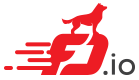 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
34 #include <classify/classify.api_enum.h>
35 #include <classify/classify.api_types.h>
37 #define REPLY_MSG_ID_BASE msg_id_base
42 #define foreach_classify_add_del_table_field \
71 if (n_skip > 5 || n_match == 0 || n_match > 5 ||
75 rv = VNET_API_ERROR_INVALID_VALUE;
86 if (table_chain != ~0)
88 mask_vec, n_skip, n_match);
94 rmp->_vl_msg_id =
ntohs (VL_API_CLASSIFY_PCAP_LOOKUP_TABLE_REPLY);
121 rv = VNET_API_ERROR_INVALID_VALUE;
135 rmp->_vl_msg_id =
ntohs (VL_API_CLASSIFY_PCAP_SET_TABLE_REPLY);
161 rv = VNET_API_ERROR_INVALID_VALUE;
166 if (table_index == ~0)
184 rmp->_vl_msg_id =
ntohs (VL_API_CLASSIFY_PCAP_GET_TABLES_REPLY);
206 u32 table_index = ~0;
216 || n_match == 0 || n_match > 5 || mask_len != n_match *
sizeof (
u32x4))
218 rv = VNET_API_ERROR_INVALID_VALUE;
229 if (table_chain != ~0)
231 mask_vec, n_skip, n_match);
236 rmp->_vl_msg_id =
ntohs ((VL_API_CLASSIFY_TRACE_LOOKUP_TABLE_REPLY));
259 rv = VNET_API_ERROR_INVALID_VALUE;
273 rmp->_vl_msg_id =
ntohs ((VL_API_CLASSIFY_TRACE_SET_TABLE_REPLY));
296 if (table_index == ~0)
314 rmp->_vl_msg_id =
ntohs (VL_API_CLASSIFY_TRACE_GET_TABLES_REPLY);
342 #define _(a) a = ntohl(mp->a);
346 if (mask_len != match_n_vectors *
sizeof (
u32x4))
348 rv = VNET_API_ERROR_INVALID_VALUE;
357 rv = VNET_API_ERROR_NO_SUCH_TABLE;
372 skip_n_vectors, match_n_vectors,
373 next_table_index, miss_next_index, &table_index,
382 t = pool_elt_at_index (cm->tables, table_index);
383 rmp->skip_n_vectors = htonl(t->skip_n_vectors);
384 rmp->match_n_vectors = htonl(t->match_n_vectors);
385 rmp->new_table_index = htonl(table_index);
389 rmp->skip_n_vectors = ~0;
390 rmp->match_n_vectors = ~0;
391 rmp->new_table_index = ~0;
401 vl_api_classify_add_del_session_reply_t *rmp;
403 u32 table_index, hit_next_index, opaque_index, metadata, match_len;
418 rv = VNET_API_ERROR_NO_SUCH_TABLE;
426 rv = VNET_API_ERROR_INVALID_VALUE;
431 (
cm, table_index, mp->
match, hit_next_index, opaque_index,
435 REPLY_MACRO (VL_API_CLASSIFY_ADD_DEL_SESSION_REPLY);
443 vl_api_policer_classify_set_interface_reply_t *rmp;
445 u32 sw_if_index, ip4_table_index, ip6_table_index, l2_table_index;
455 ip6_table_index, l2_table_index,
460 REPLY_MACRO (VL_API_POLICER_CLASSIFY_SET_INTERFACE_REPLY);
472 mp->_vl_msg_id =
ntohs (VL_API_POLICER_CLASSIFY_DETAILS);
487 u32 filter_sw_if_index;
494 if (filter_sw_if_index
498 if (filter_sw_if_index != ~0)
541 rmp->_vl_msg_id =
ntohs (VL_API_CLASSIFY_TABLE_IDS_REPLY);
579 for (if_idx = 0; if_idx <
vec_len (vec_tbl); if_idx++)
626 rmp->_vl_msg_id =
ntohs (VL_API_CLASSIFY_TABLE_INFO_REPLY);
646 rmp->_vl_msg_id =
ntohs (VL_API_CLASSIFY_TABLE_INFO_REPLY);
648 rmp->
retval = ntohl (VNET_API_ERROR_CLASSIFY_TABLE_NOT_FOUND);
664 rmp->_vl_msg_id =
ntohs (VL_API_CLASSIFY_SESSION_DETAILS);
668 rmp->
advance = ntohl (e->advance);
705 for (j = 0; j < (1<<
b->log2_pages); j++)
731 vl_api_flow_classify_set_interface_reply_t *rmp;
742 ip6_table_index, mp->
is_add);
746 REPLY_MACRO (VL_API_FLOW_CLASSIFY_SET_INTERFACE_REPLY);
758 mp->_vl_msg_id =
ntohs (VL_API_FLOW_CLASSIFY_DETAILS);
773 u32 filter_sw_if_index;
780 if (filter_sw_if_index
784 if (filter_sw_if_index != ~0)
807 vl_api_classify_set_interface_ip_table_reply_t *rmp;
822 REPLY_MACRO (VL_API_CLASSIFY_SET_INTERFACE_IP_TABLE_REPLY);
828 vl_api_classify_set_interface_l2_tables_reply_t *rmp;
830 u32 sw_if_index, ip4_table_index, ip6_table_index, other_table_index;
851 if (ip4_table_index != ~0 || ip6_table_index != ~0
852 || other_table_index != ~0)
865 REPLY_MACRO (VL_API_CLASSIFY_SET_INTERFACE_L2_TABLES_REPLY);
872 vl_api_input_acl_set_interface_reply_t *rmp;
883 ip6_table_index, l2_table_index, mp->
is_add);
887 REPLY_MACRO (VL_API_INPUT_ACL_SET_INTERFACE_REPLY);
894 vl_api_output_acl_set_interface_reply_t *rmp;
905 ip6_table_index, l2_table_index,
910 REPLY_MACRO (VL_API_OUTPUT_ACL_SET_INTERFACE_REPLY);
913 #include <classify/classify.api.c>
static void vl_api_classify_table_by_interface_t_handler(vl_api_classify_table_by_interface_t *mp)
Classify get the Trace table indices.
Add a Classify table into a PCAP chain on an interface.
u32 classify_get_pcap_chain(vnet_classify_main_t *cm, u32 sw_if_index)
#define VALIDATE_SW_IF_INDEX(mp)
static vl_api_registration_t * vl_api_client_index_to_registration(u32 index)
u32 skip_n_vectors[default=0]
Classify table ids by interface index request.
static void vl_api_classify_pcap_get_tables_t_handler(vl_api_classify_pcap_get_tables_t *mp)
struct _vnet_classify_entry vnet_classify_entry_t
int vnet_classify_add_del_session(vnet_classify_main_t *cm, u32 table_index, const u8 *match, u32 hit_next_index, u32 opaque_index, i32 advance, u8 action, u16 metadata, int is_add)
Classify get table IDs request.
static int vnet_classify_entry_is_free(vnet_classify_entry_t *e)
i16 current_data_offset[default=0]
#define REPLY_MACRO2(t, body)
Set/unset policer classify interface.
int vnet_set_input_acl_intfc(vlib_main_t *vm, u32 sw_if_index, u32 ip4_table_index, u32 ip6_table_index, u32 l2_table_index, u32 is_add)
#define clib_memcpy(d, s, n)
void vnet_l2_output_classify_enable_disable(u32 sw_if_index, int enable_disable)
Enable/disable l2 input classification on a specific interface.
static void vl_api_send_msg(vl_api_registration_t *rp, u8 *elem)
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
vl_api_interface_index_t sw_if_index
u32 classify_lookup_chain(u32 table_index, u8 *mask, u32 n_skip, u32 n_match)
Reply for classify get table IDs request.
vnet_classify_main_t vnet_classify_main
static void vl_api_classify_trace_get_tables_t_handler(vl_api_classify_trace_get_tables_t *mp)
Add/Delete classification table request.
static vnet_classify_entry_t * vnet_classify_entry_at_index(vnet_classify_table_t *t, vnet_classify_entry_t *e, u32 index)
static vnet_classify_entry_t * vnet_classify_get_entry(vnet_classify_table_t *t, uword offset)
Classify add / del session request.
u32 * classify_table_index_by_sw_if_index[FLOW_CLASSIFY_N_TABLES]
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
u32 match_n_vectors[default=1]
static void vl_api_output_acl_set_interface_t_handler(vl_api_output_acl_set_interface_t *mp)
vl_api_interface_index_t sw_if_index
Flow classify operational state response.
Find a mask-compatible Classify table in the Trace chain.
static void vl_api_classify_trace_lookup_table_t_handler(vl_api_classify_trace_lookup_table_t *mp)
Get list of policer classify interfaces and tables.
vl_api_interface_index_t sw_if_index
Set/unset flow classify interface.
vl_api_interface_index_t sw_if_index
@ IN_OUT_ACL_INPUT_TABLE_GROUP
void * vl_msg_api_alloc_as_if_client(int nbytes)
static void send_policer_classify_details(u32 sw_if_index, u32 table_index, vl_api_registration_t *reg, u32 context)
vl_api_interface_index_t sw_if_index[default=0xffffffff]
bool sort_masks[default=0]
#define pool_is_free_index(P, I)
Use free bitmap to query whether given index is free.
#define vec_elt(v, i)
Get vector value at index i.
static clib_error_t * classify_api_hookup(vlib_main_t *vm)
#define pool_foreach(VAR, POOL)
Iterate through pool.
int vnet_classify_add_del_table(vnet_classify_main_t *cm, const u8 *mask, u32 nbuckets, u32 memory_size, u32 skip, u32 match, u32 next_table_index, u32 miss_next_index, u32 *table_index, u8 current_data_flag, i16 current_data_offset, int is_add, int del_chain)
u32 opaque_index[default=0xffffffff]
u8 current_data_flag[default=0]
static void send_flow_classify_details(u32 sw_if_index, u32 table_index, vl_api_registration_t *reg, u32 context)
u32 * classify_table_index_by_sw_if_index[POLICER_CLASSIFY_N_TABLES]
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
static void vl_api_classify_trace_set_table_t_handler(vl_api_classify_trace_set_table_t *mp)
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
#define foreach_classify_add_del_table_field
static void vl_api_input_acl_set_interface_t_handler(vl_api_input_acl_set_interface_t *mp)
An API client registration, only in vpp/vlib.
static void setup_message_id_table(api_main_t *am)
void classify_set_pcap_chain(vnet_classify_main_t *cm, u32 sw_if_index, u32 table_index)
Get list of flow classify interfaces and tables.
vl_api_classify_action_t action[default=0]
int vnet_set_policer_classify_intfc(vlib_main_t *vm, u32 sw_if_index, u32 ip4_table_index, u32 ip6_table_index, u32 l2_table_index, u32 is_add)
u32 table_index[default=0xffffffff]
static void vl_api_flow_classify_set_interface_t_handler(vl_api_flow_classify_set_interface_t *mp)
Classify pcap table lookup response.
Classify sessions dump request.
vnet_classify_bucket_t * buckets
static void vl_api_classify_pcap_set_table_t_handler(vl_api_classify_pcap_set_table_t *mp)
Policer classify operational state response.
u32 match_n_vectors[default=1]
vnet_feature_config_main_t * cm
vl_api_interface_index_t sw_if_index
static void vl_api_classify_set_interface_ip_table_t_handler(vl_api_classify_set_interface_ip_table_t *mp)
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
policer_classify_main_t policer_classify_main
u32 skip_n_vectors[default=0]
vl_api_policer_classify_table_t type
Classify get the Trace tables response.
vl_api_interface_index_t sw_if_index
Find a compatible Classify table in a PCAP chain.
#define BAD_SW_IF_INDEX_LABEL
static void vl_api_classify_set_interface_l2_tables_t_handler(vl_api_classify_set_interface_l2_tables_t *mp)
Set/unset l2 classification tables for an interface request.
Add/Delete classification table response.
vl_api_interface_index_t sw_if_index
#define vec_free(V)
Free vector's memory (no header).
int vnet_set_output_acl_intfc(vlib_main_t *vm, u32 sw_if_index, u32 ip4_table_index, u32 ip6_table_index, u32 l2_table_index, u32 is_add)
vl_api_interface_index_t sw_if_index
u32 hit_next_index[default=0xffffffff]
Reply for classify table id by interface index request.
Reply for classify table info request.
Classify pcap table lookup response.
int vnet_l2_output_classify_set_tables(u32 sw_if_index, u32 ip4_table_index, u32 ip6_table_index, u32 other_table_index)
Set l2 per-protocol, per-interface output classification tables.
static void vl_api_policer_classify_set_interface_t_handler(vl_api_policer_classify_set_interface_t *mp)
static void vl_api_classify_table_ids_t_handler(vl_api_classify_table_ids_t *mp)
int vnet_set_flow_classify_intfc(vlib_main_t *vm, u32 sw_if_index, u32 ip4_table_index, u32 ip6_table_index, u32 is_add)
void classify_get_trace_chain(void)
struct _vnet_classify_main vnet_classify_main_t
static void vl_api_flow_classify_dump_t_handler(vl_api_flow_classify_dump_t *mp)
static void vl_api_policer_classify_dump_t_handler(vl_api_policer_classify_dump_t *mp)
bool sort_masks[default=0]
vl_api_interface_index_t sw_if_index
static void vl_api_classify_session_dump_t_handler(vl_api_classify_session_dump_t *mp)
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
static vlib_main_t * vlib_get_main(void)
#define vec_set(v, val)
Set all vector elements to given value.
Classify get the PCAP table indices for an interface.
u32 classify_sort_table_chain(vnet_classify_main_t *cm, u32 table_index)
int vnet_l2_input_classify_set_tables(u32 sw_if_index, u32 ip4_table_index, u32 ip6_table_index, u32 other_table_index)
Set l2 per-protocol, per-interface input classification tables.
int vnet_set_ip6_classify_intfc(vlib_main_t *vm, u32 sw_if_index, u32 table_index)
void classify_set_trace_chain(vnet_classify_main_t *cm, u32 table_index)
vl_api_interface_index_t sw_if_index
static void vl_api_classify_pcap_lookup_table_t_handler(vl_api_classify_pcap_lookup_table_t *mp)
static void send_classify_session_details(vl_api_registration_t *reg, u32 table_id, u32 match_length, vnet_classify_entry_t *e, u32 context)
Set/unset the classification table for an interface request.
Reply for classify table session dump request.
Classify get a PCAP tables response.
Classify Trace table lookup response.
in_out_acl_main_t in_out_acl_main
int vnet_set_ip4_classify_intfc(vlib_main_t *vm, u32 sw_if_index, u32 table_index)
vl_api_flow_classify_table_t type
VLIB_API_INIT_FUNCTION(classify_api_hookup)
vl_api_mac_event_action_t action
vl_api_interface_index_t sw_if_index
vl_api_interface_index_t sw_if_index
u32 table_index[default=0xffffffff]
void vnet_l2_input_classify_enable_disable(u32 sw_if_index, int enable_disable)
Enable/disable l2 input classification on a specific interface.
static void vl_api_classify_add_del_table_t_handler(vl_api_classify_add_del_table_t *mp)
Classify trace table lookup response.
flow_classify_main_t flow_classify_main
vl_api_fib_path_type_t type
Set/unset output ACL interface.
static void vl_api_classify_table_info_t_handler(vl_api_classify_table_info_t *mp)
static void vl_api_classify_add_del_session_t_handler(vl_api_classify_add_del_session_t *mp)
Add a Classify table into the Trace chain.
vl_api_interface_index_t sw_if_index
void * vl_msg_api_alloc(int nbytes)
vl_api_interface_index_t sw_if_index