimes = new_out_runtimes;
282 new_out_runtimes = t;
289 log_debug (
"skipping update of node '%U', no changes detected",
302 for (
int i = 0;
i < n_threads;
i++)
311 vec_free (per_thread_node_adaptive);
vnet_interface_main_t * im
static_always_inline void clib_interrupt_free(void **data)
void vlib_worker_thread_barrier_release(vlib_main_t *vm)
@ VNET_HW_IF_RX_MODE_ADAPTIVE
static void * vlib_node_get_runtime_data(vlib_main_t *vm, u32 node_index)
Get node runtime private data by node index.
static void vlib_node_set_state(vlib_main_t *vm, u32 node_index, vlib_node_state_t new_state)
Set node dispatch state.
static_always_inline int clib_interrupt_get_next(void *in, int last)
static char * node_state_str[]
@ VNET_HW_IF_RX_MODE_POLLING
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
#define VLIB_NODE_FLAG_ADAPTIVE_MODE
vnet_hw_if_rx_queue_t * hw_if_rx_queues
static int poll_data_sort(void *a1, void *a2)
#define pool_foreach(VAR, POOL)
Iterate through pool.
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
static_always_inline void clib_interrupt_clear(void *in, int int_num)
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
static vnet_hw_interface_t * vnet_get_hw_interface(vnet_main_t *vnm, u32 hw_if_index)
__clib_export void clib_interrupt_resize(void **data, uword n_int)
static uword clib_bitmap_count_set_bits(uword *ai)
Return the number of set bits in a bitmap.
#define vlib_worker_thread_barrier_sync(X)
#define vec_validate_aligned(V, I, A)
Make sure vector is long enough for given index (no header, specified alignment)
#define clib_bitmap_free(v)
Free a bitmap.
void vnet_hw_if_update_runtime_data(vnet_main_t *vnm, u32 hw_if_index)
@ VNET_HW_IF_RX_MODE_INTERRUPT
static void vlib_node_set_flag(vlib_main_t *vm, u32 node_index, u16 flag, u8 enable)
static_always_inline vnet_hw_if_tx_queue_t * vnet_hw_if_get_tx_queue(vnet_main_t *vnm, u32 queue_index)
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
u8 vlib_worker_thread_barrier_held(void)
Return true if the wroker thread barrier is held.
static_always_inline void vnet_hw_if_rx_queue_set_int_pending(vnet_main_t *vnm, u32 queue_index)
@ VNET_HW_IF_RX_MODE_DEFAULT
static uword * clib_bitmap_set(uword *ai, uword i, uword value)
Sets the ith bit of a bitmap to new_value Removes trailing zeros from the bitmap.
#define vec_dup_aligned(V, A)
Return copy of vector (no header, alignment specified).
#define CLIB_CACHE_LINE_BYTES
static_always_inline int clib_interrupt_get_n_int(void *d)
VLIB_REGISTER_LOG_CLASS(if_rxq_log, static)
#define vec_add2_aligned(V, P, N, A)
Add N elements to end of vector V, return pointer to new elements in P.
#define vec_free(V)
Free vector's memory (no header).
format_function_t format_vlib_node_name
#define vec_validate_init_empty(V, I, INIT)
Make sure vector is long enough for given index and initialize empty space (no header,...
static vlib_main_t * vlib_get_main_by_index(u32 thread_index)
#define vec_sort_with_function(vec, f)
Sort a vector using the supplied element comparison function.
static u32 vlib_get_n_threads()
static vlib_main_t * vlib_get_main(void)
vnet_interface_output_runtime_t * rt
#define log_debug(fmt,...)
#define clib_bitmap_foreach(i, ai)
Macro to iterate across set bits in a bitmap.
vnet_interface_main_t interface_main
static vlib_node_state_t vlib_node_get_state(vlib_main_t *vm, u32 node_index)
Get node dispatch state.
@ VNET_HW_IF_RX_MODE_UNKNOWN