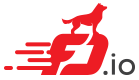 |
FD.io VPP
v21.10.1-2-g0a485f517
Vector Packet Processing
|
Go to the documentation of this file.
34 #if VALIDATION_SCAFFOLDING
51 u32 active_elements = 0;
60 for (j = 0; j < (1 <<
b->log2_pages); j++)
74 clib_warning (
"found %u expected %u elts", active_elements,
102 vec_add1 (
cm->unformat_ip_next_index_fns, fn);
110 vec_add1 (
cm->unformat_acl_next_index_fns, fn);
119 vec_add1 (
cm->unformat_policer_next_index_fns, fn);
138 nbuckets = 1 << (
max_log2 (nbuckets));
165 u32 table_index,
int del_chain)
235 int working_copy_length, required_length;
260 if (required_length > working_copy_length)
275 working_bucket.as_u64 =
b->as_u64;
278 b->as_u64 = working_bucket.as_u64;
288 int i, j, length_in_entries;
293 for (
i = 0;
i < length_in_entries;
i++)
303 key_minus_skip = (
u8 *) v->key;
308 new_hash &= (1 << new_log2_pages) - 1;
336 u32 old_log2_pages,
u32 new_log2_pages)
339 int i, j, new_length_in_entries, old_length_in_entries;
346 for (
i = 0;
i < old_length_in_entries;
i++)
352 for (; j < new_length_in_entries; j++)
358 clib_warning (
"BUG: linear rehash new entry not free!");
428 u32 old_log2_pages, new_log2_pages;
431 int resplit_once = 0;
432 int mark_bucket_linear;
436 key_minus_skip = (
u8 *) add_v->key;
441 bucket_index = hash & (t->
nbuckets - 1);
480 limit *= (1 <<
b->log2_pages);
490 for (
i = 0;
i < limit;
i++)
508 for (
i = 0;
i < limit;
i++)
529 for (
i = 0;
i < limit;
i++)
553 new_log2_pages = old_log2_pages + 1;
559 mark_bucket_linear = 0;
583 mark_bucket_linear = 1;
590 key_minus_skip = (
u8 *) add_v->key;
595 new_hash &= (1 << new_log2_pages) - 1;
598 if (mark_bucket_linear)
600 limit *= (1 << new_log2_pages);
604 for (
i = 0;
i < limit;
i++)
646 }) classify_data_or_mask_t;
669 (s,
"[%u]: next_index %d advance %d opaque %d action %d metadata %d\n",
671 e->opaque_index, e->action, e->metadata);
678 s =
format (s,
" hits %lld, last_heard %.2f\n",
679 e->hits, e->last_heard);
681 s =
format (s,
" entry is free\n");
689 int verbose = va_arg (*args,
int);
693 u64 active_elements = 0;
701 s =
format (s,
"[%d]: empty\n",
i);
707 s =
format (s,
"[%d]: heap offset %d, elts %d, %s\n",
i,
709 b->linear_search ?
"LINEAR" :
"normal");
713 for (j = 0; j < (1 <<
b->log2_pages); j++)
724 s =
format (s,
" %d: empty\n",
730 s =
format (s,
" %d: %U\n",
739 s =
format (s,
" %lld active elements\n", active_elements);
748 u32 match,
u32 next_table_index,
749 u32 miss_next_index,
u32 *table_index,
750 u8 current_data_flag,
i16 current_data_offset,
751 int is_add,
int del_chain)
757 if (*table_index == ~0)
760 return VNET_API_ERROR_INVALID_MEMORY_SIZE;
763 return VNET_API_ERROR_INVALID_VALUE;
765 if (match < 1 || match > 5)
766 return VNET_API_ERROR_INVALID_VALUE;
774 *table_index = t -
cm->tables;
790 #define foreach_tcp_proto_field \
794 #define foreach_udp_proto_field \
798 #define foreach_ip4_proto_field \
811 u8 **maskp = va_arg (*args,
u8 **);
813 u8 found_something = 0;
823 #define _(a) else if (unformat (input, #a)) a=1;
830 #define _(a) found_something += a;
834 if (found_something == 0)
841 #define _(a) if (a) clib_memset (&tcp->a, 0xff, sizeof (tcp->a));
852 u8 **maskp = va_arg (*args,
u8 **);
854 u8 found_something = 0;
864 #define _(a) else if (unformat (input, #a)) a=1;
871 #define _(a) found_something += a;
875 if (found_something == 0)
882 #define _(a) if (a) clib_memset (&udp->a, 0xff, sizeof (udp->a));
898 u8 **maskp = va_arg (*args,
u8 **);
908 else if (
unformat (input,
"src_port"))
910 else if (
unformat (input,
"dst_port"))
934 u8 **maskp = va_arg (*args,
u8 **);
936 u8 found_something = 0;
938 u32 src_prefix_len = 32;
939 u32 src_prefix_mask = ~0;
940 u32 dst_prefix_len = 32;
941 u32 dst_prefix_mask = ~0;
954 else if (
unformat (input,
"hdr_length"))
956 else if (
unformat (input,
"src/%d", &src_prefix_len))
959 src_prefix_mask &= ~((1 << (32 - src_prefix_len)) - 1);
960 src_prefix_mask = clib_host_to_net_u32 (src_prefix_mask);
962 else if (
unformat (input,
"dst/%d", &dst_prefix_len))
965 dst_prefix_mask &= ~((1 << (32 - dst_prefix_len)) - 1);
966 dst_prefix_mask = clib_host_to_net_u32 (dst_prefix_mask);
975 #define _(a) else if (unformat (input, #a)) a=1;
982 found_something =
version + hdr_length;
983 #define _(a) found_something += a;
987 if (found_something == 0)
994 #define _(a) if (a) clib_memset (&ip->a, 0xff, sizeof (ip->a));
999 ip->src_address.as_u32 = src_prefix_mask;
1002 ip->dst_address.as_u32 = dst_prefix_mask;
1004 ip->ip_version_and_header_length = 0;
1007 ip->ip_version_and_header_length |= 0xF0;
1010 ip->ip_version_and_header_length |= 0x0F;
1016 #define foreach_ip6_proto_field \
1026 u8 **maskp = va_arg (*args,
u8 **);
1030 u32 ip_version_traffic_class_and_flow_label;
1032 #define _(a) u8 a=0;
1036 u8 traffic_class = 0;
1043 else if (
unformat (input,
"traffic-class"))
1045 else if (
unformat (input,
"flow-label"))
1051 else if (
unformat (input,
"proto"))
1054 #define _(a) else if (unformat (input, #a)) a=1;
1062 found_something =
version + traffic_class + flow_label
1065 #define _(a) found_something += a;
1069 if (found_something == 0)
1076 #define _(a) if (a) clib_memset (&ip->a, 0xff, sizeof (ip->a));
1080 ip_version_traffic_class_and_flow_label = 0;
1083 ip_version_traffic_class_and_flow_label |= 0xF0000000;
1086 ip_version_traffic_class_and_flow_label |= 0x0FF00000;
1089 ip_version_traffic_class_and_flow_label |= 0x000FFFFF;
1091 ip->ip_version_traffic_class_and_flow_label =
1092 clib_host_to_net_u32 (ip_version_traffic_class_and_flow_label);
1101 u8 **maskp = va_arg (*args,
u8 **);
1118 u8 **maskp = va_arg (*args,
u8 **);
1139 else if (
unformat (input,
"proto"))
1145 else if (
unformat (input,
"ignore-tag1"))
1147 else if (
unformat (input,
"ignore-tag2"))
1153 else if (
unformat (input,
"dot1q"))
1155 else if (
unformat (input,
"dot1ad"))
1160 if ((
src +
dst +
proto + tag1 + tag2 + dot1q + dot1ad +
1161 ignore_tag1 + ignore_tag2 + cos1 + cos2) == 0)
1164 if (tag1 || ignore_tag1 || cos1 || dot1q)
1166 if (tag2 || ignore_tag2 || cos2 || dot1ad)
1227 u8 **maskp = va_arg (*args,
u8 **);
1228 u32 *skipp = va_arg (*args,
u32 *);
1229 u32 *matchp = va_arg (*args,
u32 *);
1259 if (
mask || l2 || l3 || l4)
1285 *skipp =
i /
sizeof (
u32x4);
1294 for (
i = match *
sizeof (
u32x4);
i > 0;
i -=
sizeof (
u32x4))
1304 _vec_len (
mask) = match *
sizeof (
u32x4);
1315 #define foreach_l2_input_next \
1317 _(ethernet, ETHERNET_INPUT) \
1326 u32 *miss_next_indexp = va_arg (*args,
u32 *);
1332 for (
i = 0;
i <
vec_len (
cm->unformat_l2_next_index_fns);
i++)
1334 if (
unformat (input,
"%U",
cm->unformat_l2_next_index_fns[
i], &
tmp))
1342 if (unformat (input, #n)) { next_index = L2_INPUT_CLASSIFY_NEXT_##N; goto out;}
1359 #define foreach_l2_output_next \
1366 u32 *miss_next_indexp = va_arg (*args,
u32 *);
1372 for (
i = 0;
i <
vec_len (
cm->unformat_l2_next_index_fns);
i++)
1374 if (
unformat (input,
"%U",
cm->unformat_l2_next_index_fns[
i], &
tmp))
1382 if (unformat (input, #n)) { next_index = L2_OUTPUT_CLASSIFY_NEXT_##N; goto out;}
1399 #define foreach_ip_next \
1406 u32 *miss_next_indexp = va_arg (*args,
u32 *);
1413 for (
i = 0;
i <
vec_len (
cm->unformat_ip_next_index_fns);
i++)
1415 if (
unformat (input,
"%U",
cm->unformat_ip_next_index_fns[
i], &
tmp))
1423 if (unformat (input, #n)) { next_index = IP_LOOKUP_NEXT_##N; goto out;}
1440 #define foreach_acl_next \
1446 u32 *next_indexp = va_arg (*args,
u32 *);
1453 for (
i = 0;
i <
vec_len (
cm->unformat_acl_next_index_fns);
i++)
1455 if (
unformat (input,
"%U",
cm->unformat_acl_next_index_fns[
i], &
tmp))
1463 if (unformat (input, #n)) { next_index = ACL_NEXT_INDEX_##N; goto out;}
1488 u32 *next_indexp = va_arg (*args,
u32 *);
1495 for (
i = 0;
i <
vec_len (
cm->unformat_policer_next_index_fns);
i++)
1498 (input,
"%U",
cm->unformat_policer_next_index_fns[
i], &
tmp))
1527 u32 table_index = ~0;
1528 u32 next_table_index = ~0;
1529 u32 miss_next_index = ~0;
1532 u32 current_data_flag = 0;
1533 int current_data_offset = 0;
1543 else if (
unformat (input,
"del-chain"))
1548 else if (
unformat (input,
"buckets %d", &nbuckets))
1550 else if (
unformat (input,
"skip %d", &skip))
1552 else if (
unformat (input,
"match %d", &match))
1554 else if (
unformat (input,
"table %d", &table_index))
1557 &
mask, &skip, &match))
1559 else if (
unformat (input,
"memory-size %uM", &
tmp))
1561 else if (
unformat (input,
"memory-size %uG", &
tmp))
1563 else if (
unformat (input,
"next-table %d", &next_table_index))
1581 else if (
unformat (input,
"current-data-flag %d", ¤t_data_flag))
1584 if (
unformat (input,
"current-data-offset %d", ¤t_data_offset))
1591 if (is_add &&
mask == 0 && table_index == ~0)
1594 if (is_add && skip == ~0 && table_index == ~0)
1597 if (is_add && match == ~0 && table_index == ~0)
1600 if (!is_add && table_index == ~0)
1604 skip, match, next_table_index,
1605 miss_next_index, &table_index,
1606 current_data_flag, current_data_offset,
1623 .path =
"classify table",
1625 "classify table [miss-next|l2-miss_next|acl-miss-next <next_index>]"
1626 "\n mask <mask-value> buckets <nn> [skip <n>] [match <n>]"
1627 "\n [current-data-flag <n>] [current-data-offset <n>] [table <n>]"
1628 "\n [memory-size <nn>[M][G]] [next-table <n>]"
1629 "\n [del] [del-chain]",
1712 table_index = tables[0];
1735 if (table_index == ~0)
1737 u32 old_table_index;
1750 u32 table_index = ~0;
1754 table_index =
cm->classify_table_index_by_sw_if_index[
sw_if_index];
1769 if (table_index == ~0)
1771 u32 old_table_index = ~0;
1785 cm->classify_table_index_by_sw_if_index[
sw_if_index] = table_index;
1790 hi->trace_classify_table_index = table_index;
1805 if (table_index == ~0)
1844 u32 table_index = ~0;
1845 u32 next_table_index = ~0;
1846 u32 miss_next_index = ~0;
1847 u32 current_data_flag = 0;
1848 int current_data_offset = 0;
1867 else if (
unformat (line_input,
"pcap %=", &pcap, 1))
1869 else if (
unformat (line_input,
"trace"))
1871 else if (
unformat (line_input,
"%U",
1877 else if (
unformat (line_input,
"buckets %d", &nbuckets))
1880 &
mask, &skip, &match))
1889 if (is_add &&
mask == 0)
1892 else if (is_add && skip == ~0)
1895 else if (is_add && match == ~0)
1898 else if (
sw_if_index == ~0 && pkt_trace == 0 && pcap == 0)
1901 else if (pkt_trace && pcap)
1903 (0,
"Packet trace and pcap are mutually exclusive...");
1938 if (table_index != ~0)
1948 next_table_index = table_index;
1955 if (table_index == ~0)
1964 skip, match, next_table_index,
1965 miss_next_index, &table_index,
1967 current_data_offset, 1, 0);
1974 "vnet_classify_add_del_table returned %d",
1999 cm, &match_vector, table_index) == 0)
2109 .path =
"classify filter",
2111 "classify filter <intfc> | pcap mask <mask-value> match <match-value>\n"
2112 " | trace mask <mask-value> match <match-value> [del]\n"
2113 " [buckets <nn>] [memory-size <n>]",
2131 (void)
unformat (input,
"verbose %=", &verbose, 1);
2136 limit =
vec_len (
cm->classify_table_index_by_sw_if_index);
2138 for (
i = -1;
i < limit;
i++)
2148 table_index =
cm->classify_table_index_by_sw_if_index[
i];
2153 table_index =
cm->classify_table_index_by_sw_if_index[
i];
2168 s =
format (s,
" %u", j);
2180 if (table_index != ~0)
2181 s =
format (s,
" %u", table_index);
2199 .path =
"show classify filter",
2200 .short_help =
"show classify filter [verbose [nn]]",
2209 int verbose = va_arg (*args,
int);
2215 s =
format (s,
"\n%10s%10s%10s%10s",
"TableIdx",
"Sessions",
"NextTbl",
2216 "NextNode", verbose ?
"Details" :
"");
2227 s =
format (s,
"\n nbuckets %d, skip %d match %d flag %d offset %d",
2249 u32 match_index = ~0;
2256 if (
unformat (input,
"index %d", &match_index))
2258 else if (
unformat (input,
"verbose %d", &verbose))
2260 else if (
unformat (input,
"verbose"))
2269 if (match_index == ~0 || (match_index == t -
cm->tables))
2294 .path =
"show classify tables",
2295 .short_help =
"show classify tables [index <nn>]",
2303 u8 **matchp = va_arg (*args,
u8 **);
2305 u8 *proto_header = 0;
2321 h.src_port = clib_host_to_net_u16 (
src_port);
2322 h.dst_port = clib_host_to_net_u16 (
dst_port);
2324 memcpy (proto_header, &
h,
sizeof (
h));
2326 *matchp = proto_header;
2334 u8 **matchp = va_arg (*args,
u8 **);
2349 int fragment_id = 0;
2350 u32 fragment_id_val;
2358 if (
unformat (input,
"version %d", &version_val))
2360 else if (
unformat (input,
"hdr_length %d", &hdr_length_val))
2366 else if (
unformat (input,
"proto %d", &proto_val))
2368 else if (
unformat (input,
"tos %d", &tos_val))
2370 else if (
unformat (input,
"length %d", &length_val))
2372 else if (
unformat (input,
"fragment_id %d", &fragment_id_val))
2374 else if (
unformat (input,
"ttl %d", &ttl_val))
2376 else if (
unformat (input,
"checksum %d", &checksum_val))
2383 +
ttl + checksum == 0)
2395 ip->src_address.as_u32 = src_val.
as_u32;
2398 ip->dst_address.as_u32 = dst_val.
as_u32;
2401 ip->protocol = proto_val;
2406 ip->ip_version_and_header_length |= (version_val & 0xF) << 4;
2409 ip->ip_version_and_header_length |= (hdr_length_val & 0xF);
2415 ip->length = clib_host_to_net_u16 (length_val);
2421 ip->checksum = clib_host_to_net_u16 (checksum_val);
2430 u8 **matchp = va_arg (*args,
u8 **);
2435 u8 traffic_class = 0;
2436 u32 traffic_class_val;
2440 ip6_address_t src_val, dst_val;
2443 int payload_length = 0;
2444 u32 payload_length_val;
2447 u32 ip_version_traffic_class_and_flow_label;
2451 if (
unformat (input,
"version %d", &version_val))
2453 else if (
unformat (input,
"traffic_class %d", &traffic_class_val))
2455 else if (
unformat (input,
"flow_label %d", &flow_label_val))
2461 else if (
unformat (input,
"proto %d", &proto_val))
2463 else if (
unformat (input,
"payload_length %d", &payload_length_val))
2465 else if (
unformat (input,
"hop_limit %d", &hop_limit_val))
2489 ip->protocol = proto_val;
2491 ip_version_traffic_class_and_flow_label = 0;
2494 ip_version_traffic_class_and_flow_label |= (version_val & 0xF) << 28;
2497 ip_version_traffic_class_and_flow_label |=
2498 (traffic_class_val & 0xFF) << 20;
2501 ip_version_traffic_class_and_flow_label |= (flow_label_val & 0xFFFFF);
2503 ip->ip_version_traffic_class_and_flow_label =
2504 clib_host_to_net_u32 (ip_version_traffic_class_and_flow_label);
2507 ip->payload_length = clib_host_to_net_u16 (payload_length_val);
2510 ip->hop_limit = hop_limit_val;
2519 u8 **matchp = va_arg (*args,
u8 **);
2537 u8 *tagp = va_arg (*args,
u8 *);
2542 tagp[0] = (tag >> 8) & 0x0F;
2543 tagp[1] = tag & 0xFF;
2553 u8 **matchp = va_arg (*args,
u8 **);
2580 else if (
unformat (input,
"proto %U",
2587 else if (
unformat (input,
"ignore-tag1"))
2589 else if (
unformat (input,
"ignore-tag2"))
2591 else if (
unformat (input,
"cos1 %d", &cos1_val))
2593 else if (
unformat (input,
"cos2 %d", &cos2_val))
2599 ignore_tag1 + ignore_tag2 + cos1 + cos2) == 0)
2602 if (tag1 || ignore_tag1 || cos1)
2604 if (tag2 || ignore_tag2 || cos2)
2618 match[19] = tag2_val[1];
2619 match[18] = tag2_val[0];
2621 match[18] |= (cos2_val & 0x7) << 5;
2624 match[21] = proto_val & 0xff;
2625 match[20] = proto_val >> 8;
2629 match[15] = tag1_val[1];
2630 match[14] = tag1_val[0];
2633 match[14] |= (cos1_val & 0x7) << 5;
2639 match[15] = tag1_val[1];
2640 match[14] = tag1_val[0];
2643 match[17] = proto_val & 0xff;
2644 match[16] = proto_val >> 8;
2647 match[14] |= (cos1_val & 0x7) << 5;
2653 match[18] |= (cos2_val & 0x7) << 5;
2655 match[14] |= (cos1_val & 0x7) << 5;
2658 match[13] = proto_val & 0xff;
2659 match[12] = proto_val >> 8;
2671 u8 **matchp = va_arg (*args,
u8 **);
2672 u32 table_index = va_arg (*args,
u32);
2707 if (match || l2 || l3 || l4)
2747 const u8 *match,
u32 hit_next_index,
2749 u16 metadata,
int is_add)
2752 vnet_classify_entry_5_t _max_e __attribute__ ((aligned (16)));
2757 return VNET_API_ERROR_NO_SUCH_TABLE;
2762 e->next_index = hit_next_index;
2763 e->opaque_index = opaque_index;
2764 e->advance = advance;
2778 e->metadata = metadata;
2795 return VNET_API_ERROR_NO_SUCH_ENTRY;
2806 u32 table_index = ~0;
2807 u32 hit_next_index = ~0;
2808 u64 opaque_index = ~0;
2835 else if (
unformat (input,
"policer-hit-next %U",
2838 else if (
unformat (input,
"opaque-index %lld", &opaque_index))
2841 cm, &match, table_index))
2843 else if (
unformat (input,
"advance %d", &advance))
2845 else if (
unformat (input,
"table-index %d", &table_index))
2847 else if (
unformat (input,
"action set-ip4-fib-id %d", &metadata))
2849 else if (
unformat (input,
"action set-ip6-fib-id %d", &metadata))
2851 else if (
unformat (input,
"action set-sr-policy-index %d", &metadata))
2856 for (
i = 0;
i <
vec_len (
cm->unformat_opaque_index_fns);
i++)
2858 if (
unformat (input,
"%U",
cm->unformat_opaque_index_fns[
i],
2868 if (table_index == ~0)
2871 if (is_add && match == 0)
2876 opaque_index, advance,
2877 action, metadata, is_add);
2886 "vnet_classify_add_del_session returned %d",
2895 .path =
"classify session",
2897 "classify session [hit-next|l2-input-hit-next|l2-output-hit-next|"
2898 "acl-hit-next <next_index>|policer-hit-next <policer_name>]"
2899 "\n table-index <nn> match [hex] [l2] [l3 ip4] [opaque-index <index>]"
2900 "\n [action set-ip4-fib-id|set-ip6-fib-id|set-sr-policy-index <n>] [del]",
2908 u64 *opaquep = va_arg (*args,
u64 *);
2924 u32 *next_indexp = va_arg (*args,
u32 *);
2951 u32 *next_indexp = va_arg (*args,
u32 *);
2978 u32 *next_indexp = va_arg (*args,
u32 *);
2998 u32 *next_indexp = va_arg (*args,
u32 *);
3077 classify_data_or_mask_t *
mask;
3078 classify_data_or_mask_t *
data;
3084 } test_classify_main_t;
3086 static test_classify_main_t test_classify_main;
3089 test_classify_churn (test_classify_main_t * tm)
3091 classify_data_or_mask_t *
mask, *
data;
3094 u8 *mp = 0, *dp = 0;
3101 mask = (classify_data_or_mask_t *) mp;
3102 data = (classify_data_or_mask_t *) dp;
3107 tmp = clib_host_to_net_u32 (tm->src.as_u32);
3109 for (
i = 0;
i < tm->sessions;
i++)
3112 ep->addr.as_u32 = clib_host_to_net_u32 (
tmp);
3120 tm->memory_size, 0 ,
3123 tm->table_index = tm->table - tm->classify_main->tables;
3125 tm->table_index, tm->buckets);
3128 tm->sessions / 2, tm->sessions);
3130 for (
i = 0;
i < tm->sessions / 2;
i++)
3134 data->ip.src_address.as_u32 = ep->addr.as_u32;
3157 for (
i = 0;
i < tm->iterations;
i++)
3166 data->ip.src_address.as_u32 = ep->addr.as_u32;
3169 is_add = !ep->in_table;
3173 is_add ?
"add" :
"del",
3187 "%s[%d]: %U returned %d", is_add ?
"add" :
"del",
3190 ep->in_table = is_add;
3194 tm->table->active_elements);
3196 for (
i = 0;
i < tm->sessions;
i++)
3202 ep = tm->entries +
i;
3203 if (ep->in_table == 0)
3206 data->ip.src_address.as_u32 = ep->addr.as_u32;
3214 clib_warning (
"Couldn't find %U index %d which should be present",
3219 key_minus_skip = (
u8 *) e->key;
3220 key_minus_skip -= tm->table->skip_n_vectors * sizeof (
u32x4);
3237 tm->table->active_elements);
3246 tm->table_index, 1 );
3248 tm->table_index = ~0;
3258 test_classify_main_t *tm = &test_classify_main;
3265 tm->sessions = 8192;
3266 tm->iterations = 8192;
3267 tm->memory_size = 64 << 20;
3268 tm->src.as_u32 = clib_net_to_host_u32 (0x0100000A);
3270 tm->seed = 0xDEADDABE;
3271 tm->classify_main =
cm;
3284 else if (
unformat (input,
"buckets %d", &tm->buckets))
3286 else if (
unformat (input,
"memory-size %uM", &
tmp))
3287 tm->memory_size =
tmp << 20;
3288 else if (
unformat (input,
"memory-size %uG", &
tmp))
3289 tm->memory_size =
tmp << 30;
3290 else if (
unformat (input,
"seed %d", &tm->seed))
3292 else if (
unformat (input,
"verbose"))
3295 else if (
unformat (input,
"iterations %d", &tm->iterations))
3297 else if (
unformat (input,
"churn-test"))
3306 error = test_classify_churn (tm);
3318 .path =
"test classify",
3320 "test classify [src <ip>] [sessions <nn>] [buckets <nn>] [seed <nnn>]\n"
3321 " [memory-size <nn>[M|G]]\n"
3323 .function = test_classify_command_fn,
#define vec_reset_length(v)
Reset vector length to zero NULL-pointer tolerant.
static void clib_spinlock_init(clib_spinlock_t *p)
uword unformat_l2_mask(unformat_input_t *input, va_list *args)
static void vnet_classify_entry_free(vnet_classify_table_t *t, vnet_classify_entry_t *v, u32 log2_pages)
static u64 vnet_classify_hash_packet_inline(vnet_classify_table_t *t, const u8 *h)
void vnet_classify_register_unformat_policer_next_index_fn(unformat_function_t *fn)
#define foreach_udp_proto_field
u32 classify_get_pcap_chain(vnet_classify_main_t *cm, u32 sw_if_index)
vlib_node_registration_t l2_input_classify_node
(constructor) VLIB_REGISTER_NODE (l2_input_classify_node)
uword unformat_ethernet_address(unformat_input_t *input, va_list *args)
struct _vnet_classify_entry vnet_classify_entry_t
int vnet_classify_add_del_session(vnet_classify_main_t *cm, u32 table_index, const u8 *match, u32 hit_next_index, u32 opaque_index, i32 advance, u8 action, u16 metadata, int is_add)
vnet_classify_bucket_t saved_bucket
vl_api_ip_port_and_mask_t dst_port
static uword unformat_l2_input_next_node(unformat_input_t *input, va_list *args)
int vnet_is_packet_traced(vlib_buffer_t *b, u32 classify_table_index, int func)
static int vnet_classify_entry_is_free(vnet_classify_entry_t *e)
static int vnet_classify_add_del(vnet_classify_table_t *t, vnet_classify_entry_t *add_v, int is_add)
static uword vlib_node_add_next(vlib_main_t *vm, uword node, uword next_node)
vlib_node_registration_t ip6_classify_node
(constructor) VLIB_REGISTER_NODE (ip6_classify_node)
nat44_ei_hairpin_src_next_t next_index
#define pool_get_aligned_zero(P, E, A)
Allocate an object E from a pool P with alignment A and zero it.
static void vnet_classify_entry_release_resource(vnet_classify_entry_t *e)
#define pool_elt_at_index(p, i)
Returns pointer to element at given index.
u32 classify_lookup_chain(u32 table_index, u8 *mask, u32 n_skip, u32 n_match)
struct _tcp_header tcp_header_t
typedef CLIB_PACKED(struct { ethernet_header_t eh;ip4_header_t ip;})
uword unformat_ip4_match(unformat_input_t *input, va_list *args)
static int filter_table_mask_compare(void *a1, void *a2)
static void clib_mem_free(void *p)
vnet_classify_main_t vnet_classify_main
#define clib_error_return(e, args...)
u8 * format_clib_mem_heap(u8 *s, va_list *va)
#define CLIB_SPINLOCK_ASSERT_LOCKED(_p)
static vnet_classify_entry_t * vnet_classify_entry_at_index(vnet_classify_table_t *t, vnet_classify_entry_t *e, u32 index)
#define vec_append(v1, v2)
Append v2 after v1.
static clib_error_t * classify_session_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
vnet_classify_table_t * vnet_classify_new_table(vnet_classify_main_t *cm, const u8 *mask, u32 nbuckets, u32 memory_size, u32 skip_n_vectors, u32 match_n_vectors)
static vnet_classify_entry_t * vnet_classify_get_entry(vnet_classify_table_t *t, uword offset)
uword unformat_acl_next_index(unformat_input_t *input, va_list *args)
static vlib_cli_command_t classify_session_command
(constructor) VLIB_CLI_COMMAND (classify_session_command)
#define pool_put(P, E)
Free an object E in pool P.
void vnet_classify_register_unformat_acl_next_index_fn(unformat_function_t *fn)
vlib_main_t * vm
X-connect all packets from the HOST to the PHY.
#define vec_delete(V, N, M)
Delete N elements starting at element M.
void vnet_classify_delete_table_index(vnet_classify_main_t *cm, u32 table_index, int del_chain)
#define foreach_ip6_proto_field
static_always_inline void * clib_memcpy_fast(void *restrict dst, const void *restrict src, size_t n)
vnet_classify_entry_t * vnet_classify_find_entry(vnet_classify_table_t *t, u8 *h, u64 hash, f64 now)
static vlib_cli_command_t classify_filter
(constructor) VLIB_CLI_COMMAND (classify_filter)
uword unformat_l2_input_next_index(unformat_input_t *input, va_list *args)
vlib_trace_filter_t trace_filter
static u32 random_u32(u32 *seed)
32-bit random number generator
void vnet_classify_register_unformat_opaque_index_fn(unformat_function_t *fn)
static uword max_log2(uword x)
static uword unformat_l2_output_next_node(unformat_input_t *input, va_list *args)
#define pool_is_free_index(P, I)
Use free bitmap to query whether given index is free.
static vnet_classify_entry_t * split_and_rehash_linear(vnet_classify_table_t *t, vnet_classify_entry_t *old_values, u32 old_log2_pages, u32 new_log2_pages)
vlib_node_registration_t ip4_classify_node
(constructor) VLIB_REGISTER_NODE (ip4_classify_node)
#define pool_foreach(VAR, POOL)
Iterate through pool.
int vnet_classify_add_del_table(vnet_classify_main_t *cm, const u8 *mask, u32 nbuckets, u32 memory_size, u32 skip, u32 match, u32 next_table_index, u32 miss_next_index, u32 *table_index, u8 current_data_flag, i16 current_data_offset, int is_add, int del_chain)
#define foreach_l2_output_next
static_always_inline void clib_memset_u32(void *p, u32 val, uword count)
static void vnet_classify_entry_claim_resource(vnet_classify_entry_t *e)
clib_mem_heap_t * clib_mem_create_heap(void *base, uword size, int is_locked, char *fmt,...)
void rogue(vnet_classify_table_t *t)
#define vec_len(v)
Number of elements in vector (rvalue-only, NULL tolerant)
@ IP_LOOKUP_NEXT_DROP
Adjacency to drop this packet.
uword unformat_ethernet_type_host_byte_order(unformat_input_t *input, va_list *args)
#define vec_add2(V, P, N)
Add N elements to end of vector V, return pointer to new elements in P.
#define vec_add1(V, E)
Add 1 element to end of vector (unspecified alignment).
static int vnet_classify_entry_is_busy(vnet_classify_entry_t *e)
int * working_copy_lengths
#define vec_elt_at_index(v, i)
Get vector value at index i checking that i is in bounds.
vnet_main_t * vnet_get_main(void)
void mv(vnet_classify_table_t *t)
static clib_error_t * vnet_classify_init(vlib_main_t *vm)
u32 fib_table_find_or_create_and_lock(fib_protocol_t proto, u32 table_id, fib_source_t src)
Get the index of the FIB for a Table-ID.
static_always_inline uword vlib_get_thread_index(void)
void classify_set_pcap_chain(vnet_classify_main_t *cm, u32 sw_if_index, u32 table_index)
unformat_function_t unformat_vlib_node
uword unformat_l3_match(unformat_input_t *input, va_list *args)
vlib_node_registration_t ip4_inacl_node
(constructor) VLIB_REGISTER_NODE (ip4_inacl_node)
vlib_global_main_t vlib_global_main
#define vec_validate_aligned(V, I, A)
Make sure vector is long enough for given index (no header, specified alignment)
static_always_inline void clib_spinlock_lock(clib_spinlock_t *p)
uword unformat_udp_mask(unformat_input_t *input, va_list *args)
void vnet_classify_register_unformat_ip_next_index_fn(unformat_function_t *fn)
void clib_mem_destroy_heap(clib_mem_heap_t *heap)
uword unformat_vlan_tag(unformat_input_t *input, va_list *args)
uword unformat_l3_mask(unformat_input_t *input, va_list *args)
vnet_classify_entry_t ** freelists
static clib_error_t * classify_table_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
uword unformat_ip6_match(unformat_input_t *input, va_list *args)
#define vec_append_aligned(v1, v2, align)
Append v2 after v1.
vnet_classify_bucket_t * buckets
#define VNET_CLASSIFY_ENTRY_FREE
vl_api_ip_port_and_mask_t src_port
uword unformat_classify_mask(unformat_input_t *input, va_list *args)
uword unformat_l2_output_next_index(unformat_input_t *input, va_list *args)
vnet_feature_config_main_t * cm
uword unformat_l4_mask(unformat_input_t *input, va_list *args)
vnet_classify_entry_t ** working_copies
@ FIB_SOURCE_CLASSIFY
Classify.
#define vec_validate(V, I)
Make sure vector is long enough for given index (no header, unspecified alignment)
#define VLIB_CLI_COMMAND(x,...)
#define foreach_l2_input_next
static uword unformat_ip_next_node(unformat_input_t *input, va_list *args)
#define CLIB_MEMORY_BARRIER()
@ CLASSIFY_ACTION_SET_METADATA
#define CLIB_CACHE_LINE_BYTES
u8 * format_vnet_classify_table(u8 *s, va_list *args)
static uword unformat_opaque_sw_if_index(unformat_input_t *input, va_list *args)
void vlib_cli_output(vlib_main_t *vm, char *fmt,...)
@ CLASSIFY_ACTION_SET_IP6_FIB_INDEX
static uword count_set_bits(uword x)
#define vec_free(V)
Free vector's memory (no header).
format_function_t format_vnet_sw_if_index_name
static uword unformat_acl_next_node(unformat_input_t *input, va_list *args)
static vnet_classify_entry_t * split_and_rehash(vnet_classify_table_t *t, vnet_classify_entry_t *old_values, u32 old_log2_pages, u32 new_log2_pages)
unformat_function_t unformat_vnet_sw_interface
vlib_node_registration_t ip6_inacl_node
(constructor) VLIB_REGISTER_NODE (ip6_inacl_node)
description fragment has unexpected format
vlib_node_registration_t l2_output_classify_node
(constructor) VLIB_REGISTER_NODE (l2_output_classify_node)
#define vec_validate_init_empty(V, I, INIT)
Make sure vector is long enough for given index and initialize empty space (no header,...
void fib_table_lock(u32 fib_index, fib_protocol_t proto, fib_source_t source)
Release a reference counting lock on the table.
#define VLIB_INIT_FUNCTION(x)
static vnet_hw_interface_t * vnet_get_sup_hw_interface(vnet_main_t *vnm, u32 sw_if_index)
uword unformat_l2_match(unformat_input_t *input, va_list *args)
vl_api_ip_proto_t protocol
static void make_working_copy(vnet_classify_table_t *t, vnet_classify_bucket_t *b)
int vlib_enable_disable_pkt_trace_filter(int enable)
Enable / disable packet trace filter.
for(i=1;i<=collision_buckets;i++)
struct _vnet_classify_main vnet_classify_main_t
static vnet_classify_entry_t * vnet_classify_find_entry_inline(vnet_classify_table_t *t, const u8 *h, u64 hash, f64 now)
@ CLASSIFY_ACTION_SET_IP4_FIB_INDEX
static_always_inline void clib_spinlock_unlock(clib_spinlock_t *p)
u64 vnet_classify_hash_packet(vnet_classify_table_t *t, u8 *h)
uword unformat_classify_match(unformat_input_t *input, va_list *args)
static uword vnet_classify_get_offset(vnet_classify_table_t *t, vnet_classify_entry_t *v)
#define vec_sort_with_function(vec, f)
Sort a vector using the supplied element comparison function.
clib_memset(h->entries, 0, sizeof(h->entries[0]) *entries)
static int vnet_is_packet_traced_inline(vlib_buffer_t *b, u32 classify_table_index, int func)
vnet_is_packet_traced
static vnet_classify_entry_t * vnet_classify_entry_alloc(vnet_classify_table_t *t, u32 log2_pages)
u32 classify_sort_table_chain(vnet_classify_main_t *cm, u32 table_index)
clib_error_t *() vlib_init_function_t(struct vlib_main_t *vm)
clib_spinlock_t writer_lock
uword unformat_l4_match(unformat_input_t *input, va_list *args)
void fib_table_unlock(u32 fib_index, fib_protocol_t proto, fib_source_t source)
Take a reference counting lock on the table.
u32 classify_get_trace_chain(void)
uword unformat_tcp_mask(unformat_input_t *input, va_list *args)
#define clib_warning(format, args...)
static clib_error_t * classify_filter_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
void classify_set_trace_chain(vnet_classify_main_t *cm, u32 table_index)
static void * clib_mem_alloc_aligned(uword size, uword align)
int vlib_main(vlib_main_t *volatile vm, unformat_input_t *input)
vnet_classify_flags_t current_data_flag
uword unformat_ip_next_index(unformat_input_t *input, va_list *args)
static vlib_cli_command_t show_classify_filter
(constructor) VLIB_CLI_COMMAND (show_classify_filter)
static vlib_cli_command_t show_classify_table_command
(constructor) VLIB_CLI_COMMAND (show_classify_table_command)
static clib_error_t * show_classify_tables_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
vl_api_address_union_t src_address
u8 * format_classify_table(u8 *s, va_list *args)
vl_api_mac_event_action_t action
vl_api_interface_index_t sw_if_index
#define foreach_tcp_proto_field
static u8 * format_classify_entry(u8 *s, va_list *args)
void vnet_classify_register_unformat_l2_next_index_fn(unformat_function_t *fn)
static clib_mem_heap_t * clib_mem_set_heap(clib_mem_heap_t *heap)
static clib_error_t * show_classify_filter_command_fn(vlib_main_t *vm, unformat_input_t *input, vlib_cli_command_t *cmd)
uword unformat_ip4_mask(unformat_input_t *input, va_list *args)
uword unformat_ip6_mask(unformat_input_t *input, va_list *args)
#define foreach_ip4_proto_field
uword unformat_policer_next_index(unformat_input_t *input, va_list *args)
VLIB buffer representation.
static vlib_cli_command_t classify_table
(constructor) VLIB_CLI_COMMAND (classify_table)